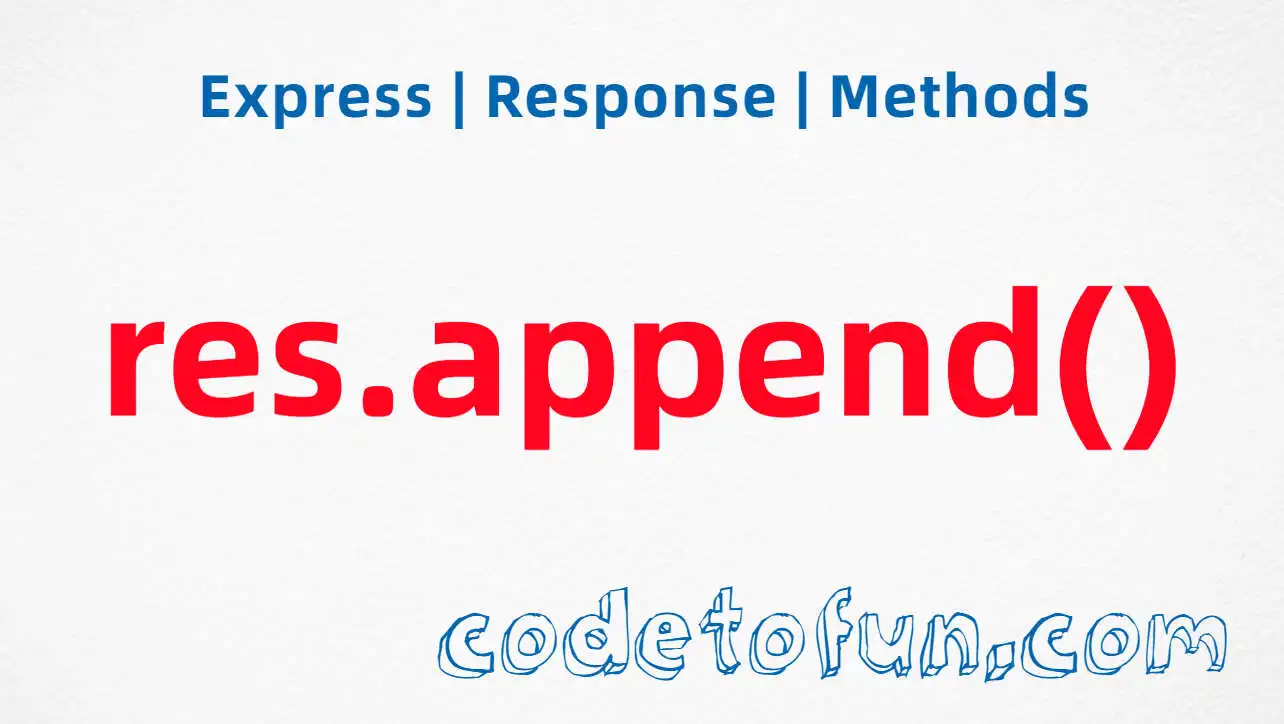
Express.js Basic
- express Intro
- express express()
- express Application
Properties
Events
Methods
- express Request
- express Response
- express Router
Express app.use() Method
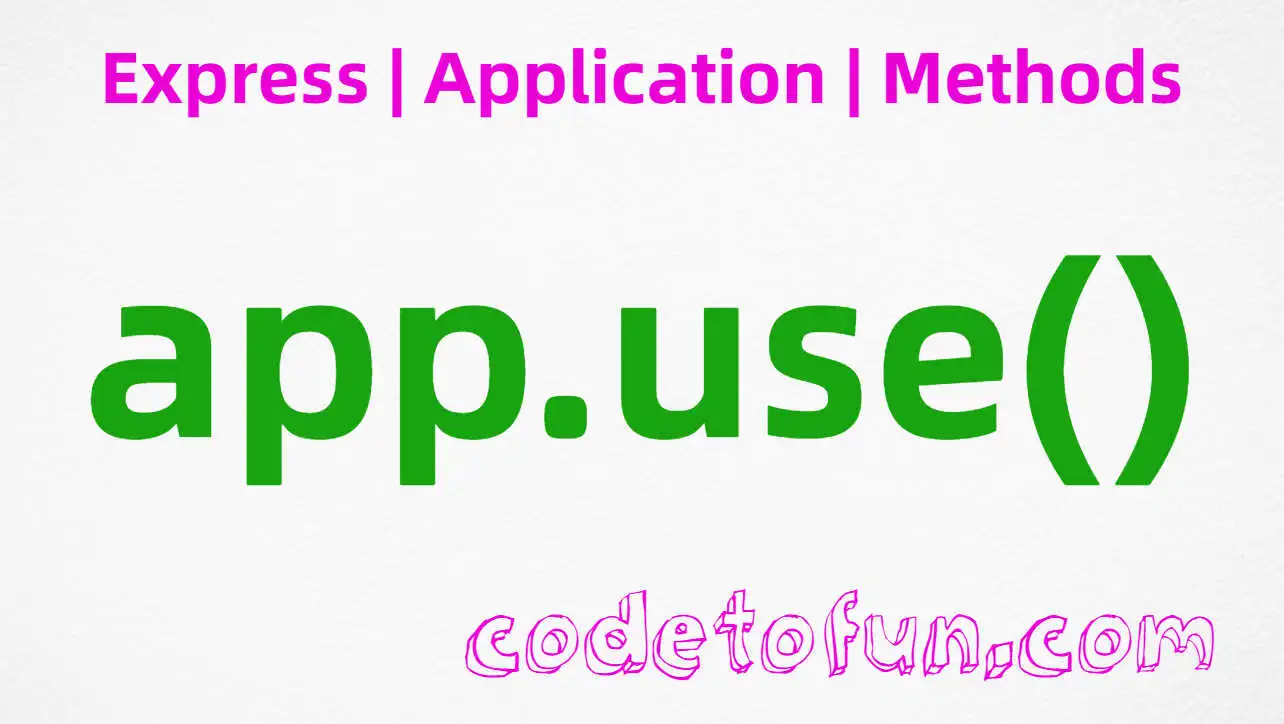
Photo Credit to CodeToFun
🙋 Introduction
Middleware plays a pivotal role in Express.js, allowing developers to intercept and modify the request and response objects. One of the key methods for implementing middleware is app.use()
in Express.js.
In this guide, we'll explore the syntax, applications, and best practices of the app.use()
method.
💡 Syntax
The syntax for the app.use()
method is flexible, allowing you to mount middleware at a specified path or use it without a path to apply it to all routes:
// Applying middleware to all routes
app.use(middlewareFunction);
// Applying middleware to a specific path
app.use('/path', middlewareFunction);
- middlewareFunction: The function that will be executed when the middleware is called.
❓ How app.use() Works
The app.use()
method is used to bind application-level middleware to your Express application. Middleware functions have access to the request object (req), the response object (res), and the next function in the application's request-response cycle.
// Example of a simple middleware function
const loggerMiddleware = (req, res, next) => {
console.log(`Request received for path: ${req.path}`);
next();
};
// Applying the middleware to all routes
app.use(loggerMiddleware);
In this example, the loggerMiddleware function is applied to all routes, logging information about the incoming request.
📚 Use Cases
Logging:
Use
app.use()
for logging information about each incoming request, aiding in debugging and monitoring.example.jsCopied// Middleware for logging requests const requestLogger = (req, res, next) => { console.log(`[${new Date().toISOString()}] ${req.method} ${req.url}`); next(); }; // Applying the logging middleware to all routes app.use(requestLogger);
Authentication:
Leverage
app.use()
to enforce authentication for specific routes, ensuring secure access.example.jsCopied// Middleware for user authentication const authenticateUser = (req, res, next) => { // Implement your authentication logic here if (req.isAuthenticated()) { return next(); } else { res.status(401).send('Unauthorized'); } }; // Applying authentication middleware to a specific route app.use('/secure', authenticateUser);
🏆 Best Practices
Order Matters:
The order in which you apply middleware with
app.use()
is crucial. Middlewares are executed in the order they are defined, so be mindful of the sequence.example.jsCopiedapp.use(middleware1); app.use(middleware2);
In this example, middleware1 will be executed before middleware2.
Modularize Middleware:
Break down complex middleware into smaller, modular functions. This promotes reusability and maintains a clean codebase.
example.jsCopied// Middleware for handling CORS headers const handleCors = (req, res, next) => { // Implement CORS logic here next(); }; // Middleware for logging requests const requestLogger = (req, res, next) => { console.log(`[${new Date().toISOString()}] ${req.method} ${req.url}`); next(); }; // Applying multiple middlewares app.use(handleCors); app.use(requestLogger);
Use Error Handling Middleware:
When using asynchronous operations in your middleware, include error handling. Use next(err) to pass errors to the next error-handling middleware.
example.jsCopied// Error handling middleware const errorHandler = (err, req, res, next) => { console.error(err.stack); res.status(500).send('Something went wrong!'); }; // Applying error handling middleware app.use(errorHandler);
🎉 Conclusion
The app.use()
method in Express.js is a versatile tool for incorporating middleware into your application. Whether you're logging requests, implementing authentication, or handling errors, understanding how to use and organize middleware with app.use()
is fundamental for building robust Express.js applications.
Now armed with knowledge about the app.use()
method, go ahead and enhance your Express.js projects with powerful middleware functionality!
👨💻 Join our Community:
Author
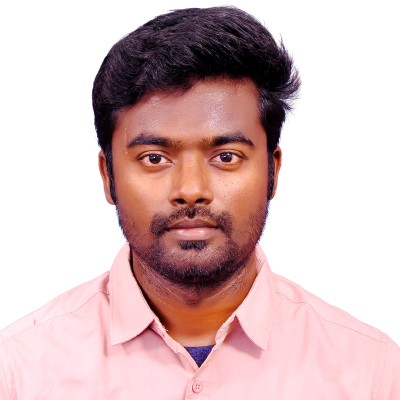
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (Express app.use() Method), please comment here. I will help you immediately.