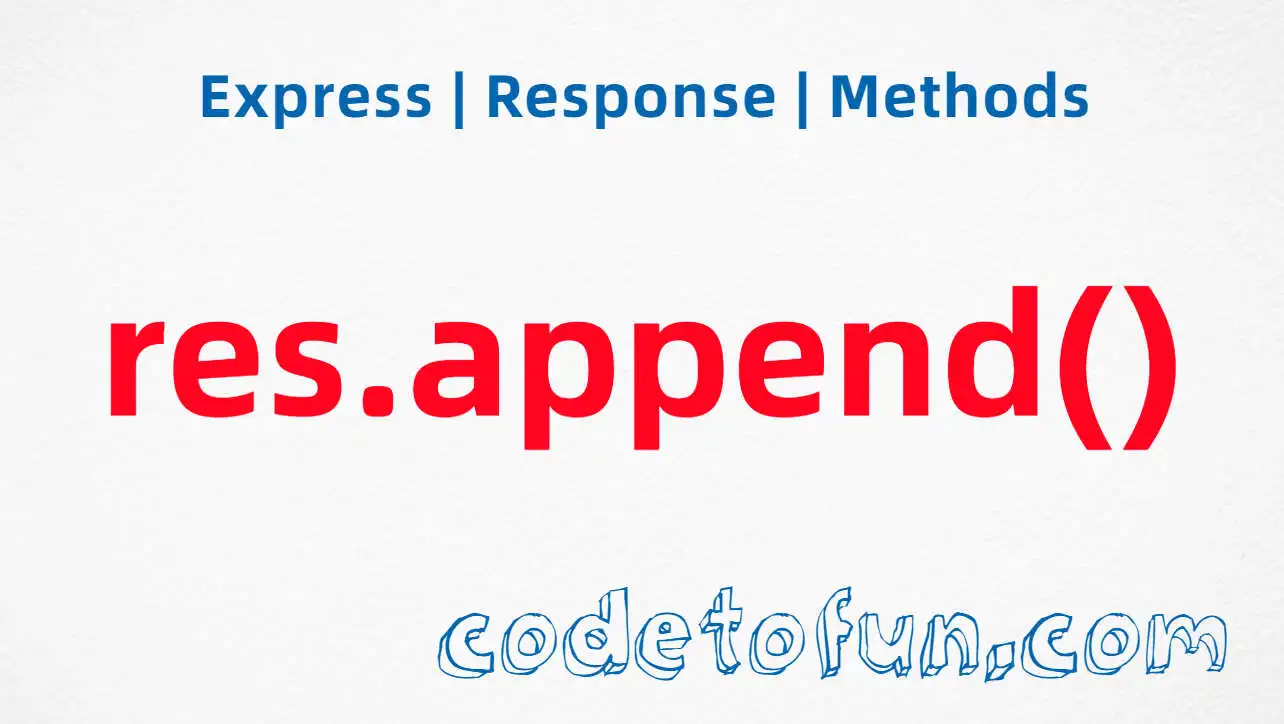
Express.js Basic
- express Intro
- express express()
- express Application
Properties
Events
Methods
- express Request
- express Response
- express Router
Express app.param() Method
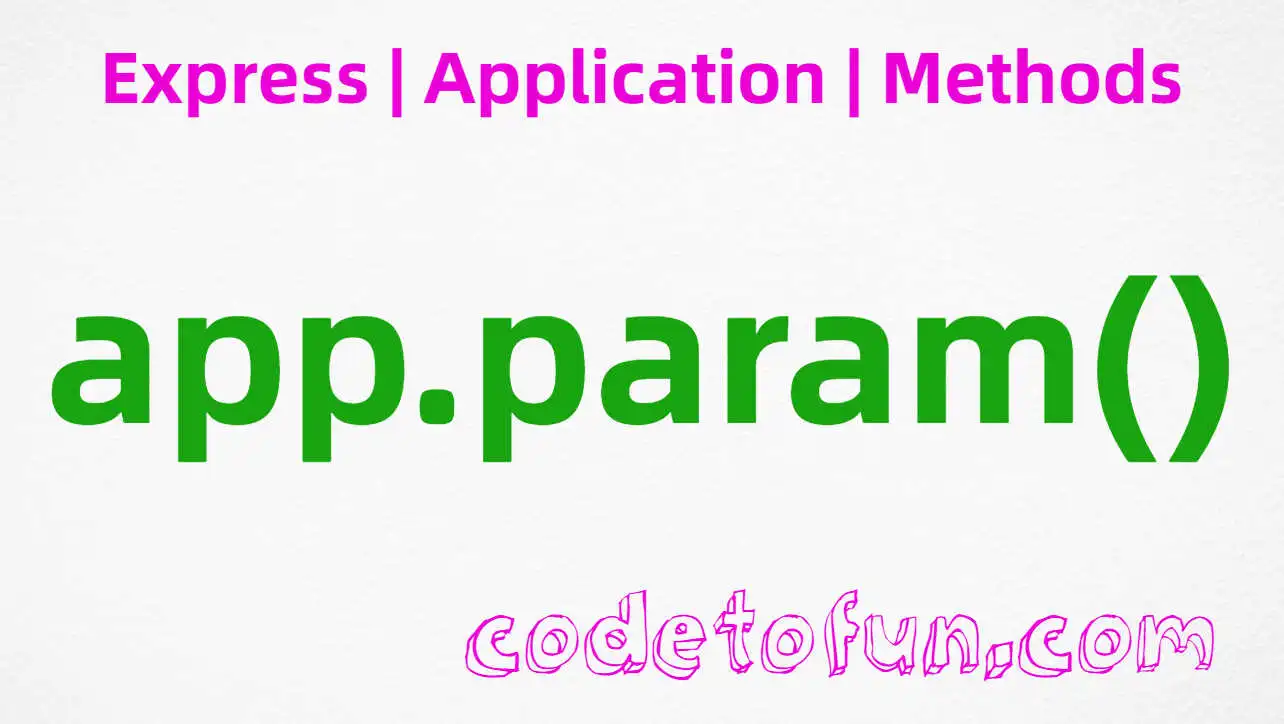
Photo Credit to CodeToFun
🙋 Introduction
Express.js provides a powerful feature called app.param()
that allows you to define middleware for handling route parameters. This method is particularly useful when you want to perform custom logic or validation on parameters passed in the URL.
In this guide, we'll explore the syntax, usage, and examples of the app.param()
method to enhance your understanding of dynamic route parameters in Express.js.
💡 Syntax
The syntax for the app.param()
method is straightforward:
app.param(name, callback)
- name: The parameter name or an array of parameter names.
- callback: The callback function that will be invoked with the value of the parameter.
❓ How app.param() Works
The app.param()
method is used to define middleware that will be executed for certain route parameters. When a route with defined parameters is matched, Express calls the associated middleware with the value of the parameter. This is particularly useful for tasks like validation, data retrieval, or any custom logic related to the parameters.
// Define middleware for 'userId' parameter
app.param('userId', (req, res, next, userId) => {
// Custom logic for 'userId', e.g., fetching user data from the database
req.user = getUserById(userId);
next();
});
// Route using the 'userId' parameter
app.get('/users/:userId', (req, res) => {
// Access user data set by the middleware
const user = req.user;
res.send(`User details: ${JSON.stringify(user)}`);
});
In this example, the app.param('userId', ...) middleware is executed whenever a route with the 'userId' parameter is matched, allowing custom logic to be applied before reaching the route handler.
📚 Use Cases
Data Retrieval:
Use
app.param()
to fetch data based on route parameters, making it available to subsequent route handlers.example.jsCopied// Middleware for retrieving data based on 'postId' app.param('postId', (req, res, next, postId) => { req.post = getPostById(postId); next(); }); // Route using the 'postId' parameter app.get('/posts/:postId', (req, res) => { const post = req.post; res.send(`Post details: ${JSON.stringify(post)}`); });
Validation:
Leverage
app.param()
to validate route parameters, ensuring they meet certain criteria before reaching the route handler.example.jsCopied// Middleware for validating 'productId' app.param('productId', (req, res, next, productId) => { if (isValidProductId(productId)) { next(); } else { res.status(400).send('Invalid product ID'); } }); // Route using the 'productId' parameter app.get('/products/:productId', (req, res) => { res.send('Product details'); });
🏆 Best Practices
Order Matters:
Define
app.param()
middleware in the order you want them to be executed. Express will execute them in the order they are declared.Reusability:
Consider creating reusable middleware functions for common parameter-related tasks and apply them to multiple routes.
Keep It Simple:
Avoid complex logic within
app.param()
middleware; instead, focus on tasks directly related to parameter handling.
🎉 Conclusion
The app.param()
method in Express.js provides a flexible and powerful mechanism for handling dynamic route parameters. Whether you need to fetch data, perform validation, or execute custom logic, app.param()
enhances the capabilities of your Express.js applications.
Now equipped with knowledge about the app.param()
method, go ahead and take advantage of dynamic route parameters in your Express.js projects!
👨💻 Join our Community:
Author
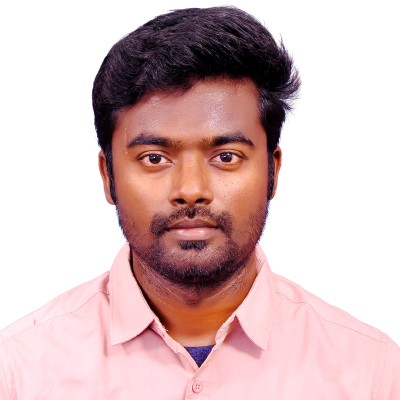
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (Express app.param() Method), please comment here. I will help you immediately.