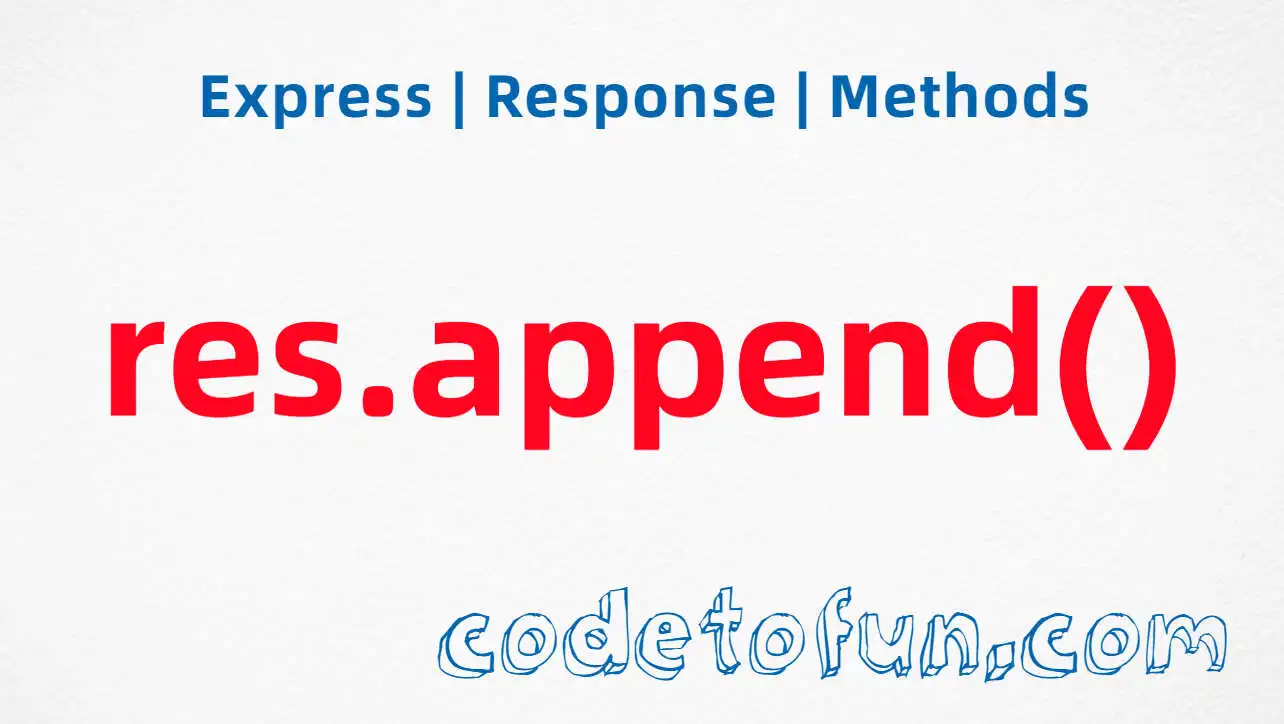
Express.js Basic
- express Intro
- express express()
- express Application
- express Request
Properties
- req.app
- req.baseUrl
- req.body
- req.cookies
- req.fresh
- req.host
- req.hostname
- req.ip
- req.ips
- req.method
- req.originalUrl
- req.params
- req.path
- req.protocol
- req.query
- req.res
- req.route
- req.secure
- req.signedCookies
- req.stale
- req.subdomains
- req.xhr
Methdos
- express Response
- express Router
Express req.accepts() Method
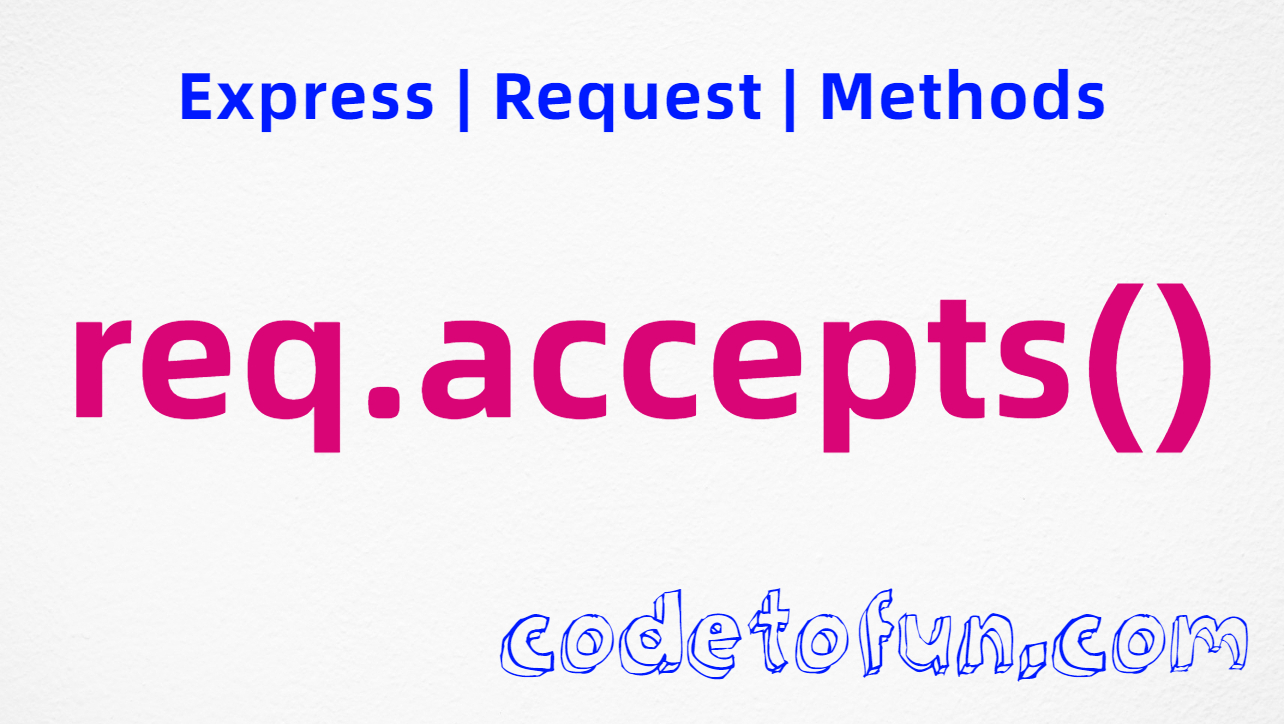
Photo Credit to CodeToFun
🙋 Introduction
In web development, serving content in the appropriate format is crucial for creating a seamless user experience.
Express.js, a popular web application framework for Node.js, provides the req.accepts()
method to help negotiate content types based on the client's preferences.
In this guide, we'll explore the syntax, use cases, and best practices of the req.accepts()
method.
💡 Syntax
The req.accepts()
method in Express has a simple syntax:
req.accepts(types)
- types: An array or string representing the content types that the server is willing to send in response to the client's request.
❓ How req.accepts() Works
The req.accepts()
method is used to determine the most suitable response content type based on the client's preferences. It analyzes the Accept header in the HTTP request, which specifies the media types that the client can process.
app.get('/api/data', (req, res) => {
const acceptedType = req.accepts(['json', 'html']);
if (!acceptedType) {
res.status(406).send('Not Acceptable');
return;
}
if (acceptedType === 'json') {
// Respond with JSON data
res.json({ message: 'Data in JSON format' });
} else {
// Respond with HTML
res.send('<h1>Data in HTML format</h1>');
}
});
In this example, the server checks the client's accepted types for the '/api/data' route and sends the appropriate response.
📚 Use Cases
Content Negotiation:
Use
req.accepts()
for content negotiation, allowing your server to respond with the appropriate content type.example.jsCopiedapp.get('/api/content', (req, res) => { const acceptedType = req.accepts(['json', 'xml']); if (!acceptedType) { res.status(406).send('Not Acceptable'); return; } if (acceptedType === 'json') { // Respond with JSON data res.json({ message: 'Content in JSON format' }); } else { // Respond with XML res.send('<message>Data in XML format</message>'); } });
Error Handling for Unsupported Content Types:
Handle cases where the client submits data in an unsupported format by checking accepted types with
req.accepts()
.example.jsCopiedapp.post('/api/data', (req, res) => { const acceptedType = req.accepts(['json', 'xml']); if (!acceptedType) { res.status(415).send('Unsupported Media Type'); return; } // Process the request based on the accepted type });
🏆 Best Practices
Provide a Default Content Type:
Always provide a default content type to handle cases where the client doesn't explicitly specify its preferences.
example.jsCopiedapp.get('/api/data', (req, res) => { const acceptedType = req.accepts(['json', 'html']) || 'json'; if (acceptedType === 'json') { // Respond with JSON data res.json({ message: 'Data in JSON format' }); } else { // Respond with HTML res.send('<h1>Data in HTML format</h1>'); } });
Use Specific Routes for Different Content Types:
Consider using specific routes for different content types to simplify content negotiation and routing.
example.jsCopiedapp.get('/api/data.json', (req, res) => { // Respond with JSON data res.json({ message: 'Data in JSON format' }); }); app.get('/api/data.html', (req, res) => { // Respond with HTML res.send('<h1>Data in HTML format</h1>'); });
🎉 Conclusion
The req.accepts()
method in Express.js enables content negotiation, allowing your server to respond with the most suitable content type based on the client's preferences. Leveraging this method enhances the flexibility and user experience of your web applications.
Now equipped with the knowledge of req.accepts()
, confidently implement content negotiation in your Express.js projects!
👨💻 Join our Community:
Author
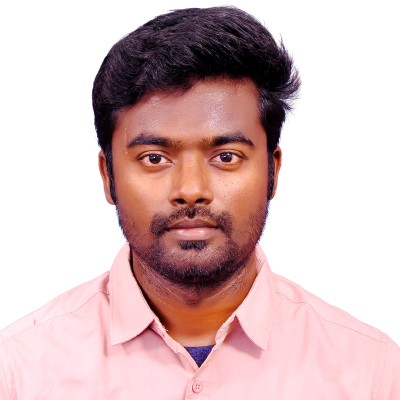
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (Express req.accepts() Method), please comment here. I will help you immediately.