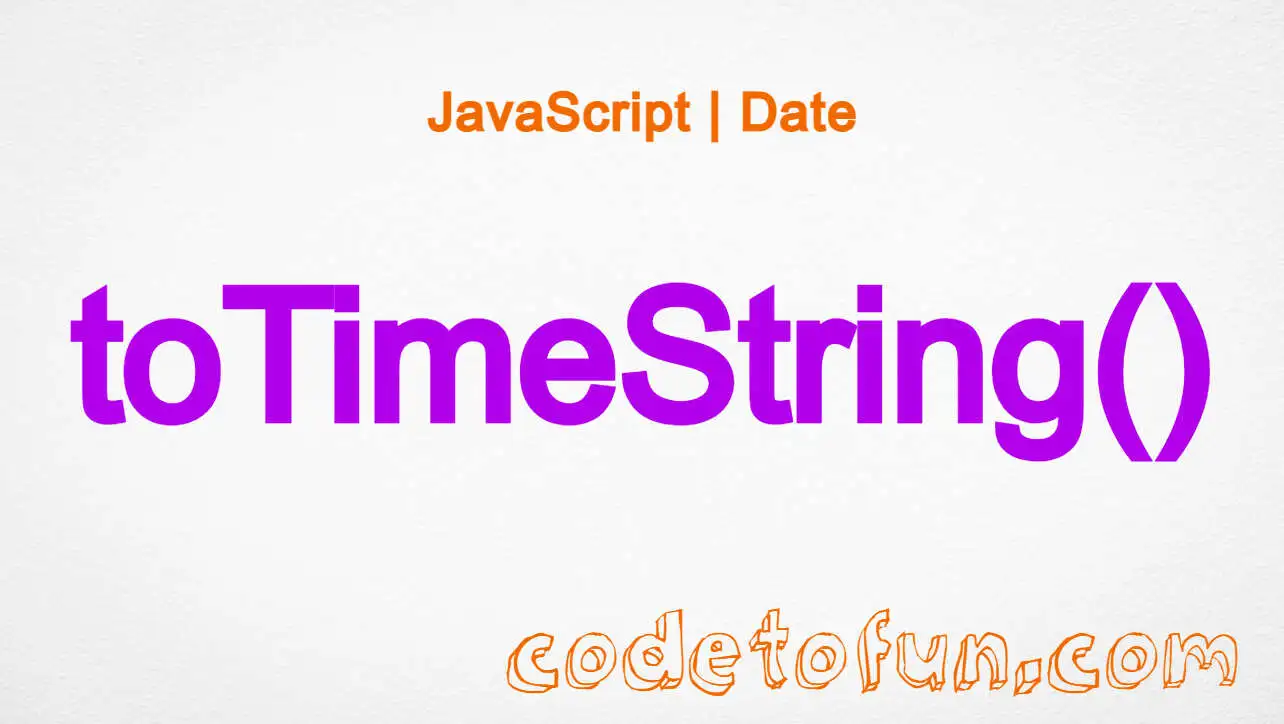
JS Array Methods
JavaScript Array push() Method
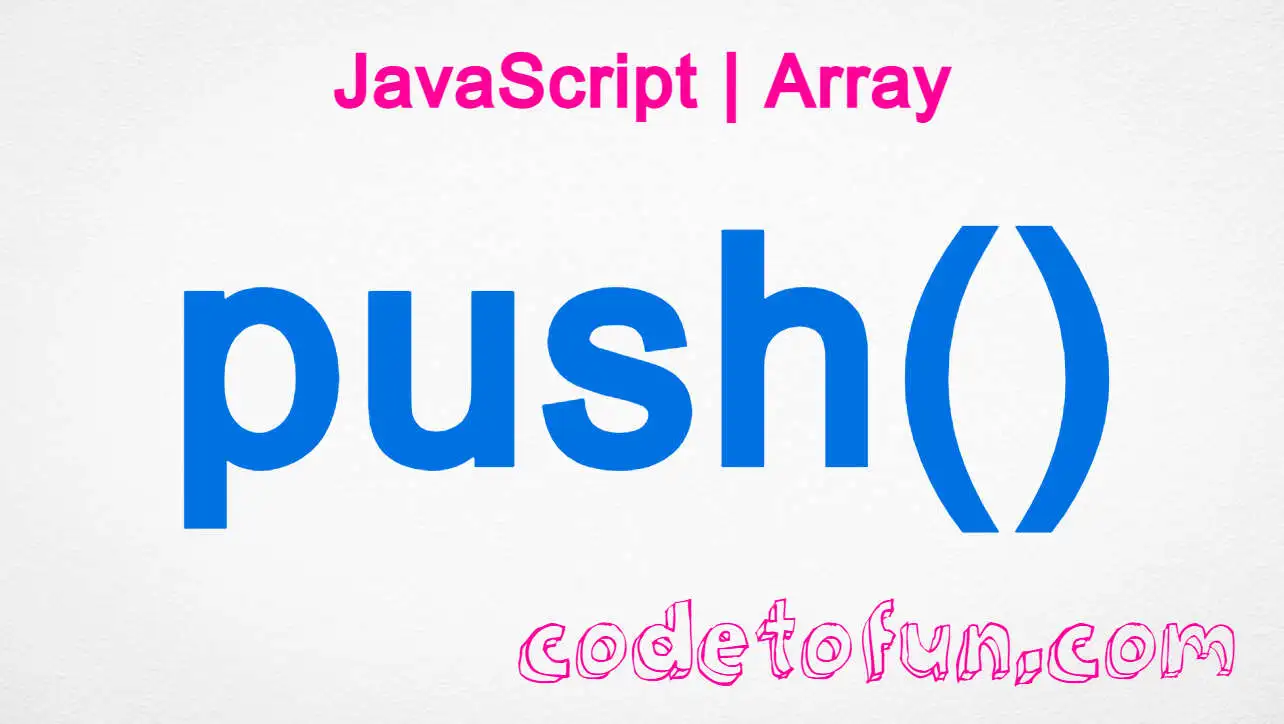
Photo Credit to CodeToFun
🙋 Introduction
JavaScript arrays are the backbone of data manipulation, and the push()
method is a fundamental tool for adding elements to the end of an array.
In this comprehensive guide, we'll explore the push()
method, examining its syntax, applications, best practices, and real-world use cases.
🧠 Understanding push() Method
The push()
method in JavaScript is used to add one or more elements to the end of an array. This method is simple yet powerful, enabling dynamic expansion of arrays during runtime.
💡 Syntax
The syntax for the push()
method is straightforward:
array.push(element1, element2, ..., elementN);
- array: The array to which elements will be added.
- element1, element2, ..., elementN: Elements to be added to the end of the array.
📝 Example
Let's dive into a basic example to illustrate the simplicity of the push()
method:
// Sample array
const numbers = [1, 2, 3];
// Using push() to add elements
numbers.push(4, 5);
console.log(numbers); // Output: [1, 2, 3, 4, 5]
In this example, the push()
method appends the values 4 and 5 to the end of the numbers array.
🏆 Best Practices
When working with the push()
method, consider the following best practices:
Chaining:
Leverage method chaining to perform multiple
push()
operations in a concise manner.example.jsCopiedconst fruits = ['apple', 'orange']; // Chaining push() for multiple elements fruits.push('banana', 'grape').push('kiwi'); console.log(fruits); // Output: ['apple', 'orange', 'banana', 'grape', 'kiwi']
Dynamic Element Addition:
Utilize variables or dynamically generated values as arguments for the
push()
method to enhance flexibility.example.jsCopiedconst newFruit = 'pineapple'; fruits.push(newFruit);
📚 Use Cases
Building Dynamic Lists:
The
push()
method is ideal for dynamically constructing lists based on user input or data retrieved from external sources:example.jsCopiedconst userPreferences = []; // User selects preferences dynamically userPreferences.push('Dark Mode', 'Notifications', 'High Contrast'); console.log(userPreferences); // Output: ['Dark Mode', 'Notifications', 'High Contrast']
Concatenating Arrays:
You can use
push()
to concatenate arrays, merging their elements into a single array:example.jsCopiedconst vegetables = ['carrot', 'broccoli']; // Concatenating arrays using push() fruits.push(...vegetables); console.log(fruits); // Output: ['apple', 'orange', 'banana', 'grape', 'kiwi', 'carrot', 'broccoli']
🎉 Conclusion
The push()
method is a cornerstone of array manipulation in JavaScript, providing a straightforward way to expand arrays dynamically.
By adhering to best practices and exploring diverse use cases, you can harness the full potential of the push()
method in your JavaScript projects.
👨💻 Join our Community:
Author
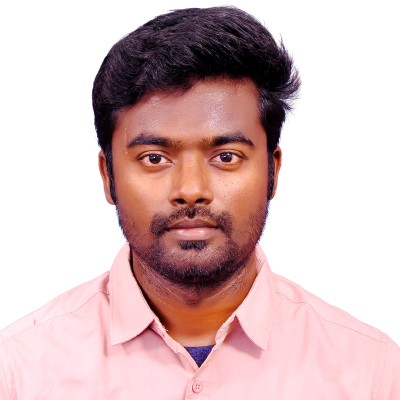
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (JavaScript Array push() Method), please comment here. I will help you immediately.