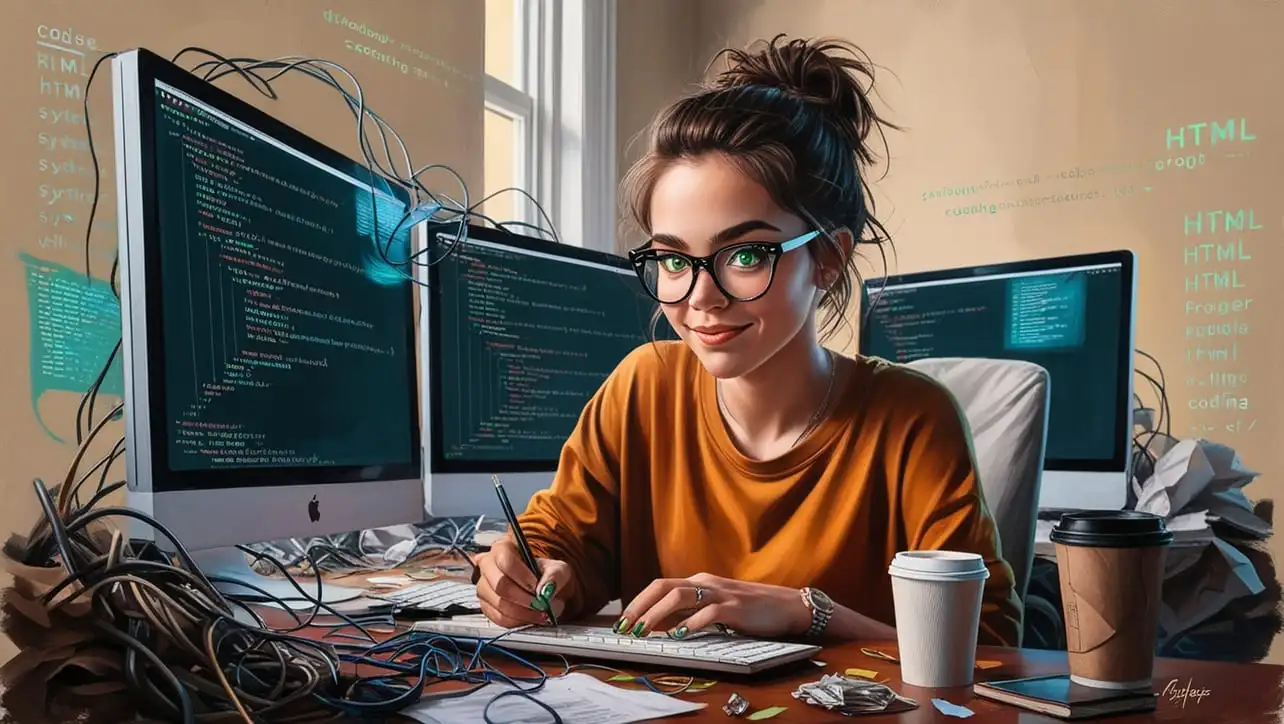
HTML Basic
HTML Entity
- HTML Entity
- HTML Alphabet Entity
- HTML Arrow Entity
- HTML Currency Entity
- HTML Math Entity
- HTML Number Entity
- HTML Punctuation Entity
- HTML Symbol Entity
HTML IndexedDB
HTML Reference
HTML Web Workers API
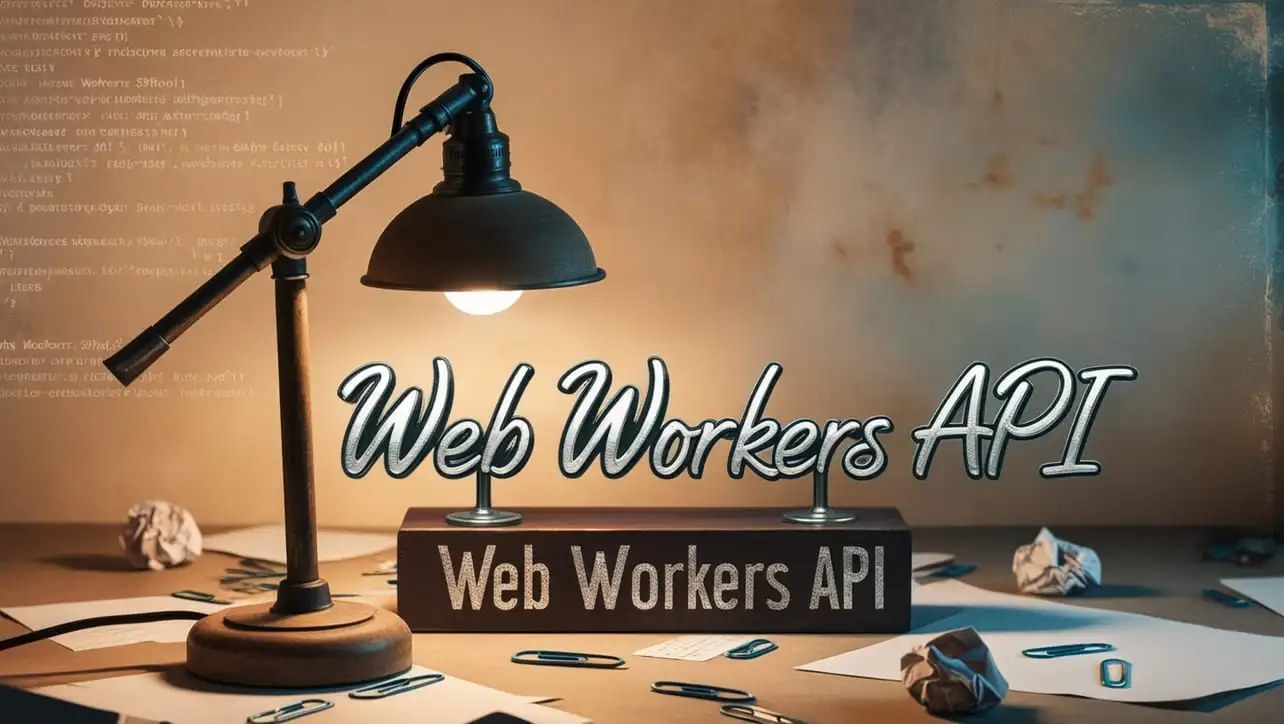
Photo Credit to CodeToFun
π Introduction
Web Workers are a powerful feature in modern web development that allow scripts to run in the background, separate from the main execution thread of a web application. This helps in performing resource-intensive tasks without blocking the user interface, leading to a smoother and more responsive experience.
β What Are Web Workers?
Web Workers are a way to run JavaScript scripts in background threads. These workers operate independently of the main UI thread and can be used to perform tasks such as computations, data processing, or other operations without affecting the user interface.
π€ How Do Web Workers Work?
Web Workers run in their own global context and do not have access to the DOM or the window object. They communicate with the main thread using a messaging system. This separation ensures that long-running tasks do not interfere with the responsiveness of the web page.
π± Creating a Web Worker
To create a Web Worker, you need to instantiate a Worker object and pass it the URL of the JavaScript file that will run in the worker thread. Here's a basic example:
// main.js
const worker = new Worker('worker.js');
In worker.js, you would have the code that the worker will execute:
// worker.js
self.onmessage = function(event) {
const result = performTask(event.data);
postMessage(result);
};
function performTask(data) {
// Perform computation or processing here
return data * 2; // Example
}
π Communicating with Web Workers
Communication between the main thread and the worker thread is done using the postMessage and onmessage methods. Hereβs an example:
// main.js
worker.postMessage(5); // Send data to the worker
worker.onmessage = function(event) {
console.log('Received from worker:', event.data);
};
π€ Handling Errors
Web Workers can throw errors which can be caught using the onerror event handler:
// main.js
worker.onerror = function(error) {
console.error('Worker error:', error.message);
};
β οΈ Common Pitfalls
- DOM Access: Web Workers cannot access the DOM. If you need to manipulate the DOM, youβll need to send the data back to the main thread.
- Global Variables: Web Workers have their own global context. They do not share global variables with the main thread or other workers.
- Browser Compatibility: While most modern browsers support Web Workers, you should check for compatibility and provide fallbacks if necessary.
π Example Usage
Hereβs a complete example showing a Web Worker that calculates the square of a number:
<!DOCTYPE html>
<html>
<head>
<title>Web Worker Example</title>
</head>
<body>
<h1>Web Worker Example</h1>
<button id="start">Start Worker</button>
<p id="result"></p>
<script>
// main.js
const worker = new Worker('worker.js');
document.getElementById('start').addEventListener('click', () => {
worker.postMessage(10); // Send number to worker
});
worker.onmessage = function(event) {
document.getElementById('result').textContent = 'Result: ' + event.data;
};
</script>
</body>
</html>
// worker.js
self.onmessage = function(event) {
const result = event.data * event.data;
postMessage(result);
};
π Conclusion
Web Workers are a great tool for improving the performance of web applications by offloading resource-intensive tasks from the main thread. Understanding how to create and use Web Workers effectively can significantly enhance the responsiveness of your web applications.
π¨βπ» Join our Community:
Author
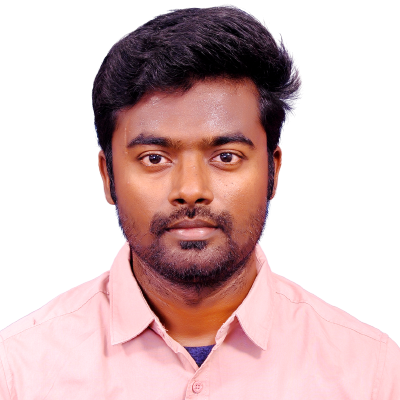
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (HTML Web Workers API), please comment here. I will help you immediately.