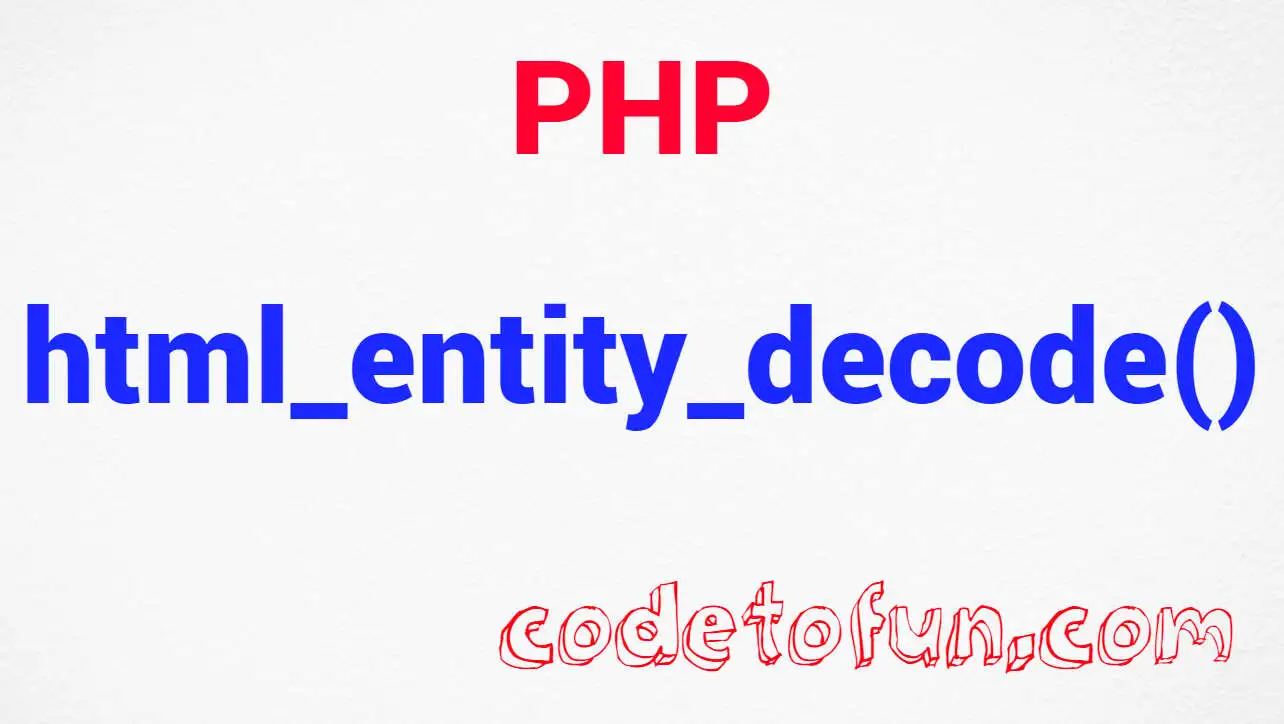
PHP Basic
- PHP Intro
- PHP Star Pattern
- PHP Number Pattern
- PHP Alphabet Pattern
- PHP String Functions
- PHP addcslashes()
- PHP addslashes()
- PHP bin2hex()
- PHP chop()
- PHP chr()
- PHP chunk_split()
- PHP convert_cyr_string()
- PHP convert_uudecode()
- PHP convert_uuencode()
- PHP count_chars()
- PHP crc32()
- PHP crypt()
- PHP explode()
- PHP fprintf()
- PHP get_html_translation_table()
- PHP hebrev()
- PHP hebrevc()
- PHP hex2bin()
- PHP html_entity_decode()
- PHP htmlentities()
- PHP htmlspecialchars_decode()
- PHP htmlspecialchars()
- PHP implode()
- PHP join()
PHP String count_chars() Function
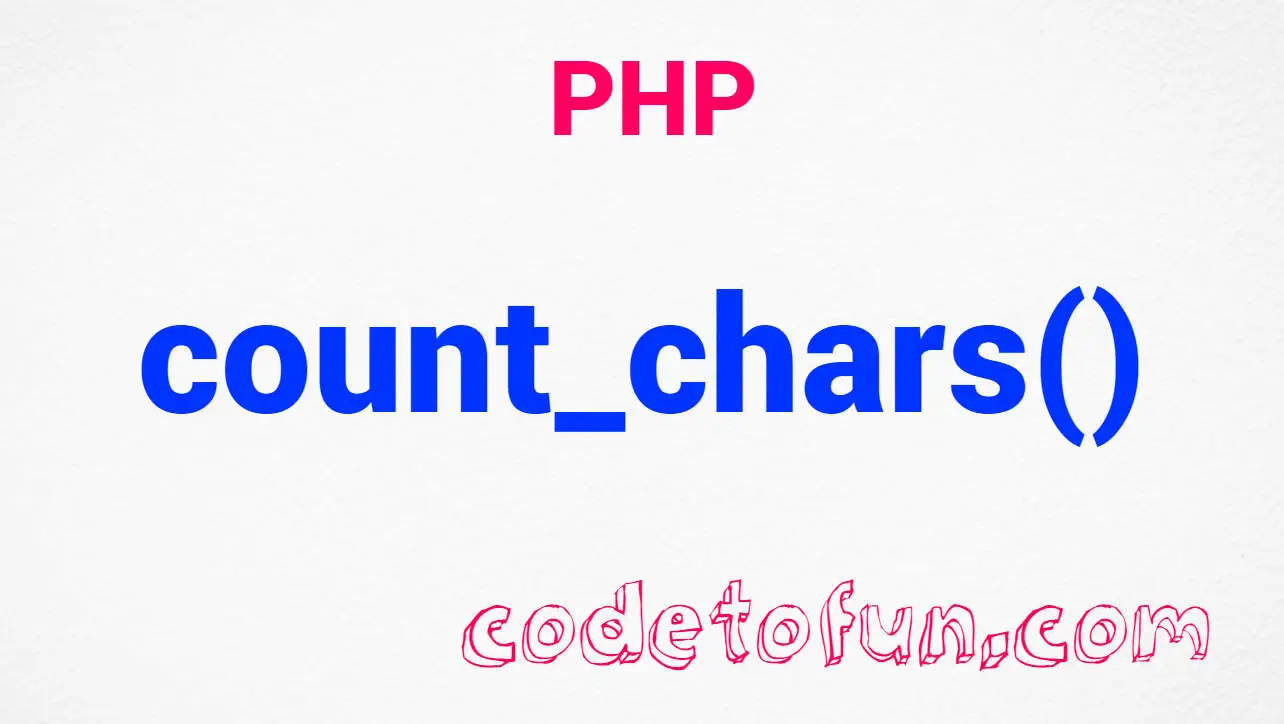
Photo Credit to CodeToFun
đ Introduction
In PHP programming, working with strings is a common and fundamental task.
The count_chars()
function is a versatile tool for analyzing and manipulating strings.
It returns information about the characters used in a string, providing insights into the string's composition.
In this tutorial, we'll explore the usage and functionality of the count_chars()
function in PHP.
đĄ Syntax
The signature of the count_chars()
function is as follows:
count_chars(string $string, int $mode = 0): mixed
- $string: The input string for analysis.
- $mode (optional): An optional parameter that specifies the return type. Default is 0. Possible values are 0, 1, 2, and 3.
đ Example 1
Let's dive into some examples to illustrate how the count_chars()
function works.
<?php
$string = "CodeToFun";
// Get character count information
$result1 = count_chars($string);
// Output the result
print_r($result1);
?>
đģ Output
Array ( [0] => 0 [1] => 0 [2] => 0 [3] => 0 [4] => 0 [5] => 0 [6] => 0 [7] => 0 [8] => 0 [9] => 0 [10] => 0 [11] => 0 [12] => 0 [13] => 0 [14] => 0 [15] => 0 [16] => 0 [17] => 0 [18] => 0 [19] => 0 [20] => 0 [21] => 0 [22] => 0 [23] => 0 [24] => 0 [25] => 0 [26] => 0 [27] => 0 [28] => 0 [29] => 0 [30] => 0 [31] => 0 [32] => 0 [33] => 0 [34] => 0 [35] => 0 [36] => 0 [37] => 0 [38] => 0 [39] => 0 [40] => 0 [41] => 0 [42] => 0 [43] => 0 [44] => 0 [45] => 0 [46] => 0 [47] => 0 [48] => 0 [49] => 0 [50] => 0 [51] => 0 [52] => 0 [53] => 0 [54] => 0 [55] => 0 [56] => 0 [57] => 0 [58] => 0 [59] => 0 [60] => 0 [61] => 0 [62] => 0 [63] => 0 [64] => 0 [65] => 0 [66] => 0 [67] => 1 [68] => 0 [69] => 0 [70] => 1 [71] => 0 [72] => 0 [73] => 0 [74] => 0 [75] => 0 [76] => 0 [77] => 0 [78] => 0 [79] => 0 [80] => 0 [81] => 0 [82] => 0 [83] => 0 [84] => 1 [85] => 0 [86] => 0 [87] => 0 [88] => 0 [89] => 0 [90] => 0 [91] => 0 [92] => 0 [93] => 0 [94] => 0 [95] => 0 [96] => 0 [97] => 0 [98] => 0 [99] => 0 [100] => 1 [101] => 1 [102] => 0 [103] => 0 [104] => 0 [105] => 0 [106] => 0 [107] => 0 [108] => 0 [109] => 0 [110] => 1 [111] => 2 [112] => 0 [113] => 0 [114] => 0 [115] => 0 [116] => 0 [117] => 1 [118] => 0 [119] => 0 [120] => 0 [121] => 0 [122] => 0 [123] => 0 [124] => 0 [125] => 0 [126] => 0 [127] => 0 [128] => 0 [129] => 0 [130] => 0 [131] => 0 [132] => 0 [133] => 0 [134] => 0 [135] => 0 [136] => 0 [137] => 0 [138] => 0 [139] => 0 [140] => 0 [141] => 0 [142] => 0 [143] => 0 [144] => 0 [145] => 0 [146] => 0 [147] => 0 [148] => 0 [149] => 0 [150] => 0 [151] => 0 [152] => 0 [153] => 0 [154] => 0 [155] => 0 [156] => 0 [157] => 0 [158] => 0 [159] => 0 [160] => 0 [161] => 0 [162] => 0 [163] => 0 [164] => 0 [165] => 0 [166] => 0 [167] => 0 [168] => 0 [169] => 0 [170] => 0 [171] => 0 [172] => 0 [173] => 0 [174] => 0 [175] => 0 [176] => 0 [177] => 0 [178] => 0 [179] => 0 [180] => 0 [181] => 0 [182] => 0 [183] => 0 [184] => 0 [185] => 0 [186] => 0 [187] => 0 [188] => 0 [189] => 0 [190] => 0 [191] => 0 [192] => 0 [193] => 0 [194] => 0 [195] => 0 [196] => 0 [197] => 0 [198] => 0 [199] => 0 [200] => 0 [201] => 0 [202] => 0 [203] => 0 [204] => 0 [205] => 0 [206] => 0 [207] => 0 [208] => 0 [209] => 0 [210] => 0 [211] => 0 [212] => 0 [213] => 0 [214] => 0 [215] => 0 [216] => 0 [217] => 0 [218] => 0 [219] => 0 [220] => 0 [221] => 0 [222] => 0 [223] => 0 [224] => 0 [225] => 0 [226] => 0 [227] => 0 [228] => 0 [229] => 0 [230] => 0 [231] => 0 [232] => 0 [233] => 0 [234] => 0 [235] => 0 [236] => 0 [237] => 0 [238] => 0 [239] => 0 [240] => 0 [241] => 0 [242] => 0 [243] => 0 [244] => 0 [245] => 0 [246] => 0 [247] => 0 [248] => 0 [249] => 0 [250] => 0 [251] => 0 [252] => 0 [253] => 0 [254] => 0 [255] => 0 )
đ§ How the Program Works
In this example, the count_chars()
function is used to get character count information for the string "CodeToFun".
đ Example 2
Let's dive into some examples to illustrate how the count_chars()
function works.
<?php
$string = "CodeToFun";
// Get character count information with mode 1
$result2 = count_chars($string, 1);
// Output the result
print_r($result2);
?>
đģ Output
Array ( [67] => 1 [70] => 1 [84] => 1 [100] => 1 [101] => 1 [110] => 1 [111] => 2 [117] => 1 )
đ§ How the Program Works
In this example, the count_chars()
function is used with mode 1 to get an associative array with character counts for the string "CodeToFun".
âŠī¸ Return Value
The count_chars()
function returns different values based on the specified mode:
- Mode 0 (default): Returns a string containing all distinct characters used in the input string.
- Mode 1: Returns an associative array with character counts.
- Mode 2: Returns an associative array with character counts, including zero counts.
- Mode 3: Returns a string containing all distinct characters used in the input string in reverse order.
đ Common Use Cases
The count_chars()
function is useful when you need detailed information about the characters present in a string. It can be applied in scenarios where you want to analyze and manipulate text data.
đ Notes
- The $mode parameter allows you to customize the function's behavior, providing flexibility in the type of information returned.
- Be aware that character case matters. The function distinguishes between uppercase and lowercase characters.
đĸ Optimization
The count_chars()
function is optimized for efficiency, and no additional optimization is typically needed. Use it as needed based on your specific requirements.
đ Conclusion
The count_chars()
function in PHP is a powerful tool for character analysis in strings. It provides valuable insights into the composition of a string, making it a valuable asset for text manipulation tasks.
Feel free to experiment with different strings and explore the behavior of the count_chars()
function in various scenarios. Happy coding!
đ¨âđģ Join our Community:
Author
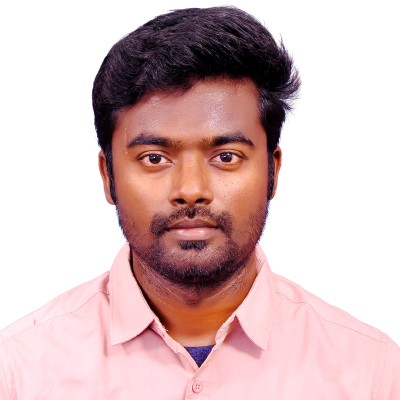
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (PHP String count_chars() Function), please comment here. I will help you immediately.