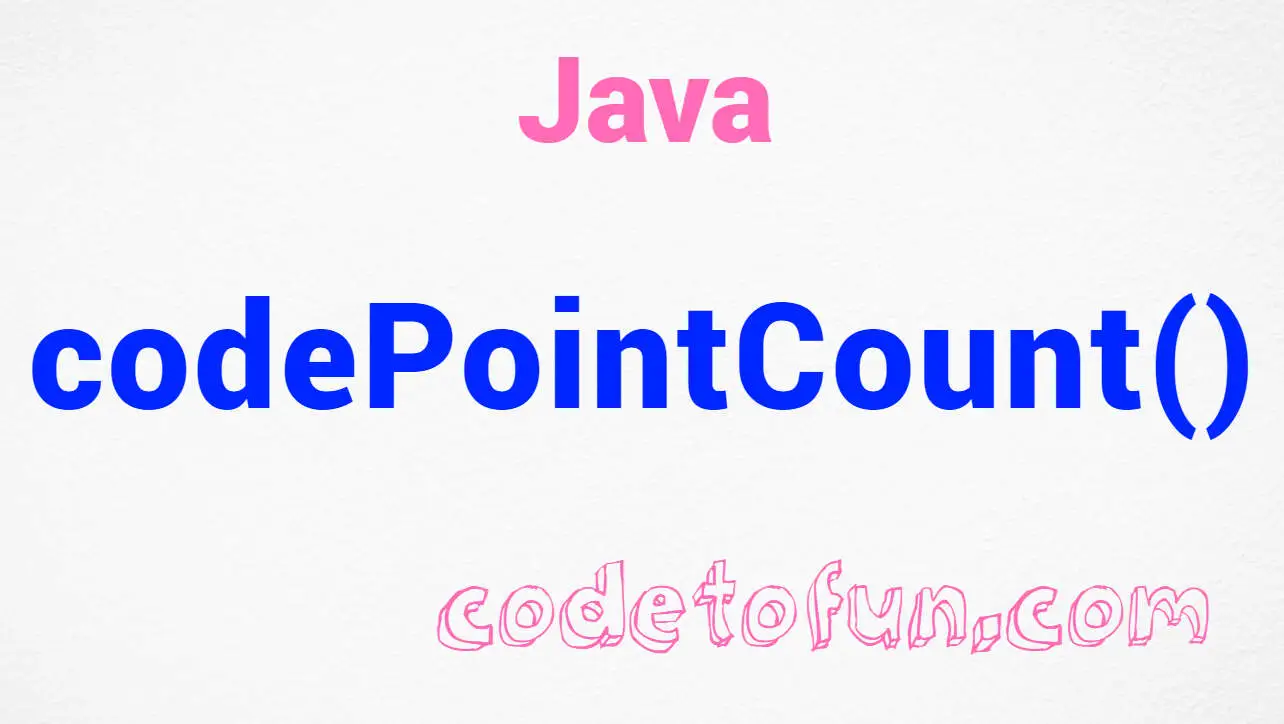
Java Basic
Java Interview Programs
- Java Interview Programs
- Java Abundant Number
- Java Amicable Number
- Java Armstrong Number
- Java Average of N Numbers
- Java Automorphic Number
- Java Biggest of three numbers
- Java Binary to Decimal
- Java Common Divisors
- Java Composite Number
- Java Condense a Number
- Java Cube Number
- Java Decimal to Binary
- Java Decimal to Octal
- Java Disarium Number
- Java Even Number
- Java Evil Number
- Java Factorial of a Number
- Java Fibonacci Series
- Java GCD
- Java Happy Number
- Java Harshad Number
- Java LCM
- Java Leap Year
- Java Magic Number
- Java Matrix Addition
- Java Matrix Division
- Java Matrix Multiplication
- Java Matrix Subtraction
- JS Matrix Transpose
- Java Maximum Value of an Array
- Java Minimum Value of an Array
- Java Multiplication Table
- Java Natural Number
- Java Number Combination
- Java Odd Number
- Java Palindrome Number
- Java Pascalβs Triangle
- Java Perfect Number
- Java Perfect Square
- Java Power of 2
- Java Power of 3
- Java Pronic Number
- Java Prime Factor
- Java Prime Number
- Java Smith Number
- Java Strong Number
- Java Sum of Array
- Java Sum of Digits
- Java Swap Two Numbers
- Java Triangular Number
Java Program to Check Harshad Number
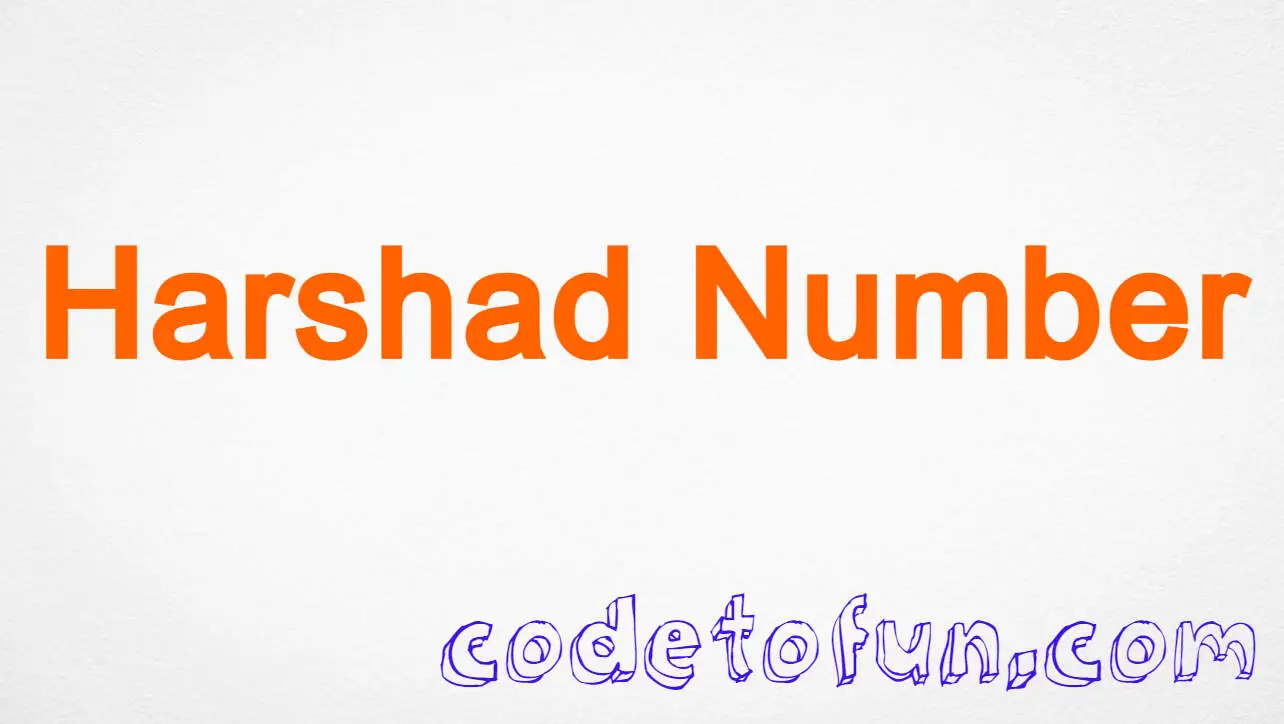
Photo Credit to CodeToFun
π Introduction
In the realm of programming, a Harshad number (or Niven number) is a positive integer that is divisible by the sum of its digits. Checking for Harshad numbers involves finding the sum of the digits and determining if the original number is divisible by this sum.
In this tutorial, we will explore a Java program designed to check whether a given number is a Harshad number.
π Example
Let's delve into the Java code that accomplishes this task.
import java.util.Scanner;
public class HarshadNumberChecker {
// Function to check if a number is a Harshad number
static boolean isHarshadNumber(int number) {
int originalNumber = number;
int sumOfDigits = 0;
// Calculate the sum of digits
while (number > 0) {
sumOfDigits += number % 10;
number /= 10;
}
// If the original number is divisible by the sum of digits, it is a Harshad number
return (originalNumber % sumOfDigits == 0);
}
// Driver program
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
// Replace this value with the number you want to check
System.out.print("Enter a number: ");
int number = scanner.nextInt();
// Call the function to check if the number is a Harshad number
if (isHarshadNumber(number)) {
System.out.println(number + " is a Harshad number.");
} else {
System.out.println(number + " is not a Harshad number.");
}
}
}
π» Testing the Program
To test the program with different numbers, replace the value of number with the desired number in the main method.
Enter a number: 18 18 is a Harshad number.
π§ How the Program Works
- The program defines a class HarshadNumberChecker containing a static method isHarshadNumber that takes an integer number as input and returns true if the number is a Harshad number, and false otherwise.
- Inside the main method, the program reads an integer from the user to check if it is a Harshad number.
- The program calls the isHarshadNumber method and prints the result.
π Between the Given Range
Let's delve into the java code that checks for Harshad numbers in the specified range.
public class HarshadNumberChecker {
// Function to check if a number is a Harshad number
static boolean isHarshad(int num) {
int sumOfDigits = 0;
int originalNum = num;
// Calculate the sum of digits
while (num > 0) {
sumOfDigits += num % 10;
num /= 10;
}
// Check if the original number is divisible by the sum of its digits
return (originalNum % sumOfDigits == 0);
}
// Driver program
public static void main(String[] args) {
System.out.println("Harshad numbers in the range 1 to 20: ");
for (int i = 1; i <= 20; i++) {
if (isHarshad(i)) {
System.out.print(i + " ");
}
}
}
}
π» Testing the Program
Harshad numbers in the range 1 to 20: 1 2 3 4 5 6 7 8 9 10 12 18 20
Compile and run the program to see the Harshad numbers in the specified range.
π§ How the Program Works
- The program defines a class HarshadNumberChecker containing a static method isHarshad that checks if a number is a Harshad number.
- Inside the method, it calculates the sum of digits and checks if the original number is divisible by the sum.
- The main function tests the Harshad numbers in the range 1 to 20 and prints the results.
π§ Understanding the Concept of Harshad Number
Before delving into the code, let's understand the concept of Harshad numbers. A Harshad number is a positive integer that is divisible by the sum of its digits.
π’ Optimizing the Program
While the provided program is effective, consider exploring and implementing alternative approaches or optimizations for checking Harshad numbers.
While the provided program is effective, consider exploring and implementing alternative approaches or optimizations for checking Harshad numbers.
π¨βπ» Join our Community:
Author
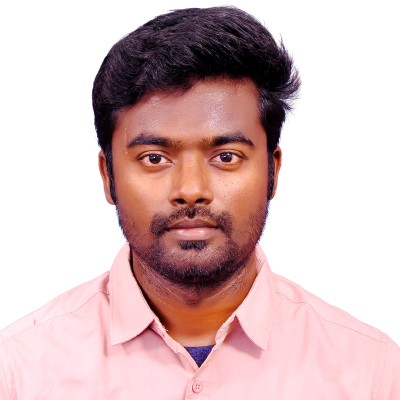
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (Java Program to Check Harshad Number), please comment here. I will help you immediately.