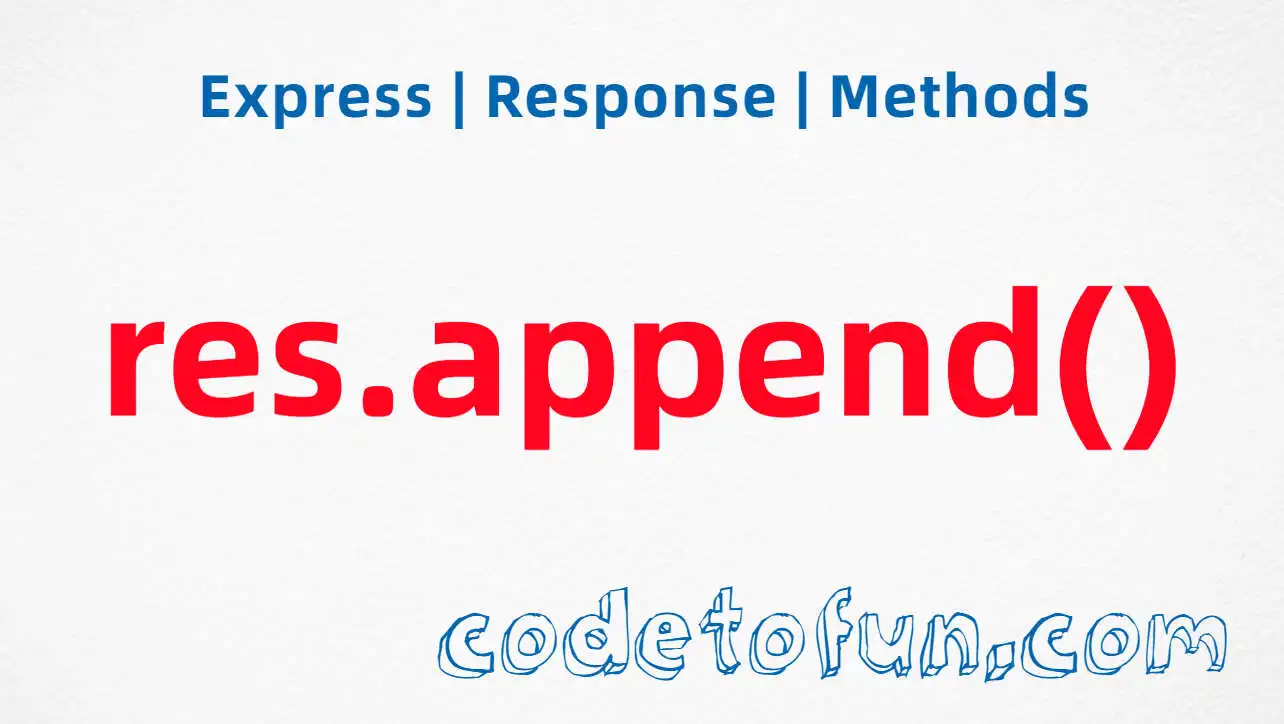
Express.js Basic
- express Intro
- express express()
- express Application
Properties
Events
Methods
- express Request
- express Response
- express Router
Express app.put() Method
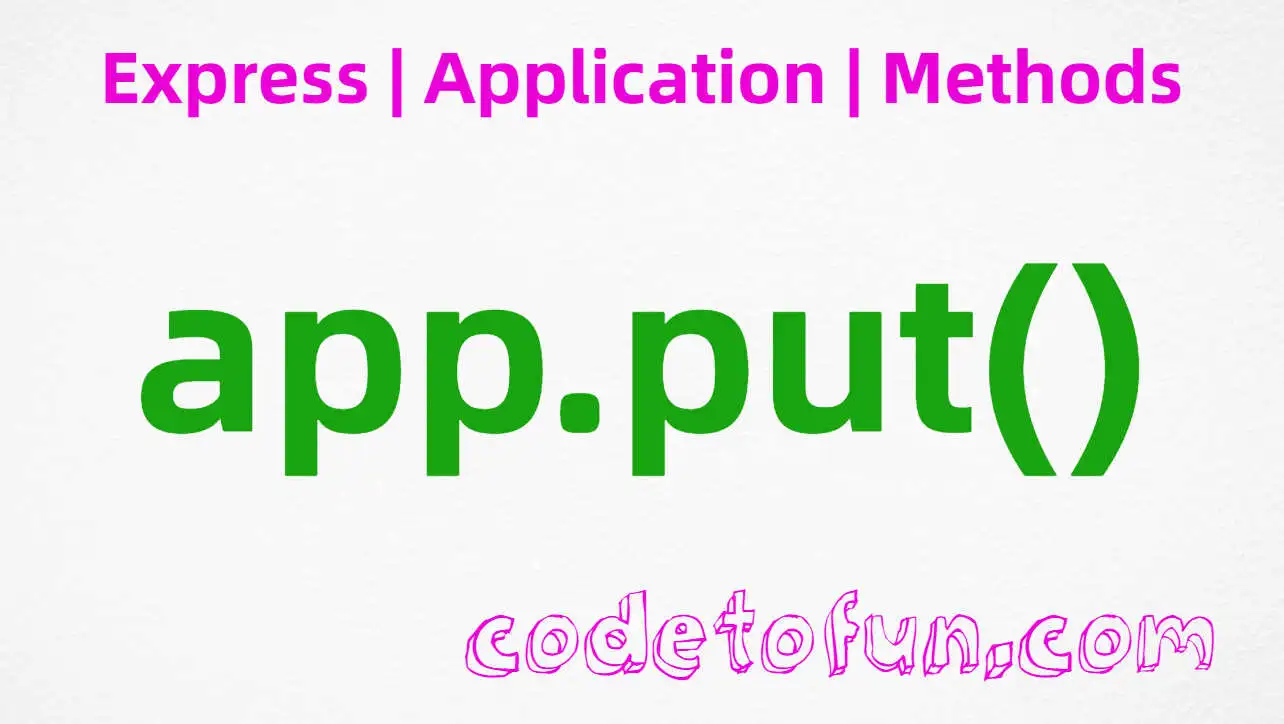
Photo Credit to CodeToFun
🙋 Introduction
In the dynamic landscape of web development, handling various HTTP methods is crucial for building effective and scalable applications. Express.js, a popular web application framework for Node.js, equips developers with specialized methods for different HTTP verbs.
This guide focuses on the app.put()
method, designed to handle HTTP PUT requests.
💡 Syntax
The syntax for the app.put()
method aligns with other route methods in Express.js:
app.put(path, callback)
- path: A string representing the route or path for which the PUT request is being handled.
- callback: The route handler function responsible for processing the PUT request.
❓ How app.put() Works
The app.put()
method enables developers to define route-specific logic to handle HTTP PUT requests. This proves invaluable when updating or modifying resources on the server.
app.put('/users/:id', (req, res) => {
const userId = req.params.id;
// Logic to update user with userId
res.send(`Updated user with ID ${userId}`);
});
In this example, the route /users/:id captures PUT requests and executes logic to update a user with the specified ID.
📚 Use Cases
Resource Modification:
Utilize
app.put()
to handle requests for modifying specific resources, such as updating posts or user details.example.jsCopiedapp.put('/posts/:postId', (req, res) => { const postId = req.params.postId; // Logic to update post with postId res.send(`Updated post with ID ${postId}`); });
Database Record Update:
Implement
app.put()
for routes dedicated to updating database records, ensuring accurate and up-to-date information.example.jsCopiedapp.put('/products/:productId', (req, res) => { const productId = req.params.productId; // Logic to update product details in the database res.send(`Updated product with ID ${productId}`); });
🏆 Best Practices
RESTful Route Design:
Follow RESTful principles when designing your routes. Utilize
app.put()
for routes involving resource updates.example.jsCopied// RESTful route for updating a user app.put('/users/:id', (req, res) => { const userId = req.params.id; // Logic to update user with userId res.send(`Updated user with ID ${userId}`); });
Error Handling for Robustness:
Implement robust error handling within your
app.put()
route handlers to gracefully manage potential issues.example.jsCopiedapp.put('/posts/:postId', (req, res) => { const postId = req.params.postId; // Simulate an error during the update if (Math.random() < 0.5) { res.status(500).send('Internal Server Error'); } else { // Logic to update post with postId res.send(`Updated post with ID ${postId}`); } });
Middleware Integration:
Combine
app.put()
with middleware functions for tasks like authentication or authorization before processing the update operation.example.jsCopied// Middleware for user authentication const authenticateUser = (req, res, next) => { // Implement your authentication logic here if (req.isAuthenticated()) { return next(); } else { res.status(401).send('Unauthorized'); } }; // Apply authentication middleware to the put route app.put('/users/:id', authenticateUser, (req, res) => { const userId = req.params.id; // Logic to update user with userId res.send(`Updated user with ID ${userId}`); });
🎉 Conclusion
The app.put()
method in Express.js provides a powerful tool for handling HTTP PUT requests efficiently. Whether you're managing resource modifications or updating database records, understanding how to leverage app.put()
is essential for building robust and maintainable applications.
Now, equipped with knowledge about the app.put()
method, go ahead and enhance your Express.js projects with seamless handling of PUT requests!
👨💻 Join our Community:
Author
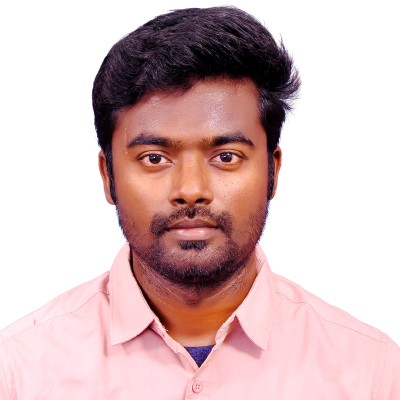
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (Express app.put() Method), please comment here. I will help you immediately.