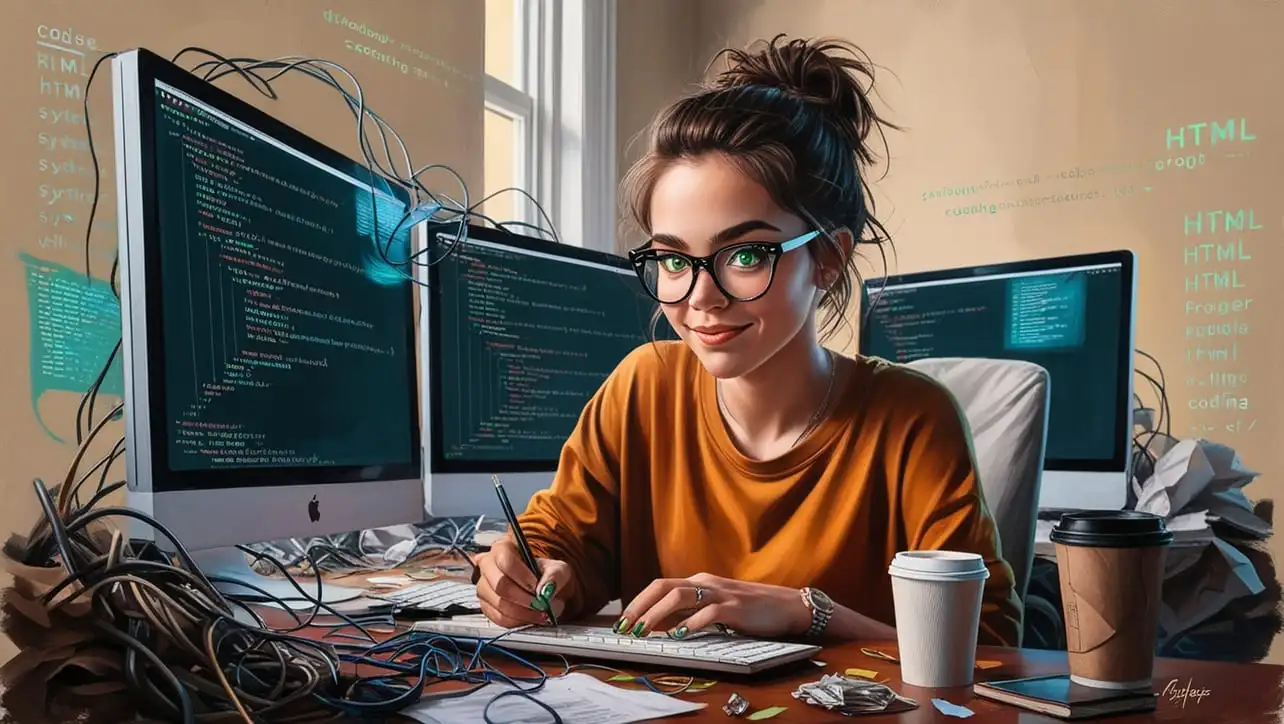
HTML Topics
- HTML Intro
- HTML Basic
- HTML Editors
- HTML CSS
- HTML Tags
- HTML Deprecated Tags
- HTML Events
- HTML Event Attributes
- HTML Global Attributes
- HTML Attributes
- HTML Comments
- HTML Entity
- HTML Head
- HTML Form
- HTML IndexedDB
- HTML Drag & Drop
- HTML Geolocation
- HTML Canvas
- HTML Status Code
- HTML Language Code
- HTML Country Code
- HTML Charset
- MIME Types
HTML IndexedDB Insert
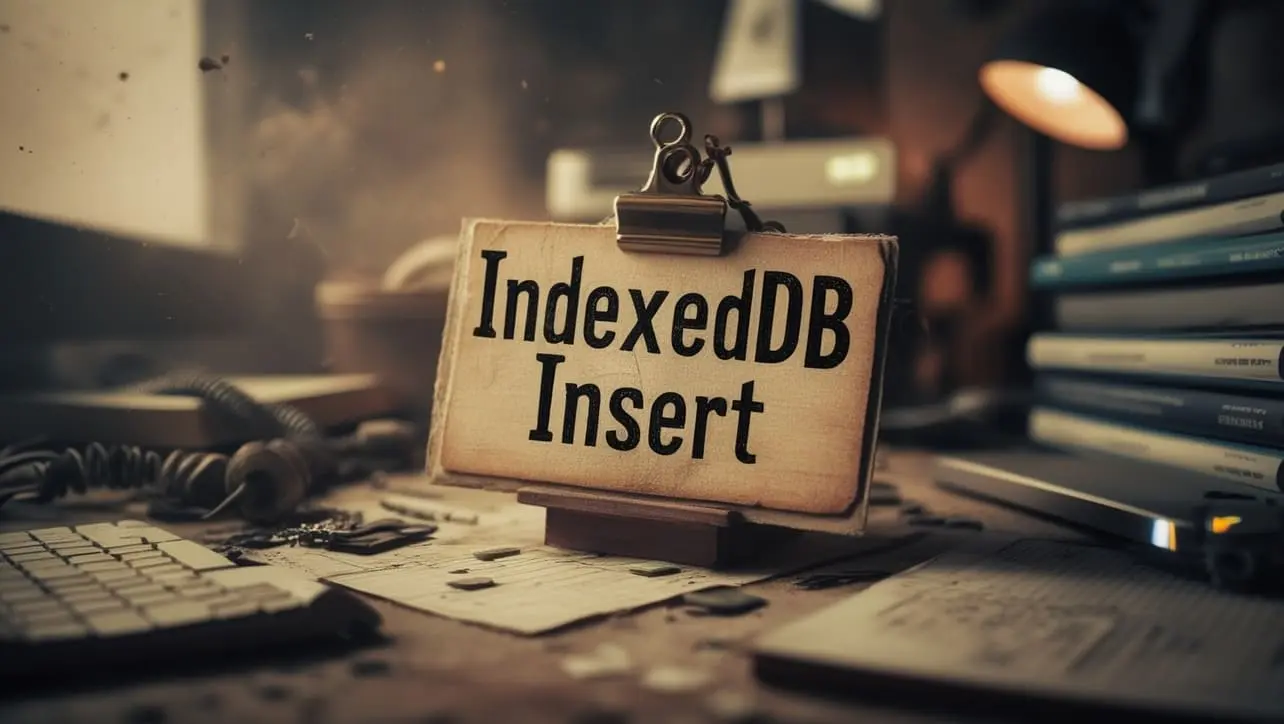
Photo Credit to CodeToFun
π Introduction
IndexedDB is a low-level API for storing large amounts of structured data in the browser. It allows you to create, read, update, and delete data in a transactional manner.
In this guide, we'll focus on how to insert data into an IndexedDB database, which is crucial for applications requiring client-side storage.
β What is IndexedDB?
IndexedDB is a database system that allows for the storage of significant amounts of structured data. It supports indexing and transactions and is designed for applications that need to store and retrieve complex data, such as offline web applications.
π¨ Creating an IndexedDB Database
Before inserting data, you need to create an IndexedDB database. This involves defining an object store, which is a structure for storing data. Here's a simple example of creating a database with an object store:
// Create or open a database
const request = indexedDB.open('myDatabase', 1);
request.onupgradeneeded = function(event) {
const db = event.target.result;
// Create an object store
const objectStore = db.createObjectStore('myObjectStore', { keyPath: 'id', autoIncrement: true });
// Define an index on a field
objectStore.createIndex('nameIndex', 'name', { unique: false });
};
request.onsuccess = function(event) {
console.log('Database created/opened successfully');
};
request.onerror = function(event) {
console.error('Database error:', event.target.errorCode);
};
π Opening a Database
Once a database is created, you can open it to perform operations like inserting data. Hereβs how to open a database and handle transactions:
const request = indexedDB.open('myDatabase');
request.onsuccess = function(event) {
const db = event.target.result;
// Database opened successfully
};
request.onerror = function(event) {
console.error('Database error:', event.target.errorCode);
};
π₯ Inserting Data
To insert data into an object store, you need to perform a transaction. Here's how you can add data to the store:
const request = indexedDB.open('myDatabase');
request.onsuccess = function(event) {
const db = event.target.result;
const transaction = db.transaction(['myObjectStore'], 'readwrite');
const objectStore = transaction.objectStore('myObjectStore');
// Create an object to store
const item = { name: 'John Doe', age: 30 };
const addRequest = objectStore.add(item);
addRequest.onsuccess = function() {
console.log('Data added to the store:', item);
};
addRequest.onerror = function(event) {
console.error('Error adding data:', event.target.errorCode);
};
};
πΈ Handling Transactions
Transactions in IndexedDB ensure data integrity by grouping operations. You can specify the transaction mode (readwrite or readonly) and handle success and error events accordingly:
const transaction = db.transaction(['myObjectStore'], 'readwrite');
transaction.oncomplete = function() {
console.log('Transaction completed successfully');
};
transaction.onerror = function(event) {
console.error('Transaction error:', event.target.errorCode);
};
π« Error Handling
Handling errors effectively is crucial for maintaining a robust application. IndexedDB operations can fail due to various reasons, such as exceeding storage limits or trying to perform operations on a closed database:
const request = indexedDB.open('myDatabase');
request.onerror = function(event) {
console.error('Database error:', event.target.errorCode);
};
const transaction = db.transaction(['myObjectStore'], 'readwrite');
transaction.onerror = function(event) {
console.error('Transaction error:', event.target.errorCode);
};
β οΈ Common Pitfalls
- Data Integrity: Ensure that the key paths and indexes are correctly defined to avoid data inconsistencies.
- Database Versioning: Handle database version changes properly using the onupgradeneeded event.
- Browser Compatibility: IndexedDB is widely supported but always check for compatibility in different browsers and provide fallbacks if needed.
π Example Usage
Hereβs a complete example that demonstrates creating a database, adding data, and handling transactions:
<!DOCTYPE html>
<html>
<head>
<title>IndexedDB Insert Example</title>
</head>
<body>
<h1>IndexedDB Insert Example</h1>
<button id="addData">Add Data</button>
<script>
// Create or open a database
const dbRequest = indexedDB.open('myDatabase', 1);
dbRequest.onupgradeneeded = function(event) {
const db = event.target.result;
db.createObjectStore('myObjectStore', { keyPath: 'id', autoIncrement: true });
};
dbRequest.onsuccess = function(event) {
const db = event.target.result;
document.getElementById('addData').addEventListener('click', () => {
const transaction = db.transaction(['myObjectStore'], 'readwrite');
const objectStore = transaction.objectStore('myObjectStore');
const item = { name: 'Jane Doe', age: 25 };
const addRequest = objectStore.add(item);
addRequest.onsuccess = function() {
console.log('Data added:', item);
};
addRequest.onerror = function(event) {
console.error('Error adding data:', event.target.errorCode);
};
});
};
dbRequest.onerror = function(event) {
console.error('Database error:', event.target.errorCode);
};
</script>
</body>
</html>
π Conclusion
Inserting data into IndexedDB involves creating a database, opening it, handling transactions, and managing errors. Mastering these steps ensures efficient and reliable client-side data storage for your web applications.
π¨βπ» Join our Community:
Author
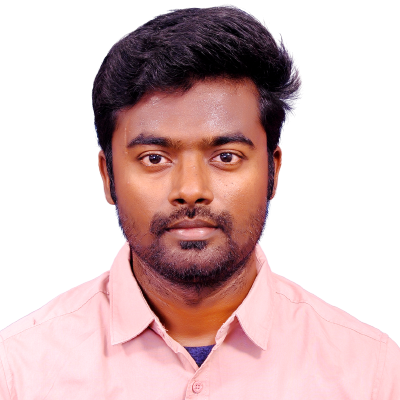
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (HTML IndexedDB Insert) please comment here. I will help you immediately.