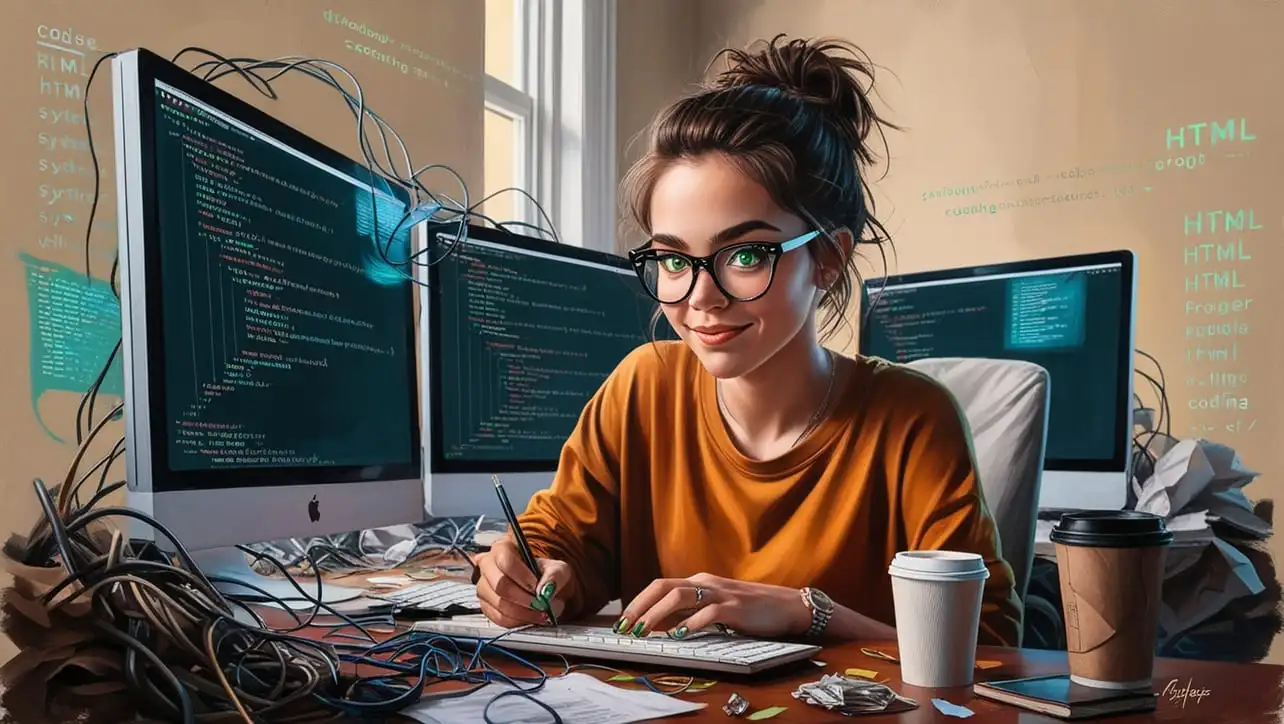
HTML Basic
HTML Entity
- HTML Entity
- HTML Alphabet Entity
- HTML Arrow Entity
- HTML Currency Entity
- HTML Math Entity
- HTML Number Entity
- HTML Punctuation Entity
- HTML Symbol Entity
HTML IndexedDB
HTML Reference
HTML Canvas
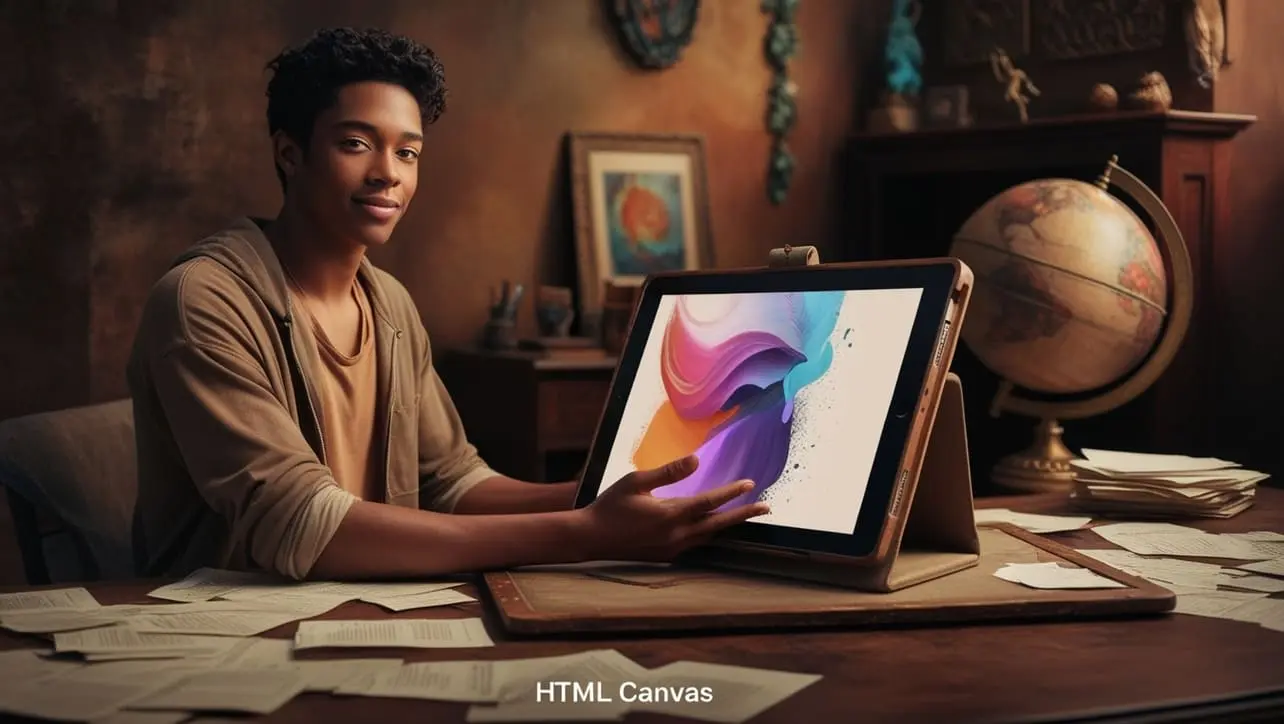
Photo Credit to CodeToFun
Introduction
The HTML <canvas>
element is a powerful tool for drawing graphics via JavaScript. It provides a drawable region on your webpage where you can create 2D shapes, render text, draw images, or even complex animations.
Canvas is widely used for games, visualizations, and interactive web applications.
What Is HTML Canvas?
The <canvas>
element is part of HTML5, used to render 2D graphics on the fly via scripting. Unlike static images, you can manipulate the contents of a canvas dynamically using JavaScript. It is widely used in applications that require real-time rendering, like games and data visualizations.
Setting Up the Canvas
To start using a canvas, you simply define it in HTML and access its context in JavaScript to start drawing.
<canvas id="myCanvas" width="400" height="300"></canvas>
To begin drawing, get the context of the canvas:
const canvas = document.getElementById('myCanvas');
const ctx = canvas.getContext('2d');
Canvas Coordinates
The canvas uses a coordinate system where the top-left corner is (0, 0). You can draw shapes and lines by specifying coordinates based on this grid.
Drawing Shapes
Canvas supports a range of drawing methods for shapes. For example, you can draw rectangles, circles, and lines using built-in methods:
Rectangles:
The
fillRect
andstrokeRect
methods draw filled and outlined rectangles.JavascriptCopiedctx.fillStyle = '#FF0000'; ctx.fillRect(50, 50, 150, 100);
Circles:
Use the
arc
method to draw circles or arcs.JavascriptCopiedctx.beginPath(); ctx.arc(150, 150, 50, 0, Math.PI * 2, true); ctx.fillStyle = 'blue'; ctx.fill();
Working with Colors and Styles
You can style your drawings using fillStyle
for filling colors and strokeStyle
for border colors.
ctx.fillStyle = 'green';
ctx.fillRect(100, 100, 200, 100);
ctx.strokeStyle = 'black';
ctx.lineWidth = 5;
ctx.strokeRect(100, 100, 200, 100);
Drawing Images
You can also draw images onto a canvas using the drawImage
method.
const img = new Image();
img.src = 'image.png';
img.onload = function() {
ctx.drawImage(img, 50, 50, 200, 150);
};
Text on Canvas
Text can be drawn using the fillText
and strokeText
methods, allowing for both filled and outlined text.
ctx.font = '30px Arial';
ctx.fillText('Hello, Canvas!', 50, 50);
Canvas Transformations
You can apply transformations like scaling, rotating, and translating (moving) the canvas context.
Scaling:
Change the size of objects drawn on the canvas.
JavascriptCopiedctx.scale(2, 2); ctx.fillRect(50, 50, 100, 50);
Rotating:
Rotate objects around the origin of the canvas.
JavascriptCopiedctx.rotate((Math.PI / 180) * 45); // Rotate 45 degrees ctx.fillRect(100, 100, 100, 50);
Translating:
Move the canvas origin to a new point.
JavascriptCopiedctx.translate(100, 100); ctx.fillRect(0, 0, 100, 50);
Best Practices
- Layering: Since Canvas is a stateful API, keep track of your transformations and reset the canvas when necessary to avoid unwanted effects.
- Performance: Redraw only the parts of the canvas that need updating to optimize performance.
- State Management: Use
save()
andrestore()
to save and reset the canvas state between complex drawings.
Example
Hereβs a complete example that demonstrates drawing shapes, text, and an image on the canvas:
<!DOCTYPE html>
<html>
<head>
<title>Canvas Example</title>
</head>
<body>
<canvas id="myCanvas" width="500" height="400"></canvas>
<script>
const canvas = document.getElementById('myCanvas');
const ctx = canvas.getContext('2d');
// Draw a rectangle
ctx.fillStyle = 'lightblue';
ctx.fillRect(50, 50, 200, 100);
// Draw text
ctx.font = '20px Arial';
ctx.fillStyle = 'black';
ctx.fillText('Hello Canvas', 100, 180);
// Draw an image
const img = new Image();
img.src = 'https://www.example.com/image.png';
img.onload = function() {
ctx.drawImage(img, 50, 200, 150, 100);
};
</script>
</body>
</html>
Conclusion
The HTML Canvas is a versatile and powerful tool for rendering dynamic graphics in web applications. With its wide range of methods for drawing shapes, text, and images, it enables developers to create rich, interactive experiences on the web. Mastering the Canvas API can significantly enhance your web development projects.
π¨βπ» Join our Community:
Author
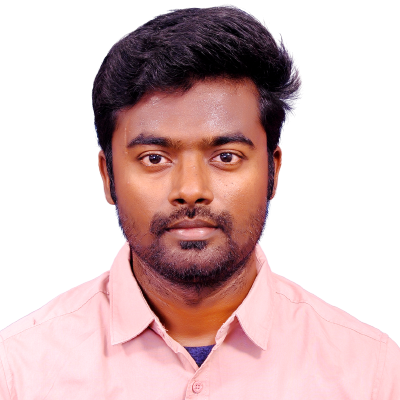
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee