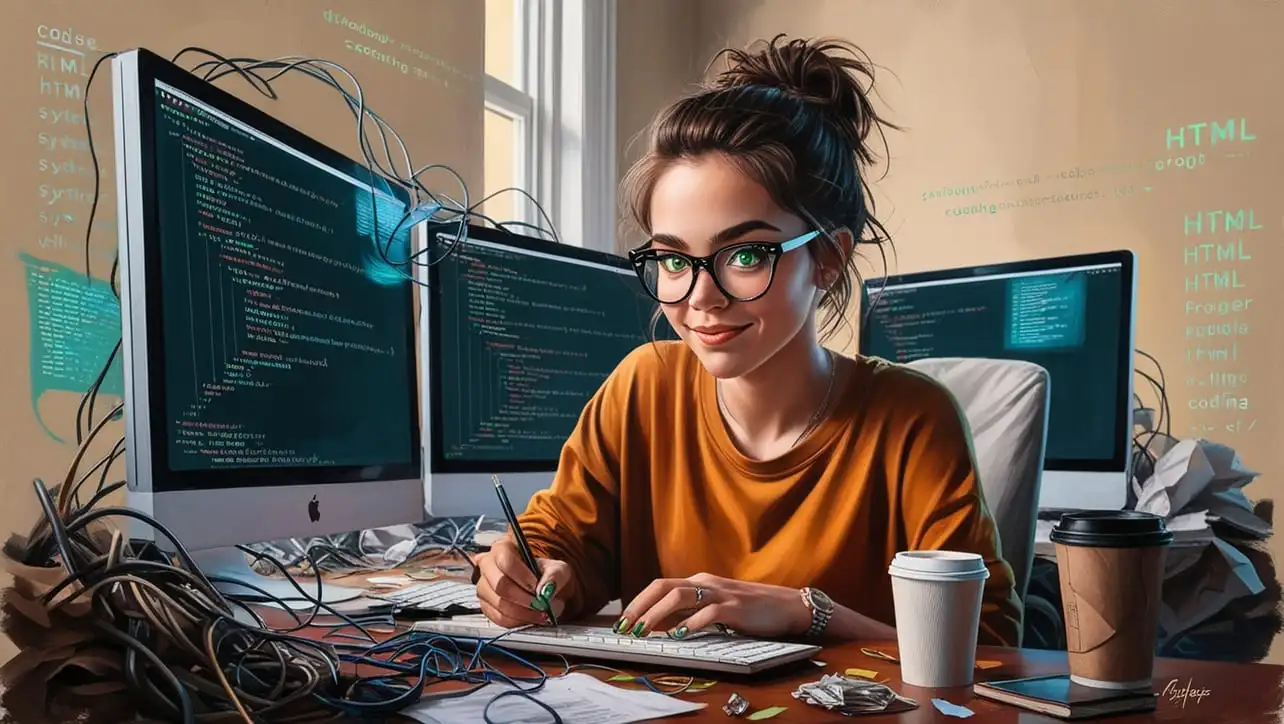
HTML Topics
- HTML Intro
- HTML Basic
- HTML Editors
- HTML CSS
- HTML Tags
- HTML Deprecated Tags
- HTML Events
- HTML Event Attributes
- HTML Global Attributes
- HTML Attributes
- HTML Comments
- HTML Entity
- HTML Head
- HTML Form
- HTML IndexedDB
- HTML Drag & Drop
- HTML Geolocation
- HTML Canvas
- HTML Status Code
- HTML Language Code
- HTML Country Code
- HTML Charset
- MIME Types
HTML IndexedDB
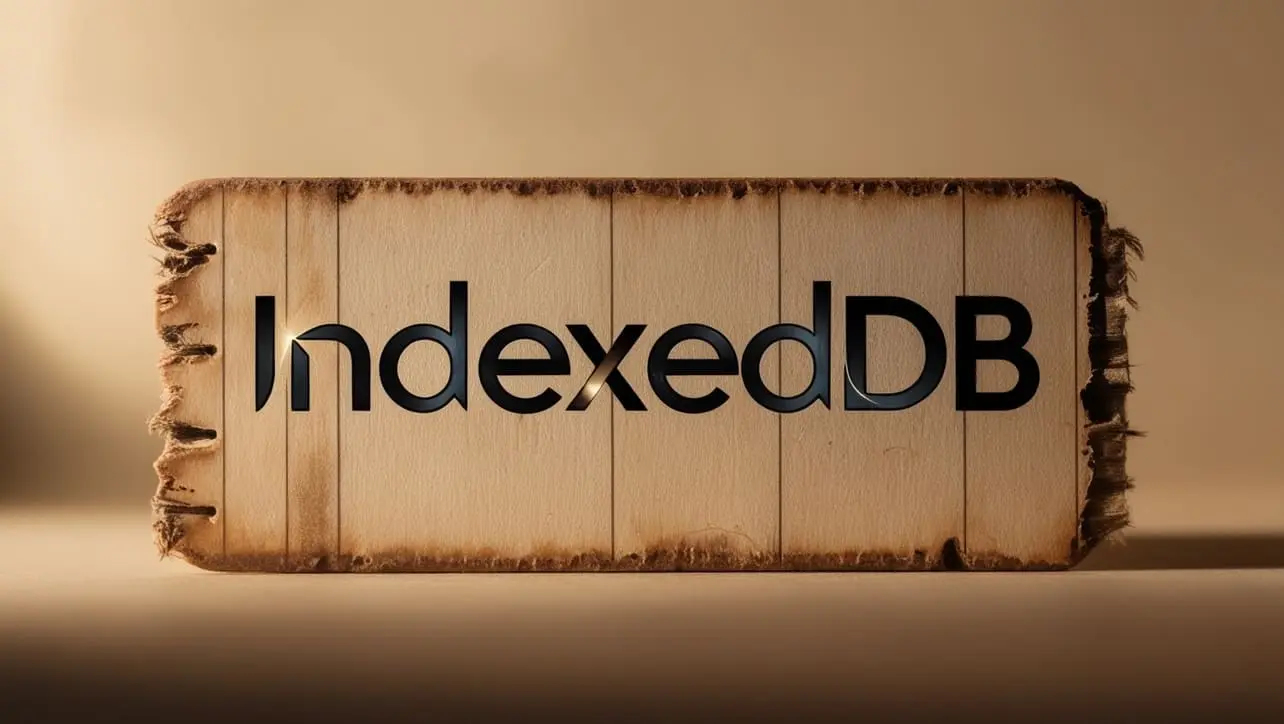
Photo Credit to CodeToFun
π Introduction
IndexedDB is a low-level API for storing large amounts of structured data, including files and blobs. Unlike Web Storage (localStorage and sessionStorage), IndexedDB provides a richer data storage mechanism with support for indexing, transactions, and key-based access. This makes it suitable for complex data structures and large-scale web applications.
β What Is IndexedDB?
IndexedDB is a transactional database system built into modern browsers. It allows you to store and retrieve objects using a key-based approach, with support for complex queries and large datasets.
It operates asynchronously, meaning it doesnβt block the main thread, which is crucial for maintaining a responsive user interface.
π Key Features of IndexedDB
- Structured Data Storage: Supports storing objects with complex data structures.
- Indexing: Allows you to create indexes for efficient querying.
- Transactions: Provides a way to group multiple operations into a single atomic transaction.
- Asynchronous API: Ensures non-blocking operations to maintain application performance.
π§ Basic Concepts
- Database: The container for all data, which holds object stores and indexes.
- Object Store: A store for data objects, similar to tables in relational databases.
- Index: A mechanism to quickly look up records based on specific properties.
- Transaction: A sequence of operations executed as a single unit, ensuring data consistency.
ποΈ Creating and Opening a Database
To use IndexedDB, you first need to create and open a database. This involves specifying a version number and handling the onupgradeneeded event to set up object stores and indexes.
let db;
const request = indexedDB.open('myDatabase', 1);
request.onupgradeneeded = function(event) {
db = event.target.result;
const objectStore = db.createObjectStore('myObjectStore', { keyPath: 'id' });
objectStore.createIndex('nameIndex', 'name', { unique: false });
};
request.onsuccess = function(event) {
db = event.target.result;
};
request.onerror = function(event) {
console.error('Database error:', event.target.errorCode);
};
πͺ Working with Object Stores
Object stores are where you save your data. Each object store has a key path or key generator for uniquely identifying objects.
function addItem(item) {
const transaction = db.transaction(['myObjectStore'], 'readwrite');
const objectStore = transaction.objectStore('myObjectStore');
const request = objectStore.add(item);
request.onsuccess = function() {
console.log('Item added successfully.');
};
request.onerror = function(event) {
console.error('Add item error:', event.target.errorCode);
};
}
πΈ Transactions and Cursors
Transactions ensure that operations are completed successfully or not at all. Cursors allow you to iterate over records in an object store or index.
function getAllItems() {
const transaction = db.transaction(['myObjectStore'], 'readonly');
const objectStore = transaction.objectStore('myObjectStore');
const request = objectStore.openCursor();
request.onsuccess = function(event) {
const cursor = event.target.result;
if (cursor) {
console.log('Item:', cursor.value);
cursor.continue();
} else {
console.log('No more items.');
}
};
request.onerror = function(event) {
console.error('Cursor error:', event.target.errorCode);
};
}
π€ Handling Errors
Errors in IndexedDB operations are handled through the onerror event. It is important to check and manage errors to ensure data integrity and provide feedback to users.
request.onerror = function(event) {
console.error('Error:', event.target.errorCode);
};
π Best Practices
- Indexing: Use indexes to improve query performance.
- Transactions: Group related operations in transactions to ensure atomicity.
- Error Handling: Implement comprehensive error handling for robust applications.
- Data Size: Be mindful of the amount of data stored to avoid performance issues.
π Example Usage
Hereβs a simple example demonstrating the creation of a database, adding an item, and retrieving all items:
<!DOCTYPE html>
<html>
<head>
<title>IndexedDB Example</title>
</head>
<body>
<h1>IndexedDB Example</h1>
<button id="add">Add Item</button>
<button id="get">Get All Items</button>
<script>
let db;
const request = indexedDB.open('myDatabase', 1);
request.onupgradeneeded = function(event) {
db = event.target.result;
db.createObjectStore('myObjectStore', { keyPath: 'id' });
};
request.onsuccess = function(event) {
db = event.target.result;
};
document.getElementById('add').addEventListener('click', () => {
const item = { id: 1, name: 'Sample Item' };
const transaction = db.transaction(['myObjectStore'], 'readwrite');
const objectStore = transaction.objectStore('myObjectStore');
objectStore.add(item);
});
document.getElementById('get').addEventListener('click', () => {
const transaction = db.transaction(['myObjectStore'], 'readonly');
const objectStore = transaction.objectStore('myObjectStore');
const request = objectStore.openCursor();
request.onsuccess = function(event) {
const cursor = event.target.result;
if (cursor) {
console.log('Item:', cursor.value);
cursor.continue();
} else {
console.log('No more items.');
}
};
});
</script>
</body>
</html>
π Conclusion
IndexedDB provides a robust and efficient way to handle complex data requirements in web applications. By understanding its core concepts and leveraging its features effectively, you can build powerful and responsive applications that handle large datasets and complex data structures seamlessly.
π¨βπ» Join our Community:
Author
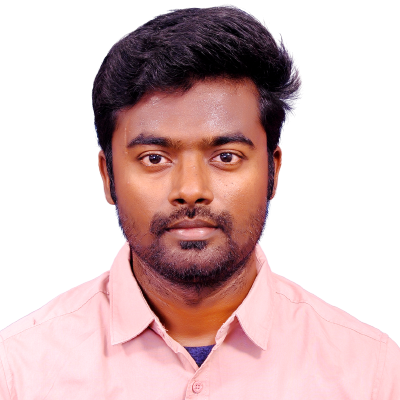
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (HTML IndexedDB) please comment here. I will help you immediately.