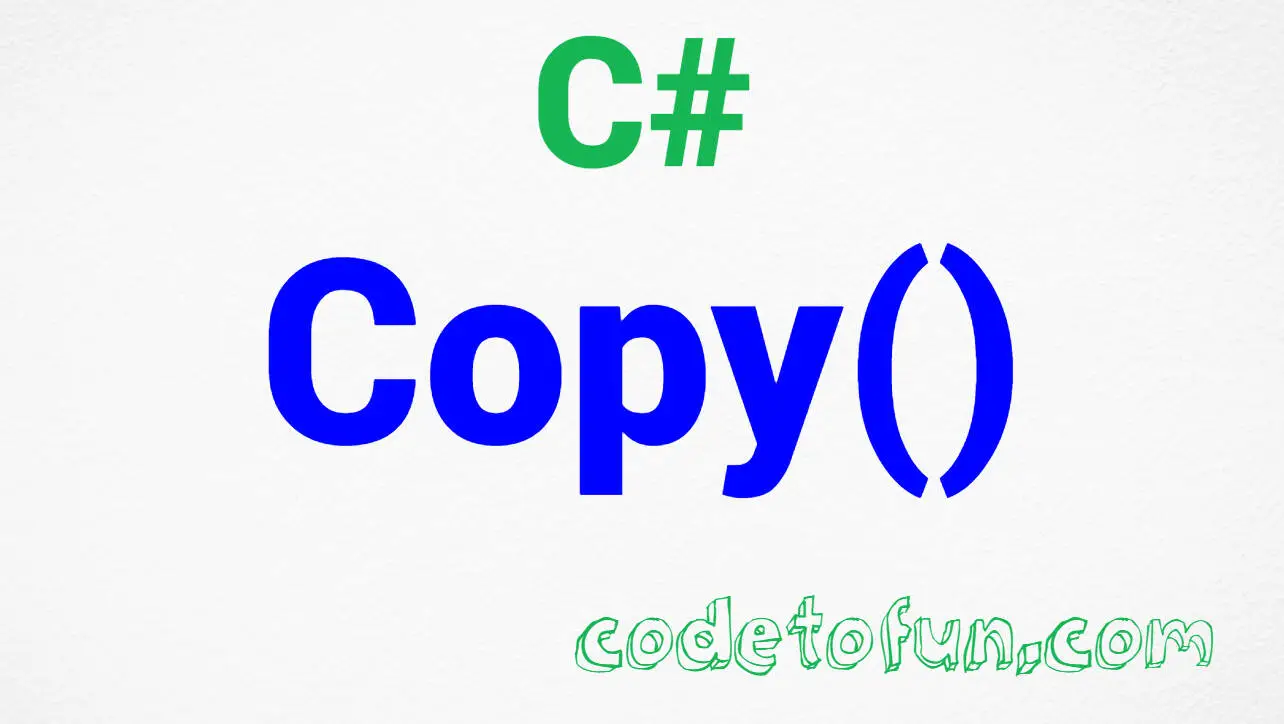
C# Basic
C# Interview Programs
- C# Interview Programs
- C# Abundant Number
- C# Amicable Number
- C# Armstrong Number
- C# Average of N Numbers
- C# Automorphic Number
- C# Biggest of three numbers
- C# Binary to Decimal
- C# Common Divisors
- C# Composite Number
- C# Condense a Number
- C# Cube Number
- C# Decimal to Binary
- C# Decimal to Octal
- C# Disarium Number
- C# Even Number
- C# Evil Number
- C# Factorial of a Number
- C# Fibonacci Series
- C# GCD
- C# Happy Number
- C# Harshad Number
- C# LCM
- C# Leap Year
- C# Magic Number
- C# Matrix Addition
- C# Matrix Division
- C# Matrix Multiplication
- C# Matrix Subtraction
- C# Matrix Transpose
- C# Maximum Value of an Array
- C# Minimum Value of an Array
- C# Multiplication Table
- C# Natural Number
- C# Number Combination
- C# Odd Number
- C# Palindrome Number
- C# Pascalβs Triangle
- C# Perfect Number
- C# Perfect Square
- C# Power of 2
- C# Power of 3
- C# Pronic Number
- C# Prime Factor
- C# Prime Number
- C# Smith Number
- C# Strong Number
- C# Sum of Array
- C# Sum of Digits
- C# Swap Two Numbers
- C# Triangular Number
C# Program to Converter a Binary to Decimal
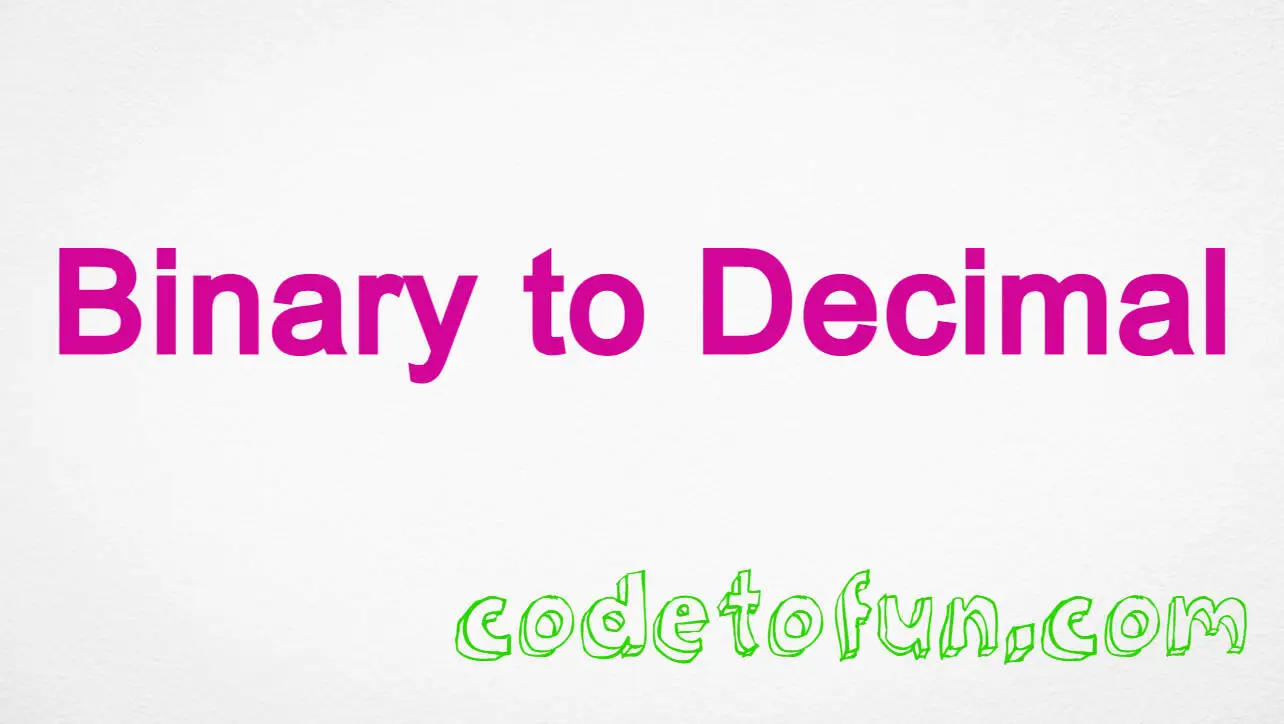
Photo Credit to CodeToFun
π Introduction
In the world of programming, understanding different number systems is crucial. One common conversion task is to convert a binary number to its decimal equivalent.
Binary is a base-2 number system, while decimal is a base-10 system.
In this tutorial, we'll explore a C# program that efficiently converts a binary number to its decimal representation.
π Example
Let's dive into the C# code that performs the binary to decimal conversion.
using System;
class Program {
// Function to convert binary to decimal
static int BinaryToDecimal(long binaryNumber) {
int decimalNumber = 0, i = 0;
while (binaryNumber != 0) {
int remainder = (int)(binaryNumber % 10);
binaryNumber /= 10;
decimalNumber += remainder * (int) Math.Pow(2, i);
++i;
}
return decimalNumber;
}
// Driver program
static void Main() {
// Replace this binary number with your desired value
long binaryNumber = 101010;
// Call the function to convert binary to decimal
int decimalNumber = BinaryToDecimal(binaryNumber);
Console.WriteLine($"Binary: {binaryNumber}");
Console.WriteLine($"Decimal: {decimalNumber}");
}
}
π» Testing the Program
To test the program with a different binary number, replace the value of binaryNumber in the main function.
Binary: 101010 Decimal: 42
Compile and run the program to see the binary-to-decimal conversion in action.
π§ How the Program Works
- The program defines a function BinaryToDecimal that takes a binary number as input and returns its decimal equivalent.
- Inside the function, it uses a while loop to iterate through each digit of the binary number.
- For each digit, it calculates the remainder and updates the decimal number accordingly.
- The Main method tests the conversion by providing a binary number and printing both the binary and decimal representations.
π§ Understanding the Concept of Binary and Decimal Systems
- Binary System (Base-2): In the binary system, numbers are represented using only two digits, 0 and 1. Each digit's place value is a power of 2.
- Decimal System (Base-10): The decimal system is the standard number system that we use every day. It uses ten digits, 0 through 9, and each digit's place value is a power of 10.
Binary-to-decimal conversion involves multiplying each binary digit by 2 raised to the power of its position and summing up the results.
For example, the binary number 101010 is converted to decimal as follows:
1 * 2^5 + 0 * 2^4 + 1 * 2^3 + 0 * 2^2 + 1 * 2^1 + 0 * 2^0 = 42
π’ Optimizing the Program
While the provided program is effective, consider exploring optimizations or handling edge cases for larger binary numbers.
Feel free to incorporate and modify this code as needed for your specific use case. Happy coding!
π¨βπ» Join our Community:
Author
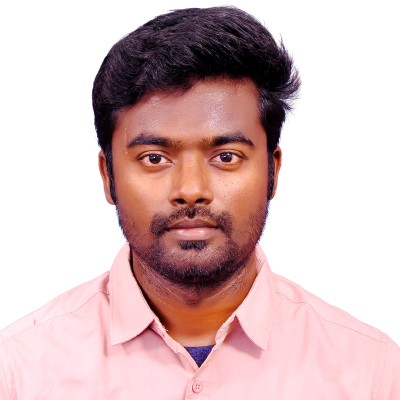
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (C# Program to Converter a Binary to Decimal), please comment here. I will help you immediately.