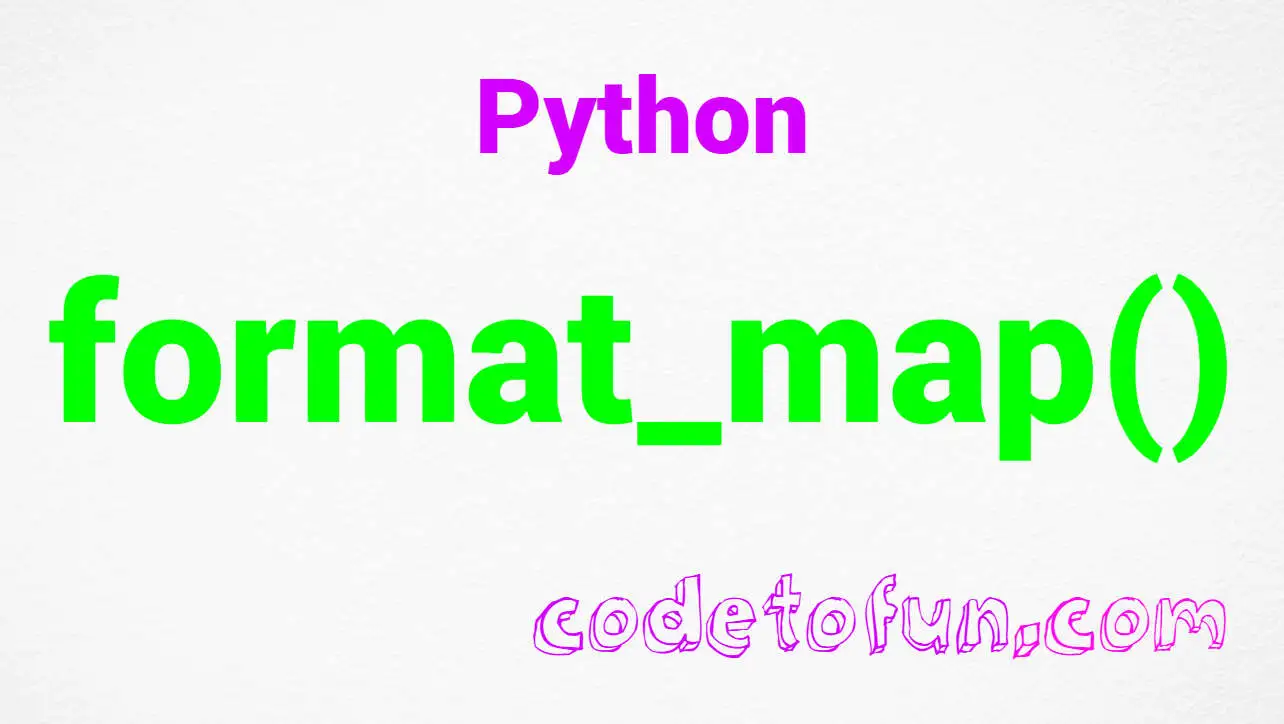
Python Basic
Python Interview Programs
- Python Interview Programs
- Python Abundant Number
- Python Amicable Number
- Python Armstrong Number
- Python Average of N Numbers
- Python Automorphic Number
- Python Biggest of three numbers
- Python Binary to Decimal
- Python Common Divisors
- Python Composite Number
- Python Condense a Number
- Python Cube Number
- Python Decimal to Binary
- Python Decimal to Octal
- Python Disarium Number
- Python Even Number
- Python Evil Number
- Python Factorial of a Number
- Python Fibonacci Series
- Python GCD
- Python Happy Number
- Python Harshad Number
- Python LCM
- Python Leap Year
- Python Magic Number
- Python Matrix Addition
- Python Matrix Division
- Python Matrix Multiplication
- Python Matrix Subtraction
- Python Matrix Transpose
- Python Maximum Value of an Array
- Python Minimum Value of an Array
- Python Multiplication Table
- Python Natural Number
- Python Number Combination
- Python Odd Number
- Python Palindrome Number
- Python Pascalβs Triangle
- Python Perfect Number
- Python Perfect Square
- Python Power of 2
- Python Power of 3
- Python Pronic Number
- Python Prime Factor
- Python Prime Number
- Python Smith Number
- Python Strong Number
- Python Sum of Array
- Python Sum of Digits
- Python Swap Two Numbers
- Python Triangular Number
Python Program to find Composite Number
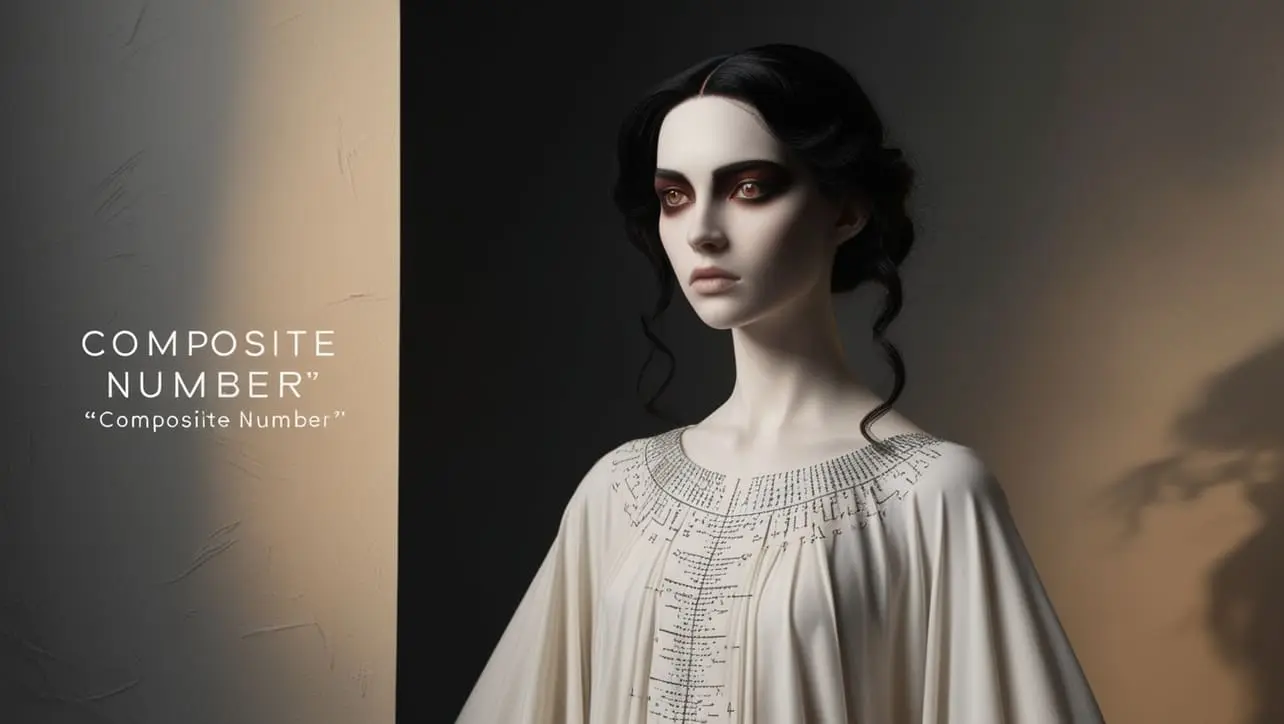
Photo Credit to CodeToFun
π Introduction
In the realm of programming, identifying and working with different types of numbers is a fundamental skill.
A composite number is one that has more than two positive divisors, excluding 1 and itself. In this tutorial, we'll explore a python program that efficiently determines whether a given number is composite or not.
π Example
Let's dive into the python code that checks whether a number is composite or not.
# Function to check if a number is composite
def is_composite(number):
if number > 1:
for i in range(2, number):
if (number % i) == 0:
return True # The number has a divisor other than 1 and itself
else:
return False # The number is not divisible by any other number
else:
return False # Numbers less than or equal to 1 are not considered composite
# Default input value
default_input = 12
# Check if the default input is a composite number
if is_composite(default_input):
print(f"{default_input} is a composite number.")
else:
print(f"{default_input} is not a composite number.")
π» Testing the Program
To test the program with a different number, simply replace the value of default_input with your desired number.
12 is a composite number.
Run the script to see whether the given number is a composite number.
π§ How the Program Works
- The program defines a function is_composite that takes a number as input and checks if it is a composite number.
- Inside the function, it iterates through numbers from 2 to the given number to check if any of them is a divisor.
- If a divisor is found, the number is composite; otherwise, it is not.
- The default input value is set to 12, and the program prints whether it is a composite number or not.
π Between the Given Range
Let's dive into the Python code that checks for composite numbers in the specified range.
# Function to check if a number is composite
def is_composite(num):
if num > 1:
for i in range(2, num):
if (num % i) == 0:
return True
else:
return False
else:
return False
# Driver program
def main():
print("Composite numbers in the range 1 to 10:")
for number in range(1, 11):
if is_composite(number):
print(number)
if __name__ == "__main__":
main()
π» Testing the Program
Composite numbers in the range 1 to 10 are: 4 6 8 9 10
Run the script, and it will display the composite numbers in the range 1 to 10.
π§ How the Program Works
- The program defines a function is_composite that takes a number as input and returns True if it is composite, and False otherwise.
- Inside the function, it iterates through numbers from 2 to the given number (exclusive) to check for divisors.
- The main function prints the composite numbers in the range 1 to 10 by calling the is_composite function.
π§ Understanding the Concept of Composite Numbers
Composite numbers are integers greater than 1 that have divisors other than 1 and themselves.
For example, the number 12 is composite because it can be formed by multiplying 2 and 6 or 3 and 4.
π’ Optimizing the Program
While the provided program is effective, consider exploring and implementing optimizations for larger numbers, such as checking divisors up to the square root of the number for improved efficiency.
Feel free to incorporate and modify this code as needed for your specific use case. Happy coding!
π¨βπ» Join our Community:
Author
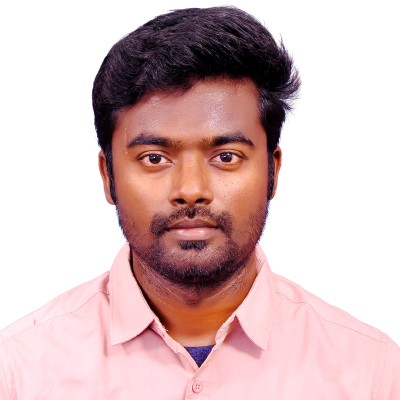
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (Python Program to find Composite Number), please comment here. I will help you immediately.