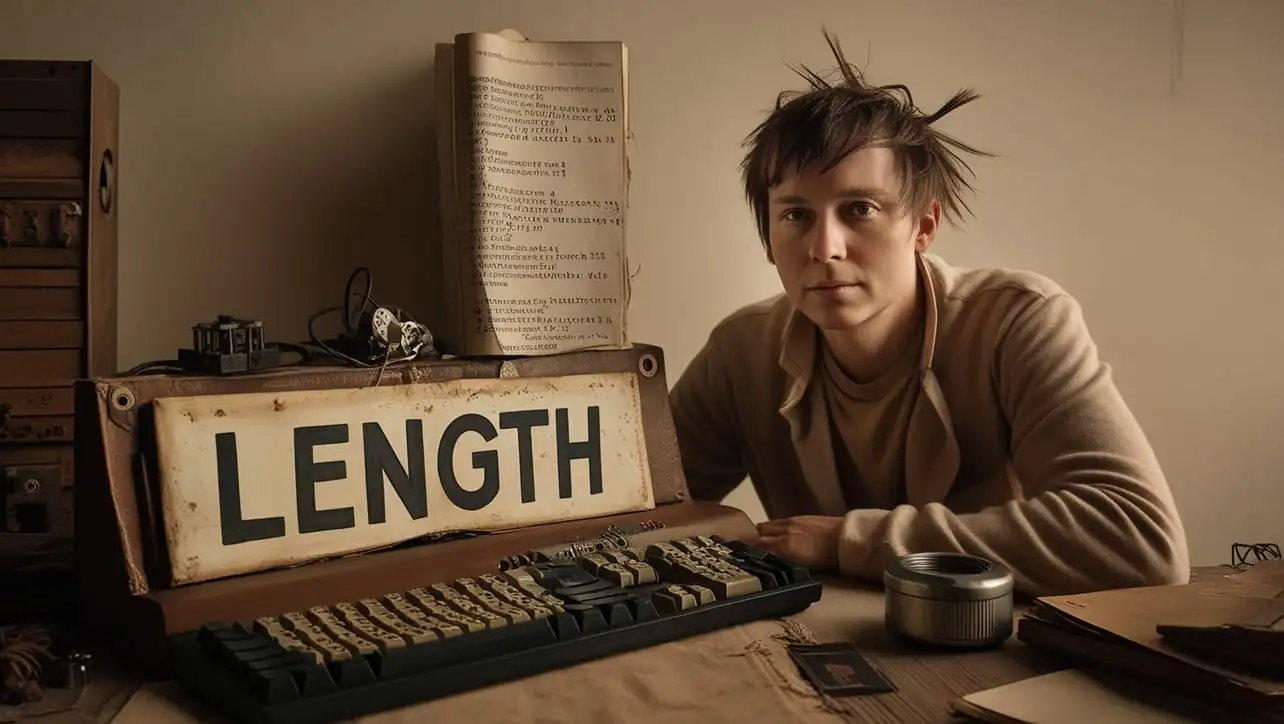
JS Topics
- JS Intro
- JS File Handling
- JS Array Methods
- at()
- concat()
- copyWithin()
- entries()
- every()
- fill()
- filter()
- find()
- findIndex()
- findLast()
- findLastIndex()
- flat()
- flatMap()
- forEach()
- from()
- includes()
- indexOf()
- isArray()
- join()
- keys()
- lastIndexOf()
- map()
- of()
- pop()
- push()
- reduce()
- reduceRight()
- reverse()
- shift()
- slice()
- some()
- sort()
- splice()
- toLocaleString()
- toString()
- unshift()
- valueOf()
- values()
- with()
- JS Console Methods
- JS Date Methods
- JS Navigator Methods
- JS Node Methods
- JS Number Methods
- JS String Properties
- JS String Methods
- JS Window Methods
- JS Cookies
- JS Interview Programs
- JS Star Pattern
- JS Number Pattern
- JS Alphabet Pattern
JavaScript Array unshift() Method
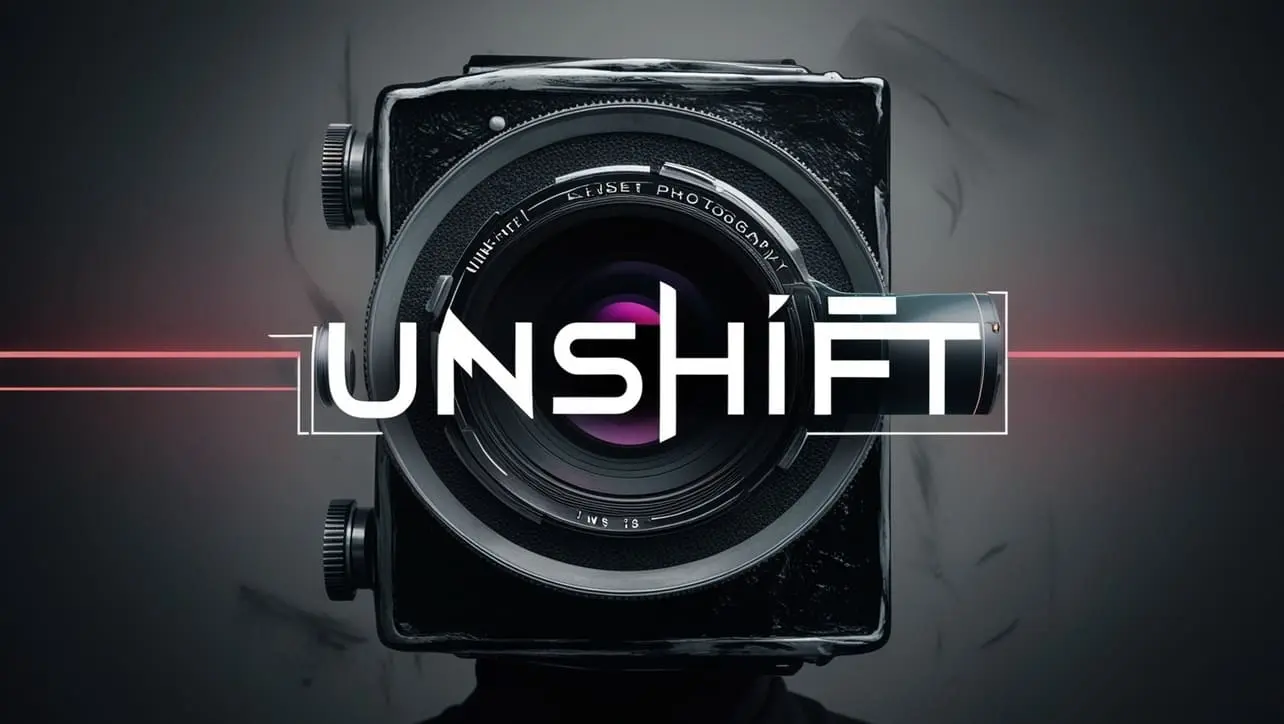
Photo Credit to CodeToFun
Introduction
JavaScript arrays provide a wealth of methods for manipulating their content, and the unshift()
method is a powerful addition to your array toolkit. This method allows you to effortlessly add one or more elements to the beginning of an array, efficiently repositioning existing elements.
In this guide, we'll explore the unshift()
method, covering its syntax, usage, best practices, and practical use cases.
Understanding unshift() Method
The unshift()
method is designed to prepend elements to the beginning of an array. Unlike push(), which adds elements to the end, unshift()
focuses on the array's front, shifting existing elements to accommodate the new additions.
Syntax
The syntax for the unshift()
method is straightforward:
array.unshift(element1, element2, ..., elementN);
- array: The array to which you want to add elements.
- element1, element2, ..., elementN: The elements to add to the beginning of the array.
Example
Let's dive into a simple example to illustrate the usage of the unshift()
method:
// Sample array
const numbers = [3, 4, 5];
// Using unshift() to add elements to the beginning
numbers.unshift(1, 2);
console.log(numbers); // Output: [1, 2, 3, 4, 5]
In this example, the unshift()
method adds elements 1 and 2 to the beginning of the numbers array.
Best Practices
When working with the unshift()
method, consider the following best practices:
Multiple Elements:
You can add multiple elements in a single
unshift()
call, improving performance and readability.example.jsCopiednumbers.unshift(-2, -1, 0); // Adds three elements to the beginning
Avoiding for...of:
While you can use for...of to iterate and add elements, it's less efficient than a direct
unshift()
call.example.jsCopied// Less efficient for (const num of [-2, -1, 0]) { numbers.unshift(num); } // More efficient numbers.unshift(-2, -1, 0);
Use Cases
Building a Queue:
The
unshift()
method is useful when implementing a queue data structure, where elements are added to the front and removed from the end.example.jsCopied// Queue implementation using unshift() and pop() const queue = []; queue.unshift('Task 3'); queue.unshift('Task 2'); queue.unshift('Task 1'); console.log(queue); // Output: ['Task 1', 'Task 2', 'Task 3'] // Process the tasks const currentTask = queue.pop(); console.log(`Processing: ${currentTask}`);
Dynamic Element Insertion:
You can dynamically insert elements at the beginning based on certain conditions or user interactions.
example.jsCopied// Dynamic element insertion const userPreference = 'dark'; if (userPreference === 'dark') { colors.unshift('black', 'gray'); } else { colors.unshift('white', 'beige'); }
Conclusion
The unshift()
method is a valuable tool for efficiently adding elements to the beginning of an array, enabling dynamic content manipulation.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the unshift()
method in your JavaScript projects.
Join our Community:
Author
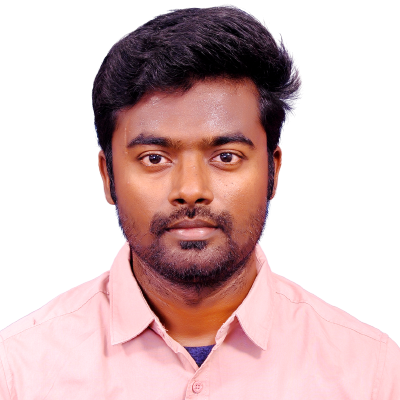
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (JavaScript Array unshift() Method), please comment here. I will help you immediately.