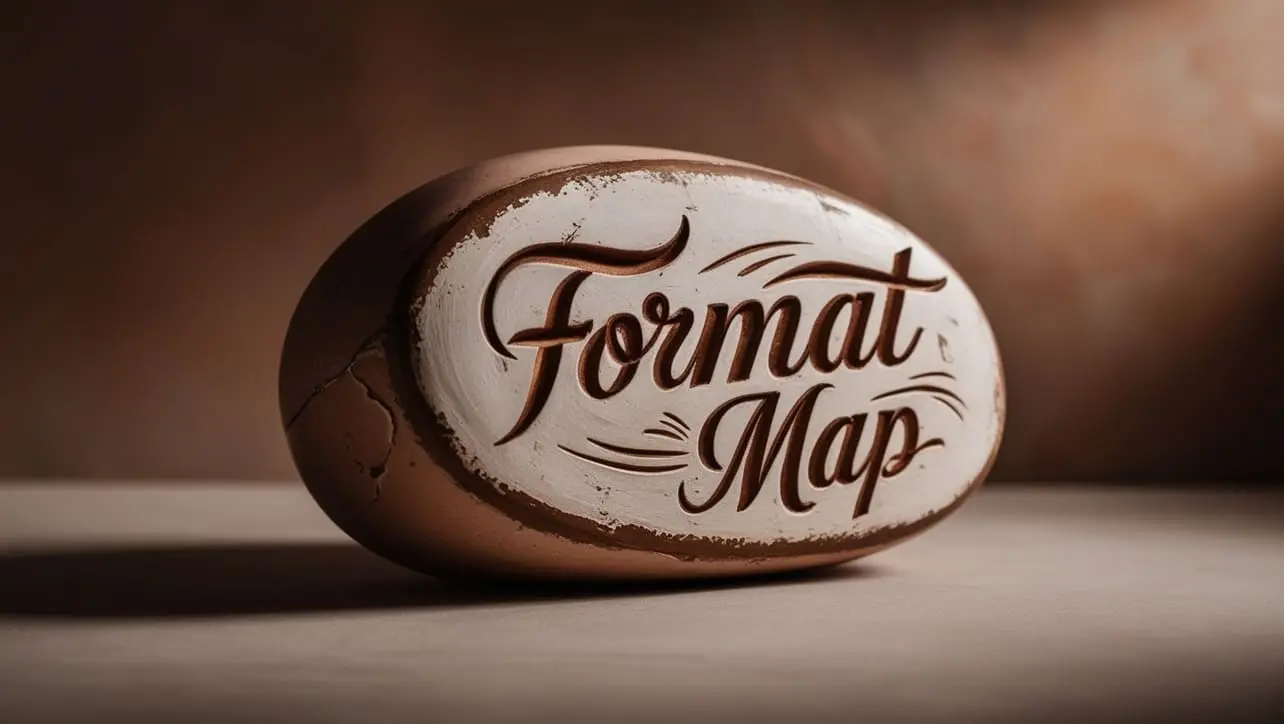
Python Topics
- Python Intro
- Python String Methods
- Python Interview Programs
- Abundant Number
- Amicable Number
- Armstrong Number
- Average of N Numbers
- Automorphic Number
- Biggest of three numbers
- Binary to Decimal
- Common Divisors
- Composite Number
- Condense a Number
- Cube Number
- Decimal to Binary
- Decimal to Octal
- Disarium Number
- Even Number
- Evil Number
- Factorial of a Number
- Fibonacci Series
- GCD
- Happy Number
- Harshad Number
- LCM
- Leap Year
- Magic Number
- Matrix Addition
- Matrix Division
- Matrix Multiplication
- Matrix Subtraction
- Matrix Transpose
- Maximum Value of an Array
- Minimum Value of an Array
- Multiplication Table
- Natural Number
- Number Combination
- Odd Number
- Palindrome Number
- Pascalβs Triangle
- Perfect Number
- Perfect Square
- Power of 2
- Power of 3
- Pronic Number
- Prime Factor
- Prime Number
- Smith Number
- Strong Number
- Sum of Array
- Sum of Digits
- Swap Two Numbers
- Triangular Number
- Python Star Pattern
- Python Number Pattern
- Python Alphabet Pattern
Python Program to Swap Two Numbers
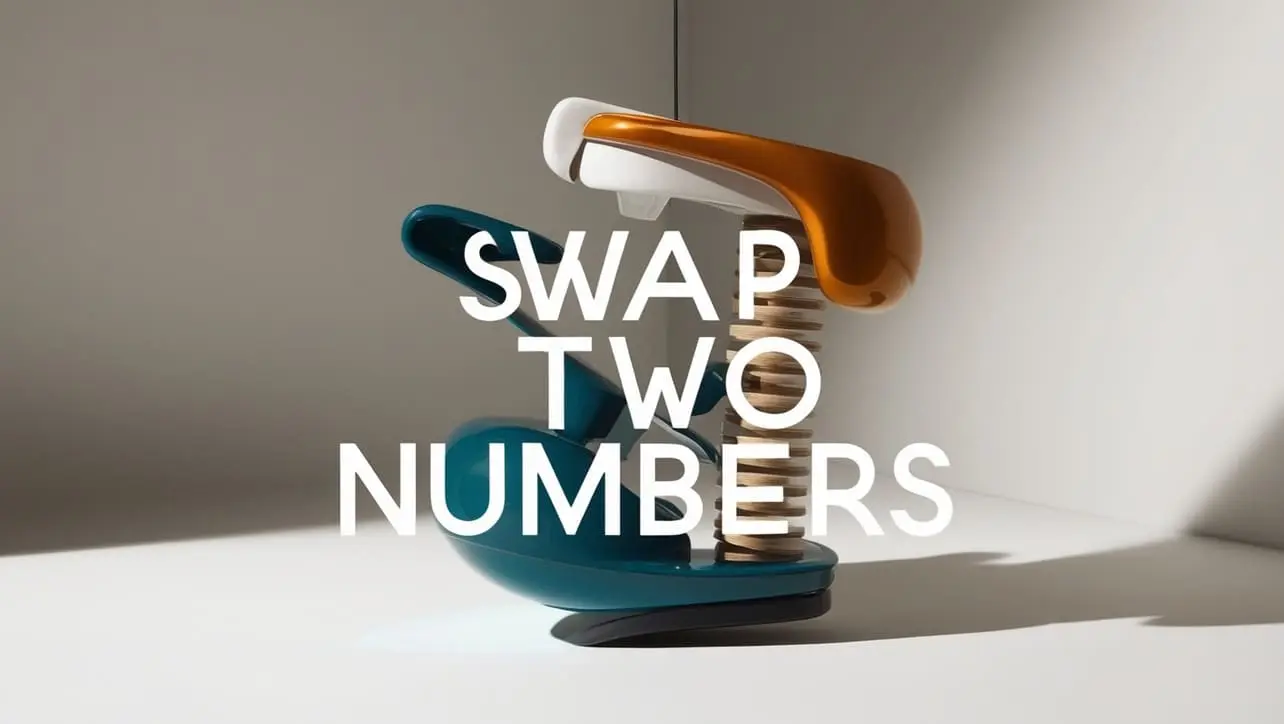
Photo Credit to CodeToFun
Introduction
In the world of programming, performing basic operations on numbers is a fundamental task. Swapping two numbers is one such operation that involves exchanging the values of two variables. This operation is commonly used in various algorithms and programs.
In this tutorial, we will explore a simple Python program designed to swap the values of two numbers.
Example
Let's delve into the Python code that accomplishes this task.
# Function to swap two numbers
def swap_numbers(a, b):
# Assign the value of 'a' to a temporary variable
temp = a
# Assign the value of 'b' to 'a'
a = b
# Assign the original value of 'a' (stored in 'temp') to 'b'
b = temp
return a, b
# Driver program
# Replace these values with the numbers you want to swap
num1 = 5
num2 = 10
print(f"Before swapping: num1 = {num1}, num2 = {num2}")
# Call the function to swap the numbers
num1, num2 = swap_numbers(num1, num2)
print(f"After swapping: num1 = {num1}, num2 = {num2}")
Testing the Program
To test the program with different numbers, modify the values of num1 and num2 in the main program.
Before swapping: num1 = 5, num2 = 10 After swapping: num1 = 10, num2 = 5
Run the script to see the result of swapping the two numbers.
How the Program Works
- The program defines a function swap_numbers that takes two parameters, a and b, and swaps their values using a temporary variable.
- Inside the main program, replace the values of num1 and num2 with the numbers you want to swap.
- The program prints the values of num1 and num2 before and after swapping.
Understanding the Concept of Swapping Two Numbers
Swapping two numbers involves exchanging their values. In the provided program, a temporary variable is used to hold the value of one variable while the values of the two variables are exchanged.
Optimizing the Program
While the provided program is a straightforward way to swap two numbers, consider exploring and implementing alternative approaches or optimizations.
Feel free to incorporate and modify this code as needed for your specific use case. Happy coding!.
Join our Community:
Author
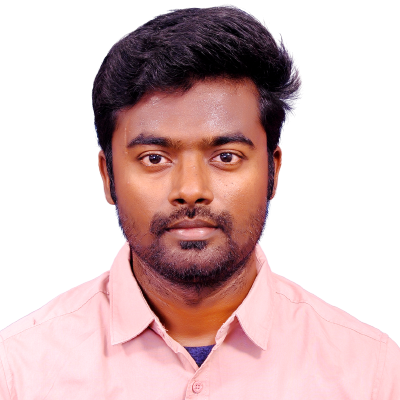
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in Python
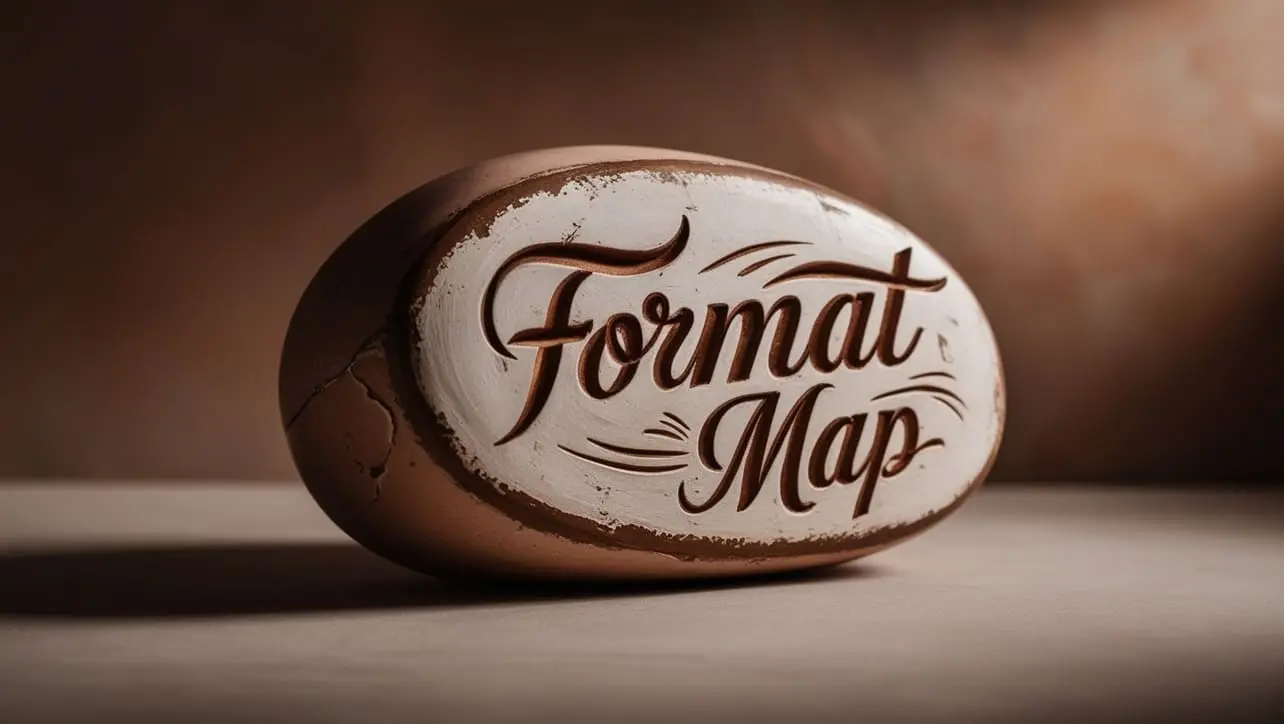
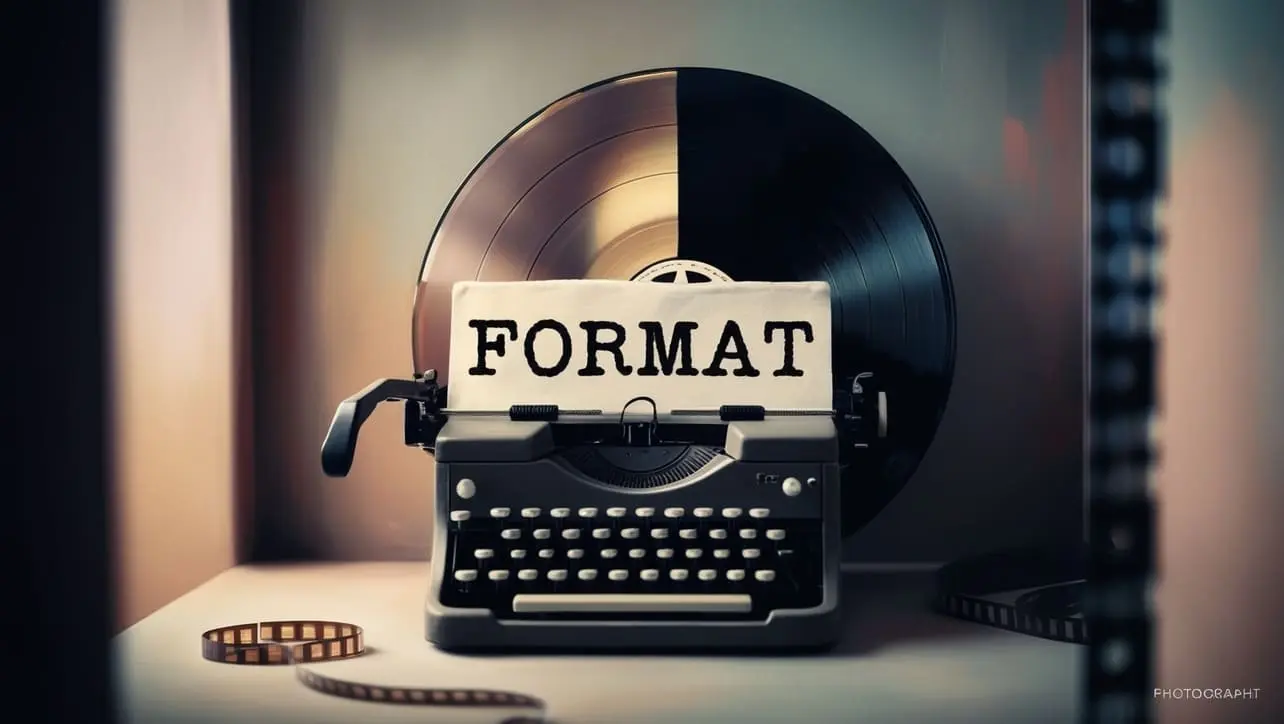
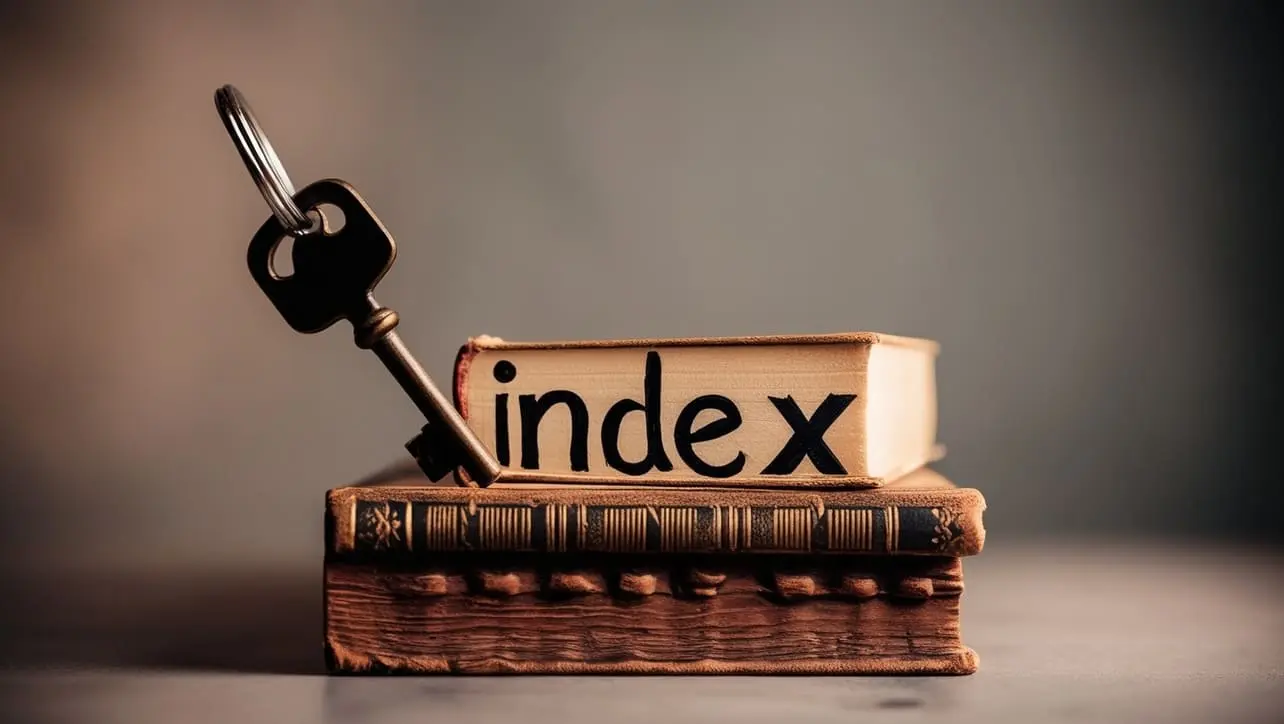
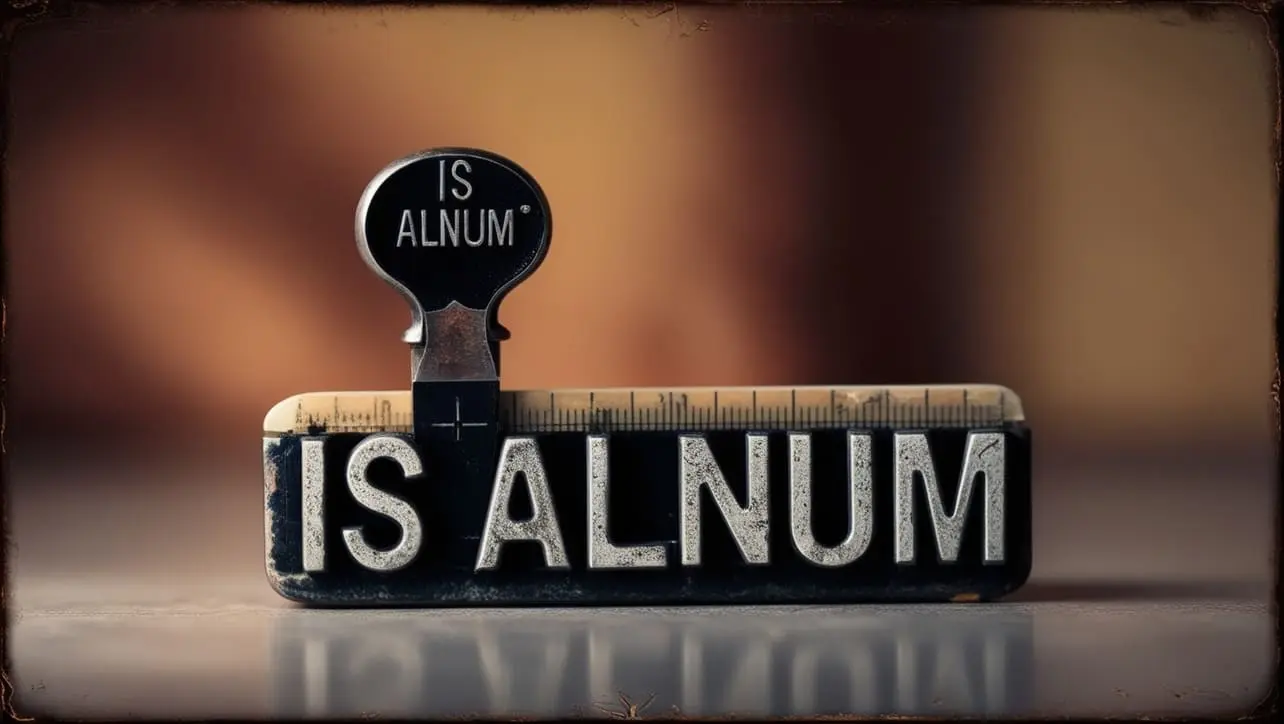
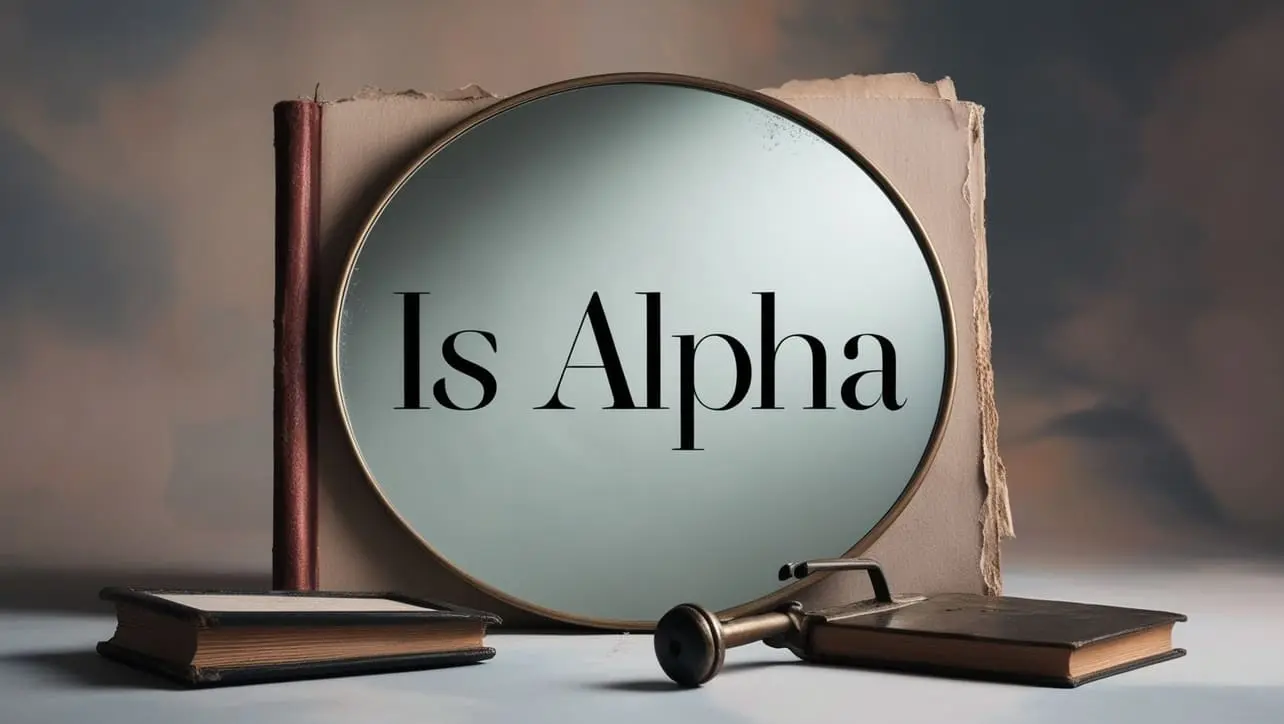
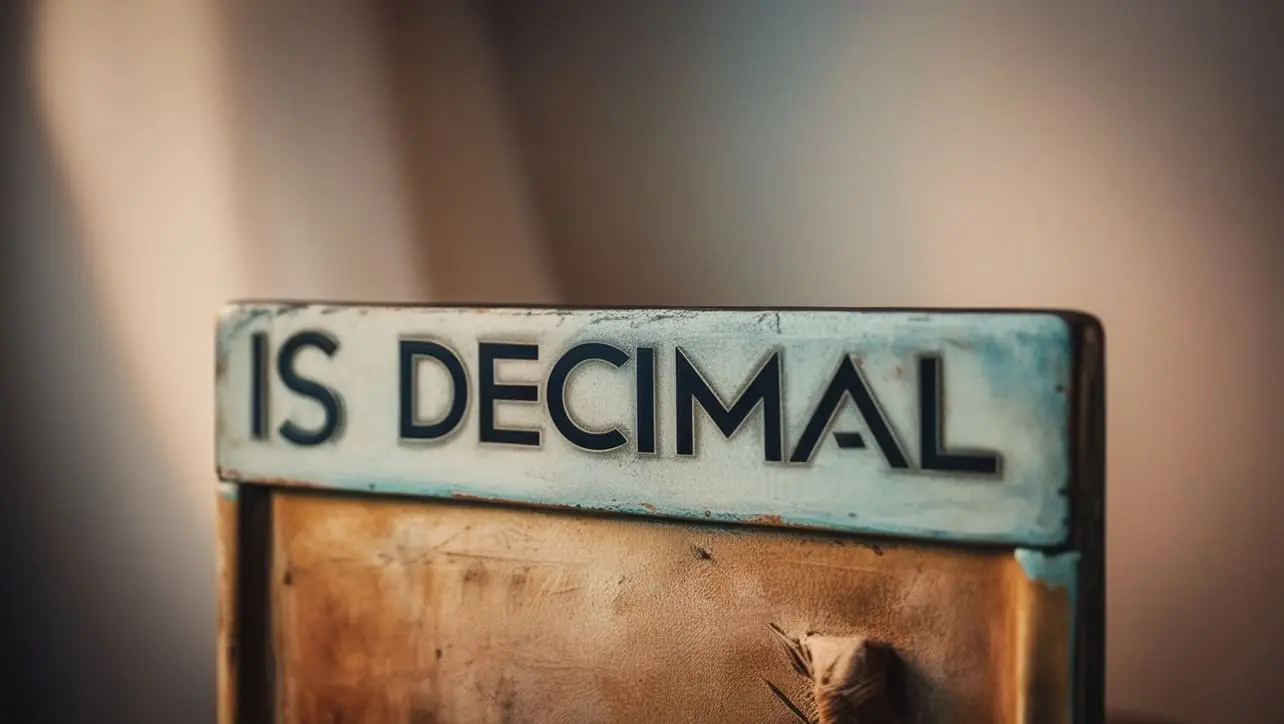
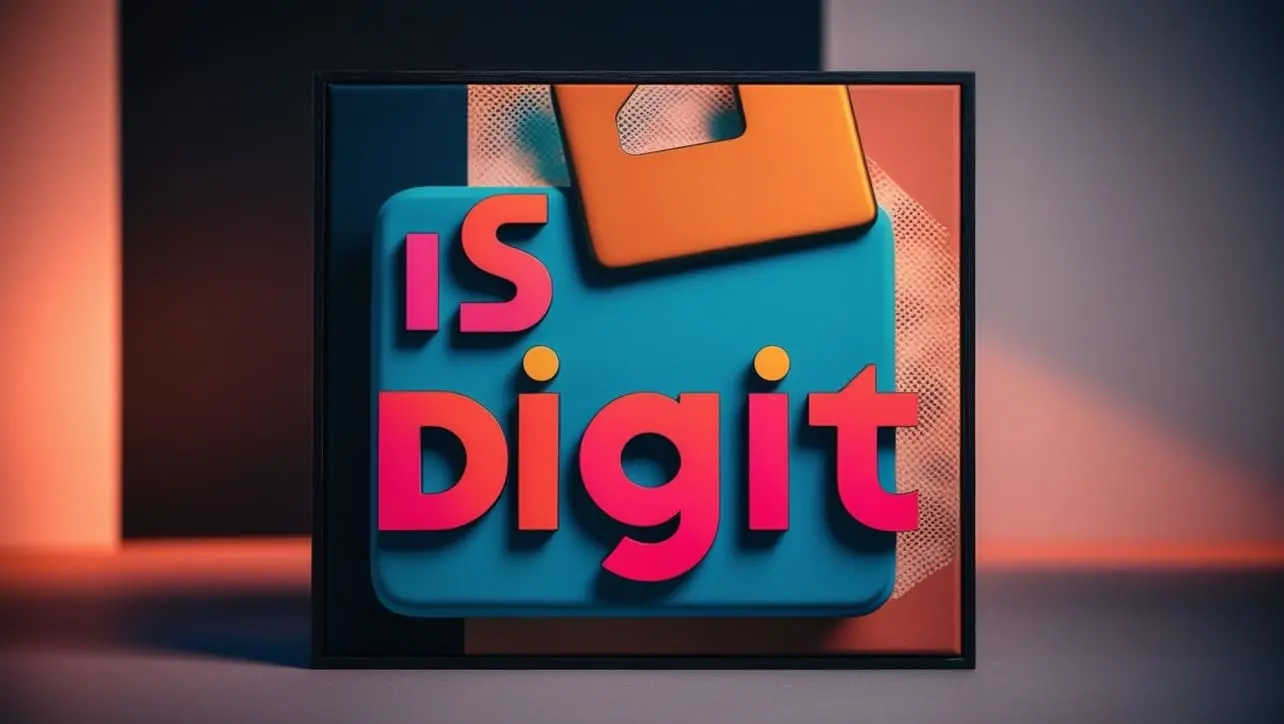
If you have any doubts regarding this article (Python Program to Swap Two Numbers), please comment here. I will help you immediately.