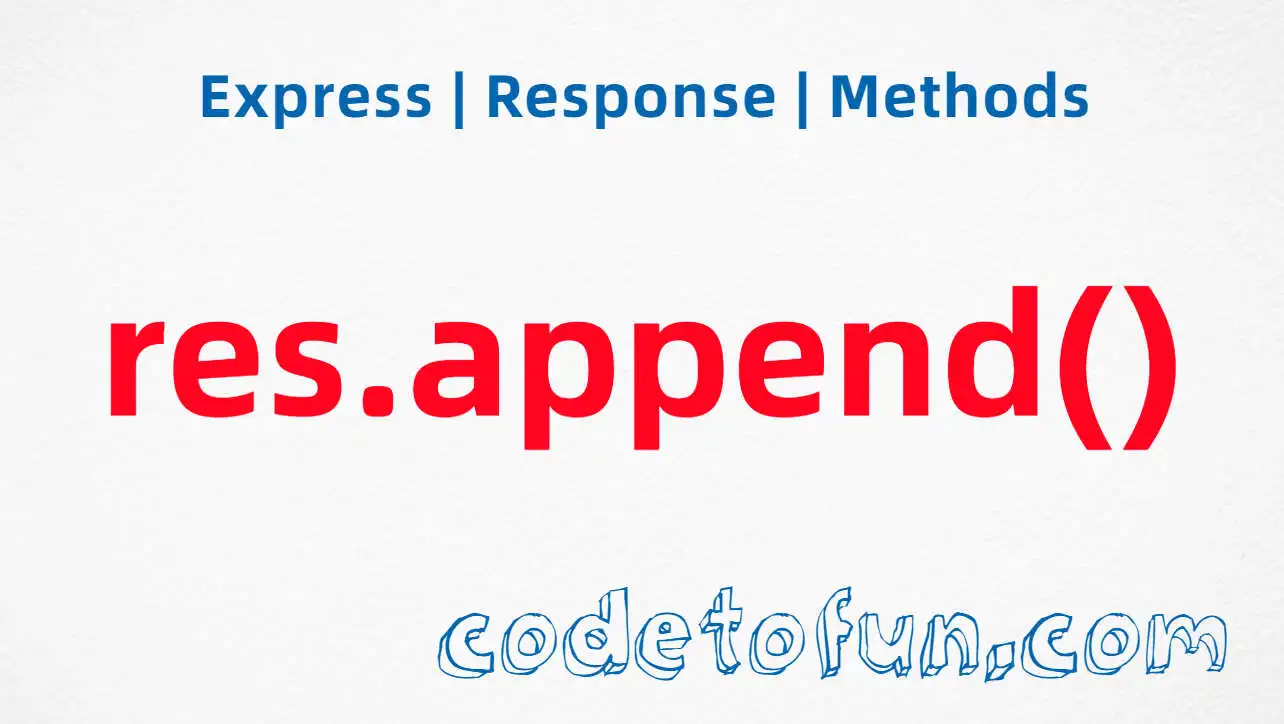
Express.js Basic
- express Intro
- express express()
- express Application
Properties
Events
Methods
- express Request
- express Response
- express Router
Express app.post() Method
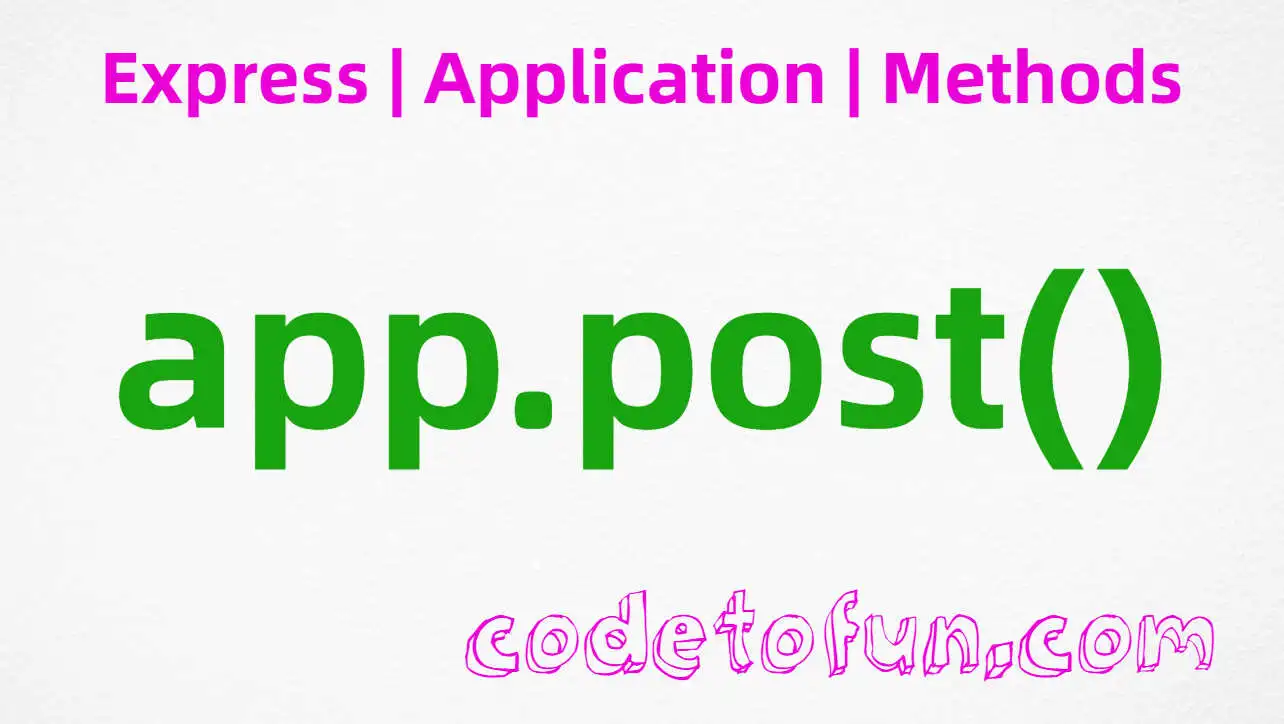
Photo Credit to CodeToFun
🙋 Introduction
As a web developer using Express.js, understanding how to handle different HTTP methods is crucial.
In this guide, we'll explore the app.post()
method, a key feature of Express.js designed specifically for handling HTTP POST requests.
💡 Syntax
The syntax for the app.post()
method is straightforward:
app.post(path, callback)
- path: A string representing the route or path for which the POST request is being handled.
- callback: The route handler function that processes the POST request.
❓ How app.post() Works
The app.post()
method in Express.js is designed to handle HTTP POST requests. This is particularly useful when dealing with form submissions or any scenario where the client is sending data to the server.
app.post('/submit-form', (req, res) => {
const formData = req.body;
// Process the submitted form data
res.send('Form submitted successfully!');
});
In this example, the route /submit-form captures POST requests and processes the form data sent by the client.
📚 Use Cases
Form Submissions:
Utilize
app.post()
to handle form submissions, processing data sent by clients through POST requests.example.jsCopiedapp.post('/contact-form', (req, res) => { const contactData = req.body; // Process the submitted contact form data res.send('Thank you for contacting us!'); });
Data Creation:
In scenarios where you need to create new data, such as creating a new user,
app.post()
is the ideal choice.example.jsCopiedapp.post('/create-user', (req, res) => { const userData = req.body; // Logic to create a new user using the submitted data res.send('User created successfully!'); });
🏆 Best Practices
Validate Input Data:
Always validate input data received in the req.body object to ensure it meets your application's requirements.
example.jsCopiedapp.post('/submit-form', (req, res) => { const formData = req.body; // Validate form data if (!formData || !formData.name || !formData.email) { res.status(400).send('Invalid form data'); return; } // Process the validated form data res.send('Form submitted successfully!'); });
Implement Robust Error Handling:
Implement error handling within your
app.post()
route handlers to handle potential issues gracefully.example.jsCopiedapp.post('/create-user', (req, res) => { const userData = req.body; // Simulate an error during user creation if (Math.random() < 0.5) { res.status(500).send('Internal Server Error'); } else { // Logic to create a new user using the submitted data res.send('User created successfully!'); } });
Middleware Integration:
Combine
app.post()
with middleware functions for tasks like data validation or authentication before processing the POST operation.example.jsCopied// Middleware for user authentication const authenticateUser = (req, res, next) => { // Implement your authentication logic here if (req.isAuthenticated()) { return next(); } else { res.status(401).send('Unauthorized'); } }; // Apply authentication middleware to the post route app.post('/create-post', authenticateUser, (req, res) => { const postData = req.body; // Logic to create a new post using the submitted data res.send('Post created successfully!'); });
🎉 Conclusion
The app.post()
method in Express.js is a powerful tool for handling HTTP POST requests, whether it's processing form submissions, creating new data, or any other scenario involving data sent from clients. By following best practices and leveraging middleware, you can build secure and efficient Express.js applications that handle POST requests seamlessly.
Now, armed with knowledge about the app.post()
method, go ahead and enhance your Express.js projects with effective handling of POST requests!
👨💻 Join our Community:
Author
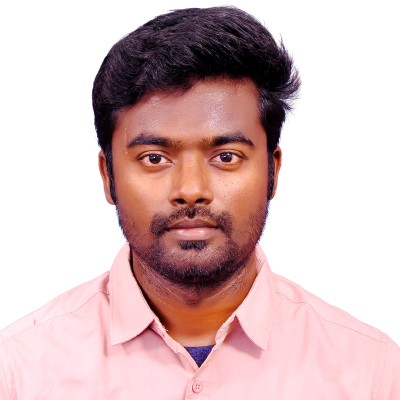
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (Express app.post() Method), please comment here. I will help you immediately.
Their posts always leave us feeling informed and entertained. We’re big fans of their style and creativity.
Thank you so much.
Your posts are always so well-researched and informative I appreciate how thorough and detailed your content is
Thank you! I strive to provide comprehensive and informative content.
Thank you for the amazing blog post!
Thank you so much! Glad you enjoyed it!
Your blog is a great source of positivity and inspiration in a world filled with negativity Thank you for making a difference
Thank you for your kind words! I’m glad to spread positivity in a world that needs it.
Thank you for creating such valuable content. Your hard work and dedication are appreciated by so many.
Your blog posts never fail to entertain and educate me. I especially enjoyed the recent one about [insert topic]. Keep up the great work!
Your posts always provide me with a new perspective and encourage me to look at things differently Thank you for broadening my horizons
The topics covered here are always so interesting and unique Thank you for keeping me informed and entertained!
Love this blog! The content is always so relevant and insightful, keep up the great work!
Thank you so much.