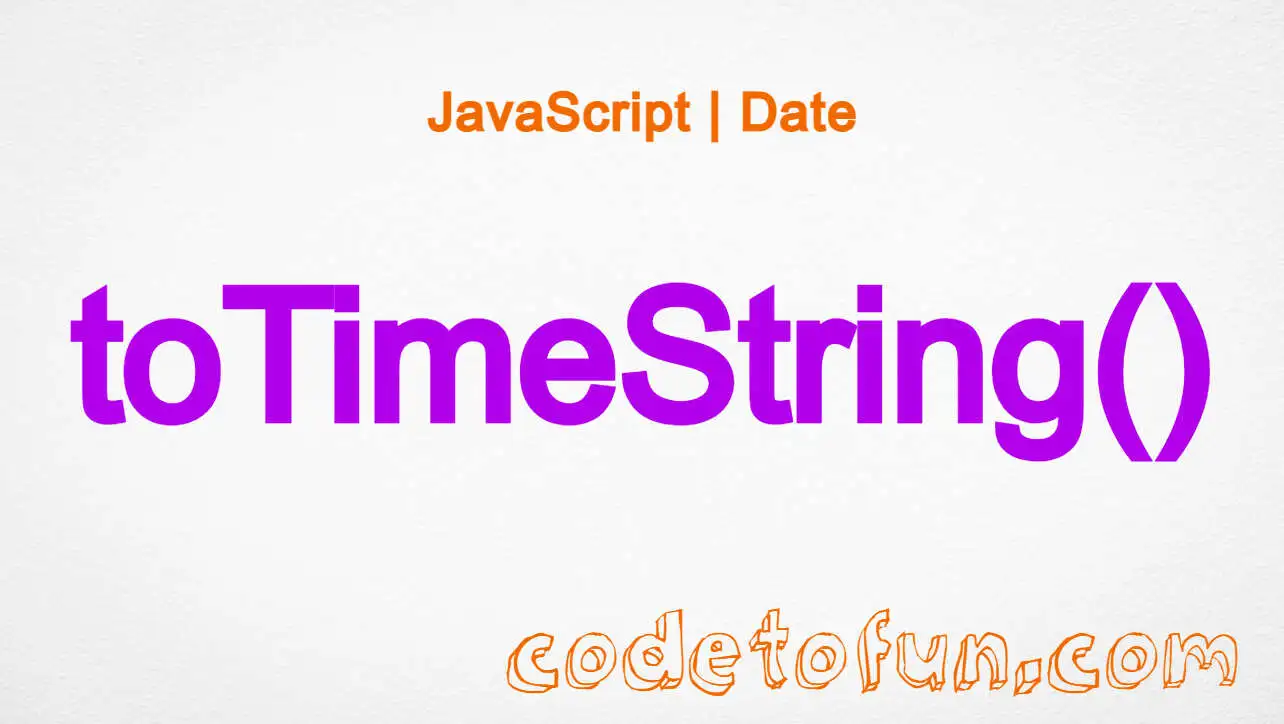
JS Array Methods
JavaScript Array concat() Method
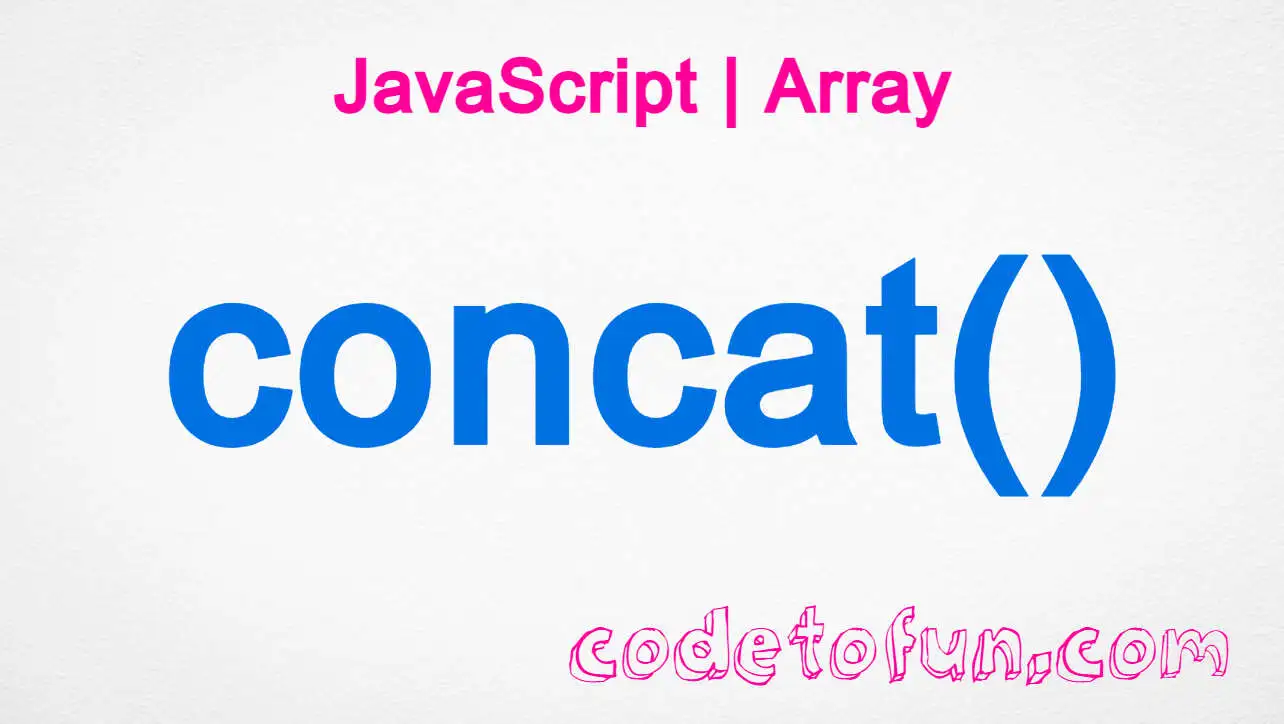
Photo Credit to CodeToFun
🙋 Introduction
JavaScript arrays offer a myriad of methods for efficient data manipulation, and the concat()
method is a powerful addition to this array toolkit. This method allows you to combine arrays, creating a new array without modifying the original ones.
In this comprehensive guide, we'll delve into the concat()
method, exploring its syntax, practical examples, best practices, and various use cases.
🧠 Understanding concat() Method
The concat()
method is employed to merge two or more arrays, producing a new array that encapsulates the elements of the original arrays. Unlike other array methods that mutate the existing array, concat()
returns a new array, leaving the source arrays unchanged.
💡 Syntax
The syntax for the concat()
method is straightforward:
const newArray = array1.concat(array2, array3, ..., arrayN);
- array1, array2, ..., arrayN: Arrays to be concatenated.
- newArray: The resulting array containing elements from all concatenated arrays.
📝 Example
Let's illustrate the usage of the concat()
method with a practical example:
// Sample arrays
const fruits1 = ['apple', 'orange'];
const fruits2 = ['banana', 'grape'];
const moreFruits = ['kiwi', 'mango'];
// Using concat() to merge arrays
const mergedFruits = fruits1.concat(fruits2, moreFruits);
console.log(mergedFruits);
// Output: ['apple', 'orange', 'banana', 'grape', 'kiwi', 'mango']
In this example, the concat()
method is used to combine three arrays (fruits1, fruits2, and moreFruits) into a new array called mergedFruits.
🏆 Best Practices
When working with the concat()
method, consider the following best practices:
Immutability:
Leverage the immutability of
concat()
to avoid unintended side effects. Since it returns a new array, the original arrays remain unchanged.example.jsCopiedconst combinedArray = array1.concat(array2); // original arrays (array1 and array2) are unaltered
Multiple Arrays:
Concatenate multiple arrays by including them as arguments, allowing for the creation of a single merged array.
example.jsCopiedconst mergedArray = array1.concat(array2, array3);
📚 Use Cases
Creating a Copy of an Array:
The
concat()
method is often used to create a shallow copy of an array, providing a convenient alternative to methods like slice().example.jsCopiedconst originalArray = [1, 2, 3]; const copiedArray = [].concat(originalArray); console.log(copiedArray); // Output: [1, 2, 3]
Merging Arrays Dynamically:
Dynamically merge arrays based on conditions, such as user input or application state:
example.jsCopiedconst selectedFruits = ['apple', 'banana']; const additionalFruits = ['kiwi', 'mango']; const finalFruits = selectedFruits.concat( // Include additional fruits based on a condition shouldIncludeMango ? additionalFruits : [] ); console.log(finalFruits);
In this example, the
concat()
method is used to merge arrays based on a condition.
🎉 Conclusion
The concat()
method is a valuable tool for array manipulation in JavaScript. Its ability to merge arrays without modifying the originals, combined with its versatility in handling multiple arrays, makes it a practical choice for a variety of scenarios
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the concat()
method in your JavaScript projects.
👨💻 Join our Community:
Author
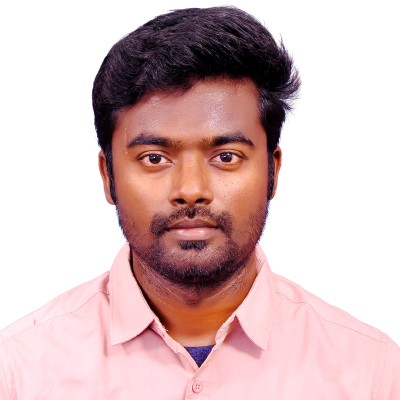
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (JavaScript Array concat() Method), please comment here. I will help you immediately.