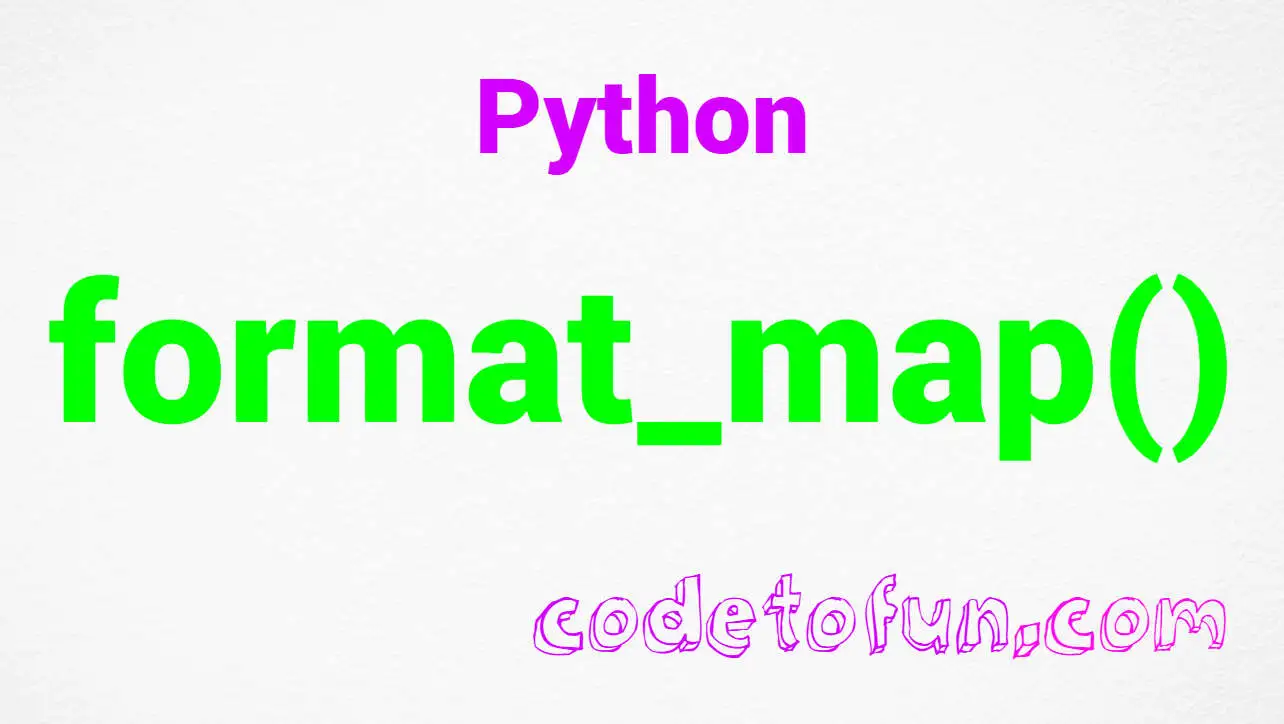
Python Basic
Python Interview Programs
- Python Interview Programs
- Python Abundant Number
- Python Amicable Number
- Python Armstrong Number
- Python Average of N Numbers
- Python Automorphic Number
- Python Biggest of three numbers
- Python Binary to Decimal
- Python Common Divisors
- Python Composite Number
- Python Condense a Number
- Python Cube Number
- Python Decimal to Binary
- Python Decimal to Octal
- Python Disarium Number
- Python Even Number
- Python Evil Number
- Python Factorial of a Number
- Python Fibonacci Series
- Python GCD
- Python Happy Number
- Python Harshad Number
- Python LCM
- Python Leap Year
- Python Magic Number
- Python Matrix Addition
- Python Matrix Division
- Python Matrix Multiplication
- Python Matrix Subtraction
- Python Matrix Transpose
- Python Maximum Value of an Array
- Python Minimum Value of an Array
- Python Multiplication Table
- Python Natural Number
- Python Number Combination
- Python Odd Number
- Python Palindrome Number
- Python Pascalβs Triangle
- Python Perfect Number
- Python Perfect Square
- Python Power of 2
- Python Power of 3
- Python Pronic Number
- Python Prime Factor
- Python Prime Number
- Python Smith Number
- Python Strong Number
- Python Sum of Array
- Python Sum of Digits
- Python Swap Two Numbers
- Python Triangular Number
Python Program to check Natural Number
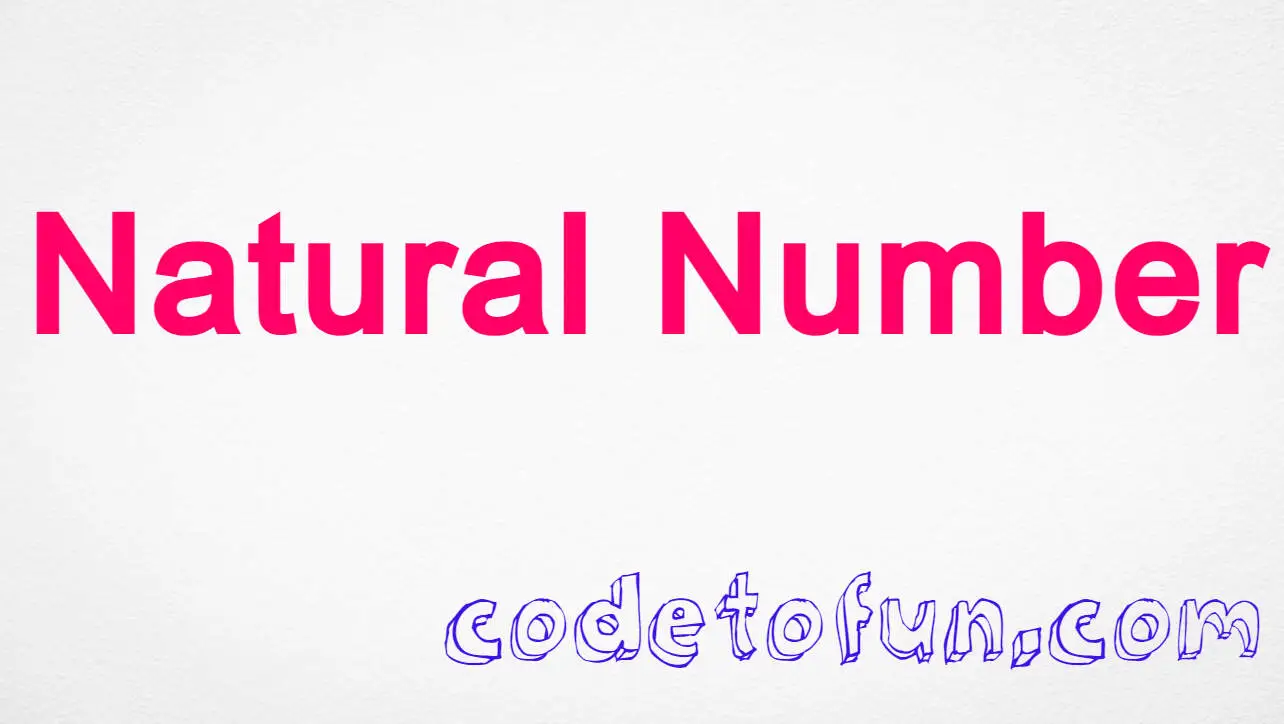
Photo Credit to CodeToFun
π Introduction
In the realm of programming, understanding and validating numbers is a fundamental task. One common classification is that of natural numbers.
A natural number is a positive integer greater than zero.
In this tutorial, we'll explore a simple Python program that checks whether a given number is a natural number.
π Example
Let's delve into the Python code that accomplishes this functionality.
# Function to check if a number is a natural number
def is_natural_number(num):
return True if num > 0 else False
# Driver program
if __name__ == "__main__":
# Replace this value with your desired number
number = 42
# Check if the number is a natural number
if is_natural_number(number):
print(f"{number} is a natural number.")
else:
print(f"{number} is not a natural number.")
π» Testing the Program
To test the program with different numbers, replace the value of number in the if __name__ == "__main__": block.
42 is a natural number.
Run the script to see if the given number is a natural number.
π§ How the Program Works
- The program defines a function is_natural_number that takes a number as input and returns True if it's a natural number (greater than 0) and False otherwise.
- In the if __name__ == "__main__": block, replace the value of number with the desired number.
- The program then checks if the number is a natural number using the is_natural_number function.
- It prints whether the number is a natural number or not based on the result.
π€ Considerations
- The program assumes the input number is an integer.
- For user input scenarios, additional validation may be required to handle non-integer inputs.
π Between the Given Range
Let's dive into the python code that checks and displays natural numbers in the range from 1 to 10.
# Function to check and display natural numbers in the range 1 to 10
def check_natural_numbers():
start_range = 1
end_range = 10
# Displaying the header
print(f"Natural Numbers in the Range {start_range} to {end_range}:")
# Loop through the range and check for natural numbers
for num in range(start_range, end_range + 1):
print(num, end=" ")
# Call the function to check and display natural numbers
check_natural_numbers()
π» Testing the Program
Natural Numbers in the range 1 to 10: 1 2 3 4 5 6 7 8 9 10
Run the script to see the natural numbers in the range from 1 to 10.
π§ How the Program Works
- The program defines a function check_natural_numbers that sets the range from 1 to 10 (inclusive).
- Inside the function, it uses a for loop to iterate through each number in the specified range.
- For each iteration, it prints the natural numbers.
π§ Understanding the Concept of Natural Numbers
Natural numbers are a set of positive integers that start from 1 and continue indefinitely. They are often used for counting and ordering.
In this program, we check whether a given number is a natural number by verifying if it is greater than zero.
π Conclusion
This python program serves as a foundational example for checking whether a number is a natural number or not. Understanding the characteristics of natural numbers and incorporating such checks can be essential in various programming scenarios
Feel free to incorporate and modify this code as needed for your specific use case. Happy coding!
π¨βπ» Join our Community:
Author
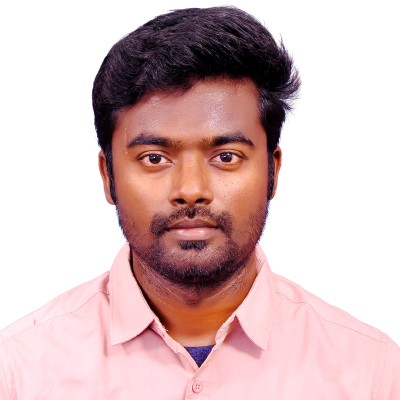
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (Python Program to check Natural Number), please comment here. I will help you immediately.