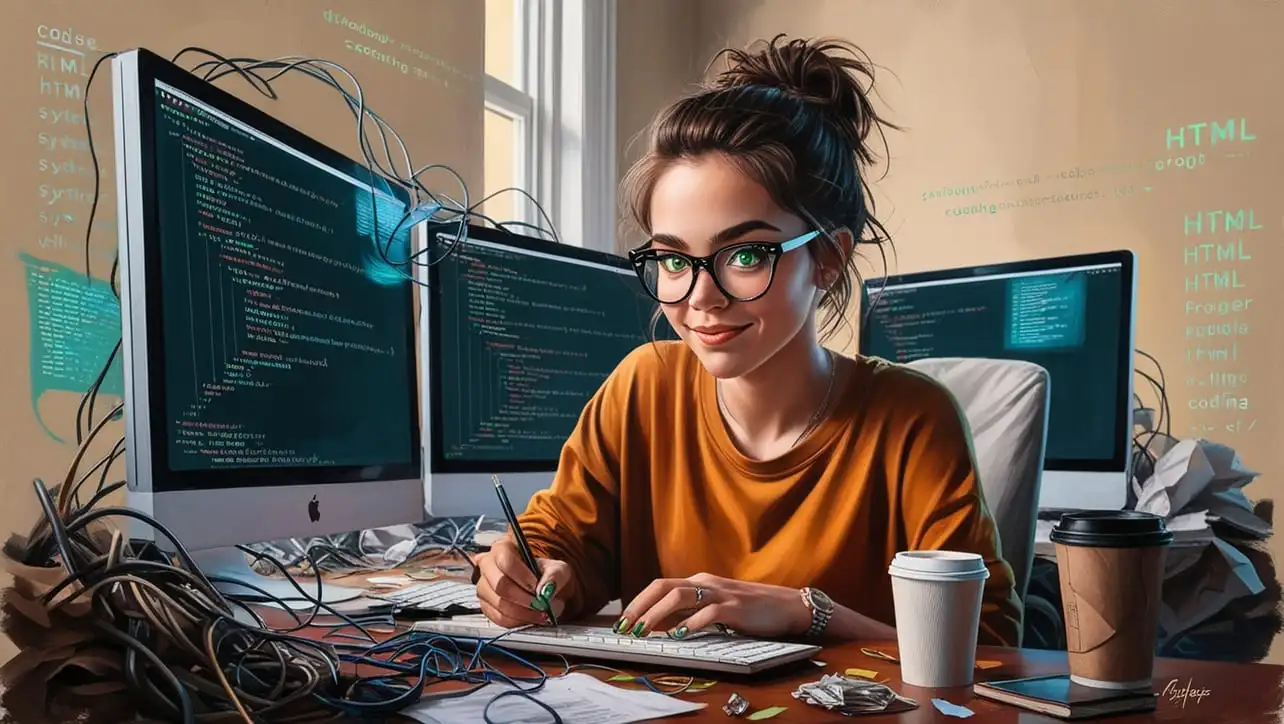
HTML Basic
HTML Entity
- HTML Entity
- HTML Alphabet Entity
- HTML Arrow Entity
- HTML Currency Entity
- HTML Math Entity
- HTML Number Entity
- HTML Punctuation Entity
- HTML Symbol Entity
HTML IndexedDB
HTML Reference
HTML IndexedDB Update

Photo Credit to CodeToFun
π Introduction
IndexedDB is a low-level API for storing large amounts of structured data in the browser, providing a way to manage and query data offline.
This guide focuses on updating records within IndexedDB, which is crucial for maintaining and modifying data efficiently in your web applications.
β What is IndexedDB?
IndexedDB is a client-side storage solution that allows you to store and retrieve data in a structured way. Unlike localStorage, IndexedDB supports more complex data types and offers powerful querying capabilities. Itβs ideal for applications that need to store a large amount of data or work offline.
π€ How to Update Records in IndexedDB
To update records in IndexedDB, you need to follow these steps:
- Open a Transaction: Transactions are used to group multiple operations into a single unit.
- Access the Object Store: Use the transaction to get access to the object store where your records are stored.
- Retrieve and Update the Record: Use the get method to retrieve the record, modify it, and then use the put method to save the updated record.
Example Code
// Open a connection to the database
const request = indexedDB.open('myDatabase', 1);
request.onsuccess = function(event) {
const db = event.target.result;
// Start a transaction
const transaction = db.transaction(['myObjectStore'], 'readwrite');
const objectStore = transaction.objectStore('myObjectStore');
// Retrieve the record
const getRequest = objectStore.get(1); // Assuming the key is 1
getRequest.onsuccess = function(event) {
const record = event.target.result;
if (record) {
// Update the record
record.value = 'Updated Value'; // Modify the record as needed
const updateRequest = objectStore.put(record);
updateRequest.onsuccess = function() {
console.log('Record updated successfully');
};
updateRequest.onerror = function() {
console.error('Error updating record');
};
} else {
console.log('Record not found');
}
};
};
request.onerror = function(event) {
console.error('Database error:', event.target.errorCode);
};
π΅ Using Transactions
Transactions are essential for ensuring data consistency and integrity in IndexedDB. When performing updates, always use a transaction to group read and write operations. Transactions can be set to 'readwrite' mode to allow modifications.
π€ Handling Errors
Handling errors effectively is crucial for debugging and maintaining data integrity. Use the onsuccess and onerror event handlers to manage success and failure scenarios. Ensure that you check for errors when opening the database, retrieving records, and updating data.
β οΈ Common Pitfalls
- Concurrency Issues: Be aware of potential concurrency issues when multiple transactions are trying to access the same data. IndexedDB handles this with its own locking mechanism, but itβs important to design your transactions to avoid conflicts.
- Key Management: Ensure that you correctly manage keys when updating records. If the key is incorrect or missing, updates may not work as expected.
- Error Handling: Always implement proper error handling to catch and address issues that may arise during database operations.
π Example Usage
Hereβs a complete example that demonstrates updating a record in IndexedDB:
<!DOCTYPE html>
<html>
<head>
<title>IndexedDB Update Example</title>
</head>
<body>
<h1>IndexedDB Update Example</h1>
<button id="update">Update Record</button>
<script>
// Open a connection to the database
const request = indexedDB.open('myDatabase', 1);
request.onupgradeneeded = function(event) {
const db = event.target.result;
db.createObjectStore('myObjectStore', { keyPath: 'id' });
};
request.onsuccess = function(event) {
const db = event.target.result;
document.getElementById('update').addEventListener('click', () => {
const transaction = db.transaction(['myObjectStore'], 'readwrite');
const objectStore = transaction.objectStore('myObjectStore');
// Retrieve the record
const getRequest = objectStore.get(1); // Assuming the key is 1
getRequest.onsuccess = function(event) {
const record = event.target.result;
if (record) {
// Update the record
record.value = 'Updated Value';
const updateRequest = objectStore.put(record);
updateRequest.onsuccess = function() {
console.log('Record updated successfully');
};
updateRequest.onerror = function() {
console.error('Error updating record');
};
} else {
console.log('Record not found');
}
};
});
};
request.onerror = function(event) {
console.error('Database error:', event.target.errorCode);
};
</script>
</body>
</html>
π Conclusion
Updating records in IndexedDB is a straightforward process that involves transactions and proper error handling. By understanding how to perform updates, you can manage your data effectively and ensure your web application operates smoothly.
π¨βπ» Join our Community:
Author
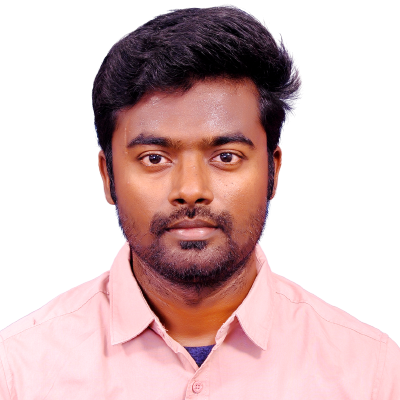
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (HTML IndexedDB Update), please comment here. I will help you immediately.