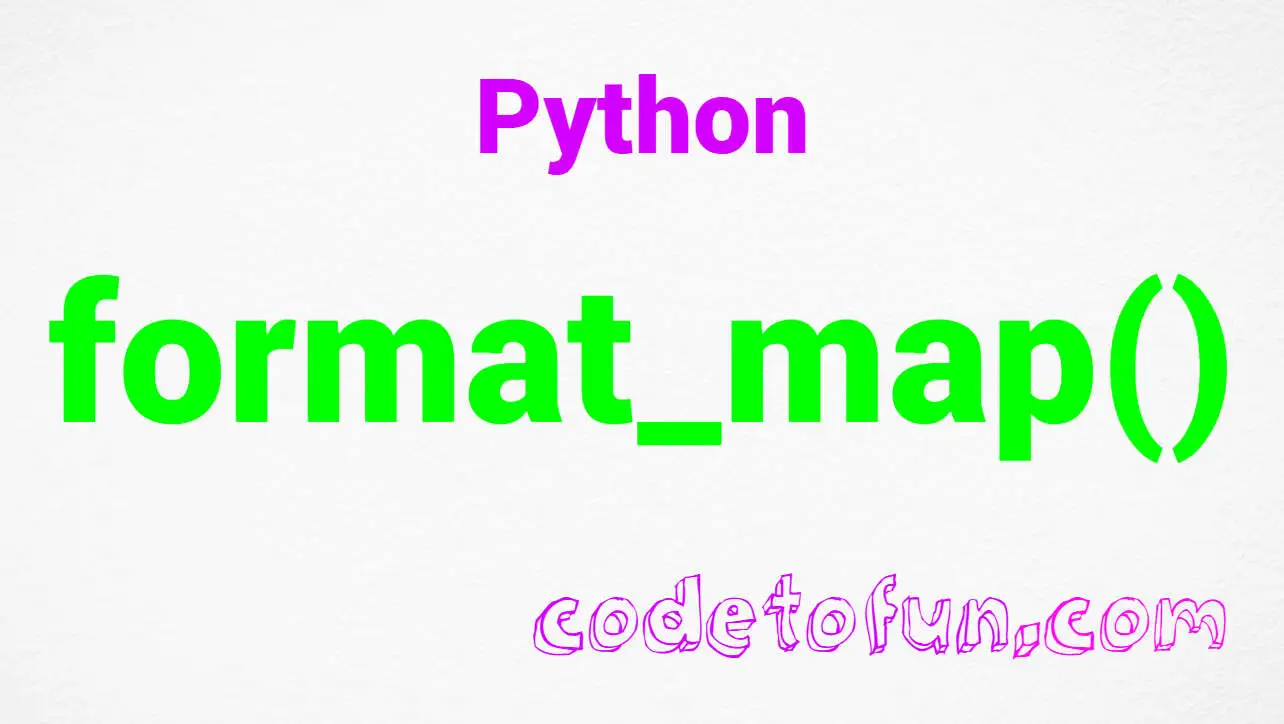
Python Basic
Python String Methods
Python string endswith() Method
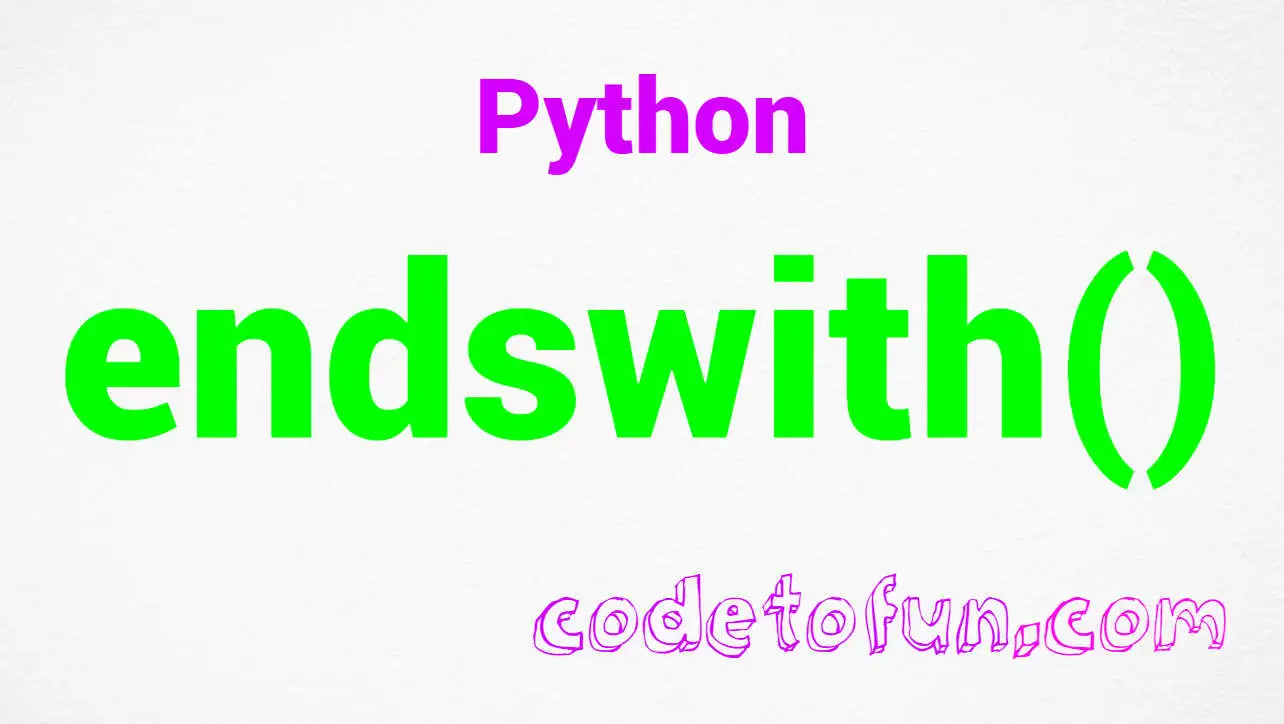
Photo Credit to CodeToFun
🙋 Introduction
In Python, working with strings involves a variety of methods that provide useful functionalities. One such method is endswith()
, which is used to determine whether a string ends with a specified suffix.
This method is valuable when you need to perform conditional checks on the endings of strings.
🤓 Understanding the endswith() Method
The endswith()
method is a built-in string method in Python that returns True if a string ends with a specified suffix and False otherwise. It is a powerful tool for string manipulation and conditional logic.
💡 Syntax
The syntax for the endswith()
method is:
string.endswith(suffix[, start[, end]])
- string: The original string.
- suffix: The suffix to check for at the end of the string.
- start (optional): The starting index for the search.
- end (optional): The ending index for the search.
📄 Example
Let's explore a simple example to understand how the endswith()
method works:
# Using endswith() method
file_name = "document.txt"
is_text_file = file_name.endswith(".txt")
# Displaying the result
print(f"The file {file_name} is a text file: {is_text_file}")
💻 Testing the Program
The file document.txt is a text file: True
🧠 How the Program Works
In this example, the endswith()
method checks if the string "document.txt" ends with the suffix ".txt," and the result is True.
📚 Common Use Cases
File Type Verification:
file-type-verification.pyCopiedfile_name = "image.jpg" is_image_file = file_name.endswith((".jpg", ".jpeg", ".png"))
URL Validation:
url-validation.pyCopiedurl = "https://example.com" is_https = url.endswith("s")
Checking File Extensions:
checking-file-extensions.pyCopiedfile_path = "/path/to/file/document.pdf" is_pdf = file_path.endswith(".pdf")
📝 Notes
- The
endswith()
method is case-sensitive, so be mindful of the case when specifying the suffix. - If you need a case-insensitive check, consider converting both the string and suffix to lowercase using the lower() method before using
endswith()
.
🎢 Optimization
While the endswith()
method is efficient for simple suffix checks, if you need more complex pattern matching, regular expressions (re module) might be a more suitable option.
🎉 Conclusion
The endswith()
method is a versatile tool for checking the endings of strings, offering a convenient way to perform conditional checks in Python. Whether you're validating file types, URLs, or specific patterns, this method provides a straightforward solution.
Feel free to incorporate and modify the code examples for your specific use case. Happy coding!
👨💻 Join our Community:
Author
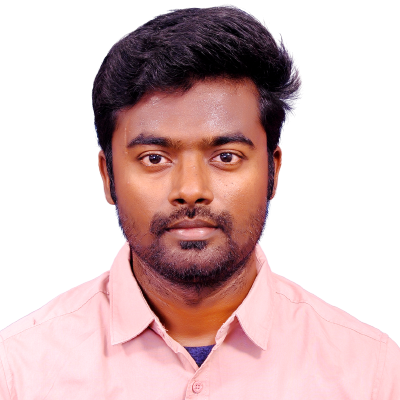
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (Python string endswith() Method), please comment here. I will help you immediately.