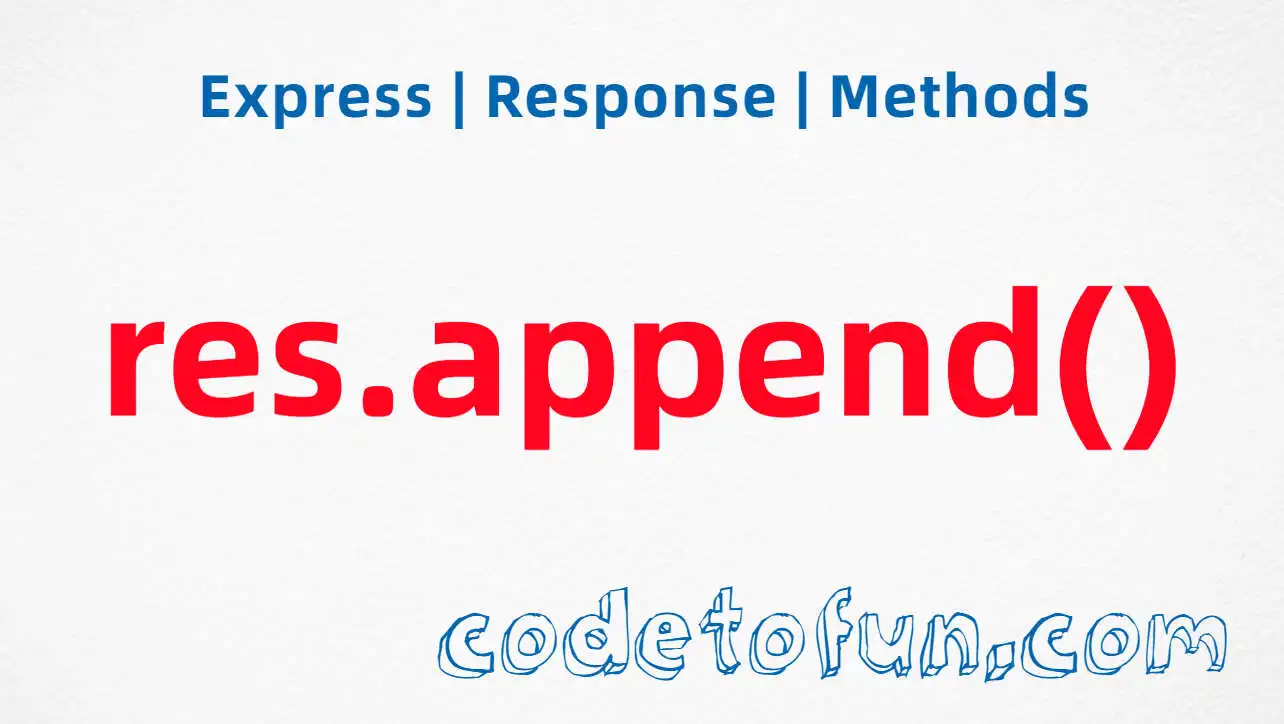
Express.js Basic
- express Intro
- express express()
- express Application
- express Request
Properties
- req.app
- req.baseUrl
- req.body
- req.cookies
- req.fresh
- req.host
- req.hostname
- req.ip
- req.ips
- req.method
- req.originalUrl
- req.params
- req.path
- req.protocol
- req.query
- req.res
- req.route
- req.secure
- req.signedCookies
- req.stale
- req.subdomains
- req.xhr
Methdos
- express Response
- express Router
Express req.query Property
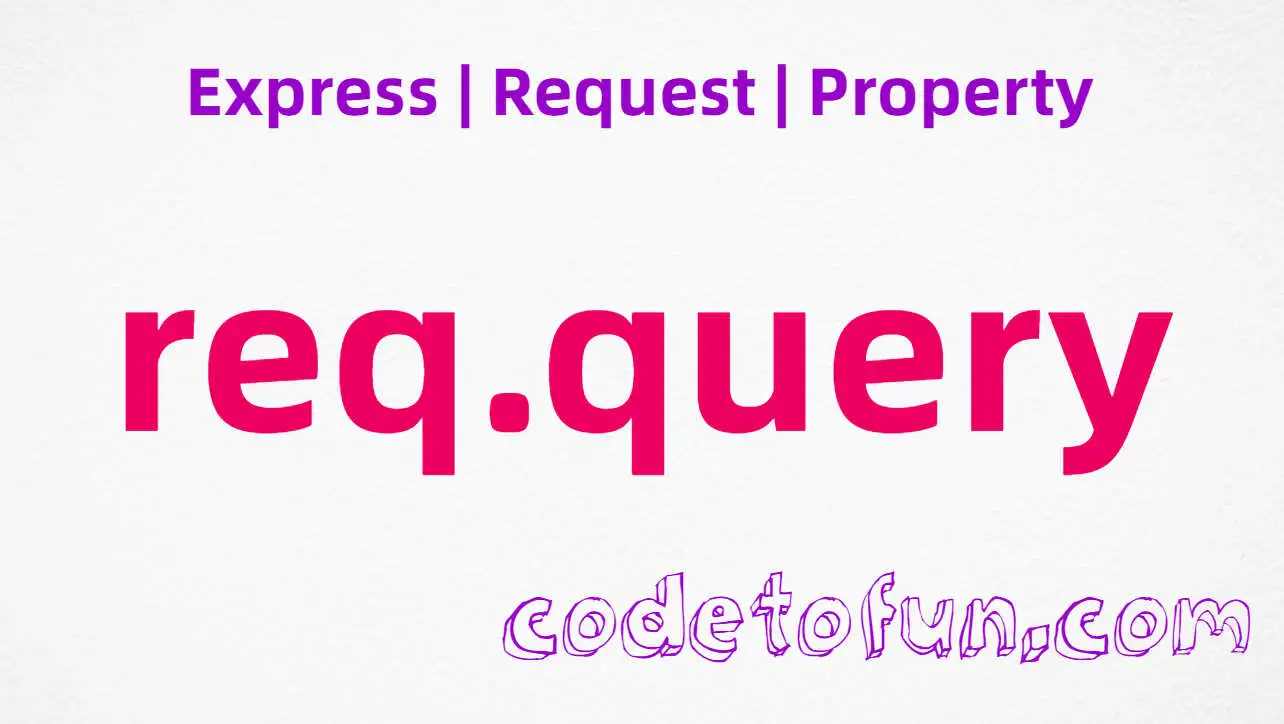
Photo Credit to CodeToFun
🙋 Introduction
In web development, handling user input is crucial, and Express.js provides a straightforward way to extract data from URL query parameters using the req.query
object.
In this guide, we'll explore the syntax, usage, and practical examples of working with req.query
to enhance your Express.js applications.
💡 Syntax
The syntax for accessing URL query parameters in Express.js using req.query
is simple:
const parameterValue = req.query.parameterName;
- parameterName: The name of the query parameter you want to retrieve.
- parameterValue: The value of the specified query parameter.
❓ How req.query Works
Express.js parses URL query parameters and makes them accessible through the req.query
object. This object contains key-value pairs representing the query parameters sent with the request.
// Route handler for handling query parameters
app.get('/search', (req, res) => {
const query = req.query.q; // Retrieve the 'q' parameter from the query string
res.send(`Search query: ${query}`);
});
In this example, a route handler captures the 'q' query parameter from the URL and responds with the search query.
📚 Use Cases
Search Functionality:
Utilize
req.query
to implement search functionality in your application by extracting the search query from the URL.example.jsCopiedapp.get('/search', (req, res) => { const query = req.query.q; // Perform search based on the 'q' parameter // Return search results });
Filtering and Pagination:
Apply query parameters to filter and paginate results, enhancing the user experience when dealing with large datasets.
example.jsCopiedapp.get('/products', (req, res) => { const category = req.query.category; const page = req.query.page || 1; // Fetch products based on category and pagination parameters });
🏆 Best Practices
Handle Missing Parameters:
Check for the existence of required query parameters and handle missing parameters gracefully to prevent errors.
example.jsCopiedapp.get('/profile', (req, res) => { const userId = req.query.userId; if (!userId) { return res.status(400).send('Missing userId parameter'); } // Logic to fetch user profile based on userId });
Sanitize and Validate:
Sanitize and validate query parameters to ensure they meet the expected criteria, preventing security vulnerabilities or unexpected behavior.
example.jsCopiedapp.get('/search', (req, res) => { const query = req.query.q; // Validate the query parameter if (!query || query.length < 3) { return res.status(400).send('Invalid search query'); } // Perform search based on the validated 'q' parameter });
🎉 Conclusion
The req.query
object in Express.js simplifies the extraction of URL query parameters, providing a convenient way to handle user input. By understanding the syntax, exploring use cases, and adopting best practices, you can leverage the power of req.query
to enhance the functionality and user experience of your Express.js applications.
Now, armed with knowledge about req.query
, go ahead and incorporate this feature into your Express.js projects for efficient handling of URL query parameters!
👨💻 Join our Community:
Author
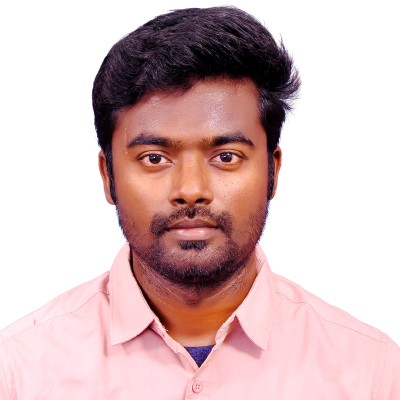
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (Express req.query Property), please comment here. I will help you immediately.