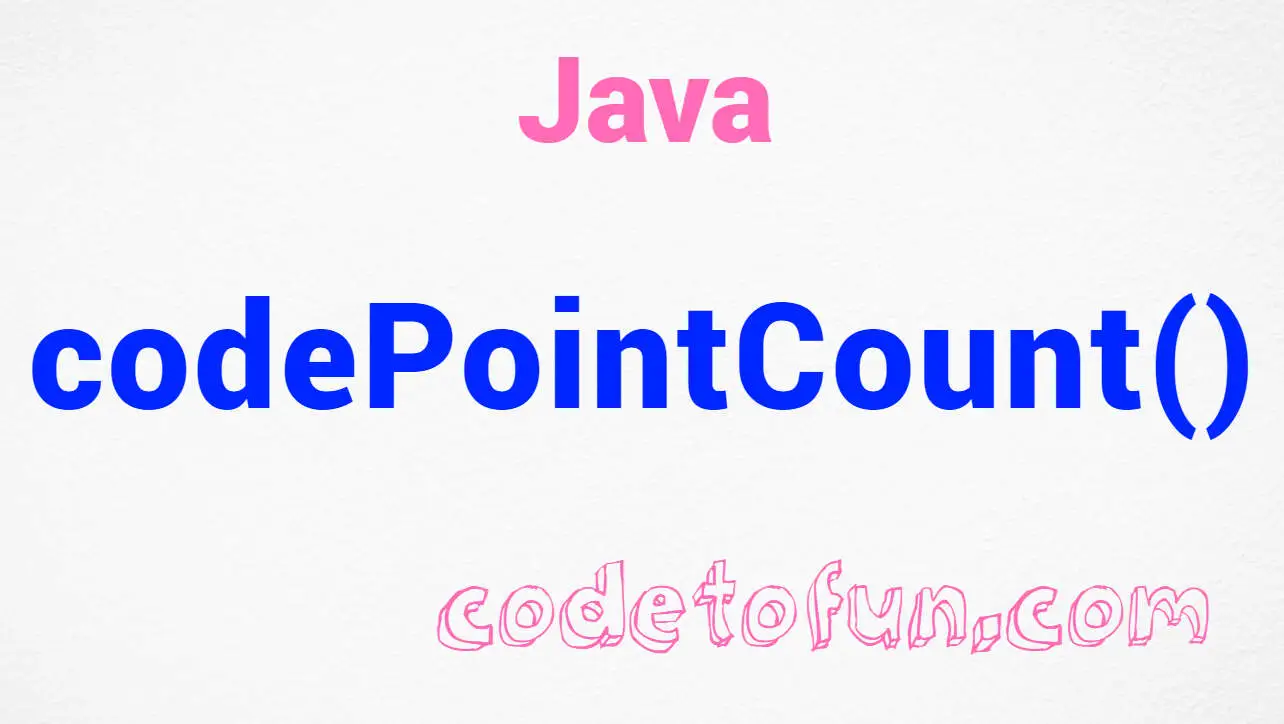
Java Basic
Java Interview Programs
- Java Interview Programs
- Java Abundant Number
- Java Amicable Number
- Java Armstrong Number
- Java Average of N Numbers
- Java Automorphic Number
- Java Biggest of three numbers
- Java Binary to Decimal
- Java Common Divisors
- Java Composite Number
- Java Condense a Number
- Java Cube Number
- Java Decimal to Binary
- Java Decimal to Octal
- Java Disarium Number
- Java Even Number
- Java Evil Number
- Java Factorial of a Number
- Java Fibonacci Series
- Java GCD
- Java Happy Number
- Java Harshad Number
- Java LCM
- Java Leap Year
- Java Magic Number
- Java Matrix Addition
- Java Matrix Division
- Java Matrix Multiplication
- Java Matrix Subtraction
- JS Matrix Transpose
- Java Maximum Value of an Array
- Java Minimum Value of an Array
- Java Multiplication Table
- Java Natural Number
- Java Number Combination
- Java Odd Number
- Java Palindrome Number
- Java Pascalβs Triangle
- Java Perfect Number
- Java Perfect Square
- Java Power of 2
- Java Power of 3
- Java Pronic Number
- Java Prime Factor
- Java Prime Number
- Java Smith Number
- Java Strong Number
- Java Sum of Array
- Java Sum of Digits
- Java Swap Two Numbers
- Java Triangular Number
Java Program to Converter a Decimal to Octal
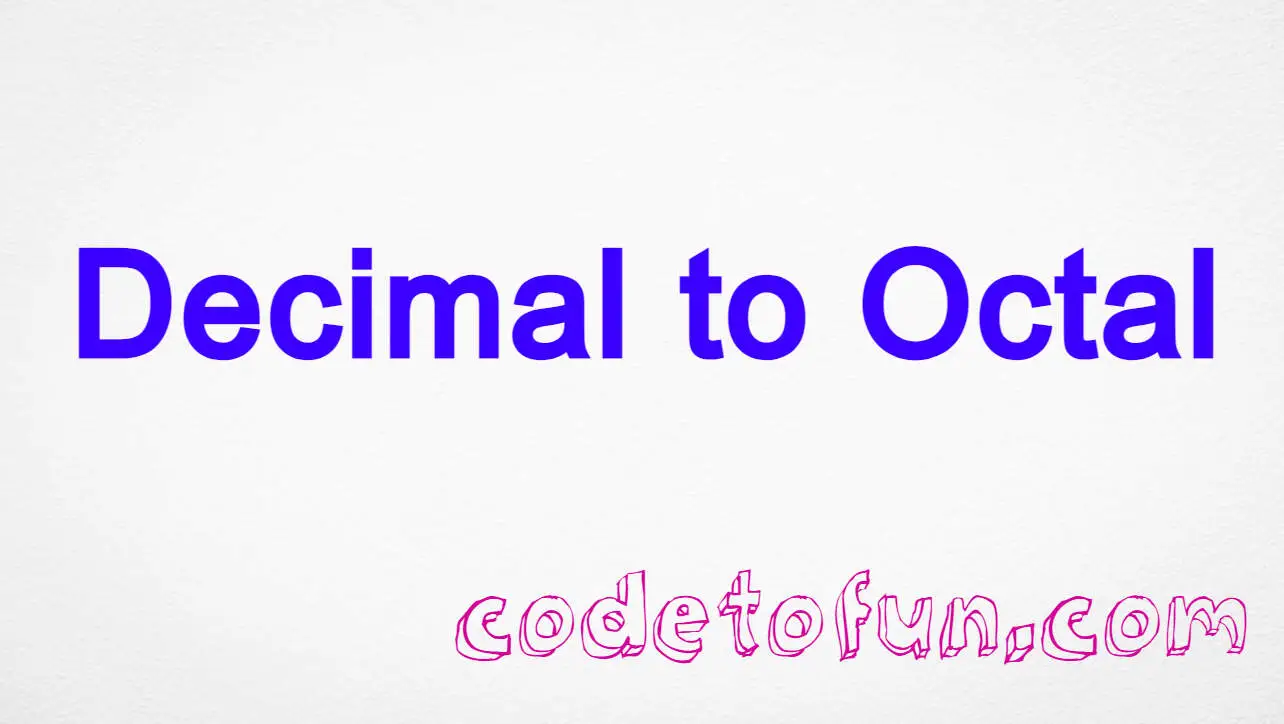
Photo Credit to CodeToFun
π Introduction
In the world of programming, converting numbers from one base to another is a common task.
Decimal to octal conversion is a fundamental operation where we transform a decimal (base-10) number into its octal (base-8) equivalent.
In this tutorial, we'll explore a java program that performs this conversion efficiently.
π Example
Let's delve into the java code that performs the decimal to octal conversion.
public class DecimalToOctal {
// Function to convert decimal to octal
static void decimalToOctal(int decimalNumber) {
int[] octalNumber = new int[100];
int i = 0;
// Convert decimal to octal
while (decimalNumber != 0) {
octalNumber[i] = decimalNumber % 8;
decimalNumber = decimalNumber / 8;
++i;
}
// Display the octal number in reverse order
System.out.print("Octal equivalent: ");
for (int j = i - 1; j >= 0; --j) {
System.out.print(octalNumber[j]);
}
System.out.println();
}
// Driver program
public static void main(String[] args) {
// Replace this value with your desired decimal number
int decimalNumber = 57;
// Call the function to convert decimal to octal
decimalToOctal(decimalNumber);
}
}
π» Testing the Program
To test the program with different decimal numbers, replace the value of decimalNumber in the main function.
Octal equivalent: 71
Compile and run the program to see the octal equivalent.
π§ How the Program Works
- The program defines a class DecimalToOctal containing a static method decimalToOctal that takes a decimal number as input and converts it to octal.
- Inside the method, it uses a while loop to repeatedly divide the decimal number by 8 and store the remainders in an array.
- The method then displays the octal equivalent by printing the elements of the array in reverse order.
π§ Understanding the Concept of Decimal to Octal
Decimal and octal are different number systems. Decimal uses base-10 (0 to 9), while octal uses base-8 (0 to 7). The process of converting a decimal number to octal involves dividing the decimal number by 8 repeatedly and noting the remainders in reverse order.
For example, let's convert the decimal number 57 to octal:
57 Γ· 8 = 7 remainder 1 7 Γ· 8 = 0 remainder 7
So, the octal equivalent is 71.
π’ Enhancements to the Program
Consider exploring enhancements to the program, such as handling negative decimal numbers, input validation, or optimizing the conversion process.
Feel free to incorporate and modify this code as needed for your specific use case. Happy coding!
π¨βπ» Join our Community:
Author
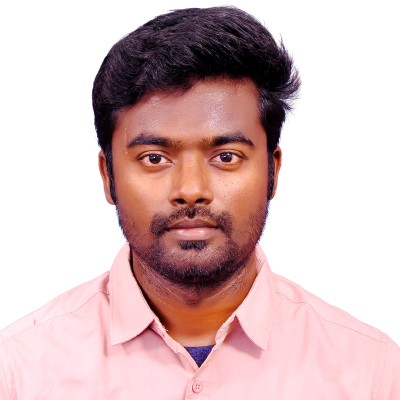
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (Java Program to Converter a Decimal to Octal), please comment here. I will help you immediately.