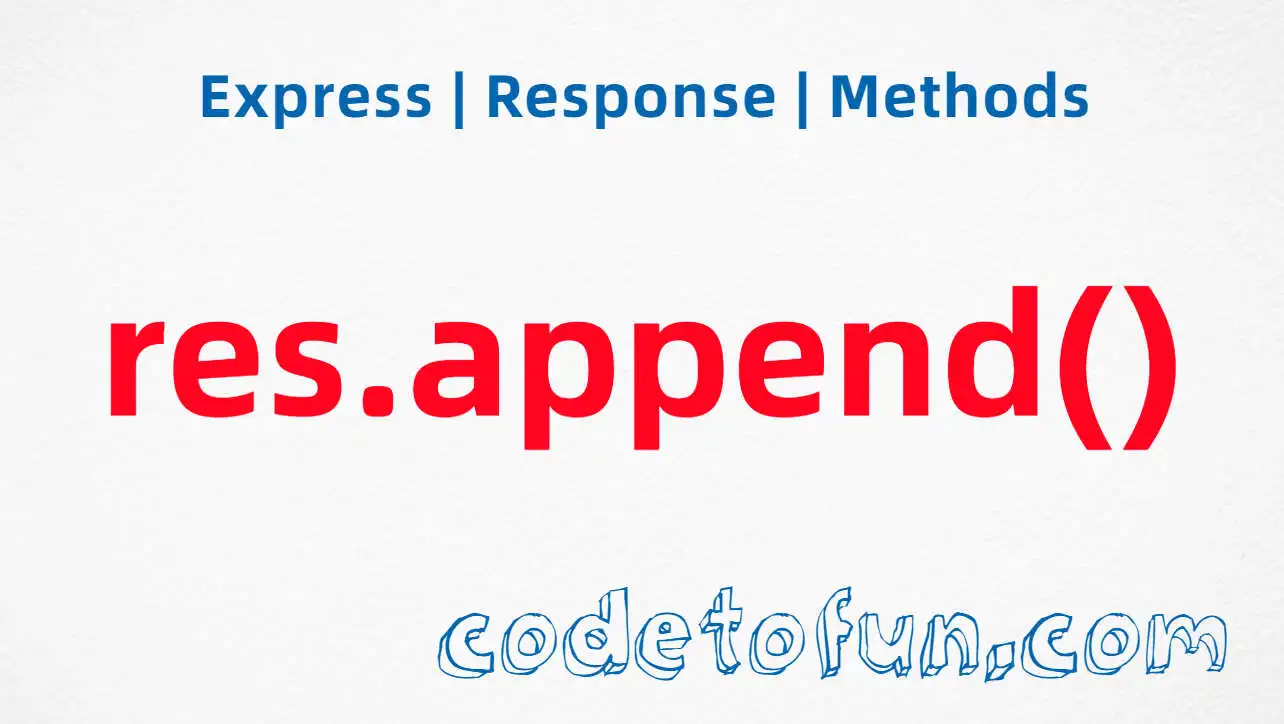
Express.js Basic
- express Intro
- express express()
- express Application
- express Request
Properties
- req.app
- req.baseUrl
- req.body
- req.cookies
- req.fresh
- req.host
- req.hostname
- req.ip
- req.ips
- req.method
- req.originalUrl
- req.params
- req.path
- req.protocol
- req.query
- req.res
- req.route
- req.secure
- req.signedCookies
- req.stale
- req.subdomains
- req.xhr
Methdos
- express Response
- express Router
Express req.xhr Property
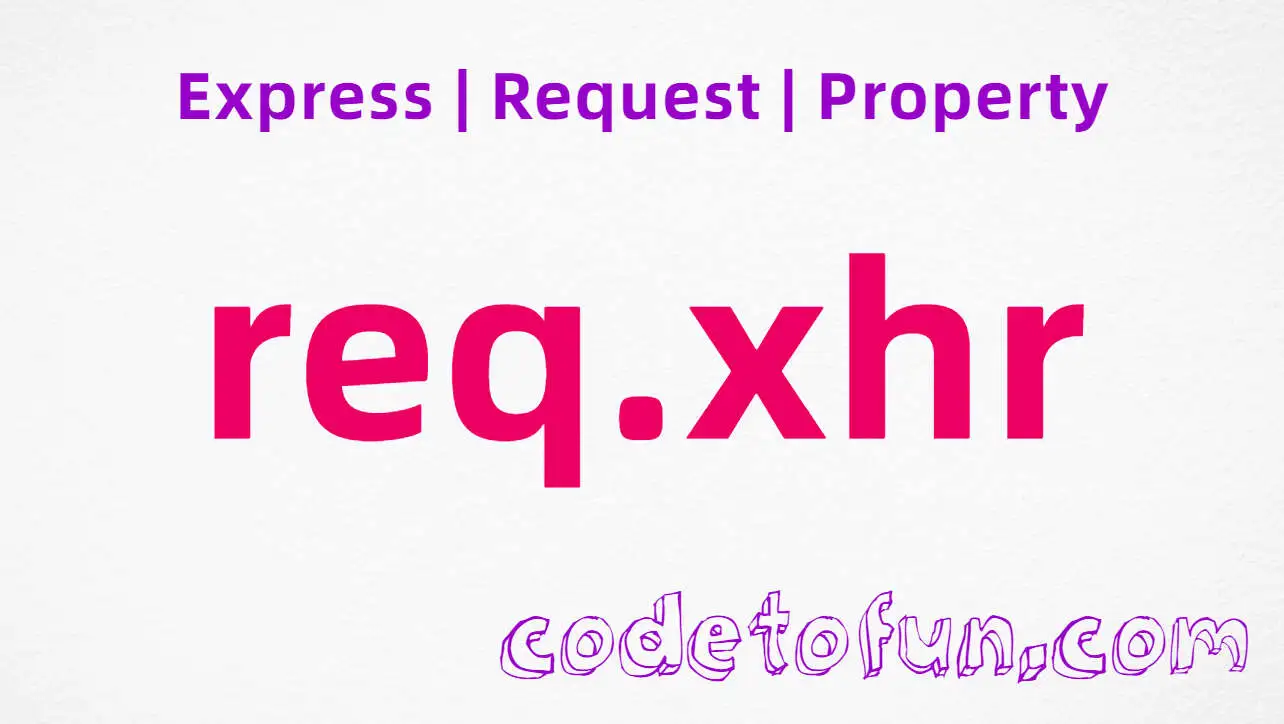
Photo Credit to CodeToFun
🙋 Introduction
In web development, distinguishing between regular HTTP requests and AJAX (Asynchronous JavaScript and XML) requests is crucial for delivering a seamless user experience.
Express.js provides the req.xhr
property, allowing you to identify AJAX requests within your route handlers and middleware functions.
This guide will delve into the syntax, usage, and practical examples of the req.xhr
property in Express.js.
💡 Syntax
The syntax for using the req.xhr
property is simple:
if (req.xhr) {
// Code to handle AJAX request
} else {
// Code to handle regular HTTP request
}
❓ How req.xhr Works
The req.xhr
property in Express.js is a boolean value that indicates whether the request was made with XMLHttpRequest (commonly used in AJAX requests). When req.xhr
is true, it signifies an AJAX request, and when false, it denotes a regular HTTP request.
app.get('/api/data', (req, res) => {
if (req.xhr) {
// Respond to AJAX request
res.json({ data: 'AJAX response' });
} else {
// Respond to regular HTTP request
res.send('Regular HTTP response');
}
});
In this example, the route /api/data checks req.xhr
to differentiate between an AJAX request and a regular HTTP request, responding accordingly.
📚 Use Cases
Dynamic Content Loading:
Utilize
req.xhr
to load partial content for AJAX requests, enhancing the user experience with dynamic updates.example.jsCopiedapp.get('/dynamic-content', (req, res) => { if (req.xhr) { // Load partial content for AJAX requests res.render('partials/dynamic-content'); } else { // Render full page for regular HTTP requests res.render('full-page'); } });
Conditional Responses:
Customize responses based on the request type using
req.xhr
, providing different formats for AJAX and regular requests.example.jsCopiedapp.get('/api/user/:id', (req, res) => { const userId = req.params.id; const userData = getUserData(userId); if (req.xhr) { // Respond with JSON for AJAX requests res.json(userData); } else { // Render user profile page for regular HTTP requests res.render('user-profile', { userData }); } });
🏆 Best Practices
Consistent API Responses:
Ensure consistency in API responses by using
req.xhr
to format JSON responses for AJAX requests.example.jsCopiedapp.get('/api/data', (req, res) => { if (req.xhr) { // Consistent JSON response for AJAX requests res.json({ data: 'AJAX response' }); } else { // Handle non-AJAX requests as needed res.send('Regular HTTP response'); } });
Graceful Degradation:
Implement graceful degradation by providing fallbacks for non-AJAX scenarios, ensuring a functional user experience in all situations.
example.jsCopiedapp.get('/fallback', (req, res) => { if (req.xhr) { // Provide AJAX-specific content res.json({ message: 'AJAX response' }); } else { // Fallback for non-AJAX requests res.send('Fallback content for regular HTTP request'); } });
🎉 Conclusion
The req.xhr
property in Express.js is a valuable tool for identifying AJAX requests and tailoring your server's responses accordingly. By understanding how to use req.xhr
effectively, you can enhance your Express.js applications with dynamic and responsive behavior.
Now, equipped with knowledge about req.xhr
, go ahead and implement AJAX-aware features in your Express.js projects!
👨💻 Join our Community:
Author
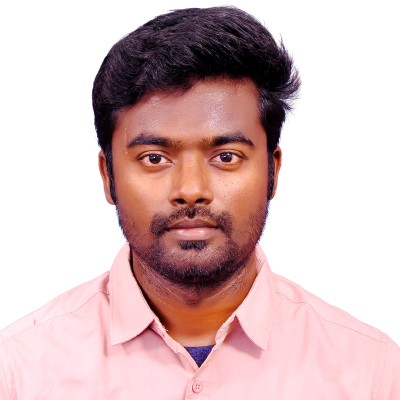
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (Express req.xhr Property), please comment here. I will help you immediately.