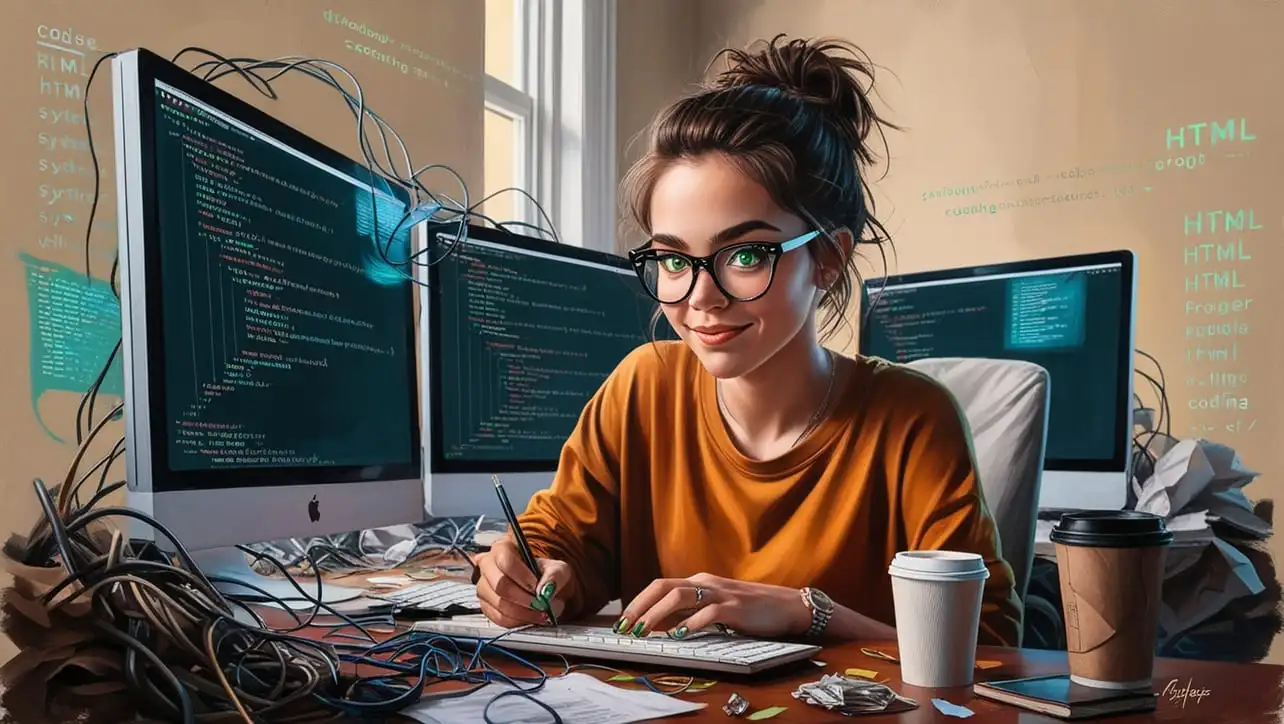
HTML Topics
- HTML Intro
- HTML Basic
- HTML Editors
- HTML CSS
- HTML Tags
- HTML Deprecated Tags
- HTML Events
- HTML Event Attributes
- HTML Global Attributes
- HTML Attributes
- HTML Comments
- HTML Entity
- HTML Head
- HTML Form
- HTML IndexedDB
- HTML Drag & Drop
- HTML Geolocation
- HTML Canvas
- HTML Status Code
- HTML Language Code
- HTML Country Code
- HTML Charset
- MIME Types
HTML IndexedDB Delete
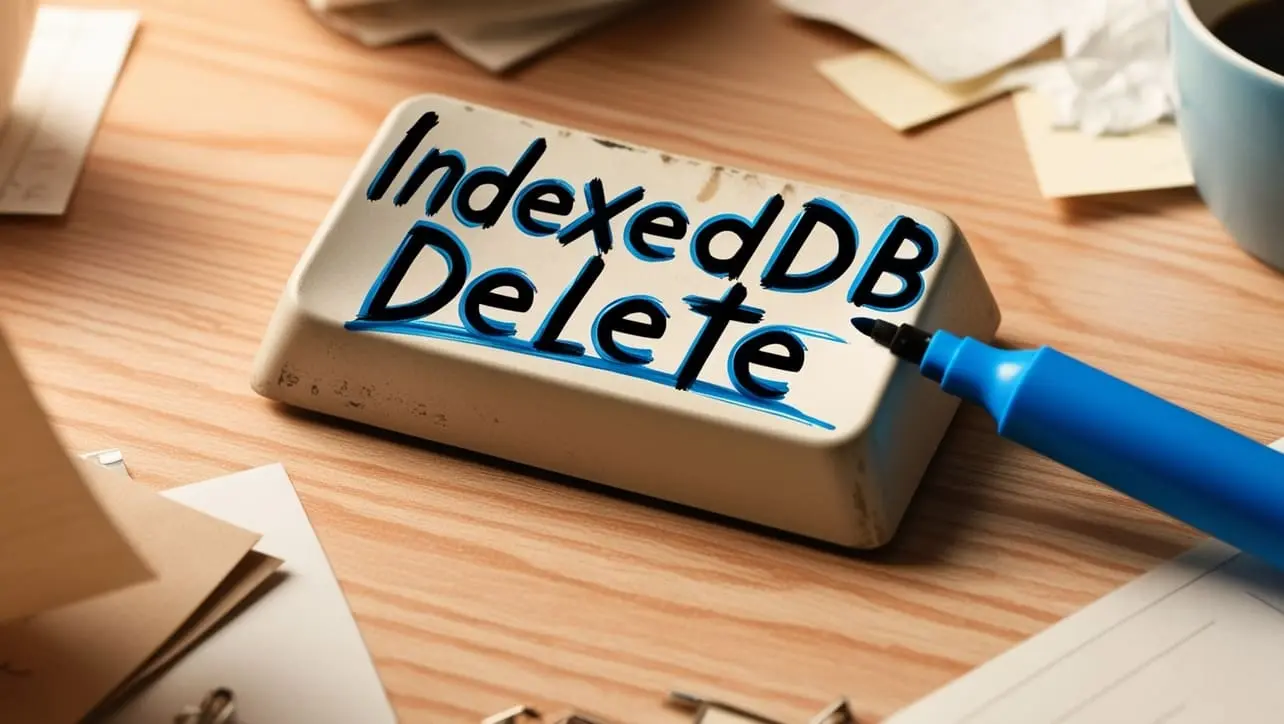
Photo Credit to CodeToFun
Introduction
IndexedDB is a low-level API for client-side storage of significant amounts of structured data, including files and blobs. It allows you to store, search, and retrieve data efficiently in web applications.
Deleting data from IndexedDB is a critical operation that ensures your application can manage storage effectively.
What is IndexedDB?
IndexedDB is a powerful storage solution in web browsers that lets developers manage large volumes of structured data. Unlike localStorage, IndexedDB allows for more complex queries and storing complex data types.
Understanding Deletion in IndexedDB
Deletion in IndexedDB can refer to removing individual records, clearing all records in an object store, or even deleting entire object stores. Understanding the appropriate deletion operation for your needs is crucial for maintaining the integrity of your data.
Deleting Data from IndexedDB
To delete specific data from an IndexedDB object store, you can use the delete method provided by the transaction. Below is a basic example:
// Open the database
const request = indexedDB.open('MyDatabase', 1);
request.onsuccess = function(event) {
const db = event.target.result;
const transaction = db.transaction(['MyObjectStore'], 'readwrite');
const objectStore = transaction.objectStore('MyObjectStore');
// Delete a record by key
const deleteRequest = objectStore.delete('someKey');
deleteRequest.onsuccess = function() {
console.log('Record deleted successfully');
};
deleteRequest.onerror = function() {
console.error('Failed to delete record');
};
};
Deleting All Records
If you need to delete all records from an object store, the clear method is the most efficient way to do so:
const transaction = db.transaction(['MyObjectStore'], 'readwrite');
const objectStore = transaction.objectStore('MyObjectStore');
// Clear all records
const clearRequest = objectStore.clear();
clearRequest.onsuccess = function() {
console.log('All records deleted successfully');
};
clearRequest.onerror = function() {
console.error('Failed to delete all records');
};
Deleting Object Stores
To delete an entire object store, you need to perform this operation during a version upgrade of the database:
const request = indexedDB.open('MyDatabase', 2); // Increment the version number
request.onupgradeneeded = function(event) {
const db = event.target.result;
// Delete the object store
if (db.objectStoreNames.contains('MyObjectStore')) {
db.deleteObjectStore('MyObjectStore');
console.log('Object store deleted successfully');
}
};
Common Pitfalls
- Transaction Mode: Ensure that you use the correct transaction mode (readwrite) when attempting to delete records. A read-only transaction will not allow deletion.
- Version Upgrades: Deleting object stores requires a version upgrade. Be cautious when handling version upgrades, as they can impact all users accessing the database.
- Error Handling: Always implement error handling when deleting records or object stores to gracefully manage any issues that may arise.
Example Usage
Hereβs a complete example that demonstrates how to delete a specific record from an IndexedDB object store:
// Open the database
const request = indexedDB.open('MyDatabase', 1);
request.onsuccess = function(event) {
const db = event.target.result;
const transaction = db.transaction(['MyObjectStore'], 'readwrite');
const objectStore = transaction.objectStore('MyObjectStore');
// Delete a record with a specific key
const deleteRequest = objectStore.delete('uniqueKey');
deleteRequest.onsuccess = function() {
console.log('Record with key "uniqueKey" deleted successfully');
};
deleteRequest.onerror = function() {
console.error('Failed to delete the record');
};
};
Conclusion
Deleting data in IndexedDB is an essential operation for managing and maintaining your database. Whether you are deleting individual records, clearing entire object stores, or upgrading your database version, understanding how to handle these operations correctly is key to ensuring your application runs smoothly.
Join our Community:
Author
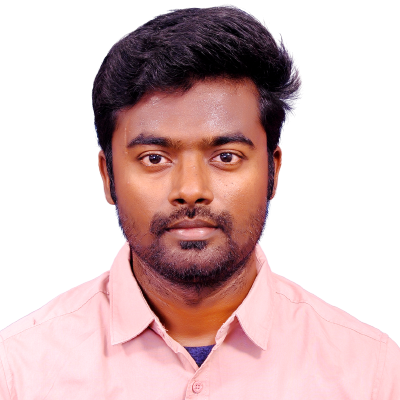
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (HTML IndexedDB Delete), please comment here. I will help you immediately.