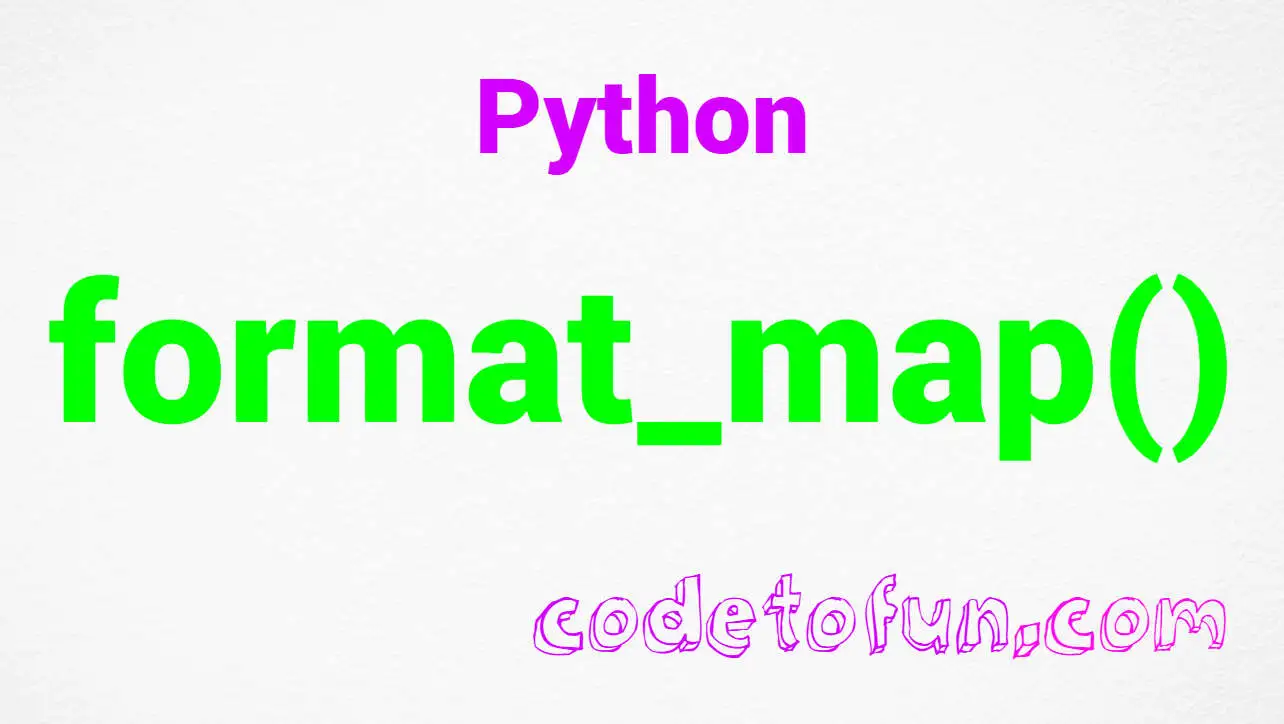
Python Basic
Python String Methods
Python string format_map() Method
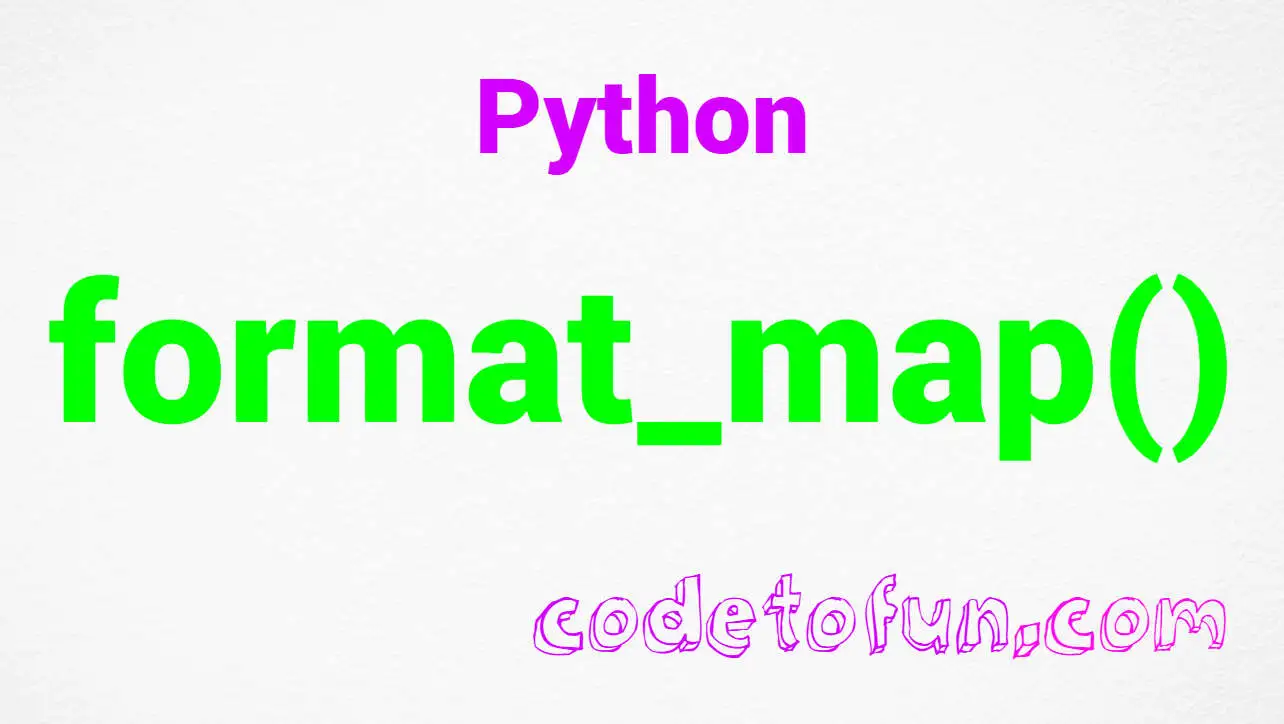
Photo Credit to CodeToFun
đ Introduction
In Python, the format_map()
method is a powerful tool for formatting strings by substituting placeholders with values from a provided dictionary.
This method is an extension of the format() method and provides a convenient way to perform string formatting with the help of a mapping.
In this tutorial, we'll delve into the syntax, usage, and some practical examples of the format_map()
method.
đĄ Syntax
The syntax for the format_map()
method is as follows:
string.format_map(mapping)
- string: The string containing placeholders to be replaced.
- mapping: A dictionary containing key-value pairs for substitution.
đ Example 1
Let's explore the usage of the format_map()
method with a example.
# Using format_map() with a dictionary
data = {'name': 'John', 'age': 25}
template = "Hello, my name is {name} and I am {age} years old."
formatted_string = template.format_map(data)
print("Example 1:", formatted_string)
đģ Testing the Program
Example 1: Hello, my name is John and I am 25 years old.
đ§ How the Program Works
In Example 1, the format_map()
method is used to substitute placeholders in the template string with values from the data dictionary, resulting in the formatted string "Hello, my name is John and I am 25 years old."
đ Example 2
Let's explore the usage of the format_map()
method with another example.
# Using format_map() with an object
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
data_object = Person(name='Alice', age=30)
template = "This is {name}. {name} is {age} years old."
formatted_string = template.format_map(vars(data_object))
print("Example 2:", formatted_string)
đģ Testing the Program
Example 2: Hello, my name is John and I am 25 years old.
đ§ How the Program Works
In Example 2, an object of the Person class is created, and its attributes are used with format_map()
to generate the string This is Alice. Alice is 30 years old.
âŠī¸ Return Value
The format_map()
method returns a new string with placeholders replaced by the values from the provided mapping.
đ Common Use Cases
The format_map()
method is particularly useful when you have a dynamic set of values to substitute into a string and want to avoid hardcoding them in the format string. It provides a clean and concise way to perform string formatting with the flexibility of dictionaries or objects.
đ Notes
- If a placeholder in the string is not present in the mapping, the method does not raise an error. Instead, the placeholder remains unchanged in the resulting string.
đĸ Optimization
The format_map()
method is optimized for efficient string formatting. However, for large-scale formatting operations, consider using this method judiciously and optimizing other aspects of your code for better performance.
đ Conclusion
The format_map()
method in Python is a valuable addition to the string formatting arsenal. It allows for dynamic substitution of placeholders using dictionaries or objects, providing a flexible and efficient way to generate formatted strings.
Feel free to experiment with different mappings and templates to deepen your understanding of the format_map()
method. Happy coding!
đ¨âđģ Join our Community:
Author
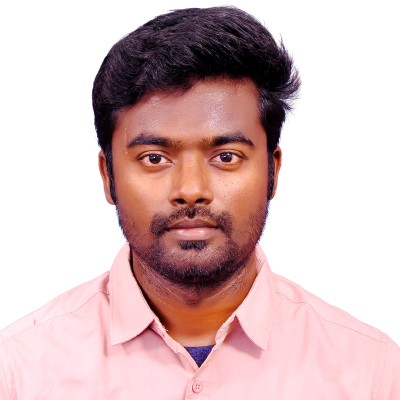
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (Python string format_map() Method), please comment here. I will help you immediately.