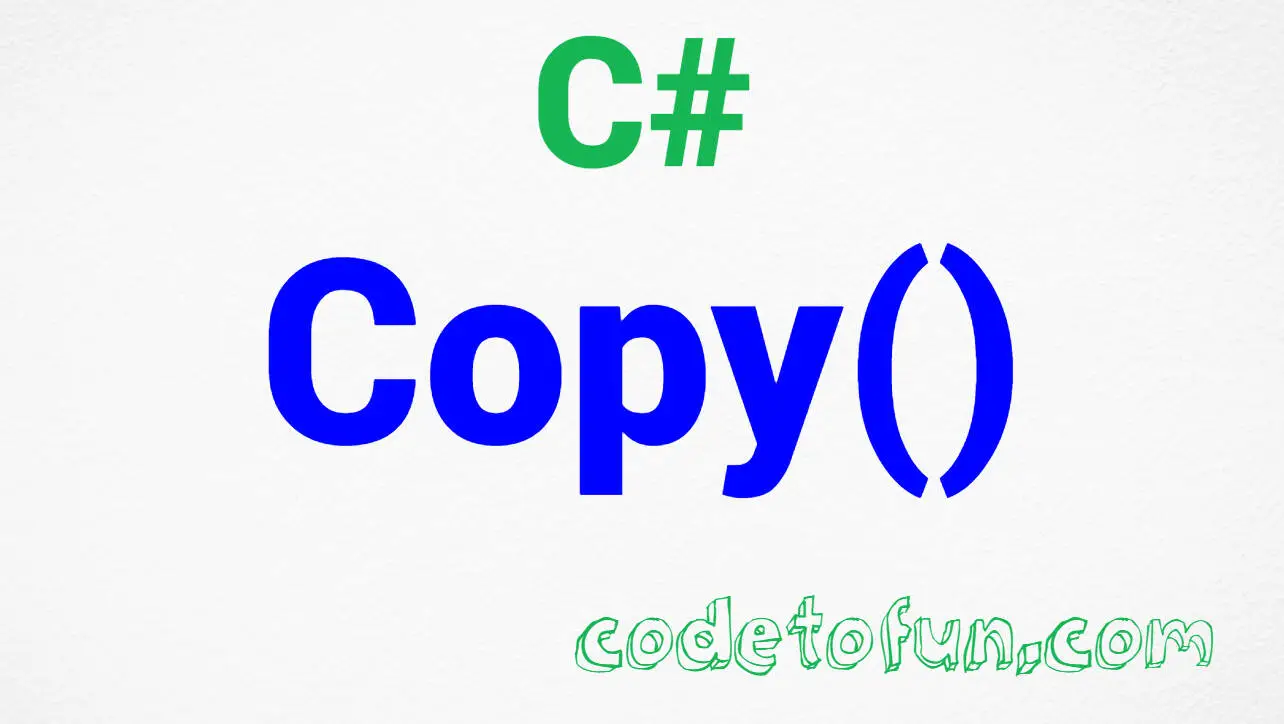
C# Basic
C# Interview Programs
- C# Interview Programs
- C# Abundant Number
- C# Amicable Number
- C# Armstrong Number
- C# Average of N Numbers
- C# Automorphic Number
- C# Biggest of three numbers
- C# Binary to Decimal
- C# Common Divisors
- C# Composite Number
- C# Condense a Number
- C# Cube Number
- C# Decimal to Binary
- C# Decimal to Octal
- C# Disarium Number
- C# Even Number
- C# Evil Number
- C# Factorial of a Number
- C# Fibonacci Series
- C# GCD
- C# Happy Number
- C# Harshad Number
- C# LCM
- C# Leap Year
- C# Magic Number
- C# Matrix Addition
- C# Matrix Division
- C# Matrix Multiplication
- C# Matrix Subtraction
- C# Matrix Transpose
- C# Maximum Value of an Array
- C# Minimum Value of an Array
- C# Multiplication Table
- C# Natural Number
- C# Number Combination
- C# Odd Number
- C# Palindrome Number
- C# Pascalβs Triangle
- C# Perfect Number
- C# Perfect Square
- C# Power of 2
- C# Power of 3
- C# Pronic Number
- C# Prime Factor
- C# Prime Number
- C# Smith Number
- C# Strong Number
- C# Sum of Array
- C# Sum of Digits
- C# Swap Two Numbers
- C# Triangular Number
C# Program to Check Smith Number
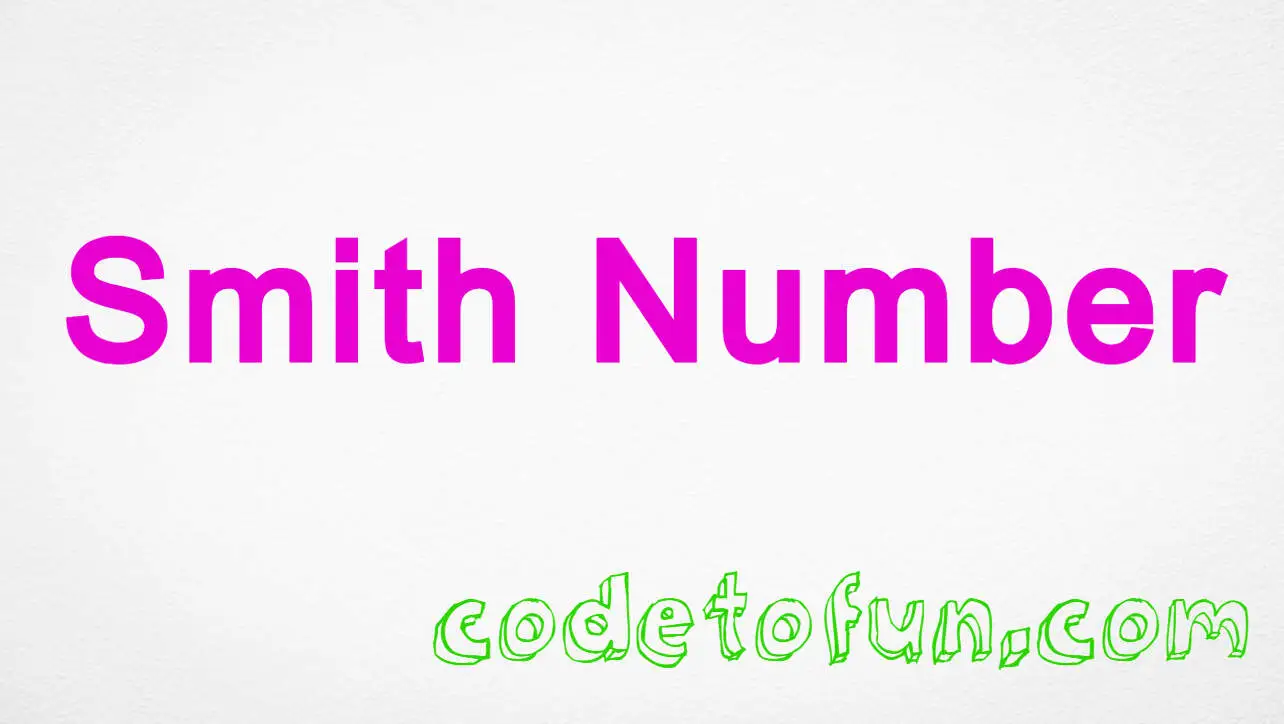
Photo Credit to CodeToFun
π Introduction
In the realm of programming, exploring number properties is a common and fascinating endeavor. One interesting type of number is the Smith Number.
A Smith Number is a composite number for which the sum of its digits is equal to the sum of the digits in its prime factorization.
In this tutorial, we'll explore a C# program that checks whether a given number is a Smith Number.
π Example
Let's take a look at the C# code that checks whether a given number is a Smith Number.
using System;
class Program {
// Function to calculate the sum of digits in a number
static int SumOfDigits(int num) {
int sum = 0;
while (num > 0) {
sum += num % 10;
num /= 10;
}
return sum;
}
// Function to calculate the sum of digits in the prime factorization of a number
static int SumOfPrimeFactors(int num) {
int i = 2, sum = 0;
while (num > 1) {
while (num % i == 0) {
sum += SumOfDigits(i);
num /= i;
}
i++;
}
return sum;
}
// Function to check if a number is a Smith Number
static bool IsSmithNumber(int num) {
return SumOfDigits(num) == SumOfPrimeFactors(num);
}
// Driver program
static void Main() {
// Replace this value with your desired number
int number = 85;
// Check if the number is a Smith Number
if (IsSmithNumber(number)) {
Console.WriteLine($"{number} is a Smith Number.");
} else {
Console.WriteLine($"{number} is not a Smith Number.");
}
}
}
π» Testing the Program
To test the program with different numbers, replace the value of number in the Main method.
85 is a Smith Number.
Compile and run the program to check whether the given number is a Smith Number.
π§ How the Program Works
- The program defines three functions: SumOfDigits to calculate the sum of digits in a number, SumOfPrimeFactors to calculate the sum of digits in the prime factorization, and IsSmithNumber to check if a number is a Smith Number.
- The Main method replaces the value of number with the desired number and checks if it is a Smith Number.
- The program utilizes loops and arithmetic operations to calculate the sum of digits and the sum of digits in the prime factorization.
π Between the Given Range
Let's take a look at the C# code that checks for Smith Numbers in the specified range.
using System;
class Program {
// Function to calculate the sum of digits
static int SumOfDigits(int n) {
int sum = 0;
while (n > 0) {
sum += n % 10;
n /= 10;
}
return sum;
}
// Function to calculate the sum of prime factors
static int SumOfPrimeFactors(int n) {
int sum = 0;
for (int i = 2; i <= n; ++i) {
while (n % i == 0) {
sum += SumOfDigits(i);
n /= i;
}
}
return sum;
}
// Function to check if a number is prime
static bool IsPrime(int n) {
if (n <= 1) {
return false;
}
for (int i = 2; i * i <= n; ++i) {
if (n % i == 0) {
return false;
}
}
return true;
}
// Function to check if a number is a Smith Number
static bool IsSmithNumber(int n) {
return IsPrime(n) ? false : SumOfDigits(n) == SumOfPrimeFactors(n);
}
// Main method
static void Main() {
Console.WriteLine("Smith Numbers in the Range 1 to 100:");
// Check numbers from 1 to 100
for (int i = 1; i <= 100; ++i) {
if (IsSmithNumber(i)) {
Console.Write($"{i} ");
}
}
Console.WriteLine();
}
}
π» Testing the Program
Smith Numbers in the Range 1 to 100: 4 22 27 58 85 94
Compile and run the program to see the Smith Numbers in the specified range.
π§ How the Program Works
- The program defines a function isPrime to check if a number is prime.
- The isSmithNumber function checks if a number is a Smith Number by comparing the sum of its digits with the sum of the digits in its prime factorization.
- The main function checks and prints Smith Numbers in the range from 1 to 100.
π§ Understanding the Concept of Smith Numbers
Before delving into the code, it's crucial to understand the concept of Smith Numbers. These numbers exhibit a unique property where the sum of their digits is equal to the sum of the digits in their prime factorization.
For example, 4, 22, 27, and 85 are Smith Numbers.
π’ Optimizing the Program
While the provided program is effective, consider exploring optimizations for larger numbers. You can further refine the code for improved efficiency or adapt it to your specific requirements.
Feel free to incorporate and modify this code as needed for your specific use case. Happy coding!
π¨βπ» Join our Community:
Author
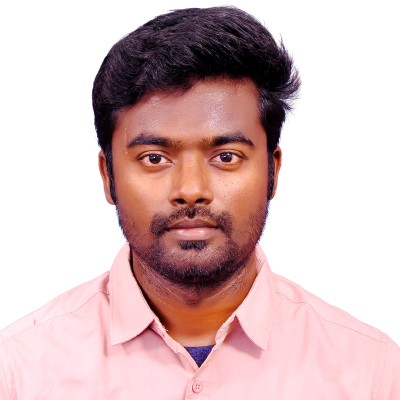
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (C# Program to Check Smith Number), please comment here. I will help you immediately.