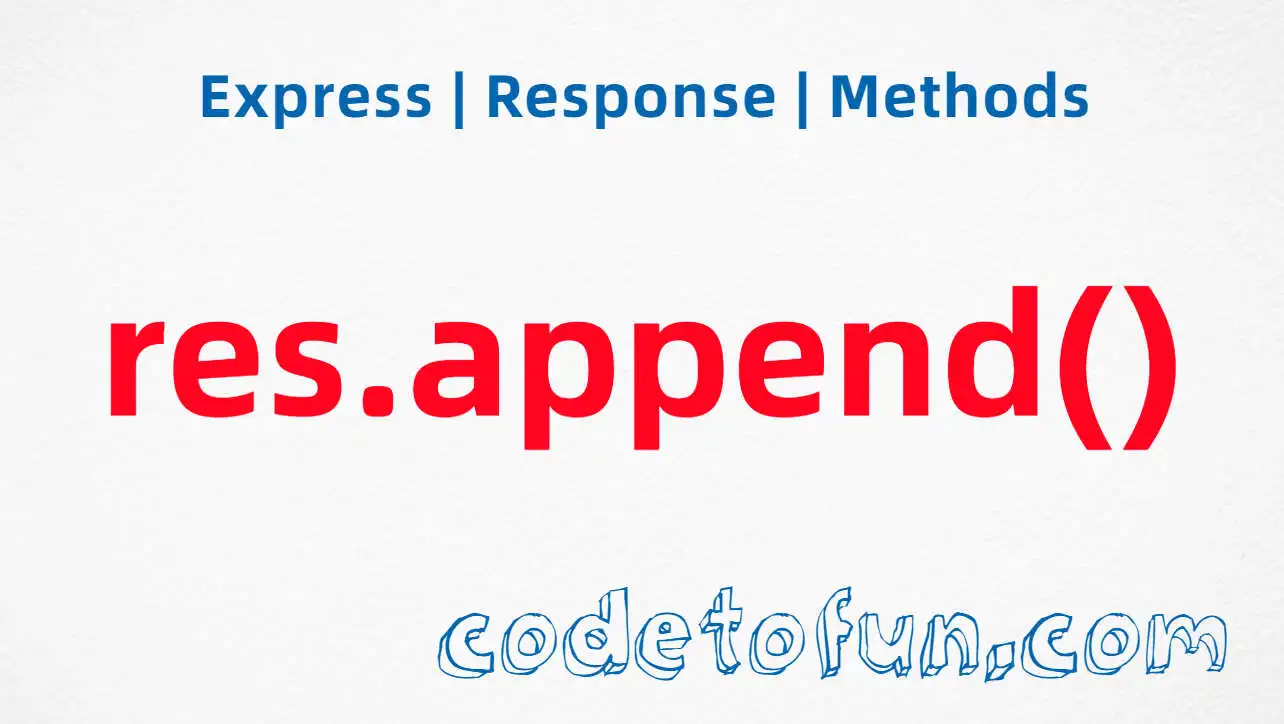
Express.js Basic
- express Intro
- express express()
- express Application
Properties
Events
Methods
- express Request
- express Response
- express Router
Express app.route() Method
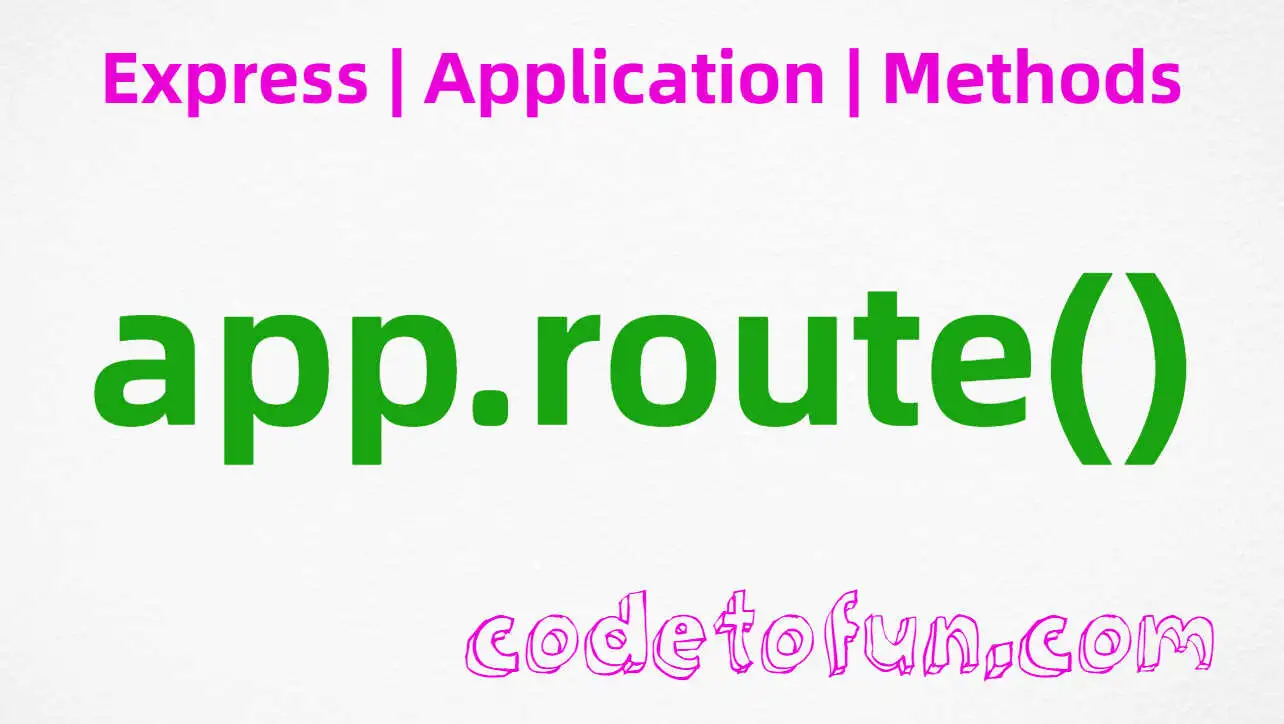
Photo Credit to CodeToFun
🙋 Introduction
In the world of Express.js, the app.route()
method stands out as a powerful tool for simplifying the creation of route handlers. This method allows you to define a chain of route handlers for a specific route path.
In this guide, we'll explore the syntax, use cases, and best practices of the app.route()
method to streamline your route handling in Express.js.
💡 Syntax
The syntax for the app.route()
method is straightforward:
const myRoute = app.route('/path');
The app.route()
method returns a route object that you can use to define HTTP methods and their respective handlers for the specified path.
🛣️ Simplifying Route Handling
With app.route()
, you can define route-specific logic more efficiently by chaining HTTP methods for a particular path.
const express = require('express');
const app = express();
const userRoute = app.route('/users');
userRoute
.get((req, res) => {
// Logic for handling GET requests to /users
res.send('Get users');
})
.post((req, res) => {
// Logic for handling POST requests to /users
res.send('Create a new user');
})
.put((req, res) => {
// Logic for handling PUT requests to /users
res.send('Update users');
})
.delete((req, res) => {
// Logic for handling DELETE requests to /users
res.send('Delete users');
});
In this example, we create a route for handling various HTTP methods on the /users path, making the code more organized and readable.
📚 Use Cases
Organizing Routes:
Use
app.route()
to organize routes for a specific path, making your codebase more modular and maintainable.example.jsCopiedconst express = require('express'); const app = express(); const adminRoute = app.route('/admin'); adminRoute .get((req, res) => { // Logic for handling GET requests to /admin res.send('Admin dashboard'); }) .post((req, res) => { // Logic for handling POST requests to /admin res.send('Create admin'); }) .put((req, res) => { // Logic for handling PUT requests to /admin res.send('Update admin'); }) .delete((req, res) => { // Logic for handling DELETE requests to /admin res.send('Delete admin'); });
Middleware Integration:
Integrate middleware functions seamlessly using
app.route()
to apply middleware to multiple HTTP methods on a specific route.example.jsCopiedconst express = require('express'); const app = express(); const apiRoute = app.route('/api'); apiRoute .all((req, res, next) => { // Middleware logic for all HTTP methods on /api console.log('Middleware for /api'); next(); }) .get((req, res) => { // Logic for handling GET requests to /api res.send('Get data from API'); }) .post((req, res) => { // Logic for handling POST requests to /api res.send('Add data to API'); });
🏆 Best Practices
Chain Methods in Logical Order:
Chain the HTTP methods in a logical order to improve code readability. For example, place get() before post() for better organization.
Reusability:
Consider using
app.route()
for paths that share common route logic, promoting code reusability and maintainability.Middleware Placement:
Place middleware functions using all() at the beginning of the chain to ensure they apply to all subsequent HTTP methods.
🎉 Conclusion
The app.route()
method in Express.js provides a concise and organized way to handle routes for a specific path. By chaining HTTP methods, you can create modular and maintainable route handlers, making your Express.js application more scalable.
Now, armed with knowledge about the app.route()
method, go ahead and streamline your route handling in Express.js!
👨💻 Join our Community:
Author
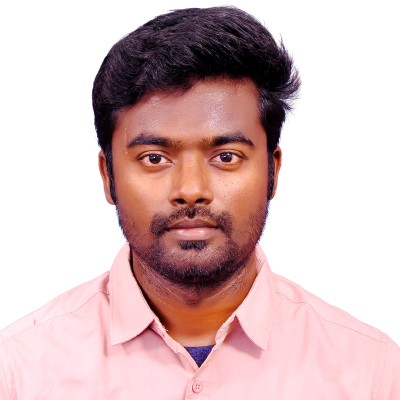
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (Express app.route() Method), please comment here. I will help you immediately.