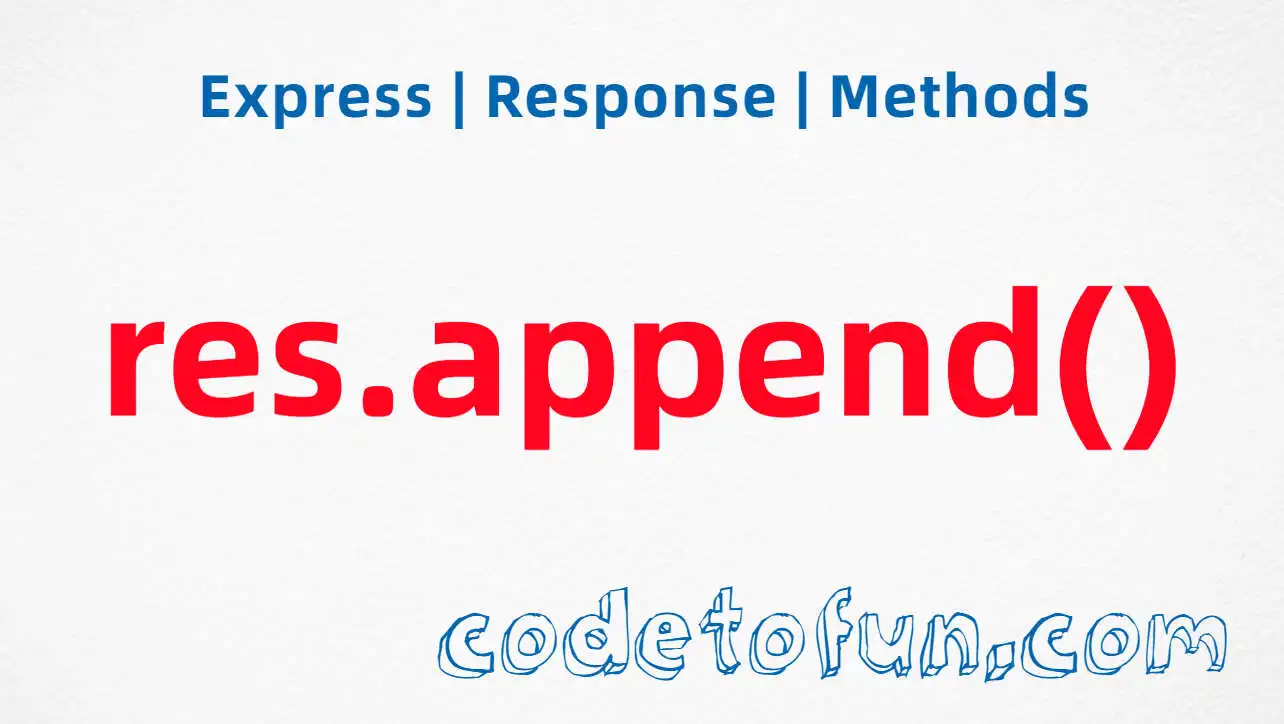
Express.js Basic
- express Intro
- express express()
- express Application
- express Request
Properties
- req.app
- req.baseUrl
- req.body
- req.cookies
- req.fresh
- req.host
- req.hostname
- req.ip
- req.ips
- req.method
- req.originalUrl
- req.params
- req.path
- req.protocol
- req.query
- req.res
- req.route
- req.secure
- req.signedCookies
- req.stale
- req.subdomains
- req.xhr
Methdos
- express Response
- express Router
Express req.params Property
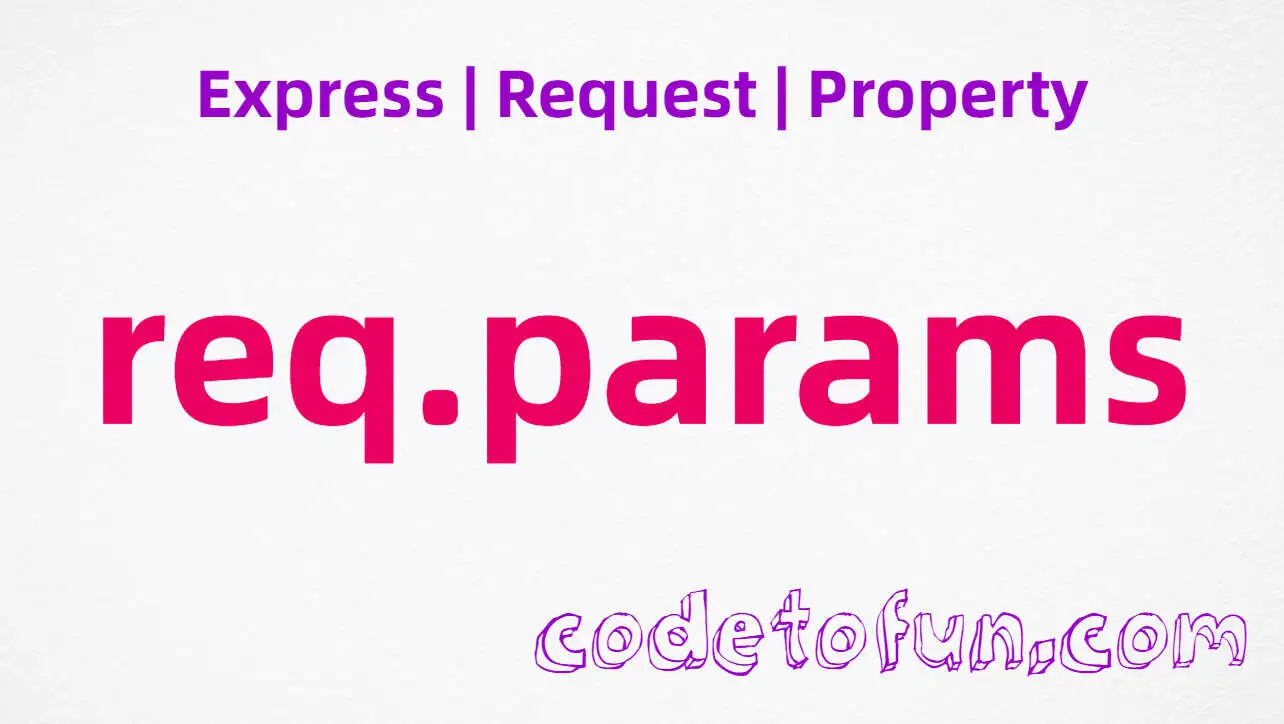
Photo Credit to CodeToFun
🙋 Introduction
Express.js, a powerful web application framework for Node.js, provides mechanisms to handle dynamic values in routes through the req.params
object.
This guide focuses on understanding the req.params
properties, which allow you to extract parameters from URL patterns dynamically.
💡 Syntax
The req.params
object in Express.js automatically captures parameters specified in the route path:
app.get('/users/:userId', (req, res) => {
const userId = req.params.userId;
// Your logic with userId
});
In this example, :userId is a route parameter that can be accessed using req.params.userId
❓ How req.params Works
When a route includes parameters defined with a colon (:) syntax, Express.js automatically populates the req.params
object with the corresponding values from the actual URL during the request lifecycle.
app.get('/users/:userId/books/:bookId', (req, res) => {
const userId = req.params.userId;
const bookId = req.params.bookId;
// Your logic with userId and bookId
});
Here, :userId and :bookId are parameters that can be accessed using req.params.userId and req.params.bookId respectively.
📚 Use Cases
Retrieving User Information:
Use
req.params
to dynamically retrieve user information based on the specified username in the route.example.jsCopiedapp.get('/profile/:username', (req, res) => { const username = req.params.username; // Fetch user information based on the username });
Handling Product IDs:
Leverage
req.params
to handle requests for specific product details by extracting the product ID from the route.example.jsCopiedapp.get('/products/:productId', (req, res) => { const productId = req.params.productId; // Fetch product details using the productId });
🏆 Best Practices
Validate Parameters:
Always validate parameters obtained from
req.params
to ensure they meet your application's requirements.example.jsCopiedapp.get('/users/:userId', (req, res) => { const userId = req.params.userId; // Validate userId if (!isValidUserId(userId)) { return res.status(400).send('Invalid user ID'); } // Continue with the logic });
Combine with Other Middleware:
Integrate
req.params
with other middleware functions for additional processing or validation before reaching the route handler.example.jsCopied// Middleware to check if the user is authenticated const authenticateUser = (req, res, next) => { // Your authentication logic }; app.get('/secured/:resourceId', authenticateUser, (req, res) => { // This route is only accessible for authenticated users });
🎉 Conclusion
Understanding and effectively using the req.params
properties in Express.js allows you to build dynamic and parameterized routes in your web applications. By following best practices, you can ensure the security and reliability of your route parameter handling.
Now, equipped with knowledge about req.params
, go ahead and create dynamic routes in your Express.js projects with confidence!
👨💻 Join our Community:
Author
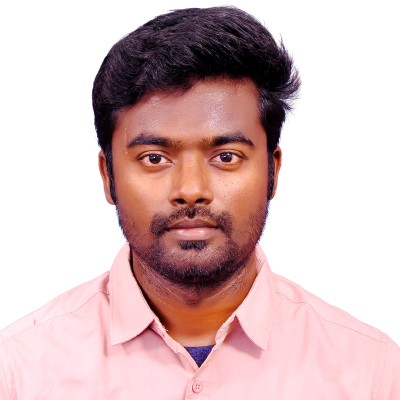
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (Express req.params Property), please comment here. I will help you immediately.