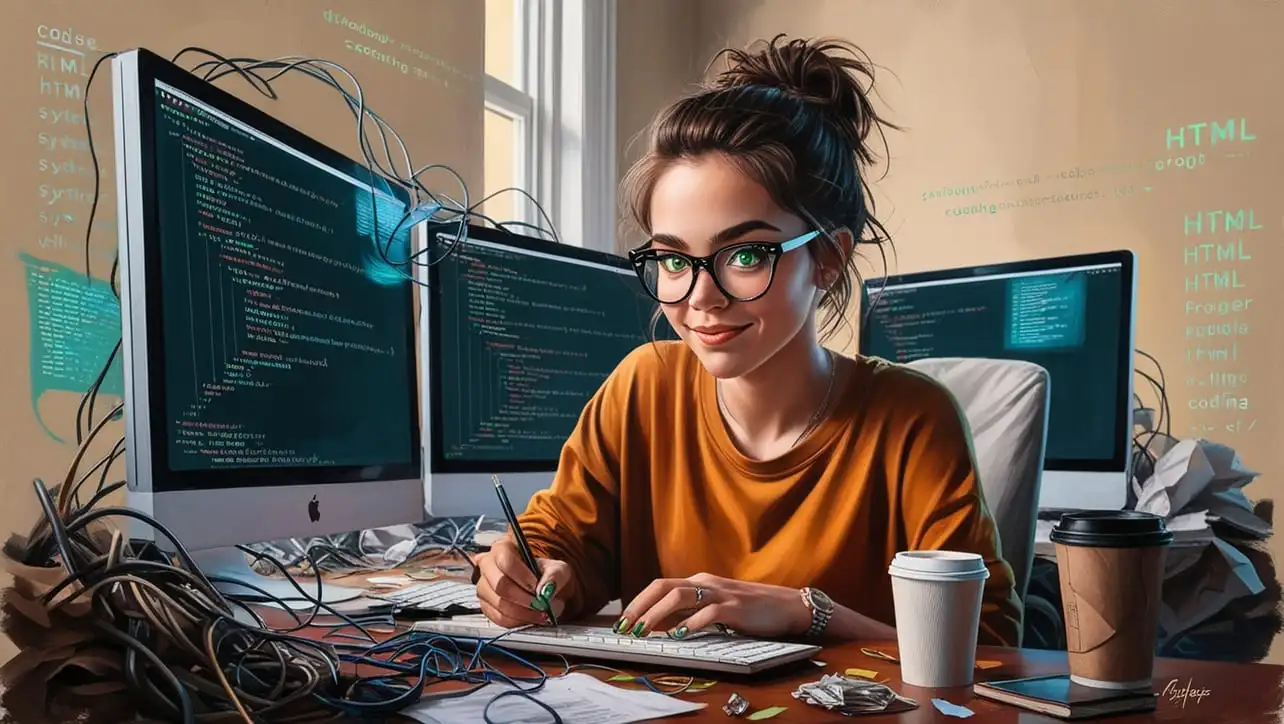
HTML Basic
HTML Entity
- HTML Entity
- HTML Alphabet Entity
- HTML Arrow Entity
- HTML Currency Entity
- HTML Math Entity
- HTML Number Entity
- HTML Punctuation Entity
- HTML Symbol Entity
HTML IndexedDB
HTML Reference
HTML Server-Sent Events
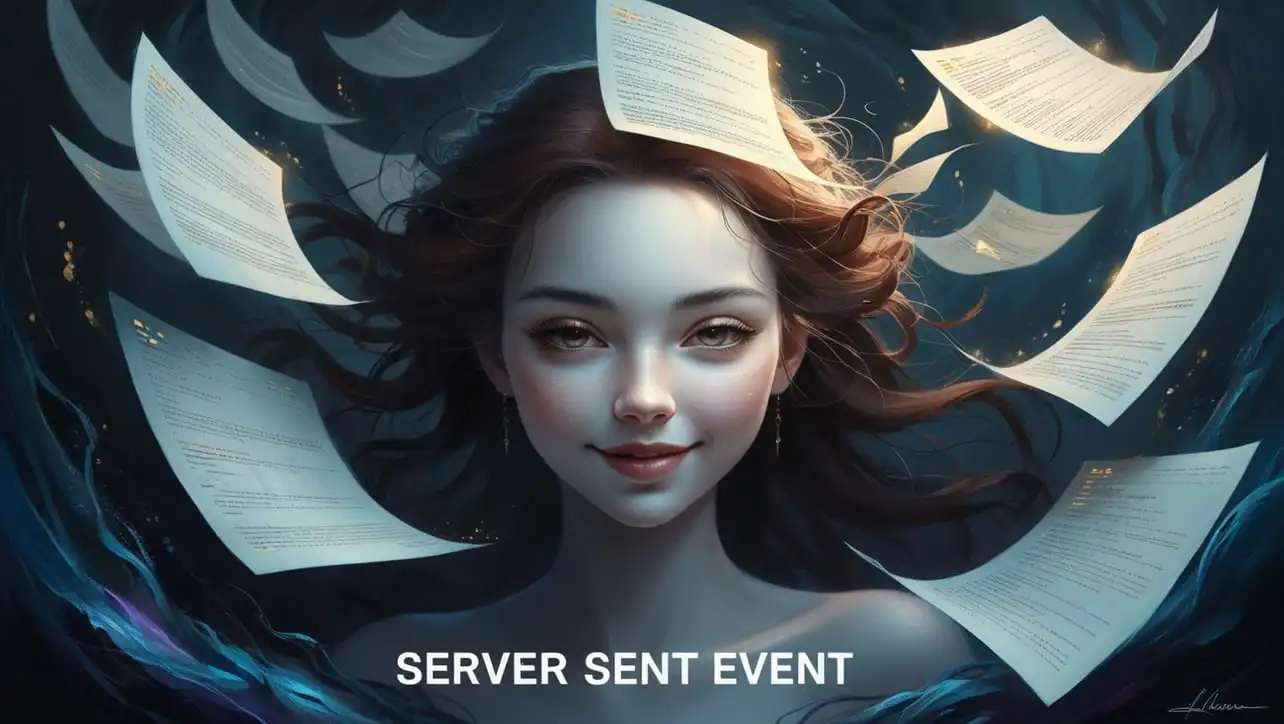
Photo Credit to CodeToFun
π Introduction
Server-Sent Events (SSE) is a technology that allows a server to push updates to a web application over a single, long-lived HTTP connection.
This is particularly useful for real-time updates, such as news feeds, live sports scores, or stock market data. SSE is a simple yet powerful alternative to other real-time communication methods like WebSockets.
β What Are Server-Sent Events?
Server-Sent Events (SSE) is a web technology where the server sends updates to the client as they happen, without the client explicitly requesting them. This allows for real-time data streams from the server to the client, making it ideal for applications that require live updates.
π€ How Do Server-Sent Events Work?
SSE works over HTTP and uses a single persistent connection to send multiple updates to the client. The server sends a stream of text data encoded in a specific format, which the client can listen to and process as events. Unlike WebSockets, SSE is one-way, meaning data is only sent from the server to the client.
βοΈ Setting Up Server-Sent Events
To set up SSE on the server side, you need to set the Content-Type to text/event-stream and ensure the response is continuously flushed to the client.
Hereβs an example using Node.js:
const http = require('http');
http.createServer((req, res) => {
res.writeHead(200, {
'Content-Type': 'text/event-stream',
'Cache-Control': 'no-cache',
'Connection': 'keep-alive',
});
setInterval(() => {
res.write(`data: ${new Date().toLocaleTimeString()}\n\n`);
}, 1000);
}).listen(8080);
π Receiving Events on the Client Side
On the client side, you can use the EventSource API to receive and process events sent by the server. Hereβs how you can do it:
const eventSource = new EventSource('http://localhost:8080');
eventSource.onmessage = function(event) {
console.log('New message:', event.data);
};
π Reconnecting Automatically
One of the key features of SSE is its ability to automatically reconnect if the connection is lost. The EventSource object will attempt to reconnect after a disconnection, making it reliable for unstable network conditions.
eventSource.onerror = function(event) {
console.error('EventSource failed:', event);
};
π Handling Different Event Types
SSE supports different event types, which can be specified using the event field in the serverβs message. This allows you to handle multiple types of events separately on the client side.
// Server-side
res.write('event: customEvent\n');
res.write('data: This is a custom event\n\n');
// Client-side
eventSource.addEventListener('customEvent', function(event) {
console.log('Custom event:', event.data);
});
π Security Considerations
While SSE is over HTTP, it is important to consider security, especially when dealing with sensitive data. Always use HTTPS for secure communication and validate incoming data to prevent injection attacks. Also, consider implementing CORS policies to control who can access your SSE streams.
π Example
Hereβs a complete example demonstrating SSE with a simple real-time clock:
<!DOCTYPE html>
<html>
<head>
<title>Server-Sent Events Example</title>
</head>
<body>
<h1>Real-Time Clock</h1>
<div id="clock"></div>
<script>
const eventSource = new EventSource('http://localhost:8080');
eventSource.onmessage = function(event) {
document.getElementById('clock').textContent = event.data;
};
eventSource.onerror = function() {
console.error('Connection error. Retrying...');
};
</script>
</body>
</html>
π Conclusion
Server-Sent Events offer a straightforward way to implement real-time updates in your web applications. By understanding how to set up and use SSE, you can build responsive applications that keep users updated with the latest information without needing to constantly poll the server.
π¨βπ» Join our Community:
Author
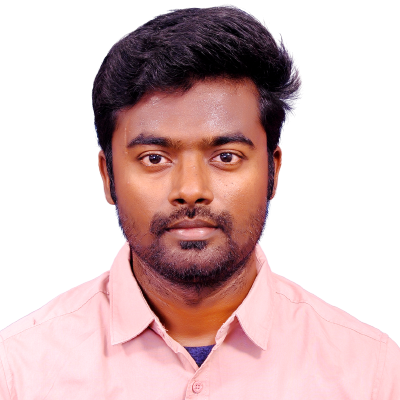
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (HTML Server-Sent Events), please comment here. I will help you immediately.