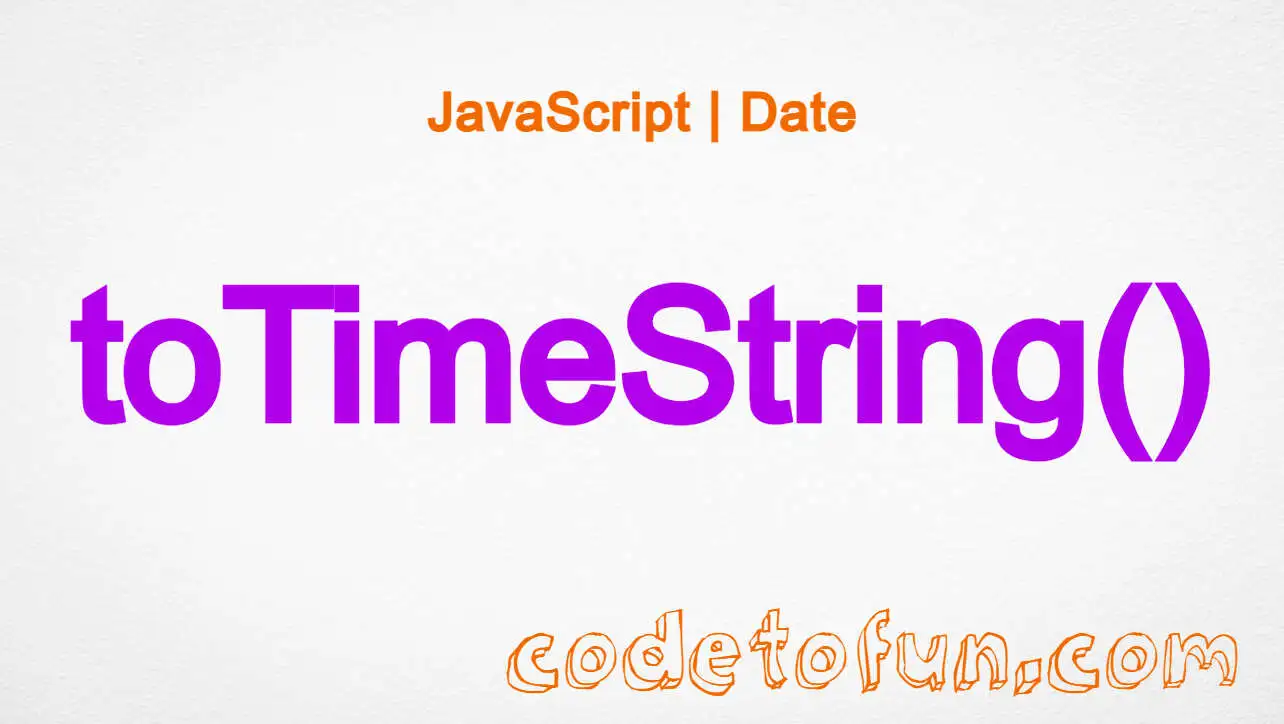
JS Array Methods
JavaScript Array Methods
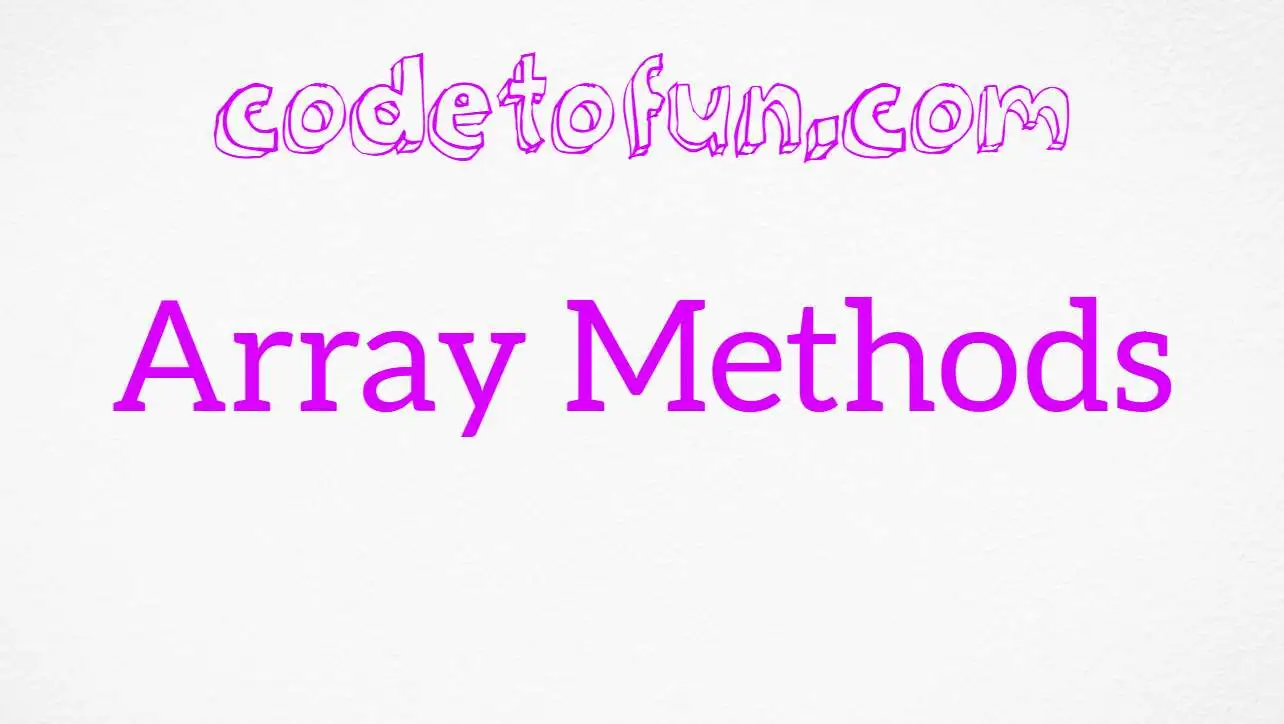
Photo Credit to CodeToFun
🙋 Introduction
JavaScript arrays are versatile data structures that allow you to store and manipulate collections of elements. JavaScript provides a variety of built-in methods for working with arrays, enabling you to perform operations such as adding, removing, and transforming elements.
In this reference guide, we'll explore some commonly used JavaScript array methods to enhance your skills in array manipulation.
📑 Table of Contents
Some of the commonly used array methods in JavaScript includes:
Methods | Explanation |
---|---|
at() | Retrieves an element at a specified index from an array. |
copyWithin() | Copies a sequence of elements within the array to another location, allowing for efficient in-place modification. |
concat() | Combines two or more arrays into a single array. |
entries() | Returns an iterator containing key/value pairs for each index in the array. |
every() | Tests whether all elements in an array pass a provided function, returning a Boolean value. |
fill() | Efficiently populates all elements of an array with a static value. |
filter() | Creates a new array with elements that pass a specified test function. |
find() | Returns the first array element that satisfies a provided testing function. |
findIndex() | Returns the index of the first array element that satisfies a testing function, providing a powerful way to locate elements based on custom criteria. |
findLast() | Returns the last element in an array that satisfies a provided testing function. |
findLastIndex() | Returns the index of the last array element that satisfies a provided testing function. |
flat() | Flattens nested arrays into a single-level array, simplifying array manipulation and traversal. |
flatMap() | Simultaneously maps and flattens arrays, applying a function to each element and then flattening the result into a new array. |
forEach() | Iterates over each element in an array, allowing the execution of a provided function for each element. |
from() | Creates a new array instance from an iterable object or array-like structure. |
includes() | Checks if an array contains a specified element and returns a boolean value, simplifying the search for values within an array. |
indexOf() | Returns the first index at which a specified element is found in an array, or -1 if the element is not present. |
isArray() | Checks whether a given value is an array, returning true if it is and false otherwise. |
join() | Converts all elements of an array into a string, concatenating them with a specified separator. |
keys() | Returns an iterator containing the keys for each index in the array. |
lastIndexOf() | Returns the last index at which a specified element is found in an array, searching backward from a specified index or the end of the array. |
map() | Transforms each element of an array using a provided function and creates a new array with the results. |
of() | Creates a new array instance with the provided elements, offering a concise way to initialize arrays irrespective of the data types of the elements. |
pop() | Removes the last element from an array and returns that element. |
push() | Adds one or more elements to the end of an array, modifying the array and returning the new length. |
reduce() | Iterates over an array, accumulating a single result by applying a specified function to each element. |
reduceRight() | Iterates over an array's elements from right to left, accumulating a single value by applying a provided function. |
reverse() | Reverses the elements of an array in place, altering the original array. |
shift() | Efficiently removes and returns the first element from an array, shifting the remaining elements to lower indices. |
slice() | Returns a shallow copy of a portion of an array, allowing for the extraction of elements based on specified start and end indices. |
some() | Checks if at least one element in the array satisfies a specified condition, returning true if any element passes the test. |
sort() | Used to arrange the elements of an array either alphabetically or numerically in ascending or descending order. |
splice() | Used to change the contents of an array by removing or replacing existing elements and/or adding new elements at a specified index. |
toLocaleString() | Converts elements of an array to strings using localized formats, such as currency or date, based on the user's locale. |
toString() | Converts an array to a string by joining its elements with commas. |
unshift() | Adds one or more elements to the beginning of an array, shifting existing elements to higher indexes. |
valueOf() | Returns the array itself, providing a convenient way to access the array's primitive value representation. |
with() | Creates a new array or converts an existing object into an array, providing a concise way to generate arrays. |
🚀 Usage Tips
Enhance your array manipulation skills with these tips:
Chaining Methods:
Combine multiple array methods to perform complex operations in a single line.
Callback Functions:
Leverage callback functions for custom logic in methods like filter, map, and reduce.
Immutable Operations:
Some methods, like map and filter, create new arrays without modifying the original array.
Method Parameters:
Explore optional parameters and their default values to customize method behavior.
📝 Example
Let's look at a practical example of using JavaScript array methods to manipulate an array of numbers:
// Example: Filtering even numbers and mapping to their squares
const numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10];
const evenSquares = numbers
.filter(num => num % 2 === 0)
.map(evenNum => evenNum ** 2);
console.log("Original Numbers:", numbers);
console.log("Filtered and Squared:", evenSquares);
This example showcases the use of filter to select even numbers and map to square each even number. Experiment with different methods and parameters to harness the power of JavaScript array methods.
🎉 Conclusion
This reference guide provides an overview of essential JavaScript array methods. By incorporating these methods into your code, you can efficiently manipulate and analyze arrays in various ways. Experiment with these methods to discover their versatility and enhance your JavaScript programming skills.
👨💻 Join our Community:
Author
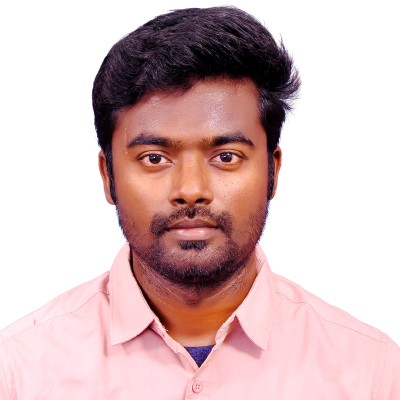
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (JavaScript Array Methods), please comment here. I will help you immediately.