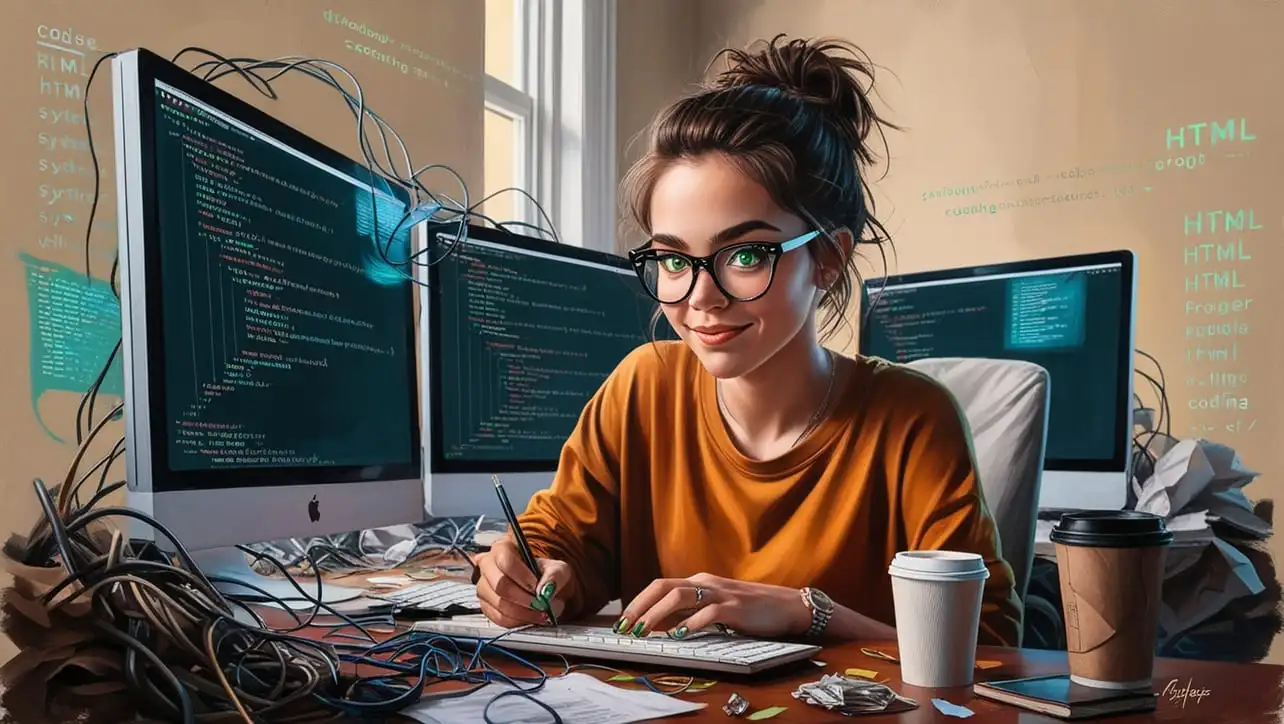
HTML Topics
- HTML Intro
- HTML Basic
- HTML Editors
- HTML CSS
- HTML Tags
- HTML Deprecated Tags
- HTML Events
- HTML Event Attributes
- HTML Global Attributes
- HTML Attributes
- HTML Comments
- HTML Entity
- HTML Head
- HTML Form
- HTML IndexedDB
- HTML Drag & Drop
- HTML Geolocation
- HTML Canvas
- HTML Status Code
- HTML Language Code
- HTML Country Code
- HTML Charset
- MIME Types
HTML Javascript
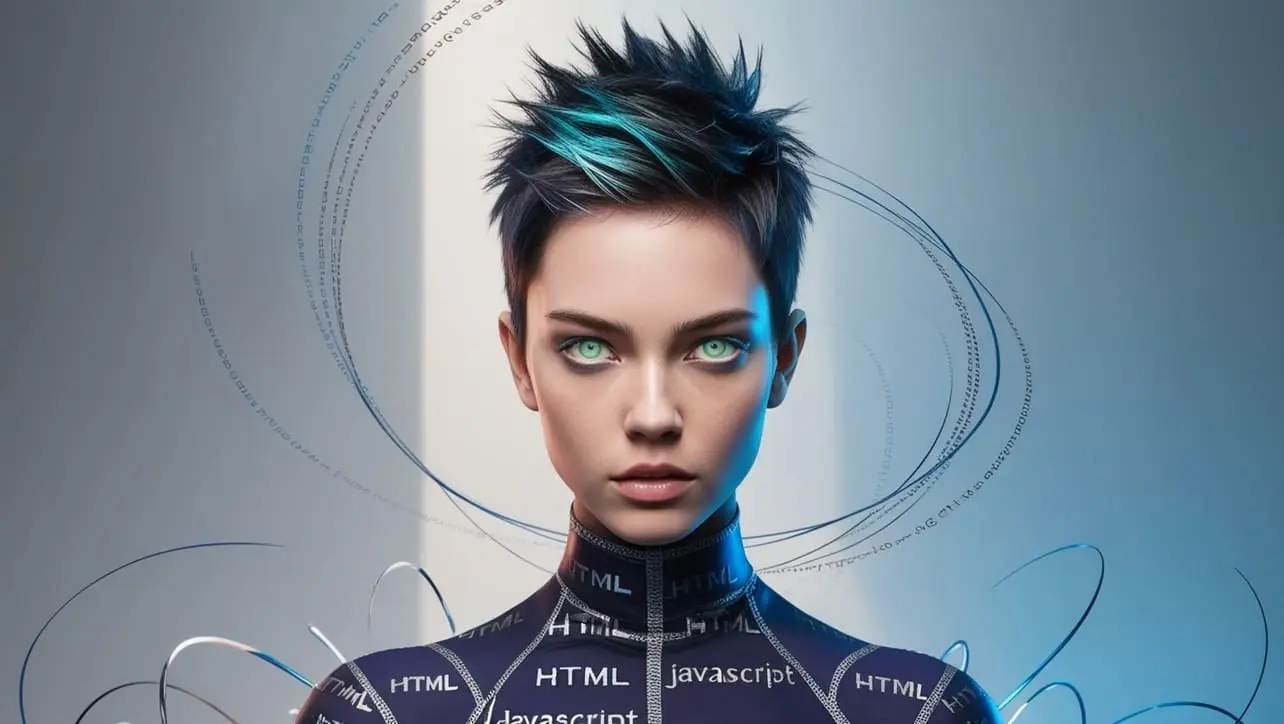
Photo Credit to CodeToFun
Introduction
JavaScript is a dynamic scripting language widely used in web development to enhance HTML pages.
By embedding or linking JavaScript to your HTML files, you can create interactive web elements, respond to user actions, and modify the content dynamically. JavaScript is an essential part of building modern web applications.
What is JavaScript?
JavaScript is a lightweight, interpreted programming language used to create dynamic and interactive elements on web pages. It can manipulate HTML and CSS, handle events, validate data, and more. It runs in the browser, making it a client-side scripting language. However, JavaScript can also be used server-side with environments like Node.js.
Adding JavaScript to HTML
You can add JavaScript directly into HTML documents using the <script> tag. The script can either be placed within the HTML file or linked as an external JavaScript file.
<!DOCTYPE html>
<html>
<head>
<title>HTML with JavaScript</title>
</head>
<body>
<h1>Welcome to My Website</h1>
<script>
alert('Hello, JavaScript is working!');
</script>
</body>
</html>
In the example above, JavaScript is embedded within the HTML file, which will display an alert when the page is loaded.
JavaScript in the <head> and <body>
JavaScript code can be placed either in the <head> or <body> sections of an HTML document. Typically, JavaScript is added at the bottom of the <body> to ensure that the HTML elements are fully loaded before the script executes.
- In the <head> section: JavaScript runs before the page content is loaded, which may cause issues if the script tries to access HTML elements that haven’t been rendered yet.
- In the <body> section: JavaScript runs after the page has loaded, which is a common practice to avoid issues with rendering.
External JavaScript Files
To keep your HTML files clean and maintainable, you can store JavaScript in separate files and link them using the <script src> attribute.
<!DOCTYPE html>
<html>
<head>
<title>External JavaScript Example</title>
<script src="app.js"></script>
</head>
<body>
<h1>External JavaScript File Example</h1>
</body>
</html>
In the app.js file:
console.log('This message is from an external JavaScript file.');
Common JavaScript Features in HTML
Event Handling:
JavaScript can respond to user actions like clicks, keypresses, or form submissions.
HTMLCopied<button onclick="alert('Button clicked!')">Click Me</button>
DOM Manipulation:
JavaScript can modify HTML elements dynamically. For example, changing the text of an element:
HTMLCopied<h1 id="myTitle">Original Title</h1> <script> document.getElementById('myTitle').textContent = 'New Title'; </script>
Form Validation:
You can use JavaScript to validate form inputs before submitting to the server.
HTMLCopied<form onsubmit="return validateForm()"> <input type="text" id="name"> <input type="submit" value="Submit"> </form> <script> function validateForm() { const name = document.getElementById('name').value; if (name === '') { alert('Name must be filled out'); return false; } return true; } </script>
Example
Here’s a complete example demonstrating some basic JavaScript functionality in an HTML page:
<!DOCTYPE html>
<html>
<head>
<title>JavaScript Example</title>
</head>
<body>
<h1>Welcome to JavaScript with HTML</h1>
<p id="demo">JavaScript can change this text.</p>
<button onclick="changeText()">Click me</button>
<script>
function changeText() {
document.getElementById('demo').textContent = 'Text changed by JavaScript!';
}
</script>
</body>
</html>
In this example, clicking the button will change the text content of the paragraph.
Conclusion
JavaScript is a crucial component for adding interactivity and dynamic behavior to HTML pages. Whether you use inline scripts or external files, understanding how JavaScript interacts with HTML is key to building responsive and engaging web applications.
Join our Community:
Author
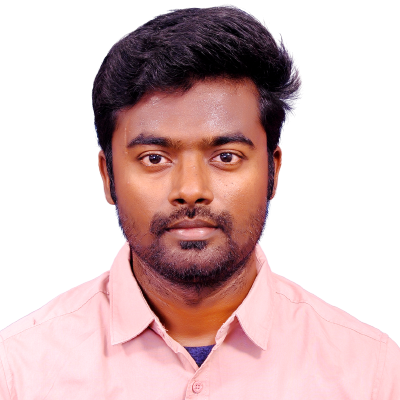
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (HTML Javascript), please comment here. I will help you immediately.