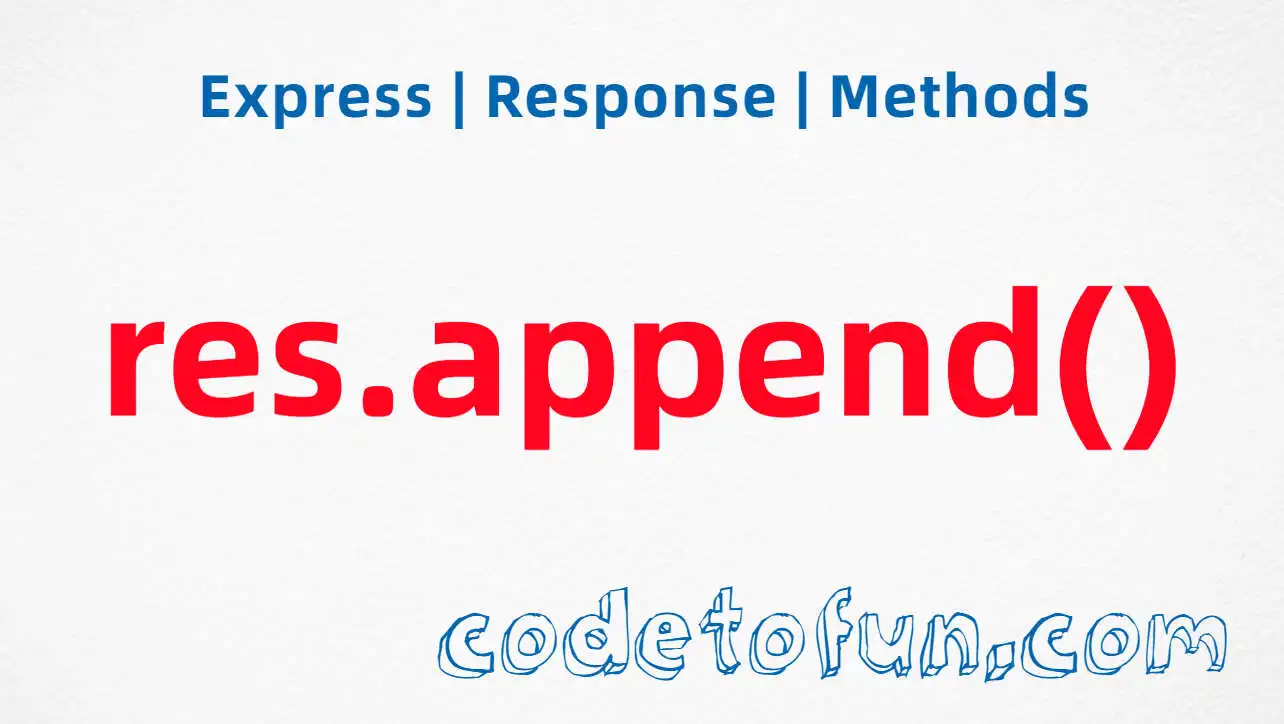
Express.js Basic
- express Intro
- express express()
- express Application
Properties
Events
Methods
- express Request
- express Response
- express Router
Express app.get() Method
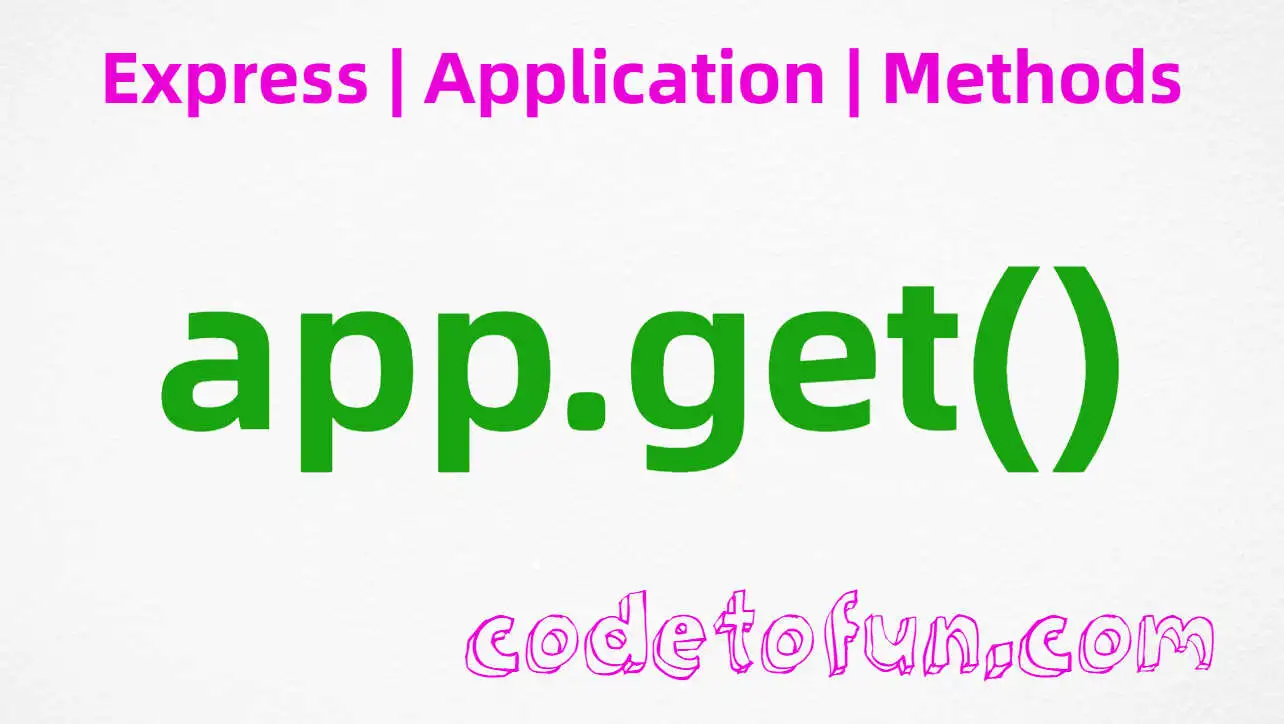
Photo Credit to CodeToFun
🙋 Introduction
Express.js, a popular web application framework for Node.js, simplifies handling various HTTP methods, including the ubiquitous GET request.
In this comprehensive guide, we'll delve into the syntax, usage, and real-world examples of the app.get()
method in Express.js, providing you with the insights needed to harness its power for building dynamic web applications.
💡 Syntax
The app.get()
method in Express.js follows a straightforward syntax:
app.get(path, callback)
- path: A string representing the route or path for which the GET request is being handled.
- callback: The route handler function that processes the GET request.
❓ How app.get() Works
app.get()
is designed to handle HTTP GET requests, allowing you to define specific logic for different routes. This method is pivotal for retrieving information from the server and rendering dynamic content.
app.get('/products/:productId', (req, res) => {
const productId = req.params.productId;
// Logic to fetch product details based on productId
res.render('product-details', { productId });
});
In this example, the route /products/:productId captures GET requests and dynamically renders the product details based on the provided productId.
📚 Use Cases
Fetching Data:
Use
app.get()
to handle requests for fetching data, commonly employed in RESTful APIs.example.jsCopiedapp.get('/api/users', (req, res) => { // Logic to fetch user data from the database const users = getUsersFromDatabase(); res.json(users); });
Rendering Views:
Leverage
app.get()
to render views and dynamically generate HTML content for specific routes.example.jsCopiedapp.get('/about', (req, res) => { // Logic to render the 'about' view res.render('about'); });
🏆 Best Practices
Route Organization:
Organize your routes logically to maintain a clean and structured codebase. Group related
app.get()
routes together for better readability.example.jsCopied// Grouping product-related routes app.get('/products', (req, res) => { // Logic to fetch and render a list of products res.render('product-list'); }); app.get('/products/:productId', (req, res) => { const productId = req.params.productId; // Logic to fetch and render product details res.render('product-details', { productId }); });
Error Handling:
Implement robust error handling within your
app.get()
route handlers to gracefully manage unexpected situations.example.jsCopiedapp.get('/profile/:username', (req, res) => { const username = req.params.username; // Simulate an error if the username is not found if (!userExists(username)) { res.status(404).send('User not found'); } else { // Logic to fetch and render user profile res.render('profile', { username }); } });
Middleware Integration:
Integrate middleware functions with
app.get()
for tasks like authentication or logging before processing the GET request.example.jsCopied// Middleware for logging request information const logRequest = (req, res, next) => { console.log(`GET request received for path: ${req.path}`); next(); }; // Apply logging middleware to all GET requests app.get('*', logRequest, (req, res) => { // Actual route logic res.send('Hello, World!'); });
🎉 Conclusion
The app.get()
method in Express.js is a fundamental tool for handling HTTP GET requests, providing flexibility and control in your web application development. Armed with the knowledge presented in this guide, you can confidently employ app.get()
to fetch data, render views, and create dynamic and responsive web applications.
Now, go ahead and explore the possibilities of app.get()
in your Express.js projects!
👨💻 Join our Community:
Author
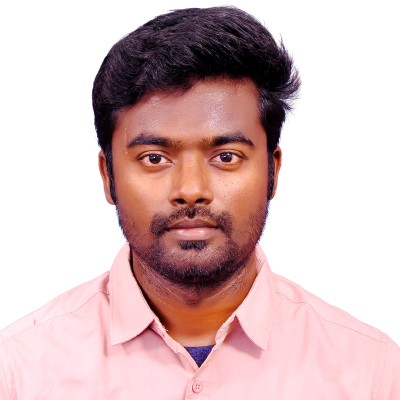
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (Express app.get() Method), please comment here. I will help you immediately.