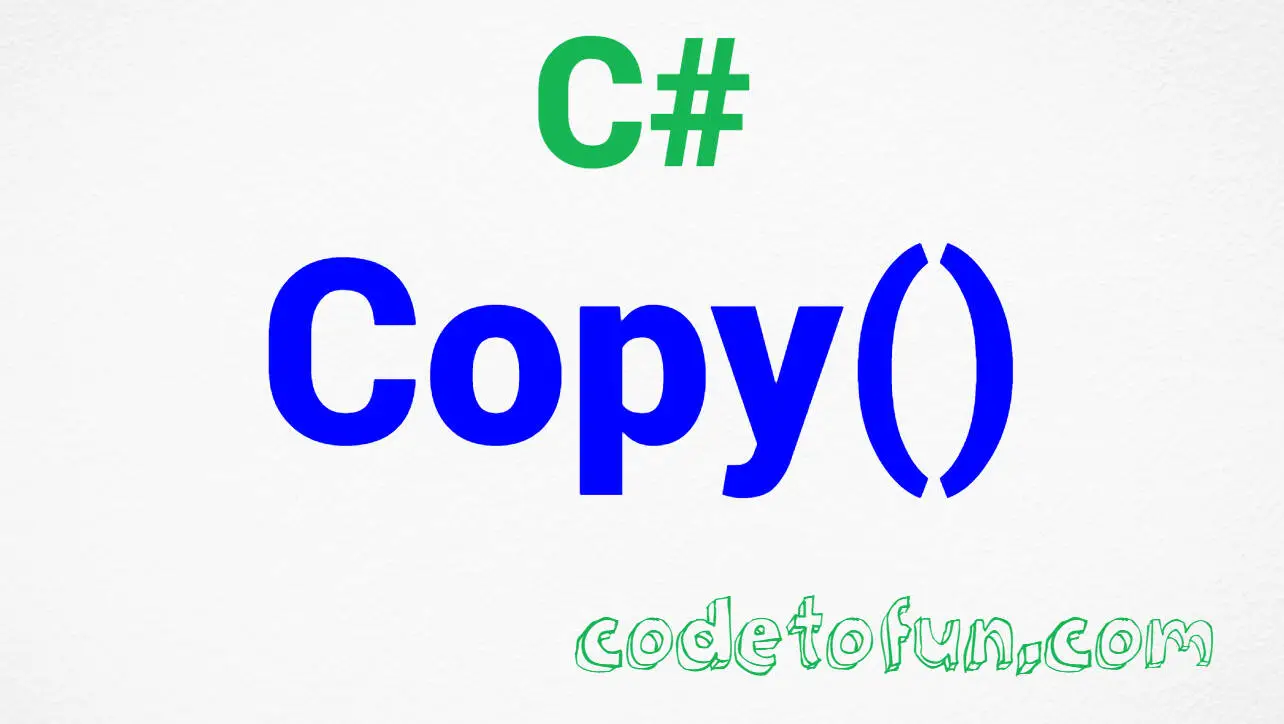
C# Basic
C# Interview Programs
- C# Interview Programs
- C# Abundant Number
- C# Amicable Number
- C# Armstrong Number
- C# Average of N Numbers
- C# Automorphic Number
- C# Biggest of three numbers
- C# Binary to Decimal
- C# Common Divisors
- C# Composite Number
- C# Condense a Number
- C# Cube Number
- C# Decimal to Binary
- C# Decimal to Octal
- C# Disarium Number
- C# Even Number
- C# Evil Number
- C# Factorial of a Number
- C# Fibonacci Series
- C# GCD
- C# Happy Number
- C# Harshad Number
- C# LCM
- C# Leap Year
- C# Magic Number
- C# Matrix Addition
- C# Matrix Division
- C# Matrix Multiplication
- C# Matrix Subtraction
- C# Matrix Transpose
- C# Maximum Value of an Array
- C# Minimum Value of an Array
- C# Multiplication Table
- C# Natural Number
- C# Number Combination
- C# Odd Number
- C# Palindrome Number
- C# Pascalβs Triangle
- C# Perfect Number
- C# Perfect Square
- C# Power of 2
- C# Power of 3
- C# Pronic Number
- C# Prime Factor
- C# Prime Number
- C# Smith Number
- C# Strong Number
- C# Sum of Array
- C# Sum of Digits
- C# Swap Two Numbers
- C# Triangular Number
C# Program to Check Disarium Number
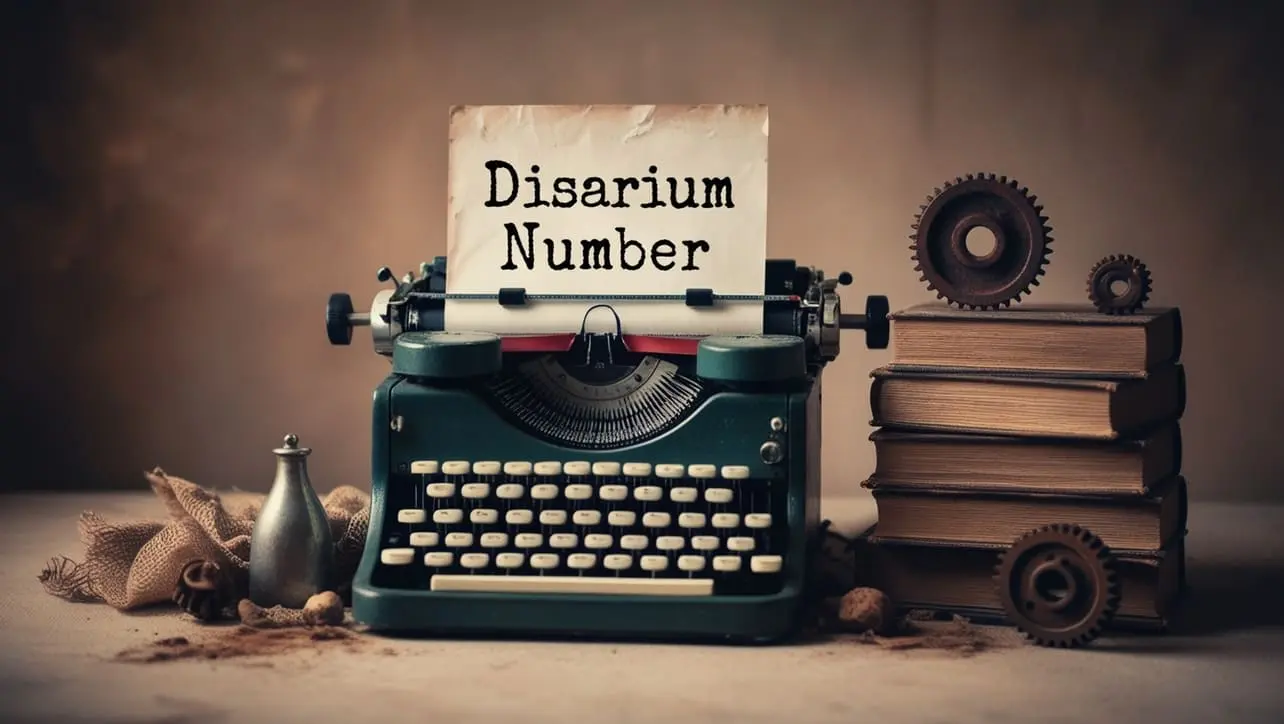
Photo Credit to CodeToFun
π Introduction
In the realm of programming, exploring mathematical patterns is a fascinating endeavor. One such numeric pattern is the Disarium number.
A Disarium number is a number defined by the sum of its digits each raised to the power of its respective position.
In this tutorial, we'll delve into a C# program designed to check whether a given number is a Disarium number.
π Example
Let's explore the C# code that checks whether a given number is a Disarium number.
using System;
class Program {
// Function to count the number of digits in a given number
static int CountDigits(int number) {
int count = 0;
while (number != 0) {
count++;
number /= 10;
}
return count;
}
// Function to check if a number is a Disarium number
static bool IsDisarium(int number) {
int originalNumber = number;
int digitCount = CountDigits(number);
int sum = 0;
while (number != 0) {
int digit = number % 10;
sum += (int) Math.Pow(digit, digitCount);
digitCount--;
number /= 10;
}
return (sum == originalNumber);
}
// Driver program
static void Main() {
// Replace this value with your desired number
int inputNumber = 89;
// Call the function to check if the number is Disarium
if (IsDisarium(inputNumber)) {
Console.WriteLine($"{inputNumber} is a Disarium number.");
} else {
Console.WriteLine($"{inputNumber} is not a Disarium number.");
}
}
}
π» Testing the Program
To test the program with different numbers, replace the value of inputNumber in the Main method.
89 is a Disarium number.
Compile and run the program to check if the number is a Disarium number.
π§ How the Program Works
- The program defines a class Program containing static methods CountDigits to count the number of digits and IsDisarium to check if a number is a Disarium number.
- The Main method tests the IsDisarium method with a sample number (replace it with your desired number).
π Between the Given Range
Let's delve into the C# code that checks for Disarium numbers in the specified range.
using System;
class Program {
// Function to check if a number is a Disarium number
static bool IsDisarium(int number) {
int originalNumber = number;
int digitCount = (int) Math.Floor(Math.Log10(number) + 1);
int sum = 0;
while (number > 0) {
int digit = number % 10;
sum += (int) Math.Pow(digit, digitCount);
number /= 10;
digitCount--;
}
return sum == originalNumber;
}
// Driver program
static void Main() {
Console.Write("Disarium numbers in the range 1 to 100: \n");
for (int i = 1; i <= 100; i++) {
if (IsDisarium(i)) {
Console.Write(i + " ");
}
}
Console.WriteLine();
}
}
π» Testing the Program
Disarium numbers in the range 1 to 100: 1 2 3 4 5 6 7 8 9 89
Run the program to see the Disarium numbers in the specified range.
π§ How the Program Works
- The program defines a function IsDisarium that checks if a given number is a Disarium number.
- Inside the function, it calculates the number of digits in the given number.
- It then iterates through each digit, raising it to the power of its position, and calculates the sum.
- The main function tests the Disarium condition for numbers in the range from 1 to 100 and prints the results.
π§ Understanding the Concept of Disarium Numbers
Before we dive into the code, let's grasp the concept of Disarium numbers.
A Disarium number is a number such that the sum of its digits, each raised to the power of its position, equals the number itself.
For example, the number 89 is a Disarium number because 8^1 + 9^2 equals 89.
π Conclusion
Understanding and implementing programs to identify numeric patterns, such as Disarium numbers, is a valuable skill in the world of programming.
The provided C# program offers a practical example of checking whether a given number follows the Disarium pattern.
Feel free to use and modify this code for your specific use cases. Happy coding!
π¨βπ» Join our Community:
Author
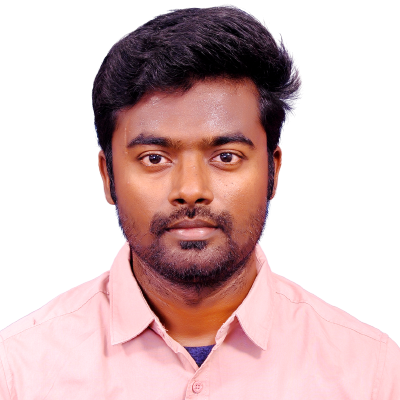
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (C# Program to Check Disarium Number), please comment here. I will help you immediately.