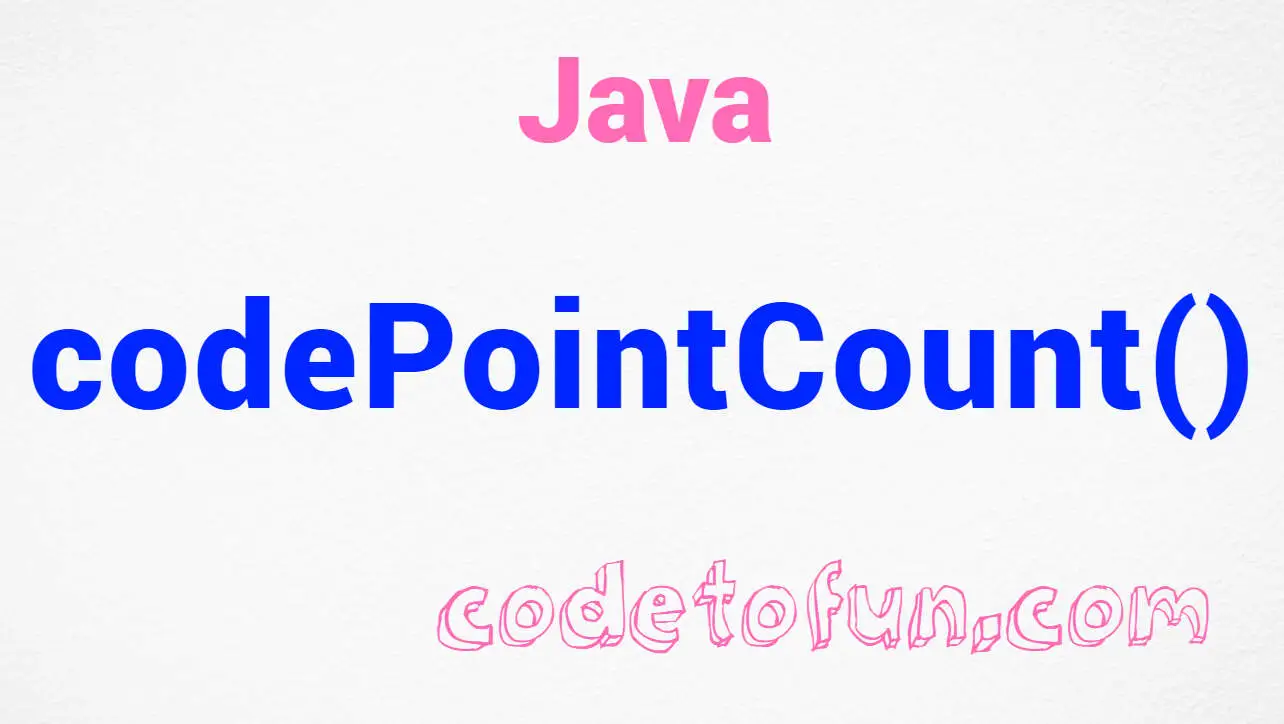
Java Basic
Java Interview Programs
- Java Interview Programs
- Java Abundant Number
- Java Amicable Number
- Java Armstrong Number
- Java Average of N Numbers
- Java Automorphic Number
- Java Biggest of three numbers
- Java Binary to Decimal
- Java Common Divisors
- Java Composite Number
- Java Condense a Number
- Java Cube Number
- Java Decimal to Binary
- Java Decimal to Octal
- Java Disarium Number
- Java Even Number
- Java Evil Number
- Java Factorial of a Number
- Java Fibonacci Series
- Java GCD
- Java Happy Number
- Java Harshad Number
- Java LCM
- Java Leap Year
- Java Magic Number
- Java Matrix Addition
- Java Matrix Division
- Java Matrix Multiplication
- Java Matrix Subtraction
- JS Matrix Transpose
- Java Maximum Value of an Array
- Java Minimum Value of an Array
- Java Multiplication Table
- Java Natural Number
- Java Number Combination
- Java Odd Number
- Java Palindrome Number
- Java Pascalβs Triangle
- Java Perfect Number
- Java Perfect Square
- Java Power of 2
- Java Power of 3
- Java Pronic Number
- Java Prime Factor
- Java Prime Number
- Java Smith Number
- Java Strong Number
- Java Sum of Array
- Java Sum of Digits
- Java Swap Two Numbers
- Java Triangular Number
Java Program to Converter a Decimal to Binary
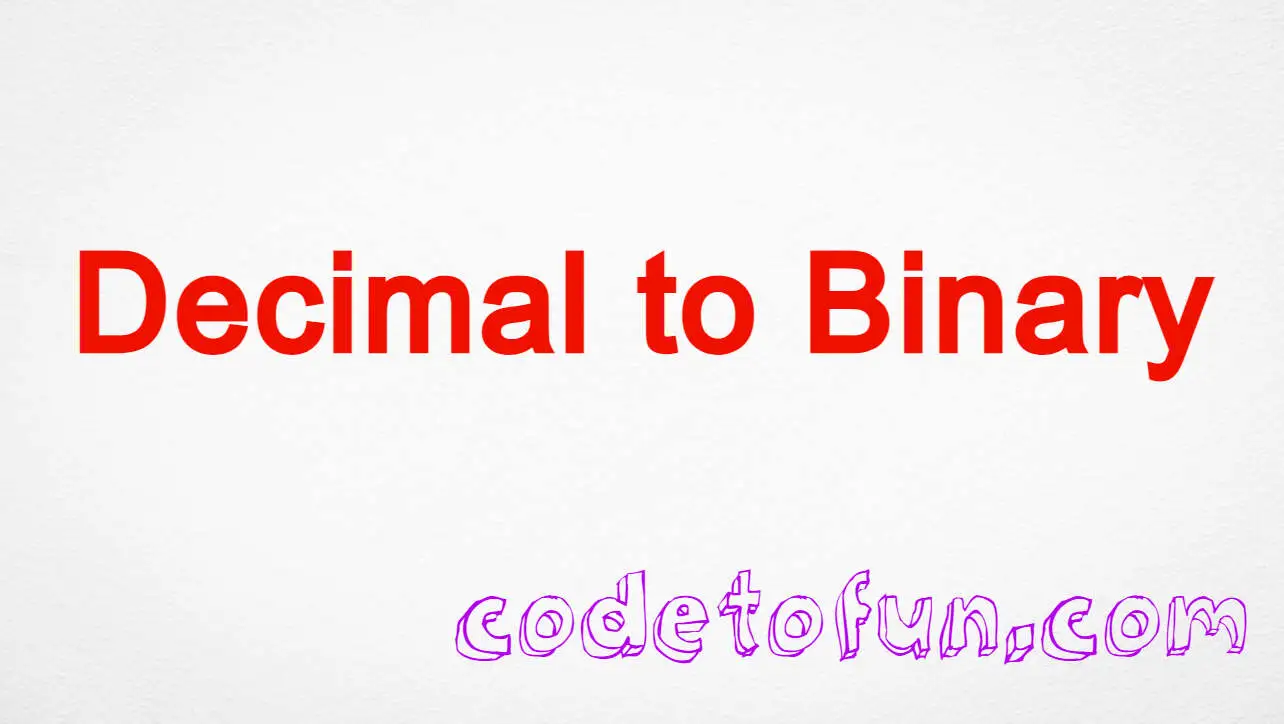
Photo Credit to CodeToFun
π Introduction
In the world of programming, converting numbers from one base to another is a common and essential task. One interesting conversion is from decimal to binary. Decimal is the base-10 number system, and binary is the base-2 system.
Converting a decimal number to its binary equivalent involves representing the decimal value using only the digits 0 and 1.
In this tutorial, we'll explore a java program that efficiently converts a decimal number to its binary representation.
π Example
Let's dive into the java code that achieves this functionality.
public class DecimalToBinary {
// Function to convert decimal to binary
static void decimalToBinary(int decimalNumber) {
int[] binaryNumber = new int[32]; // To store binary digits
int i = 0;
// Convert decimal to binary
while (decimalNumber > 0) {
binaryNumber[i] = decimalNumber % 2;
decimalNumber = decimalNumber / 2;
i++;
}
// Print binary number in reverse order
System.out.print("Binary equivalent: ");
for (int j = i - 1; j >= 0; j--) {
System.out.print(binaryNumber[j]);
}
System.out.println();
}
// Driver program
public static void main(String[] args) {
// Replace this value with your desired decimal number
int decimalNumber = 15;
// Call the function to convert decimal to binary
decimalToBinary(decimalNumber);
}
}
π» Testing the Program
To test the program with a different decimal number, simply replace the value of decimalNumber in the main function.
Binary equivalent: 1111
Compile and run the program to see the binary equivalent.
π§ How the Program Works
- The program defines a class DecimalToBinary containing a static method decimalToBinary that takes a decimal number as input and prints its binary equivalent.
- Inside the method, it uses an array (binaryNumber) to store the binary digits.
- It iterates through the process of dividing the decimal number by 2 and storing the remainder until the decimal number becomes 0.
- The binary digits are stored in reverse order in the array.
- Finally, the binary equivalent is printed.
π§ Understanding the Concept of Decimal to Binary
Before delving into the code, let's briefly understand the binary number system. In binary, each digit represents a power of 2, starting from the rightmost digit as 2^0, then 2^1, 2^2, and so on.
Converting a decimal number to binary involves repeatedly dividing the decimal number by 2 and recording the remainders in reverse order.
For example, the decimal number 15 is equivalent to the binary number 1111.
π’ Optimizing the Program
While the provided program is effective, consider exploring and implementing optimizations for handling larger decimal numbers.
Feel free to incorporate and modify this code as needed for your specific use case. Happy coding!
π¨βπ» Join our Community:
Author
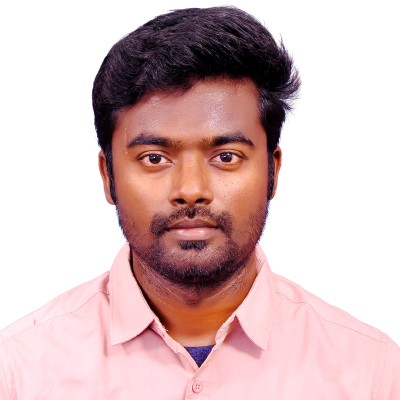
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (Java Program to Converter a Decimal to Binary), please comment here. I will help you immediately.