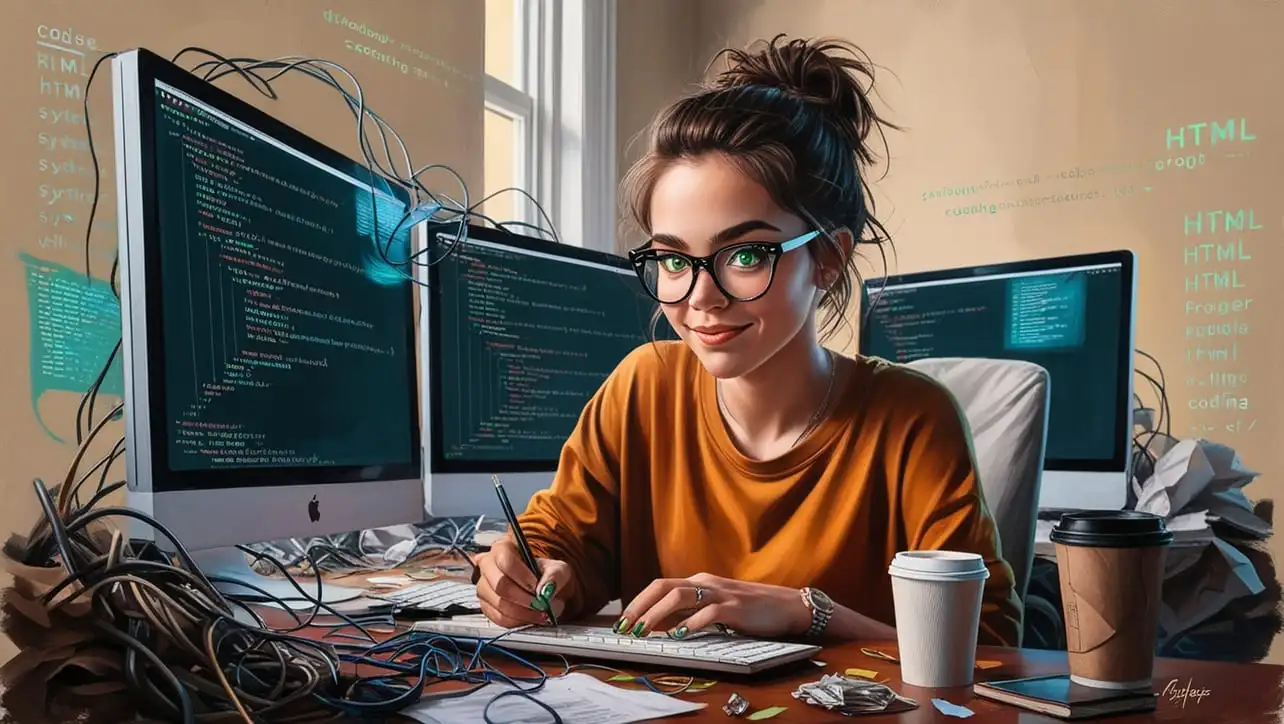
HTML Topics
- HTML Intro
- HTML Basic
- HTML Editors
- HTML CSS
- HTML Tags
- HTML Deprecated Tags
- HTML Events
- HTML Event Attributes
- HTML Global Attributes
- HTML Attributes
- HTML Comments
- HTML Entity
- HTML Head
- HTML Form
- HTML IndexedDB
- HTML Drag & Drop
- HTML Geolocation
- HTML Canvas
- HTML Status Code
- HTML Language Code
- HTML Country Code
- HTML Charset
- MIME Types
HTML Form
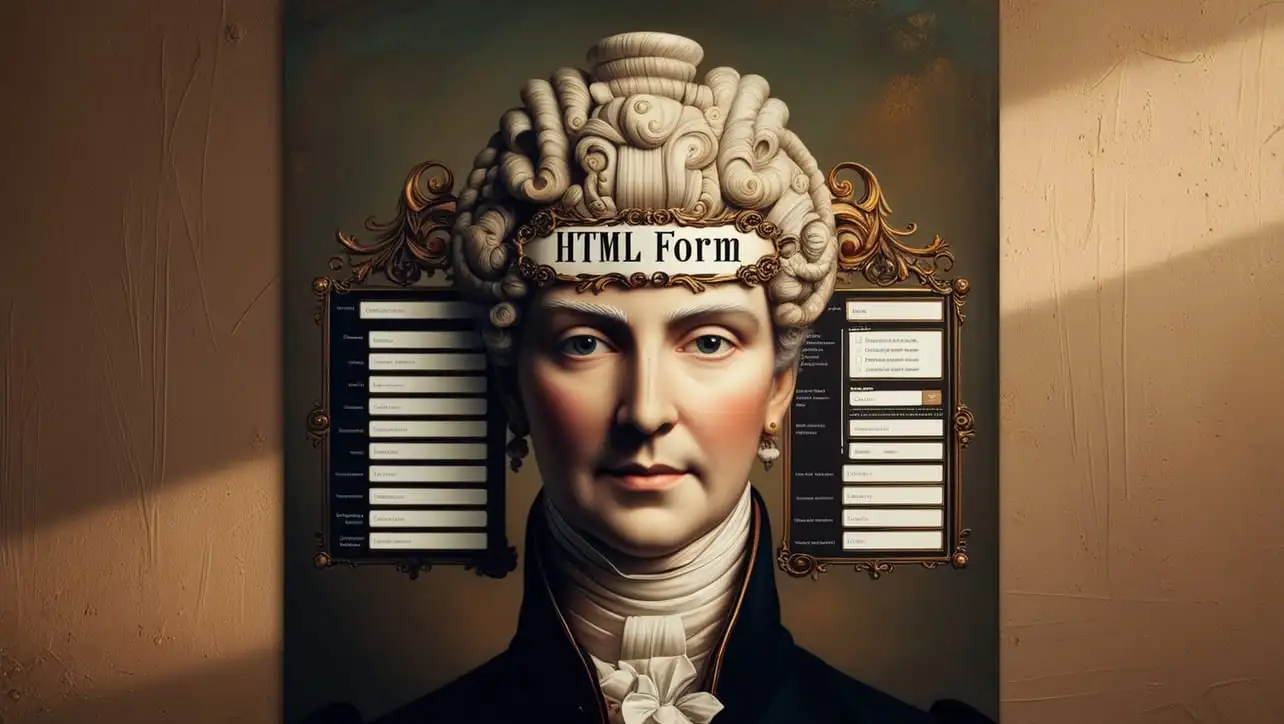
Photo Credit to CodeToFun
Introduction
HTML forms are essential components in web development, allowing users to submit data to a server for processing. Forms are used in various applications, from simple contact forms to complex data entry interfaces.
Understanding how to create and manage forms is crucial for building interactive web applications.
What Is an HTML Form?
An HTML form is a container for various input elements that users interact with to submit data. Forms use the <form> element and can include a variety of input types, such as text fields, radio buttons, checkboxes, and submit buttons.
Form Elements
HTML forms consist of several key elements:
- <input>: Used for various types of user input, including text, password, and file uploads.
- <textarea>: Allows users to enter multi-line text.
- <select>: Creates a drop-down list of options.
- <button>: Defines clickable buttons, including submit and reset buttons.
- <label>: Provides labels for form controls to improve accessibility and usability.
Example
<form>
<label for="name">Name:</label>
<input type="text" id="name" name="name">
<label for="email">Email:</label>
<input type="email" id="email" name="email">
<label for="message">Message:</label>
<textarea id="message" name="message"></textarea>
<button type="submit">Submit</button>
</form>
Form Attributes
Form elements can be customized using various attributes:
- action: Specifies the URL to which the form data is sent.
- method: Defines the HTTP method to be used (e.g., GET, POST).
- enctype: Specifies how the form data should be encoded when submitting it to the server (e.g.,
application/x-www-form-urlencoded
,multipart/form-data
). - name: Identifies the form or input element, useful for accessing form data programmatically.
Form Validation
HTML5 introduced built-in form validation to ensure that data entered by users meets specific criteria. Common validation attributes include:
- required: Specifies that an input field must be filled out before submitting the form.
- pattern: Defines a regular expression that the input value must match.
- min and max: Set the minimum and maximum values for numeric inputs.
Example
<form></form>
<label for="username">Username:</label>
<input type="text" id="username" name="username" required pattern="[A-Za-z]{5,}">
<label for="age">Age:</label>
<input type="number" id="age" name="age" min="1" max="100">
<button type="submit">Submit</button>
</form>
Form Submission
Forms can be submitted either by clicking a submit button or programmatically using JavaScript. The form data is sent to the server specified in the action
attribute using the method defined in the method
attribute.
Example
<form action="/submit" method="post"></form>
<!-- Form elements here -->
<button type="submit">Submit</button>
</form>
Handling Form Data
Form data can be handled on the server side (e.g., using PHP, Python, Node.js) or on the client side using JavaScript.
When using JavaScript, you can access form data through the FormData
API or directly through form element properties.
Example
<script>
document.querySelector('form').addEventListener('submit', function(event) {
event.preventDefault(); // Prevents default form submission
const formData = new FormData(this);
// Access form data
console.log('Name:', formData.get('name'));
console.log('Email:', formData.get('email'));
// Handle form data (e.g., send to server via fetch API)
});
</script>
Common Pitfalls
- Missing Labels: Ensure that each form control has an associated label to improve accessibility.
- Inadequate Validation: Use both HTML5 validation attributes and server-side validation to ensure data integrity.
- Form Security: Be aware of potential security issues, such as cross-site scripting (XSS) and cross-site request forgery (CSRF).
Example
Hereβs a complete example of a simple form with various input types, validation, and JavaScript handling:
<!DOCTYPE html>
<html>
<head>
<title>HTML Form Example</title>
</head>
<body>
<h1>Contact Us</h1>
<form id="contactForm" action="/submit" method="post">
<label for="name">Name:</label>
<input type="text" id="name" name="name" required>
<label for="email">Email:</label>
<input type="email" id="email" name="email" required>
<label for="message">Message:</label>
<textarea id="message" name="message" required></textarea>
<button type="submit">Send</button>
</form>
<script>
document.getElementById('contactForm').addEventListener('submit', function(event) {
event.preventDefault(); // Prevents default form submission
alert('Form submitted successfully!');
});
</script>
</body>
</html>
Conclusion
HTML forms are a fundamental part of web development, enabling users to interact with web applications and submit data. By understanding the key elements, attributes, and best practices, you can create effective and user-friendly forms for various purposes.
Join our Community:
Author
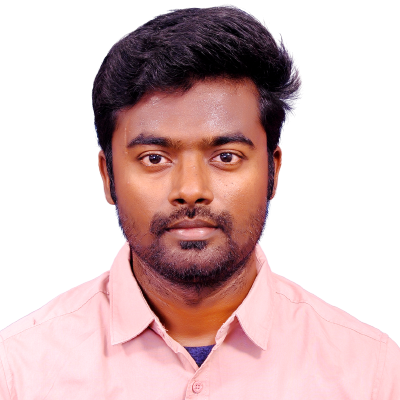
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (HTML Form), please comment here. I will help you immediately.