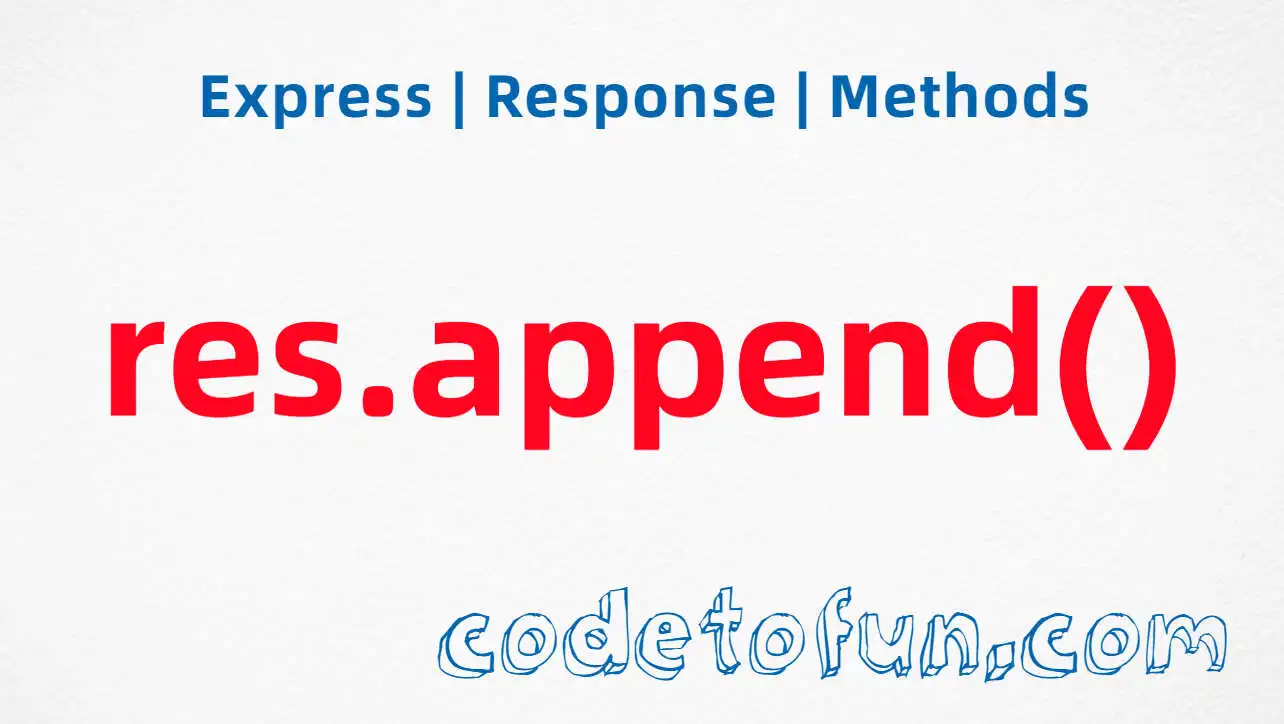
Express.js Basic
- express Intro
- express express()
- express Application
- express Request
Properties
- req.app
- req.baseUrl
- req.body
- req.cookies
- req.fresh
- req.host
- req.hostname
- req.ip
- req.ips
- req.method
- req.originalUrl
- req.params
- req.path
- req.protocol
- req.query
- req.res
- req.route
- req.secure
- req.signedCookies
- req.stale
- req.subdomains
- req.xhr
Methdos
- express Response
- express Router
Express req.range() Method
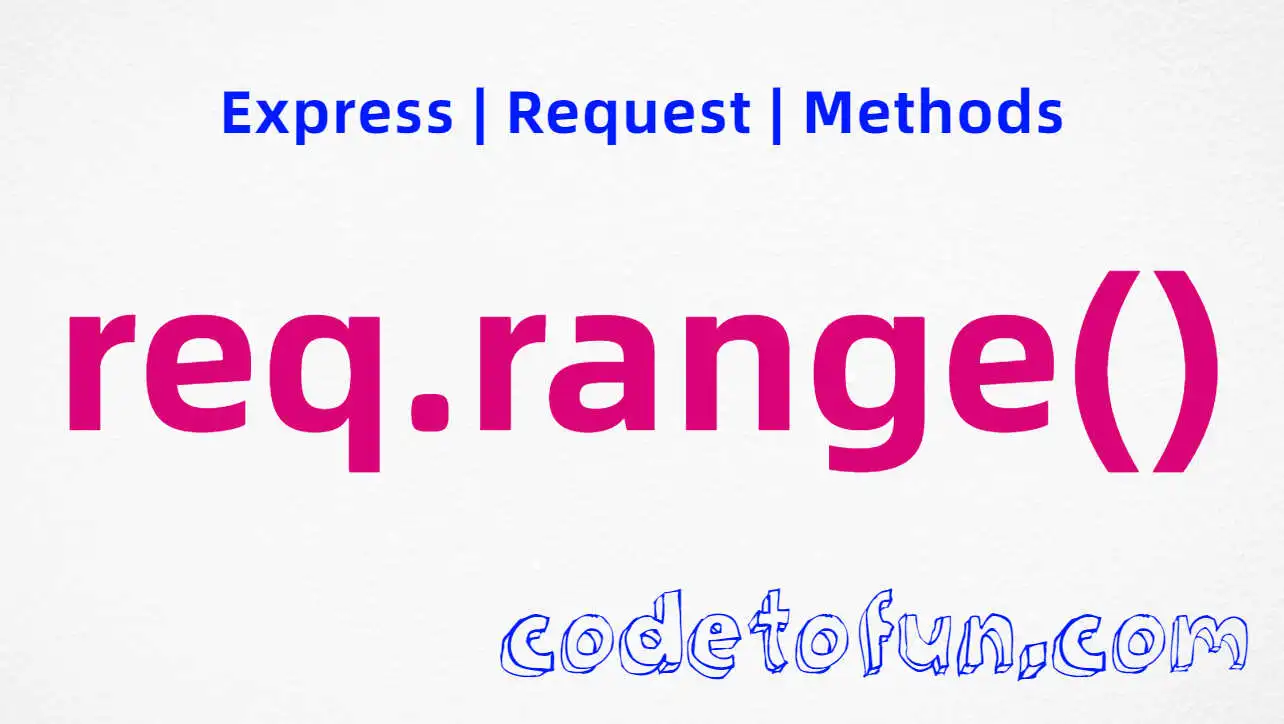
Photo Credit to CodeToFun
🙋 Introduction
Handling range requests efficiently is crucial for optimizing the performance of web applications. Express.js provides a helpful method, req.range()
, which simplifies the process of dealing with HTTP range requests.
In this guide, we'll delve into the syntax, functionality, and practical use cases of the req.range()
method.
💡 Syntax
The req.range()
method is used within a route handler to parse and extract the range information from the HTTP request headers. The syntax is as follows:
const range = req.range(size, options);
- size (optional): The total size of the resource being requested. It helps determine the length of the requested range.
- options (optional): Additional options that can be provided, such as combine to merge overlapping ranges.
❓ How req.range() Works
The req.range()
method simplifies the handling of Range requests specified by the Range HTTP header. It parses the header and returns an array of ranges, making it easier for developers to respond with the requested portion of a resource.
app.get('/video', (req, res) => {
const videoPath = 'path/to/video.mp4';
const videoSize = fs.statSync(videoPath).size;
// Parse the Range header
const range = req.range(videoSize);
// If no valid ranges are specified, or if the range is invalid, respond with 416 Range Not Satisfiable
if (!range) {
res.status(416).end();
return;
}
// Respond with the requested range
res.status(206).sendFile(videoPath, {
start: range[0].start,
end: range[0].end,
});
});
In this example, the route handler for /video uses req.range()
to parse the Range header and responds with the specified portion of the video file.
📚 Use Cases
Streaming Large Files:
Use
req.range()
to efficiently stream large files by responding with the specified range, facilitating smoother downloads.example.jsCopiedapp.get('/large-file', (req, res) => { const filePath = 'path/to/large-file.zip'; const fileSize = fs.statSync(filePath).size; // Parse the Range header const range = req.range(fileSize); // If no valid ranges are specified, or if the range is invalid, respond with 416 Range Not Satisfiable if (!range) { res.status(416).end(); return; } // Respond with the requested range res.status(206).sendFile(filePath, { start: range[0].start, end: range[0].end, }); });
🏆 Best Practices
Check for Valid Ranges:
Always check the result of
req.range()
to ensure valid range requests. Respond with an appropriate status code (e.g., 416 Range Not Satisfiable) if the requested range is invalid.example.jsCopiedconst range = req.range(size); if (!range) { res.status(416).end(); return; }
Combine Overlapping Ranges:
Consider using the combine option when calling
req.range()
to merge overlapping ranges. This can simplify your logic when responding to multiple range requests.example.jsCopiedconst range = req.range(size, { combine: true });
🎉 Conclusion
The req.range()
method in Express.js provides a convenient way to handle Range requests, making it easier to implement efficient streaming of partial content. By understanding its syntax, functionality, and best practices, you can optimize your Express.js applications for better performance when serving partial content.
Now, armed with knowledge about the req.range()
method, go ahead and enhance your Express.js projects for improved handling of range requests!
👨💻 Join our Community:
Author
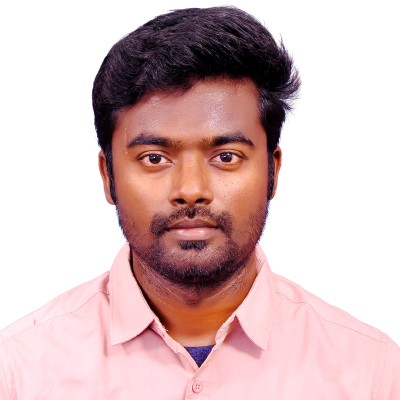
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (Express req.range() Method), please comment here. I will help you immediately.