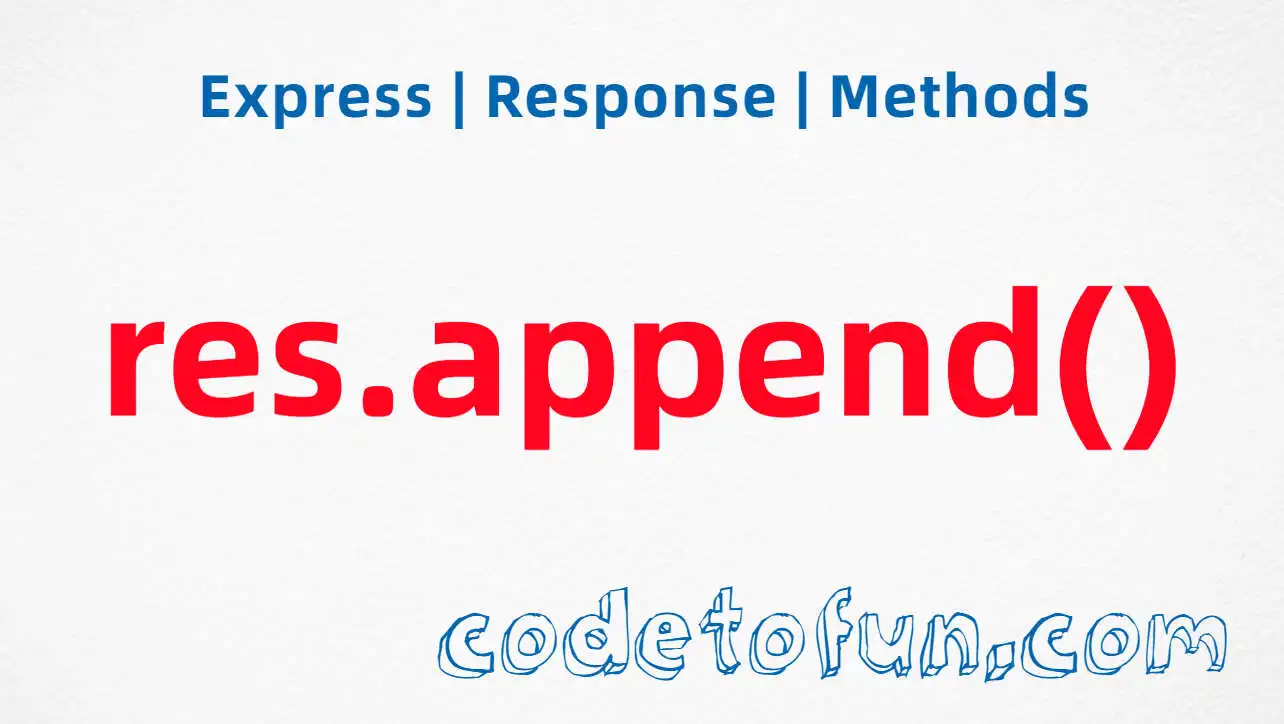
Express.js Basic
- express Intro
- express express()
- express Application
- express Request
Properties
- req.app
- req.baseUrl
- req.body
- req.cookies
- req.fresh
- req.host
- req.hostname
- req.ip
- req.ips
- req.method
- req.originalUrl
- req.params
- req.path
- req.protocol
- req.query
- req.res
- req.route
- req.secure
- req.signedCookies
- req.stale
- req.subdomains
- req.xhr
Methdos
- express Response
- express Router
Express req.fresh Property
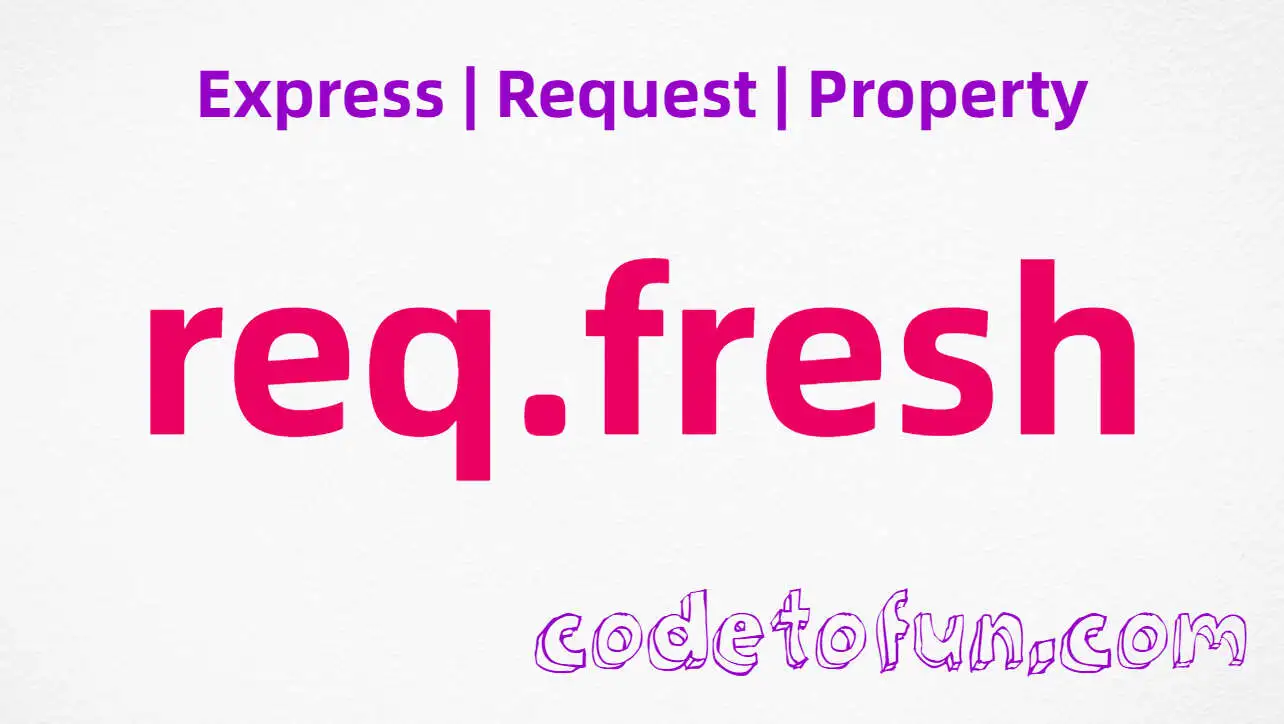
Photo Credit to CodeToFun
🙋 Introduction
Conditional GET requests play a crucial role in optimizing web performance by reducing unnecessary data transfer. In Express.js, the req.fresh
property is a handy tool for handling conditional requests and efficiently managing the delivery of content.
In this guide, we'll explore the syntax, usage, and practical examples of req.fresh
.
💡 Syntax
The req.fresh
property is a Boolean value that indicates whether the requested resource is considered fresh.
if (req.fresh) {
// Resource is fresh; send 304 Not Modified
res.status(304).end();
} else {
// Resource is not fresh; proceed with sending the updated content
res.send('Updated content');
}
❓ How req.fresh Works
When a client sends a conditional GET request with the If-None-Match and If-Modified-Since headers, Express.js compares these headers with the resource's current ETag and last-modified timestamp. The req.fresh
property is then set to true if the resource is considered fresh and hasn't been modified since the client's last request.
app.get('/content', (req, res) => {
// Check if the resource is fresh
if (req.fresh) {
// Resource is fresh; send 304 Not Modified
res.status(304).end();
} else {
// Resource is not fresh; proceed with sending the updated content
res.send('Updated content');
}
});
In this example, the route handler uses req.fresh
to determine whether the content should be sent or if a 304 Not Modified status should be returned.
📚 Use Cases
Efficient Resource Delivery:
Use
req.fresh
to efficiently deliver resources like images by avoiding unnecessary data transfer when the client already has the latest version.efficient-resource-delivery.jsCopiedapp.get('/images/:id', (req, res) => { const imageId = req.params.id; // Check if the image is fresh before sending if (req.fresh) { res.status(304).end(); } else { // Logic to fetch and send the image const image = fetchImageById(imageId); res.send(image); } });
Conditional Content Updates:
Leverage
req.fresh
to conditionally update content only when the client requests the latest version, reducing server load and bandwidth usage.conditional-content-updates.jsCopiedapp.get('/news', (req, res) => { // Check if the news content is fresh if (req.fresh) { res.status(304).end(); } else { // Logic to fetch and send the latest news const latestNews = fetchLatestNews(); res.send(latestNews); } });
🏆 Best Practices
Set ETag and Last-Modified Headers:
Ensure your routes set appropriate ETag and Last-Modified headers for the resources. Express.js will use these headers to compare with the client's request during conditional GET.
etag.jsCopiedapp.get('/content', (req, res) => { const content = fetchContent(); res.set('ETag', generateETag(content)); res.set('Last-Modified', generateLastModified(content)); res.send(content); });
Combine with Caching Strategies:
Integrate
req.fresh
with caching strategies like client-side caching to further optimize resource delivery.caching-strategies.jsCopiedapp.get('/styles.css', (req, res) => { // Check freshness and respond accordingly if (req.fresh) { res.status(304).end(); } else { // Logic to fetch and send the CSS file const cssContent = fetchCssFile(); res.send(cssContent); } });
🎉 Conclusion
The req.fresh
property in Express.js offers an efficient way to handle conditional GET requests, optimizing the delivery of resources and minimizing unnecessary data transfer. By understanding its usage and integrating it with proper headers and caching strategies, you can enhance the performance of your Express.js applications.
Now, armed with knowledge about req.fresh
, go ahead and implement smart and efficient conditional GET handling in your Express.js projects!
👨💻 Join our Community:
Author
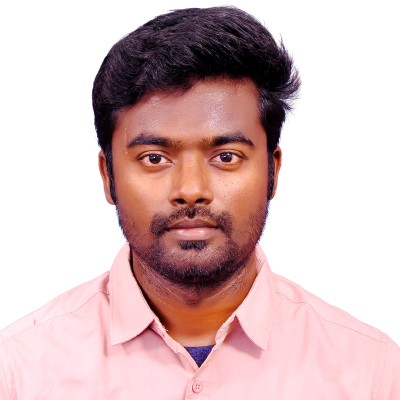
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (Express req.fresh Property), please comment here. I will help you immediately.