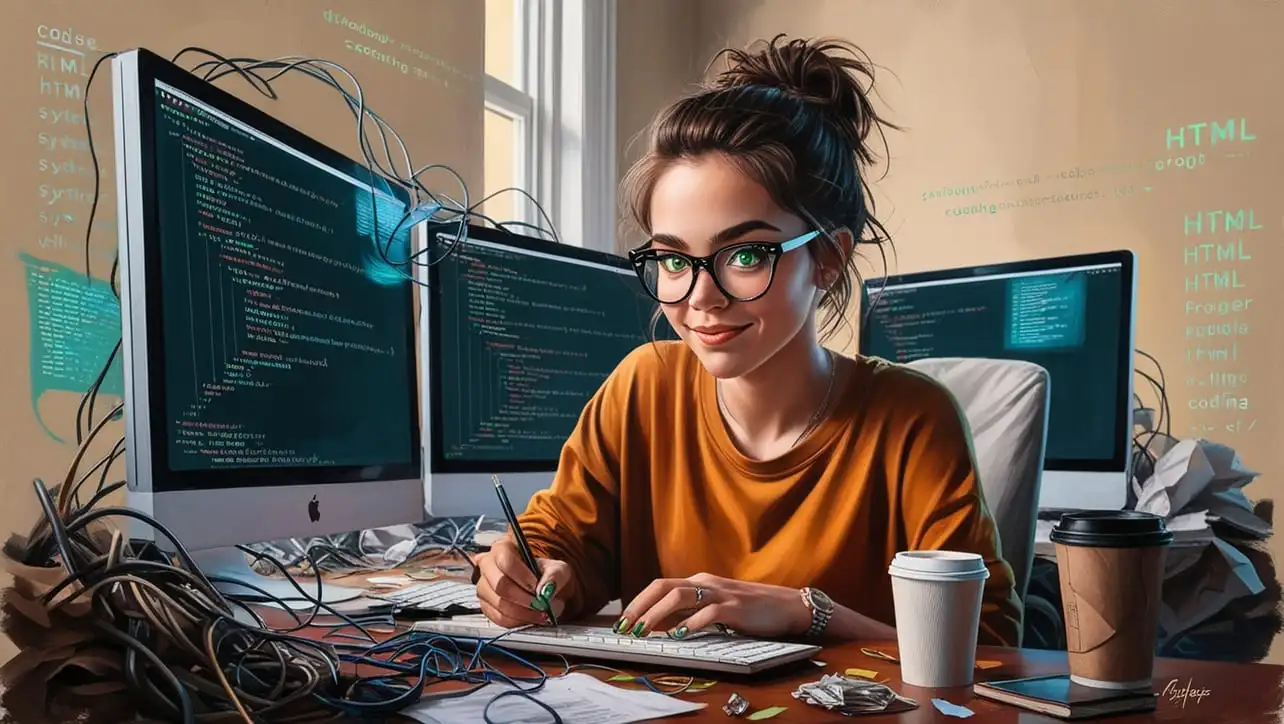
HTML Basic
HTML Entity
- HTML Entity
- HTML Alphabet Entity
- HTML Arrow Entity
- HTML Currency Entity
- HTML Math Entity
- HTML Number Entity
- HTML Punctuation Entity
- HTML Symbol Entity
HTML IndexedDB
HTML Reference
HTML Events
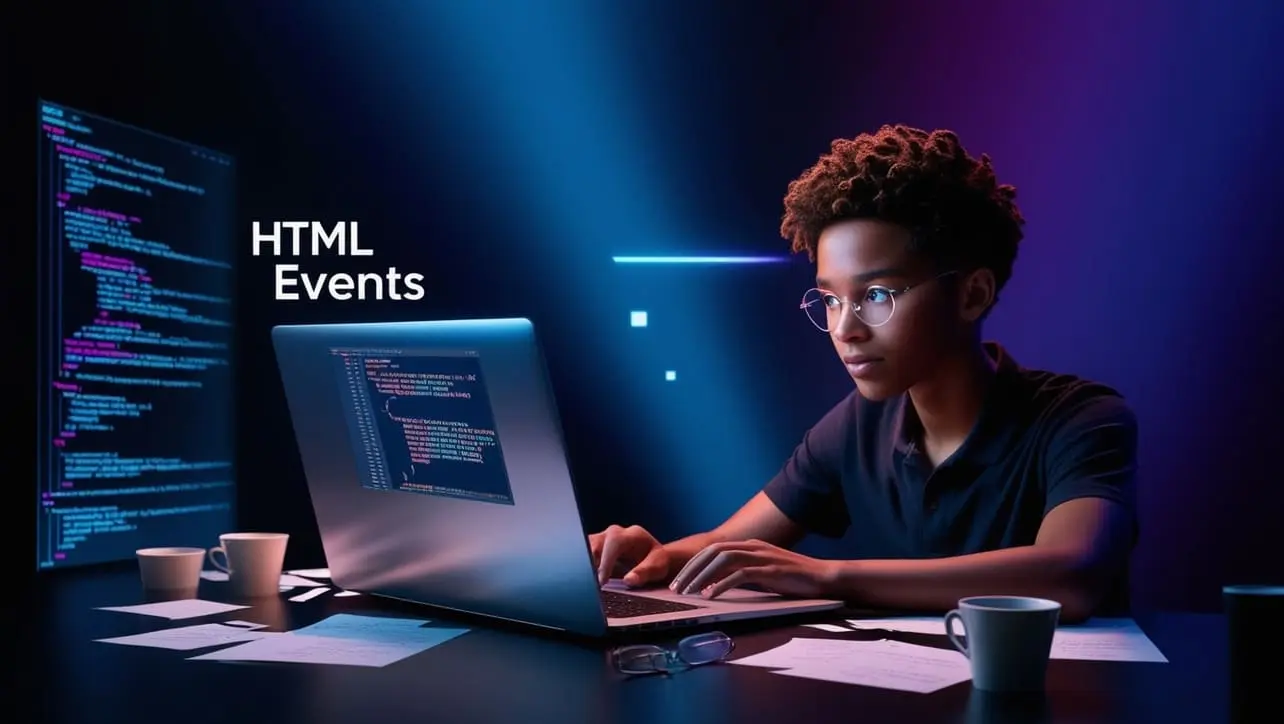
Photo Credit to CodeToFun
Introduction
HTML events are actions or occurrences that happen in the web browser. These events are triggered by user interactions like clicking, hovering, pressing a key, or system-generated events like page load or resize. By responding to these events, developers can create dynamic, interactive web applications.
What Are HTML Events?
HTML events represent actions triggered either by users or the browser. These events are vital for making web pages interactive and responsive. They range from basic interactions like mouse clicks to complex system events like resizing the browser window.
Types of Events
HTML events can be broadly classified into different categories based on the type of interaction:
- Mouse Events: Triggered by actions like clicking, hovering, or scrolling.
- Keyboard Events: Triggered by key presses or releases on the keyboard.
- Form Events: Related to form elements such as submission or input changes.
- Window Events: Events that are triggered by the browser or window actions, like resizing or scrolling.
Event Handlers
An event handler is a function that responds to a specific event. You can add event handlers using inline HTML attributes, properties in JavaScript, or modern addEventListener()
methods.
Inline Event Handler:
HTMLCopied<button onclick="alert('Button clicked!')">Click me</button>
JavaScript Event Handler::
JavascriptCopieddocument.getElementById('myButton').onclick = function() { alert('Button clicked!'); };
Using addEventListener():
JavascriptCopieddocument.getElementById('myButton').addEventListener('click', function() { alert('Button clicked!'); });
Event Bubbling and Capturing
Events propagate through the DOM in two phases: capturing and bubbling. During the capturing phase, the event moves from the document's root down to the target element. In the bubbling phase, the event propagates back up from the target to the root.
element.addEventListener('click', function() {
console.log('Bubbling phase');
}, false); // false means bubbling phase
element.addEventListener('click', function() {
console.log('Capturing phase');
}, true); // true means capturing phase
Common Events
- Mouse Events:
- click: Triggered when an element is clicked.
- mouseover: Triggered when the mouse hovers over an element.
- mousedown: Triggered when a mouse button is pressed.
- Keyboard Events:
- keydown: Triggered when a key is pressed.
- keyup: Triggered when a key is released.
- Form Events:
- submit: Triggered when a form is submitted.
- change: Triggered when the value of an input changes.
- Window Events:
- resize: Triggered when the window is resized.
- scroll: Triggered when the page is scrolled.
Handling Custom Events
You can also create and dispatch custom events using JavaScript, which allows you to trigger your own specific event logic.
const event = new CustomEvent('myCustomEvent', {
detail: { message: 'Hello, World!' }
});
document.addEventListener('myCustomEvent', function(e) {
console.log(e.detail.message); // Output: Hello, World!
});
// Dispatch the custom event
document.dispatchEvent(event);
Best Practices
- Use addEventListener(): It’s more flexible than using inline event handlers or properties and supports multiple events on the same element.
- Avoid Polluting Global Scope: Avoid using inline handlers as they can create clutter and conflict with other JavaScript functions.
- Debouncing: For events like
scroll
orresize
, consider debouncing to prevent performance issues from too many rapid event triggers. - Event Delegation: Use event delegation to handle events efficiently for dynamically generated elements, especially when working with lists or tables.
Example
Here's an example demonstrating the use of event listeners and event bubbling:
<!DOCTYPE html>
<html>
<head>
<title>Event Example</title>
</head>
<body>
<div id="parent">
<button id="myButton">Click Me</button>
</div>
<script>
document.getElementById('myButton').addEventListener('click', function(event) {
alert('Button clicked!');
});
document.getElementById('parent').addEventListener('click', function(event) {
alert('Parent clicked!');
}, false); // Bubbling phase
</script>
</body>
</html>
In this example, clicking the button will first trigger the button’s event listener, followed by the parent’s listener due to event bubbling.
Conclusion
HTML events are key to creating interactive, dynamic web pages. By understanding how to handle and manipulate events, you can build responsive and engaging user interfaces. Make sure to manage events efficiently using techniques like event delegation and proper error handling.
👨💻 Join our Community:
Author
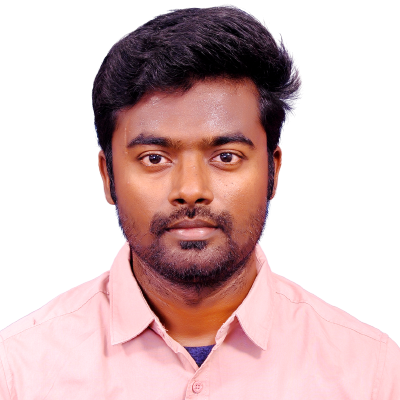
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee