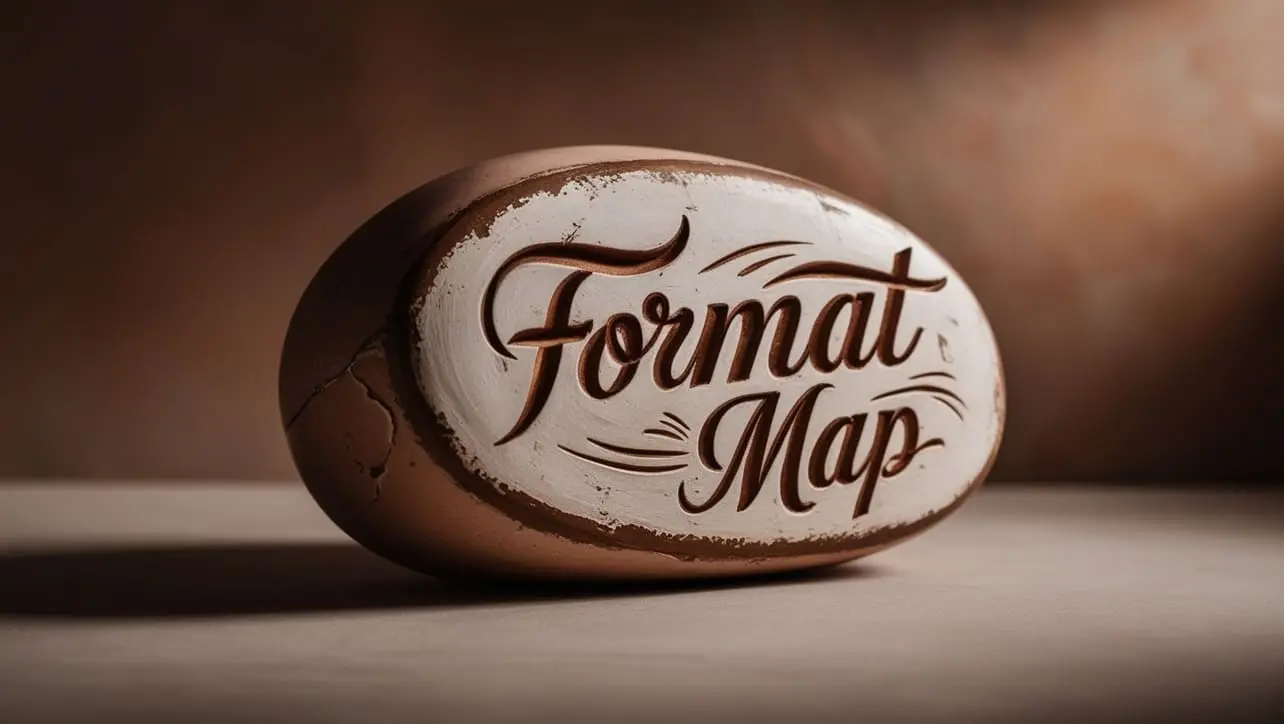
Python string format() Method
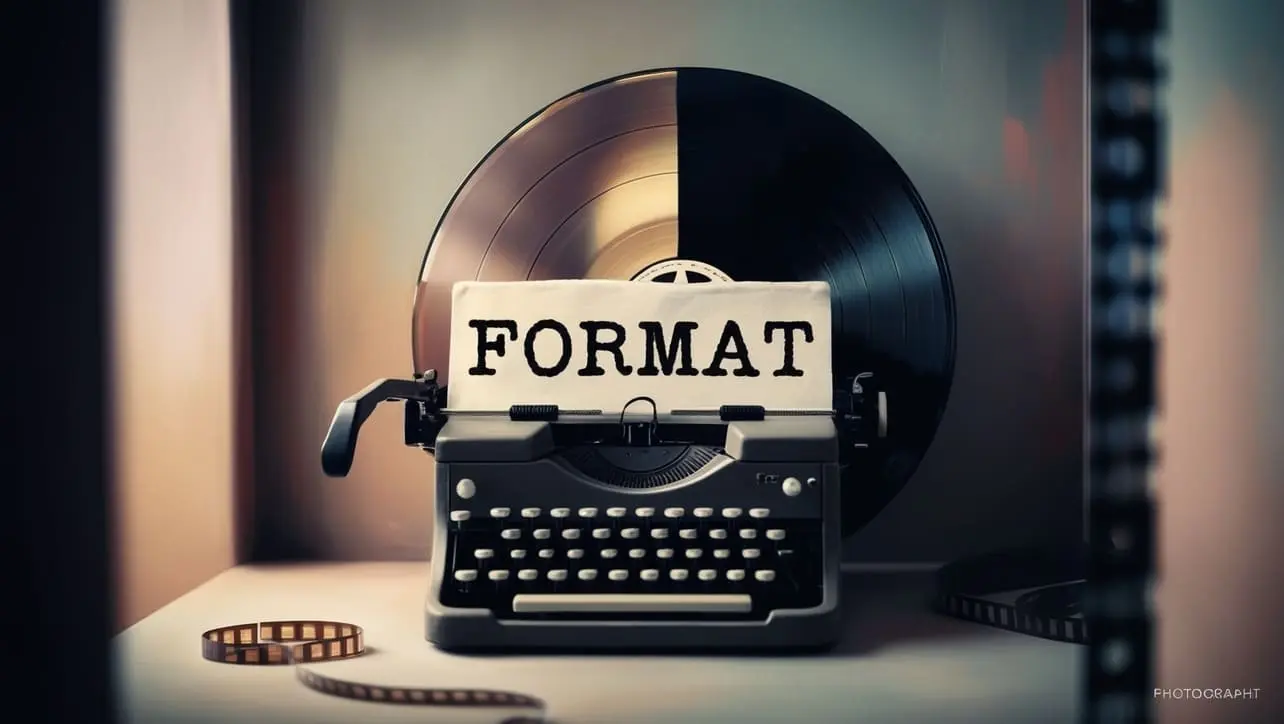
Photo Credit to CodeToFun
Introduction
In Python, the format()
method is a versatile and powerful tool for formatting strings.
It allows you to create dynamic strings by inserting values into placeholders within a string template.
In this tutorial, we'll explore the syntax, usage, and various features of the format()
method in Python.
Syntax
The syntax for the format()
method is as follows:
string.format(value1, value2, ...)
- string: The string containing placeholders to be replaced.
- value1, value2, ...: Values to be inserted into the placeholders.
Example
Let's start with a basic example to illustrate how the format()
method works.
# Example 1
name = "Alice"
age = 30
sentence = "My name is {} and I am {} years old.".format(name, age)
print("Example 1:", sentence)
Testing the Program
Example 1: My name is Alice and I am 30 years old.
How the Program Works
In Example 1, the format()
method replaces the curly braces {} in the string with the values of name and age, resulting in the output: My name is Alice and I am 30 years old.
Return Value
The format()
method does not return a new string; instead, it modifies the original string in place. Therefore, the return value is None. Keep this in mind when using the format()
method within expressions.
Common Use Cases
Placeholder Indexing:
You can use numerical indices inside the curly braces to specify the order of the values.
placeholder-indexing.pyCopied# Example 2 item1 = "apple" item2 = "banana" sentence = "I have {1} and {0}.".format(item1, item2) print("Example 2:", sentence)
OutPut
OutputExample 2: I have banana and apple.
How the Program Works
In Example 2, the values of item1 and item2 are inserted based on their index positions, resulting in the output: "I have banana and apple."
Named Placeholders:
Named placeholders provide a clear way to specify which value corresponds to which placeholder.
named-placeholders.pyCopied# Example 3 sentence = "The {fruit} is {adjective}.".format(fruit="orange", adjective="delicious") print("Example 3:", sentence)
OutPut
OutputExample 3: The orange is delicious.
How the Program Works
In Example 3, named placeholders fruit and adjective are replaced with the specified values, resulting in the output: "The orange is delicious."
Formatting Numbers:
The
format()
method allows you to control the formatting of numerical values.formatting-numbers.pyCopied# Example 4 price = 19.95 formatted_price = "The price is ${:.2f}.".format(price) print("Example 4:", formatted_price)
OutPut
OutputExample 4: The price is $19.95.
How the Program Works
In Example 4, the :.2f inside the placeholder formats the price as a floating-point number with two decimal places, resulting in the output: "The price is $19.95."
Notes
- The
format()
method is more flexible and recommended over the older % formatting method. - Python 3.6 introduced f-strings, which provide a more concise and readable way to format strings. However, the
format()
method remains a powerful option.
Optimization
The format()
method is optimized for performance and readability. However, if you are working with Python 3.6 or later, you may consider using f-strings for even more concise and readable string formatting
# Using f-strings (Python 3.6+)
name = "Bob"
age = 25
formatted_string = f"Hello, my name is {name} and I'm {age} years old."
print(formatted_string)
Conclusion
The format()
method in Python is a versatile and essential tool for string formatting. Whether you're dealing with simple substitutions or complex formatting requirements, the format()
method provides a robust solution.
Feel free to experiment with different string templates and values to explore the full capabilities of the format()
method. Happy coding!
Join our Community:
Author
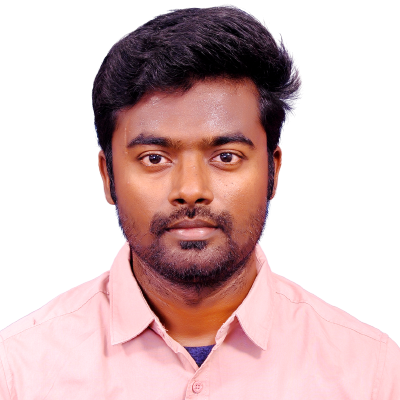
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in Python
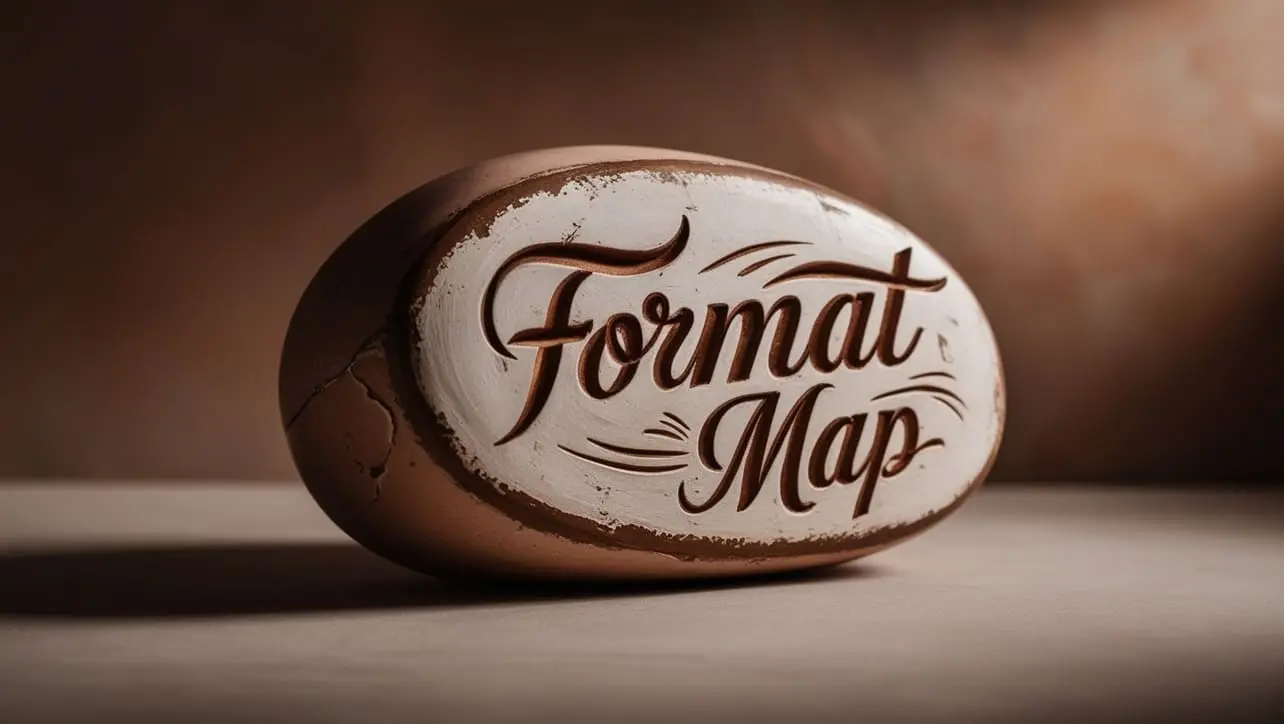
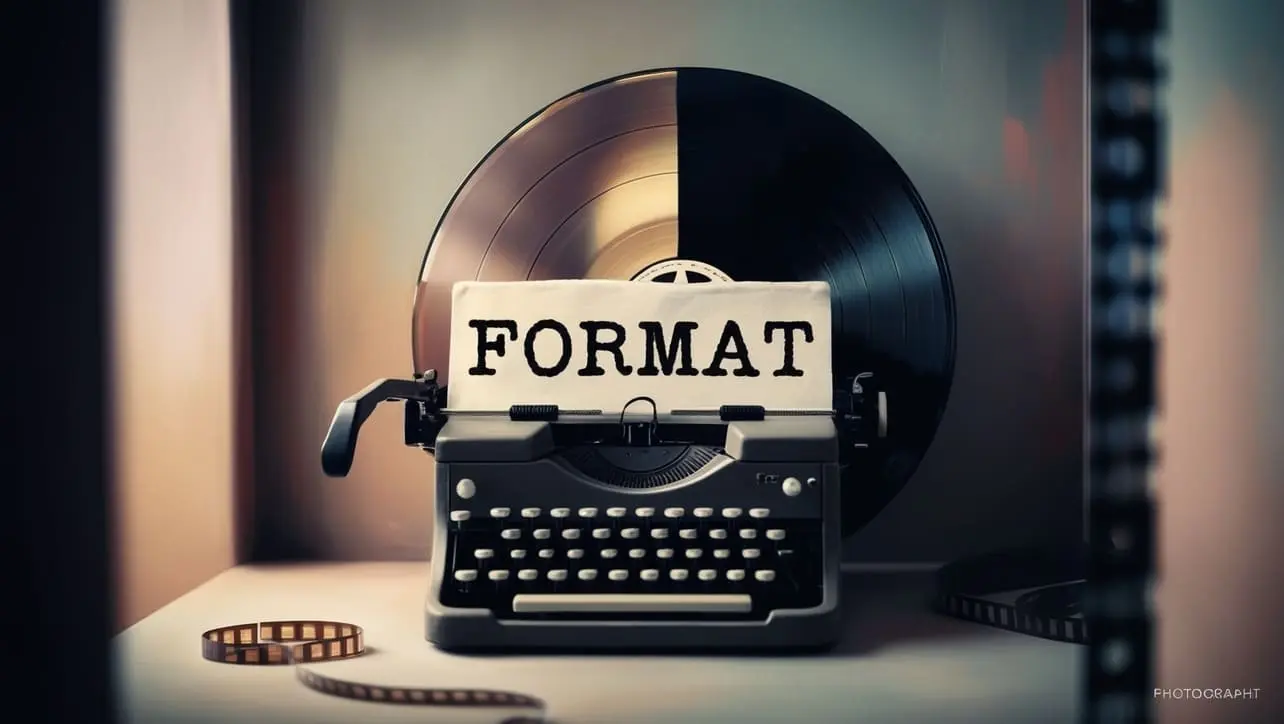
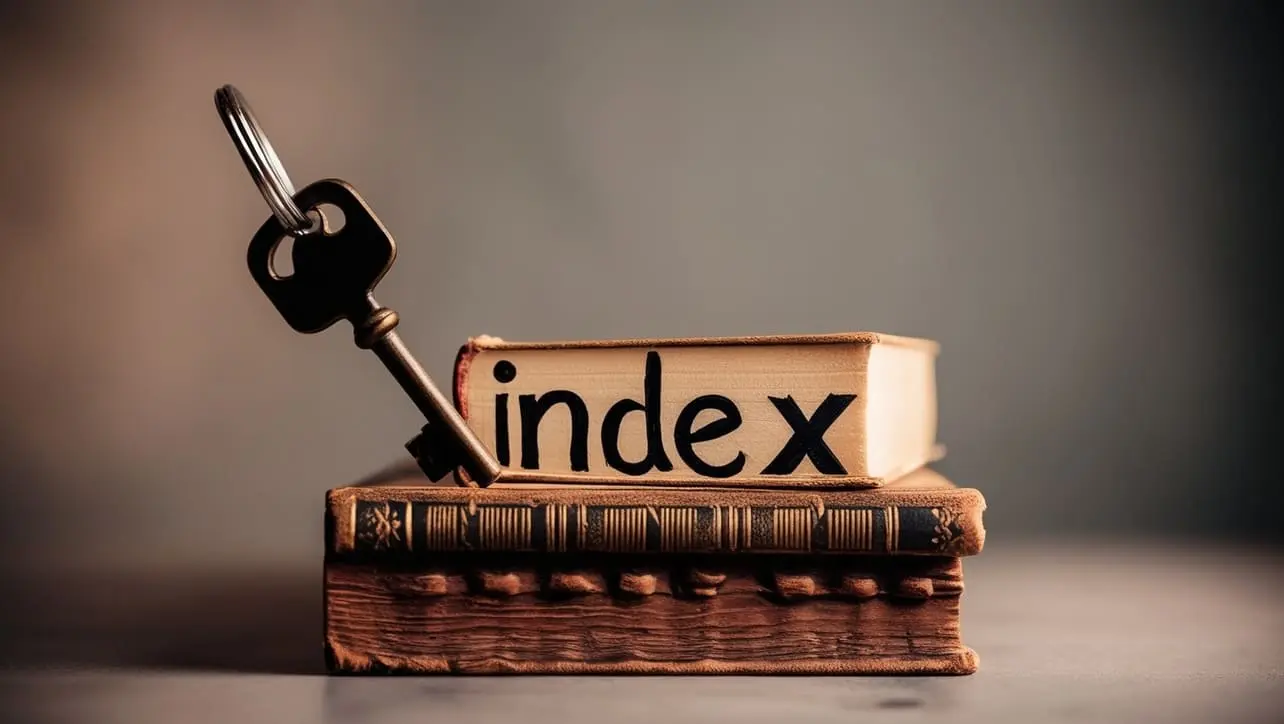
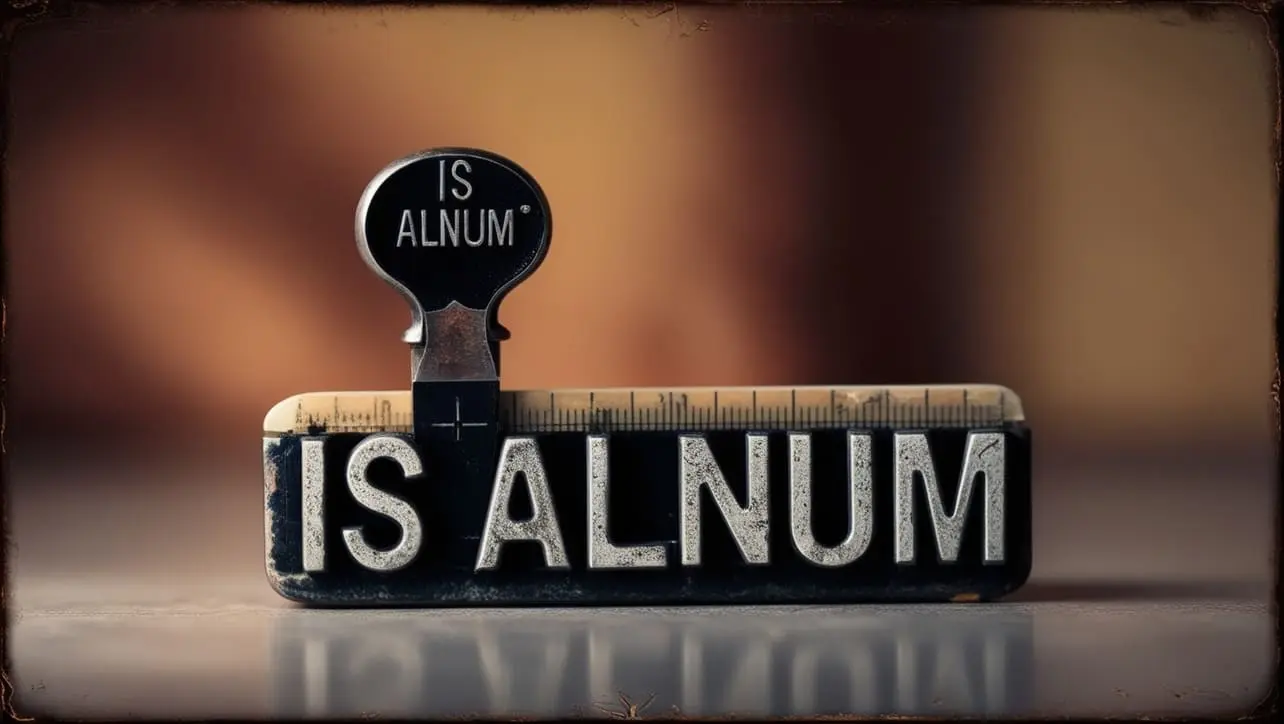
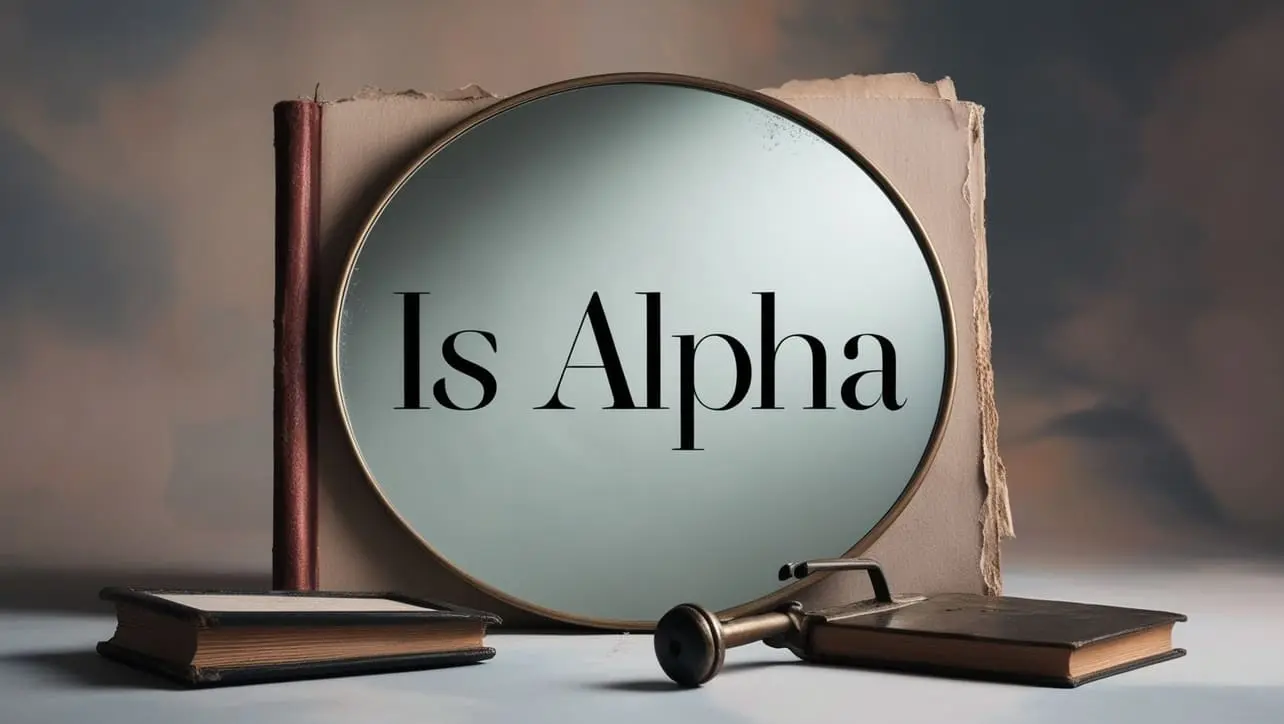
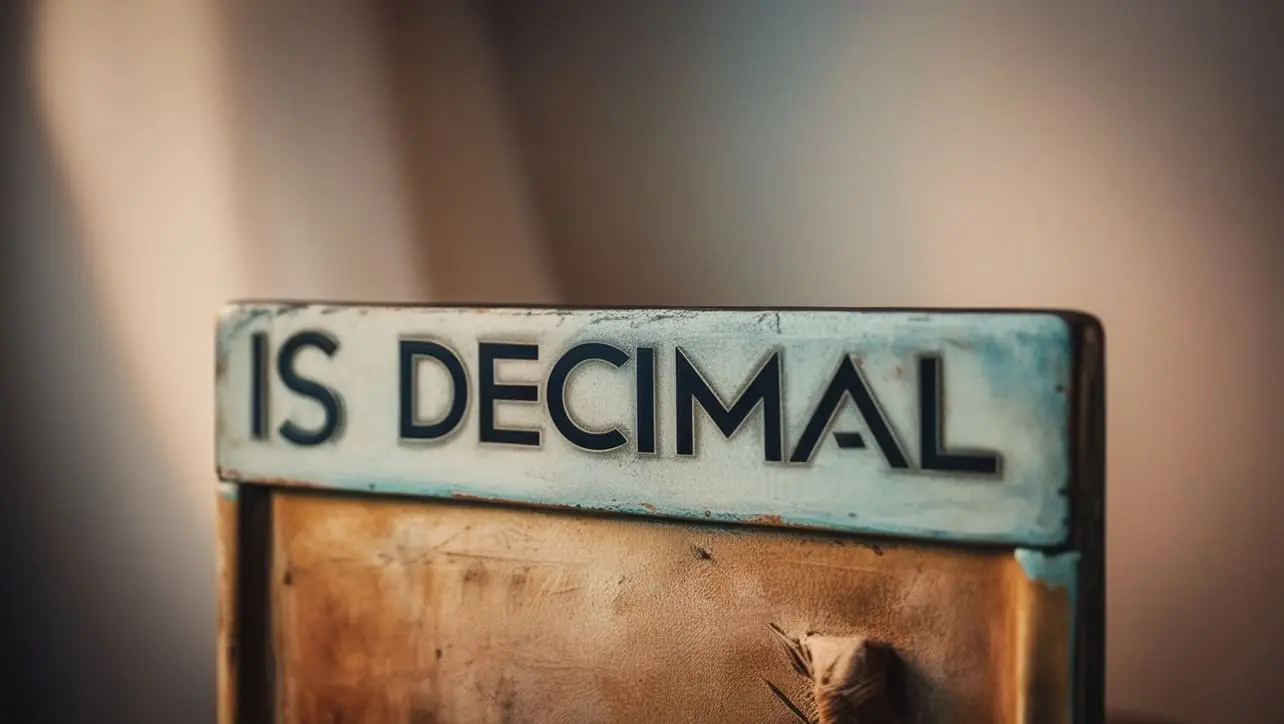
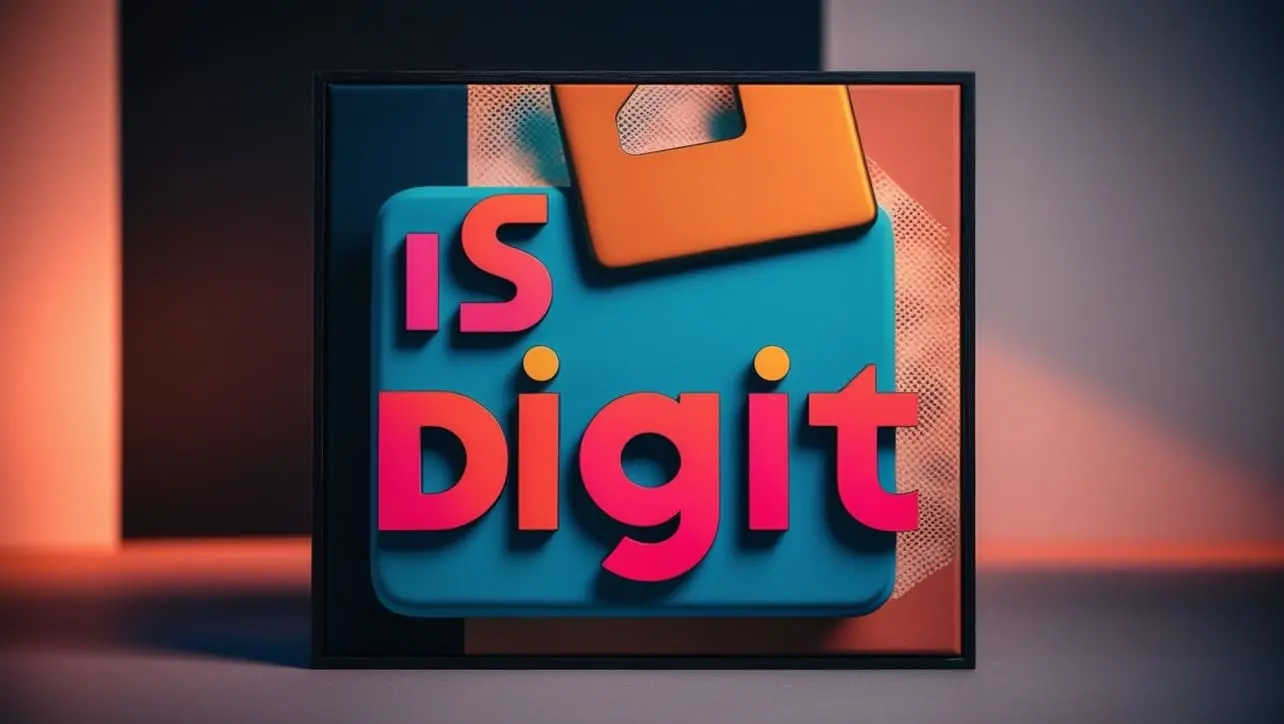
If you have any doubts regarding this article (Python string format() Method), please comment here. I will help you immediately.