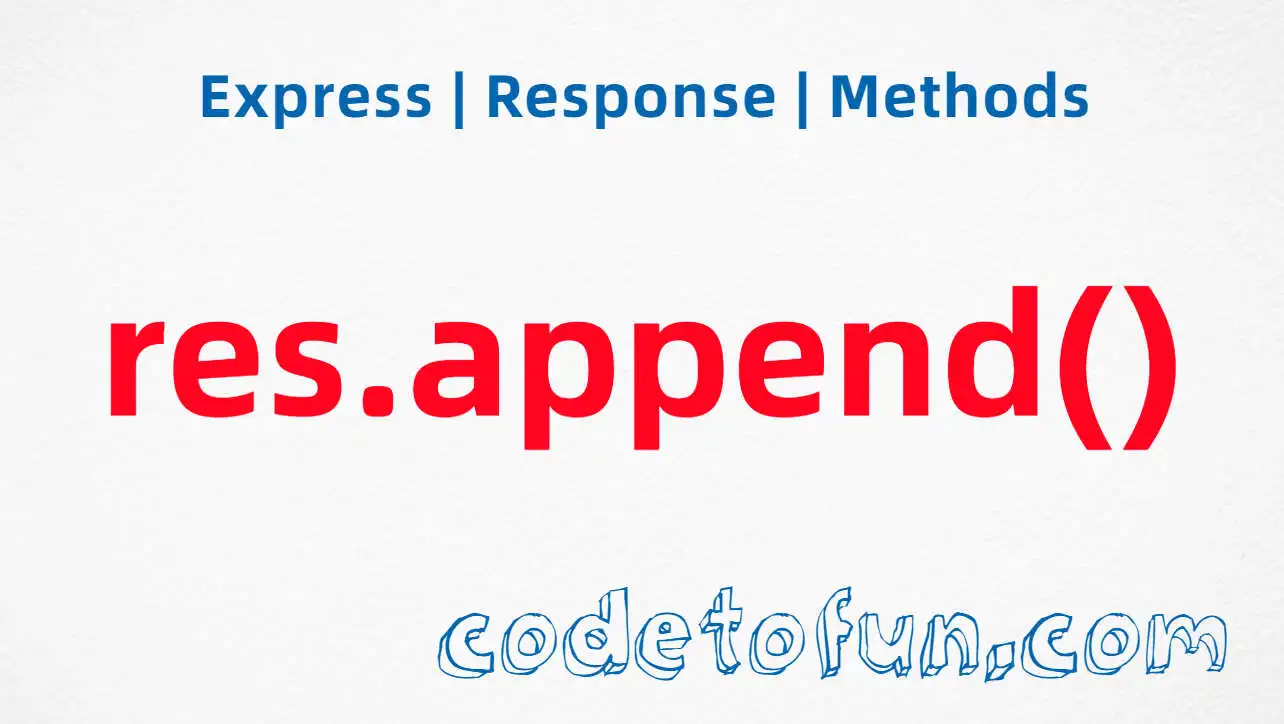
Express.js Basic
- express Intro
- express express()
- express Application
- express Request
Properties
- req.app
- req.baseUrl
- req.body
- req.cookies
- req.fresh
- req.host
- req.hostname
- req.ip
- req.ips
- req.method
- req.originalUrl
- req.params
- req.path
- req.protocol
- req.query
- req.res
- req.route
- req.secure
- req.signedCookies
- req.stale
- req.subdomains
- req.xhr
Methdos
- express Response
- express Router
Express req.signedCookies Property
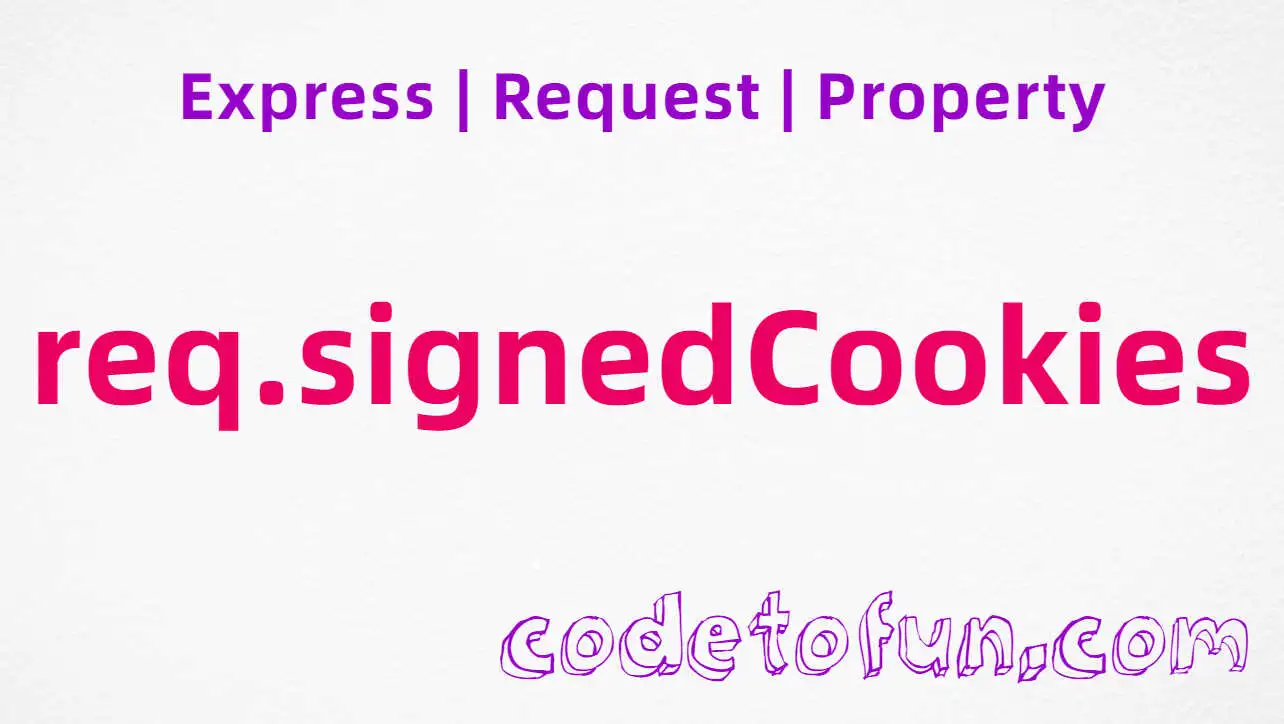
Photo Credit to CodeToFun
🙋 Introduction
In web development, handling cookies is a common practice for storing and retrieving user-related information. Express.js, a popular Node.js web application framework, provides the req.signedCookies
property to securely handle signed cookies.
This guide will walk you through the syntax, usage, and best practices for utilizing req.signedCookies
in your Express.js applications.
💡 Syntax
The syntax for accessing signed cookies using req.signedCookies
is straightforward:
const value = req.signedCookies.cookieName;
- cookieName: The name of the signed cookie you want to access.
❓ How req.signedCookies Works
When cookies are signed, they include a cryptographic signature, ensuring that the cookie data has not been tampered with. The req.signedCookies
property in Express.js allows you to access the values of signed cookies in a secure manner. This is particularly useful for sensitive data that should not be modified by clients.
// Set a signed cookie
app.get('/setcookie', (req, res) => {
res.cookie('user', { name: 'John Doe' }, { signed: true });
res.send('Cookie set');
});
// Access the signed cookie
app.get('/getcookie', (req, res) => {
const userName = req.signedCookies.user.name;
res.send(`Hello, ${userName}!`);
});
In this example, a signed cookie named 'user' is set in one route, and its value is accessed securely using req.signedCookies
in another route.
📚 Use Cases
User Authentication:
Utilize
req.signedCookies
for securely handling user authentication information stored in signed cookies.example.jsCopied// Middleware for user authentication using signed cookies const authenticateUser = (req, res, next) => { const userId = req.signedCookies.userId; // Implement your authentication logic using the userId // ... next(); }; // Apply the authentication middleware to a route app.get('/securepage', authenticateUser, (req, res) => { res.send('Welcome to the secure page!'); });
Session Management:
Securely manage user sessions by using
req.signedCookies
for signed cookie-based session data.example.jsCopied// Middleware for session management using signed cookies const manageSession = (req, res, next) => { const sessionId = req.signedCookies.sessionId; // Implement session management logic using the sessionId // ... next(); }; // Apply the session management middleware to all routes app.use(manageSession);
🏆 Best Practices
Enable Cookie Signing:
Always enable cookie signing when setting cookies that contain sensitive information.
example.jsCopied// Enable cookie signing globally app.use(cookieParser('your-secret-key'));
Secure Sensitive Data:
Reserve the use of signed cookies for sensitive data that should not be manipulated by clients.
example.jsCopied// Set a signed cookie with sensitive information app.get('/setsensitivecookie', (req, res) => { res.cookie('token', 'sensitiveTokenValue', { signed: true }); res.send('Sensitive cookie set'); });
🎉 Conclusion
The req.signedCookies
property in Express.js is a valuable tool for securely handling signed cookies in your web applications. By following best practices and leveraging this feature, you can enhance the security of user authentication, session management, and other scenarios where secure cookie handling is crucial.
Now equipped with knowledge about req.signedCookies
, go ahead and implement secure cookie handling in your Express.js projects!
👨💻 Join our Community:
Author
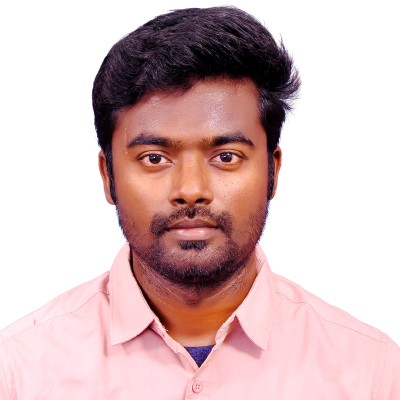
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (Express req.signedCookies Property), please comment here. I will help you immediately.