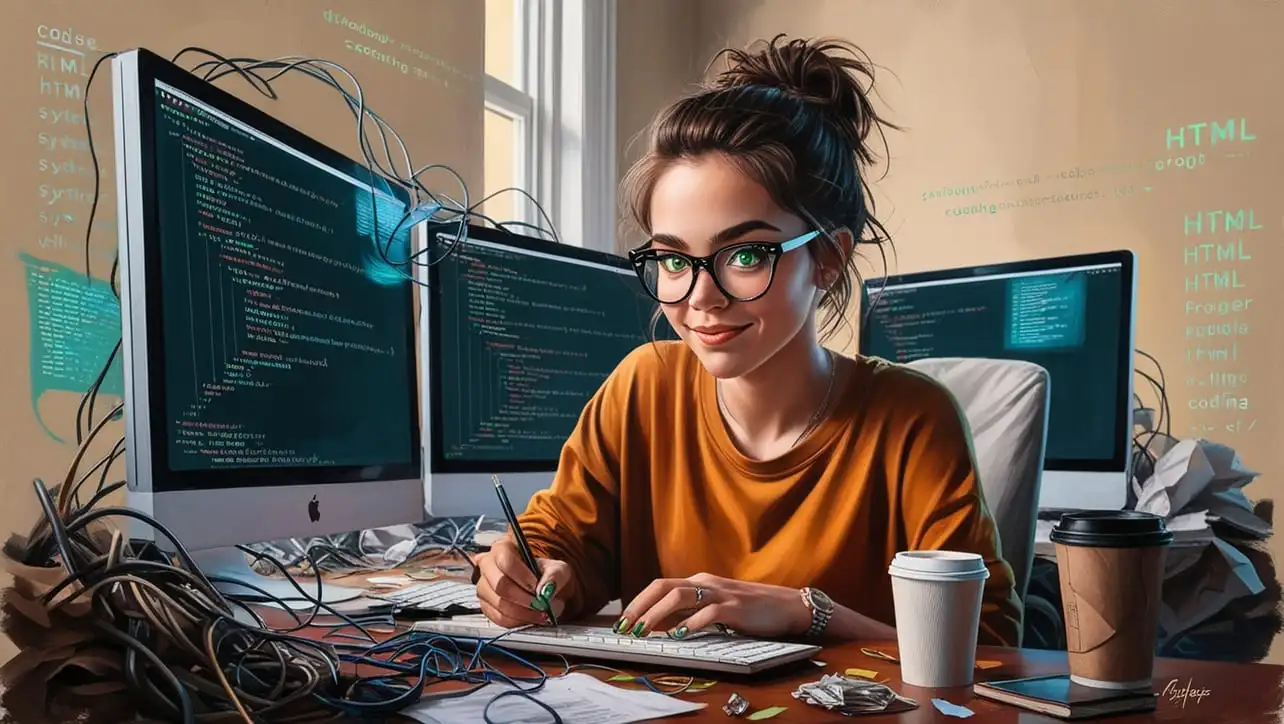
HTML Basic
HTML Entity
- HTML Entity
- HTML Alphabet Entity
- HTML Arrow Entity
- HTML Currency Entity
- HTML Math Entity
- HTML Number Entity
- HTML Punctuation Entity
- HTML Symbol Entity
HTML IndexedDB
HTML Reference
HTML IndexedDB Retrieve
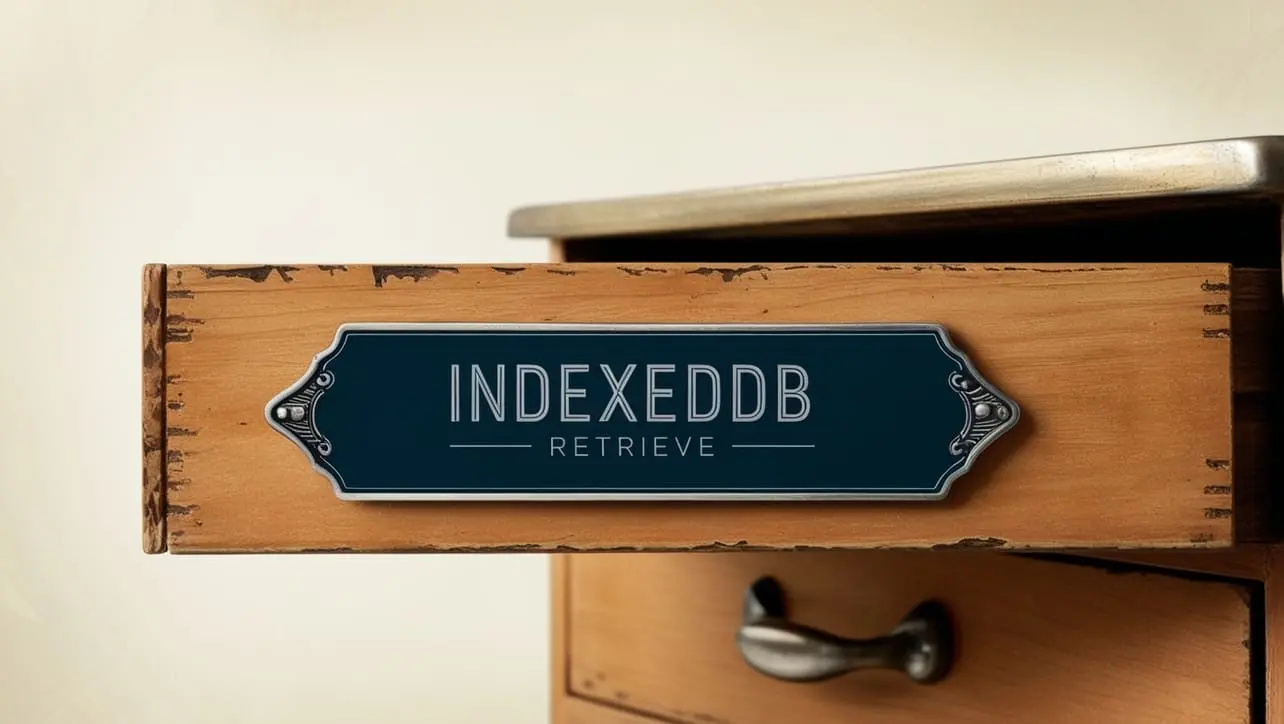
Photo Credit to CodeToFun
π Introduction
IndexedDB is a low-level API for storing significant amounts of structured data in the browser. It allows developers to create rich, offline-capable web applications.
Retrieving data from IndexedDB is a key operation that enables applications to access and utilize stored data effectively.
β What is IndexedDB?
IndexedDB is a client-side database that enables you to store large amounts of structured data, including files and blobs. It is asynchronous, meaning that it does not block the main thread, making it ideal for performing complex operations without affecting the performance of the web page.
βοΈ Setting Up IndexedDB
Before you can retrieve data, you need to set up and open a connection to an IndexedDB database. Hereβs a quick setup:
let db;
const request = indexedDB.open('myDatabase', 1);
request.onupgradeneeded = function(event) {
db = event.target.result;
const objectStore = db.createObjectStore('myStore', { keyPath: 'id' });
objectStore.createIndex('name', 'name', { unique: false });
};
request.onsuccess = function(event) {
db = event.target.result;
};
request.onerror = function(event) {
console.error('Database error:', event.target.errorCode);
};
π Retrieving Data from IndexedDB
To retrieve data from an IndexedDB object store, you typically use the get method. Hereβs an example of how to retrieve data by its key:
const transaction = db.transaction(['myStore']);
const objectStore = transaction.objectStore('myStore');
const request = objectStore.get(1); // Retrieving the record with key 1
request.onsuccess = function(event) {
const data = event.target.result;
console.log('Data retrieved:', data);
};
request.onerror = function(event) {
console.error('Error retrieving data:', event.target.errorCode);
};
π±οΈ Using Cursors for Data Retrieval
Cursors are used when you want to retrieve multiple records or iterate over all records in an object store or index. Hereβs how you can use a cursor to retrieve all records:
const transaction = db.transaction(['myStore'], 'readonly');
const objectStore = transaction.objectStore('myStore');
const request = objectStore.openCursor();
request.onsuccess = function(event) {
const cursor = event.target.result;
if (cursor) {
console.log('Key:', cursor.key, 'Data:', cursor.value);
cursor.continue(); // Move to the next record
} else {
console.log('No more entries');
}
};
request.onerror = function(event) {
console.error('Cursor error:', event.target.errorCode);
};
π€ Handling Errors
When retrieving data from IndexedDB, you may encounter errors such as database access issues or data not found. Always handle errors gracefully to ensure your application can respond appropriately:
request.onerror = function(event) {
console.error('Error retrieving data:', event.target.errorCode);
};
π Example Usage
Hereβs a full example that shows how to retrieve data from an IndexedDB database:
<!DOCTYPE html>
<html>
<head>
<title>IndexedDB Retrieve Example</title>
</head>
<body>
<h1>IndexedDB Retrieve Example</h1>
<button id="retrieve">Retrieve Data</button>
<p id="result"></p>
<script>
let db;
const request = indexedDB.open('myDatabase', 1);
request.onupgradeneeded = function(event) {
db = event.target.result;
const objectStore = db.createObjectStore('myStore', { keyPath: 'id' });
objectStore.createIndex('name', 'name', { unique: false });
};
request.onsuccess = function(event) {
db = event.target.result;
};
request.onerror = function(event) {
console.error('Database error:', event.target.errorCode);
};
document.getElementById('retrieve').addEventListener('click', () => {
const transaction = db.transaction(['myStore'], 'readonly');
const objectStore = transaction.objectStore('myStore');
const request = objectStore.get(1);
request.onsuccess = function(event) {
const data = event.target.result;
document.getElementById('result').textContent = 'Retrieved data: ' + JSON.stringify(data);
};
request.onerror = function(event) {
console.error('Error retrieving data:', event.target.errorCode);
};
});
</script>
</body>
</html>
π Conclusion
Retrieving data from IndexedDB is a crucial operation for any web application that relies on client-side data storage. By understanding how to set up IndexedDB, retrieve data, and handle errors, you can create robust, offline-capable web applications.
π¨βπ» Join our Community:
Author
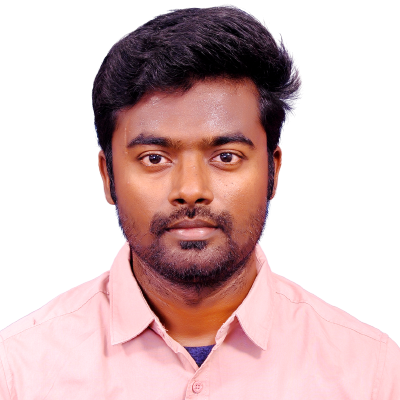
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (HTML IndexedDB Retrieve), please comment here. I will help you immediately.