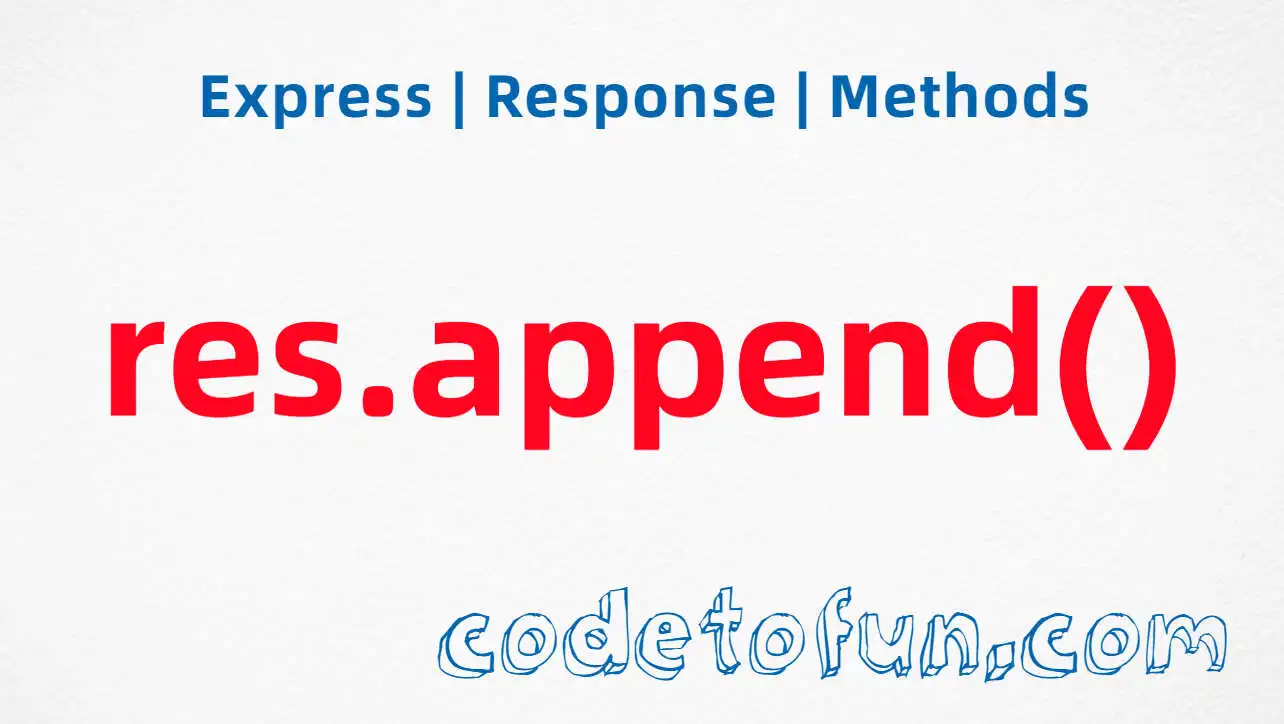
Express express.urlencoded() Method
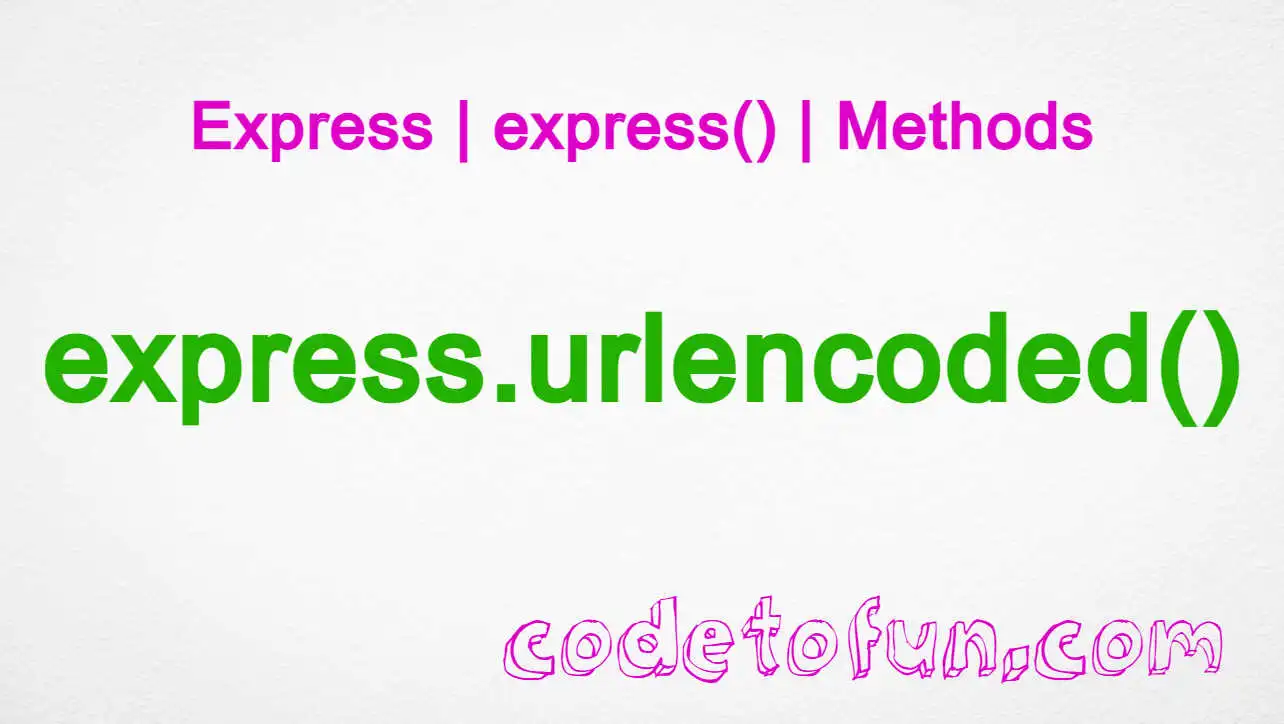
Photo Credit to CodeToFun
🙋 Introduction
Handling form submissions is a common task in web development. Express.js simplifies this process with the express.urlencoded()
method, which is designed to parse URL-encoded form data from incoming requests.
In this guide, we'll explore the syntax, usage, and practical examples of express.urlencoded()
to enhance your understanding of form data processing in Express.js.
💡 Syntax
The syntax for using express.urlencoded()
is straightforward. It is typically used as middleware in an Express application:
const express = require('express');
const app = express();
app.use(express.urlencoded({ extended: true }));
The extended option allows you to choose between parsing the URL-encoded data with the querystring library (when false) or the qs library (when true).
❓ How express.urlencoded() Works
express.urlencoded()
is middleware that parses incoming request bodies containing URL-encoded data. It adds the parsed data to the request object under the body property, making it accessible for further processing in your route handlers.
app.post('/submit-form', (req, res) => {
// Access form data from the request body
const formData = req.body;
// Process the form data as needed
// ...
res.send('Form submission successful');
});
In this example, the express.urlencoded()
middleware allows you to access form data submitted to the /submit-form route through req.body.
📚 Use Cases
Form Submissions:
Use
express.urlencoded()
to handle form submissions, extracting data from the request body for registration or other user-related actions.example.jsCopiedapp.post('/register', (req, res) => { const { username, password, email } = req.body; // Logic to register a new user // ... res.send('User registration successful'); });
Processing Webhooks:
Leverage
express.urlencoded()
to parse data from incoming webhooks and take appropriate actions based on the received information.example.jsCopiedapp.post('/webhook', (req, res) => { const { event, data } = req.body; // Process the webhook data // ... res.send('Webhook processed successfully'); });
🏆 Best Practices
Specify the extended Option:
Be explicit about whether to use the querystring library (extended: false) or the qs library (extended: true). This helps in avoiding ambiguity and ensures compatibility with your specific use case.
example.jsCopiedapp.use(express.urlencoded({ extended: true }));
Handle Errors Gracefully:
Implement error handling for cases where the submitted form data cannot be parsed correctly. This prevents server crashes and provides a better user experience.
example.jsCopiedapp.post('/submit-form', (req, res) => { try { const formData = req.body; // Process the form data // ... res.send('Form submission successful'); } catch (error) { console.error('Error processing form data:', error); res.status(500).send('Internal Server Error'); } });
🎉 Conclusion
The express.urlencoded()
method in Express.js streamlines the handling of URL-encoded form data, making it easy to work with submissions from HTML forms or other sources. By understanding its syntax, usage, and best practices, you can enhance your Express.js applications and efficiently process form data.
Now, equipped with knowledge about express.urlencoded()
, go ahead and optimize your form-handling workflows in Express.js!
👨💻 Join our Community:
Author
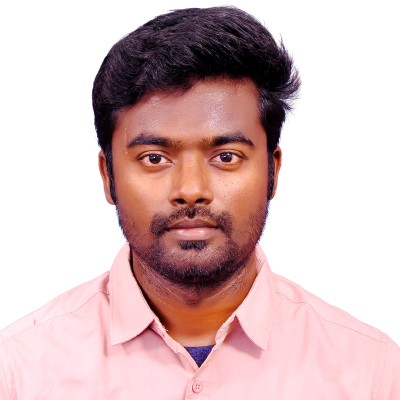
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (Express express.urlencoded() Method), please comment here. I will help you immediately.