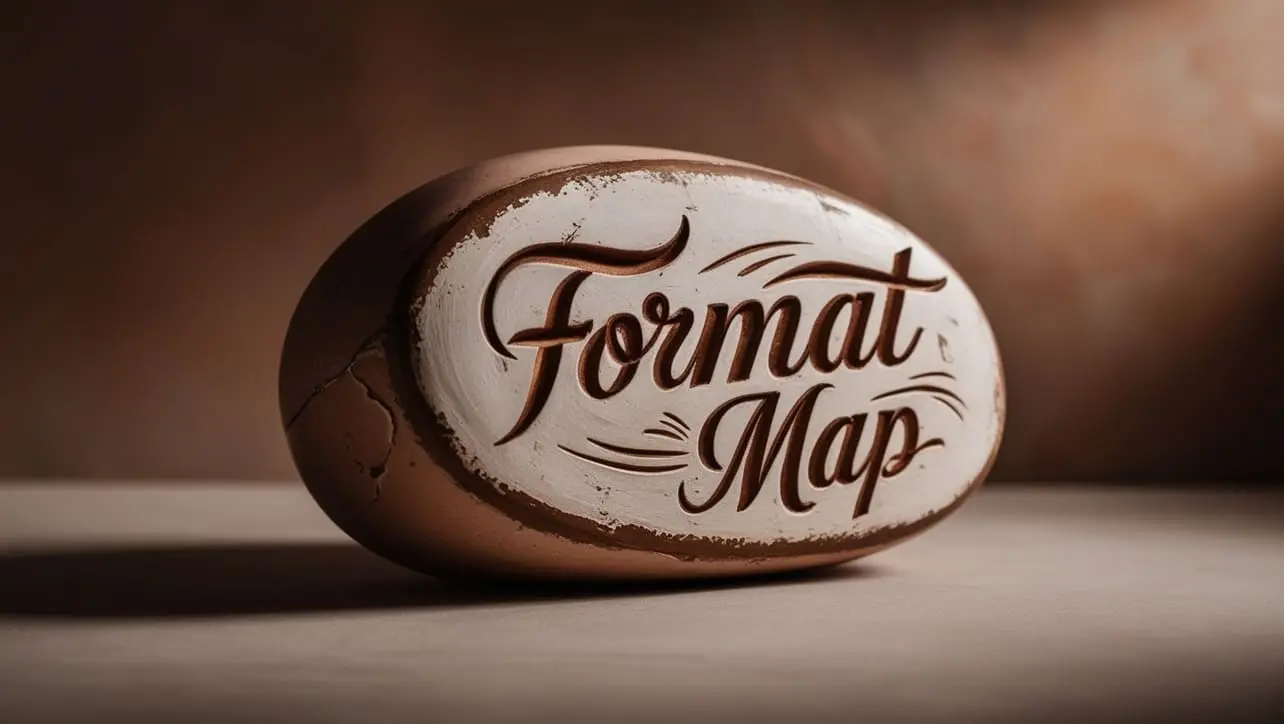
Python string isdecimal() Method
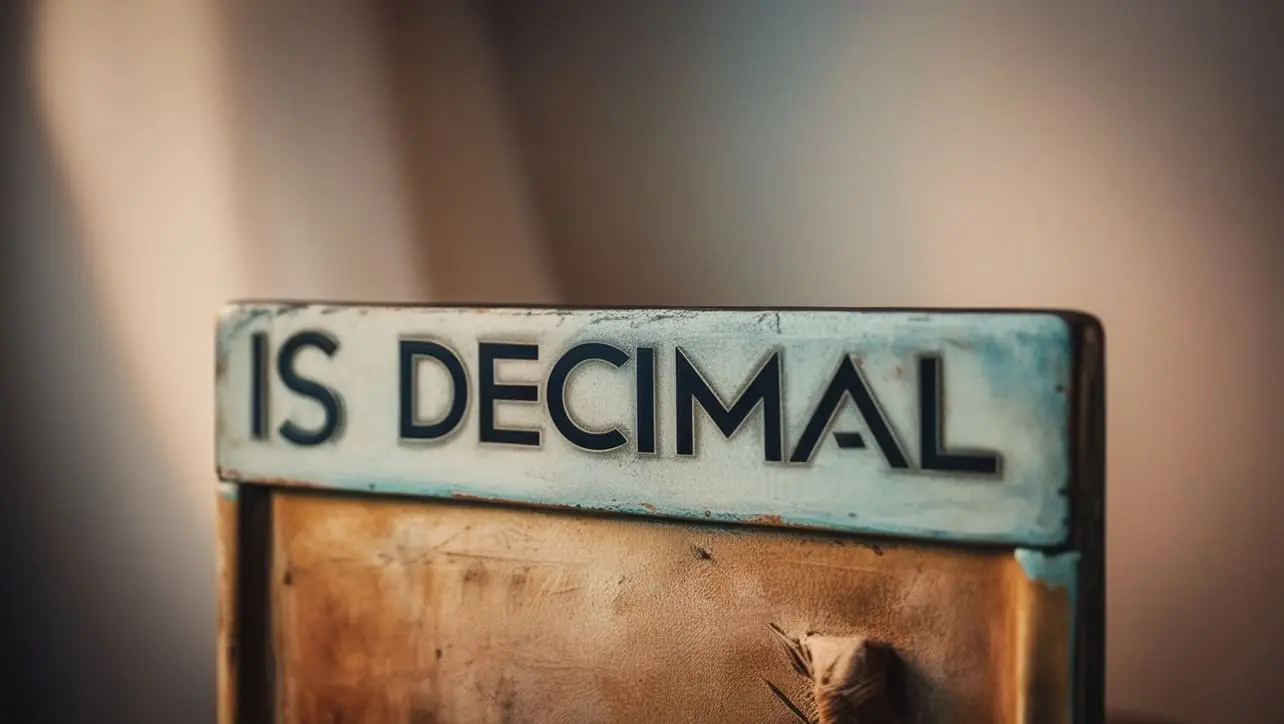
Photo Credit to CodeToFun
Introduction
In Python, string manipulation is a fundamental aspect of programming.
The isdecimal()
method is a built-in string method that allows you to check if a string contains only decimal characters.
In this tutorial, we'll explore the usage and functionality of the isdecimal()
method in Python.
Syntax
The syntax for the isdecimal()
method is as follows:
string.isdecimal()
string: The string to be checked for decimal characters.
Example 1
Let's dive into some examples to illustrate how the isdecimal()
method works.
num_str = "12345"
result1 = num_str.isdecimal()
print("Example 1:", result1)
Testing the Program
Example 1: True
How the Program Works
In Example 1, the isdecimal()
method checks if the string "12345" contains only decimal characters, and the result is True.
Example 2
Let's dive into another examples to illustrate how the isdecimal()
method works.
text_str = "Hello123"
result2 = text_str.isdecimal()
print("Example 2:", result2)
Testing the Program
Example 2: False
How the Program Works
In Example 2, the string "Hello123" contains non-decimal characters, so the result of isdecimal()
is False.
Return Value
The isdecimal()
method returns a boolean value:
- True: if all characters in the string are decimal.
- False: if the string contains at least one non-decimal character.
Common Use Cases
The isdecimal()
method is useful when you need to validate user input or verify that a string represents a decimal number before performing mathematical operations.
Notes
- The
isdecimal()
method only considers characters that are considered decimal digits in Unicode. This includes digits from various scripts, not just the ASCII digits 0-9. - The method returns False for strings containing digits with special meanings, such as superscript digits.
Optimization
The isdecimal()
method is optimized for efficiency. However, keep in mind that it checks the entire string, so for very long strings, consider its performance impact.
Conclusion
The isdecimal()
method in Python is a valuable tool for checking whether a string consists of only decimal characters. It provides a quick and straightforward way to validate and process numeric input.
Feel free to experiment with different strings to explore the behavior of the isdecimal()
method further. Happy coding!
Join our Community:
Author
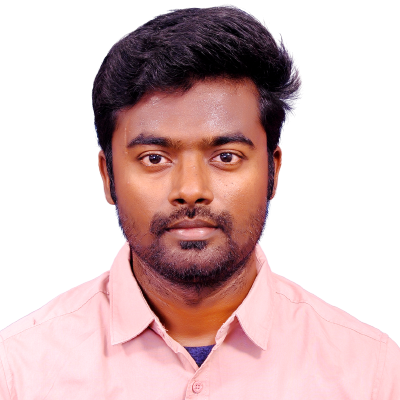
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in Python
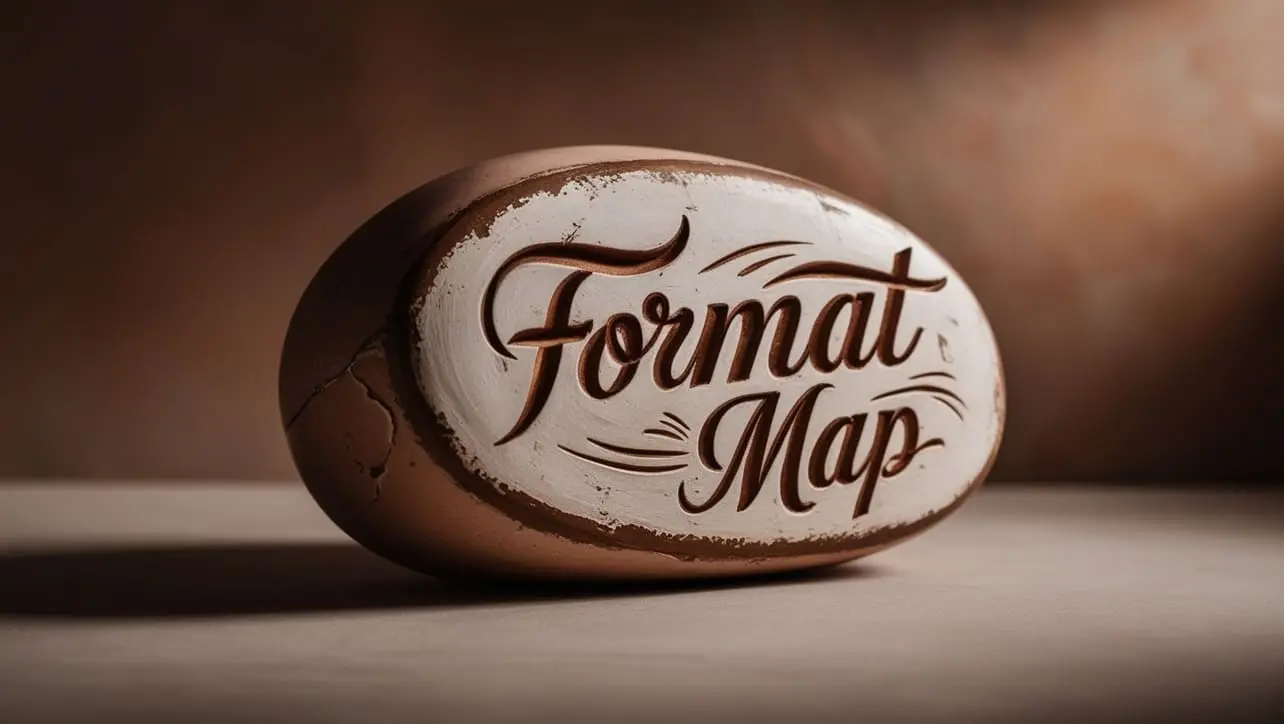
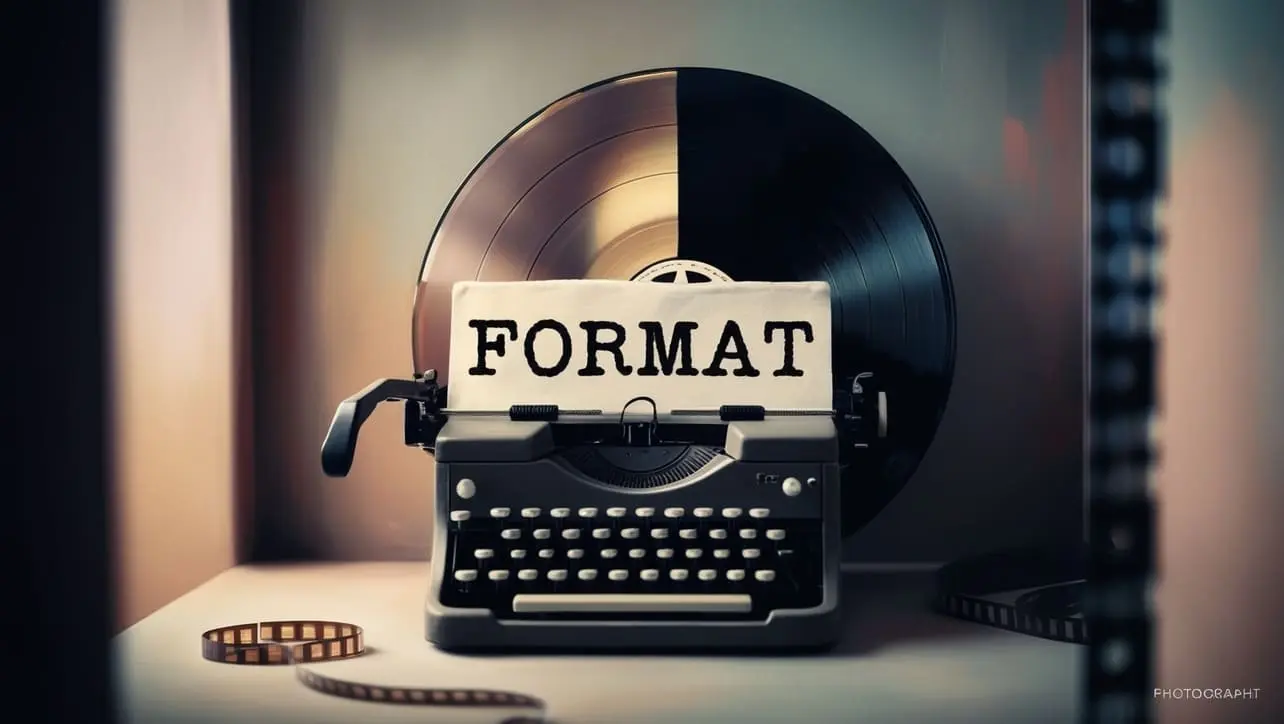
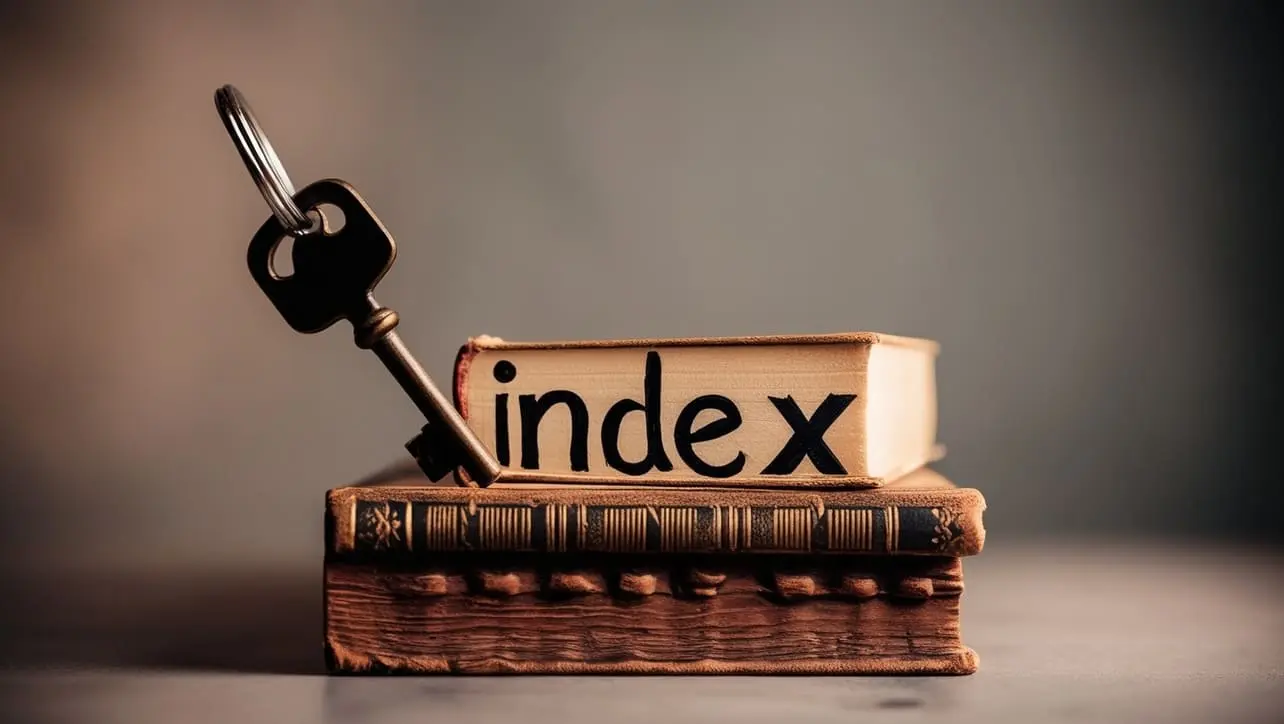
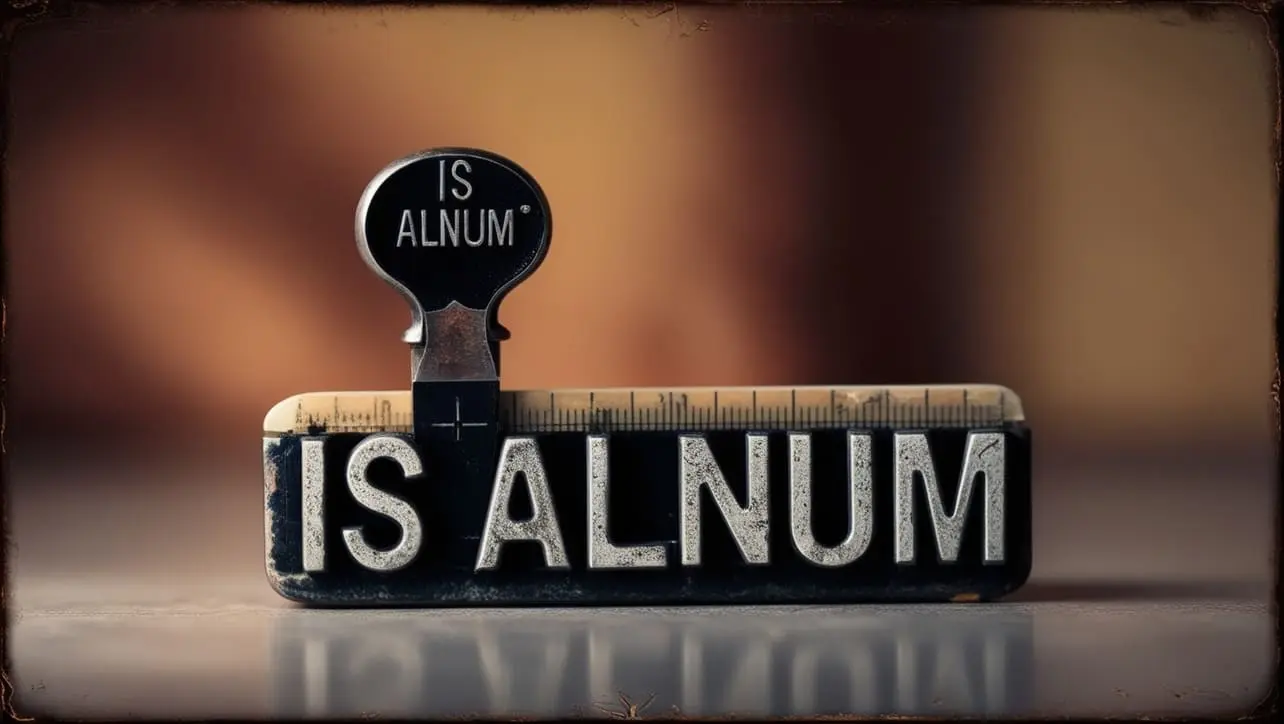
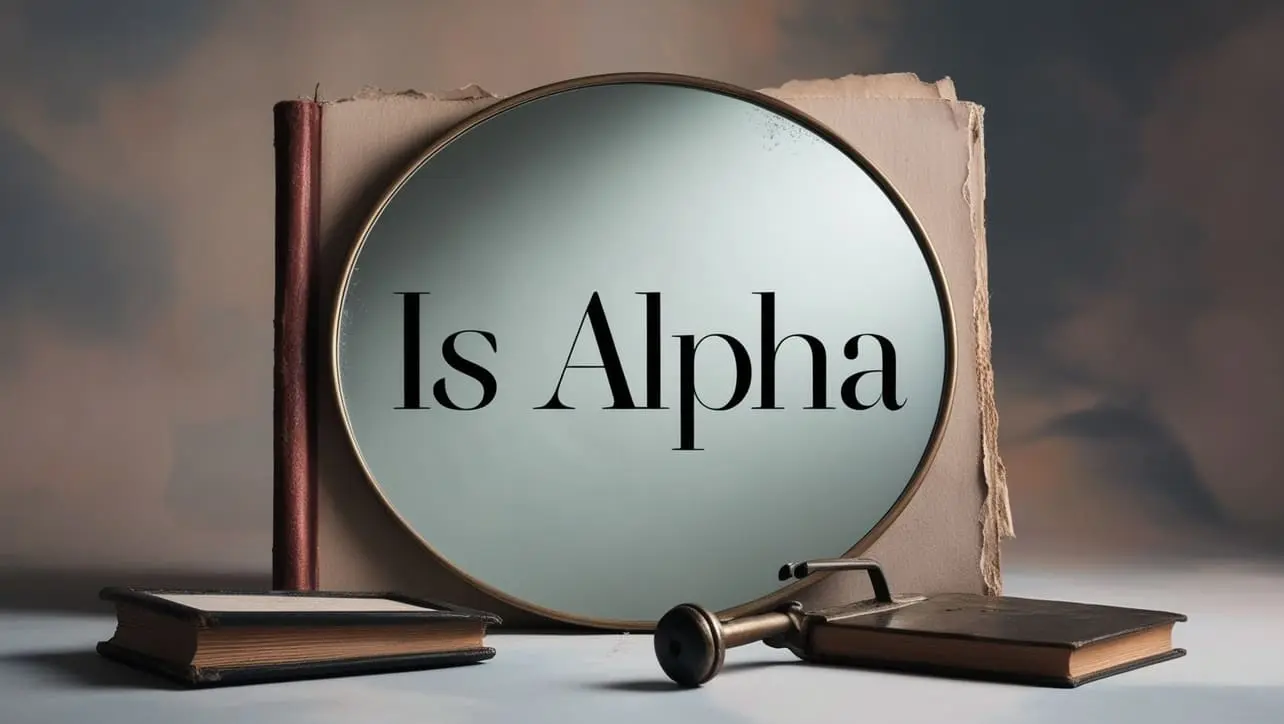
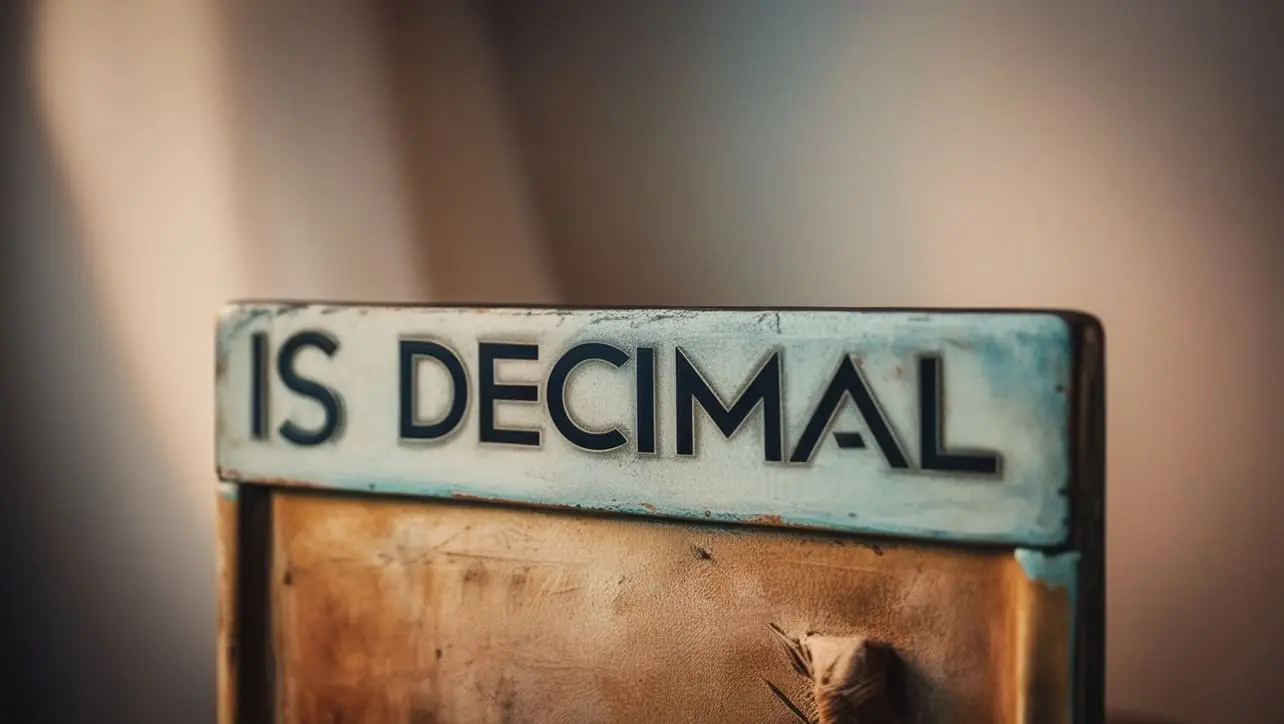
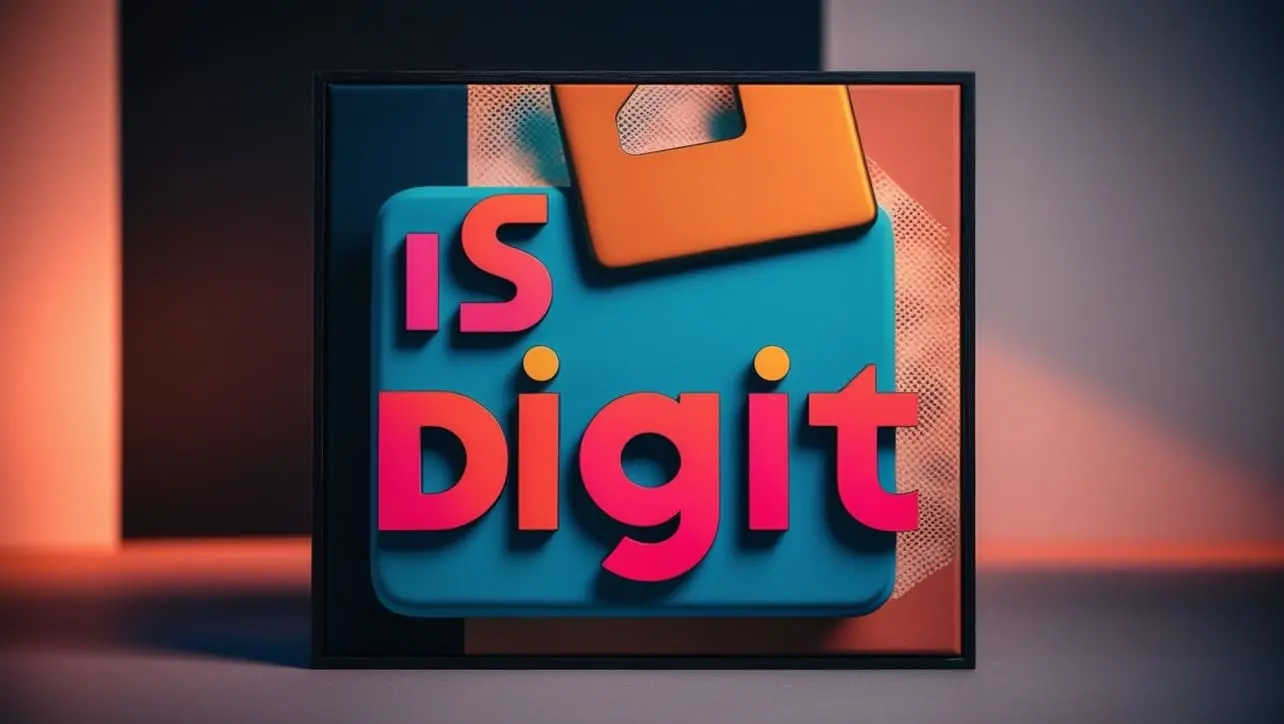
If you have any doubts regarding this article (Python string isdecimal() Method), please comment here. I will help you immediately.