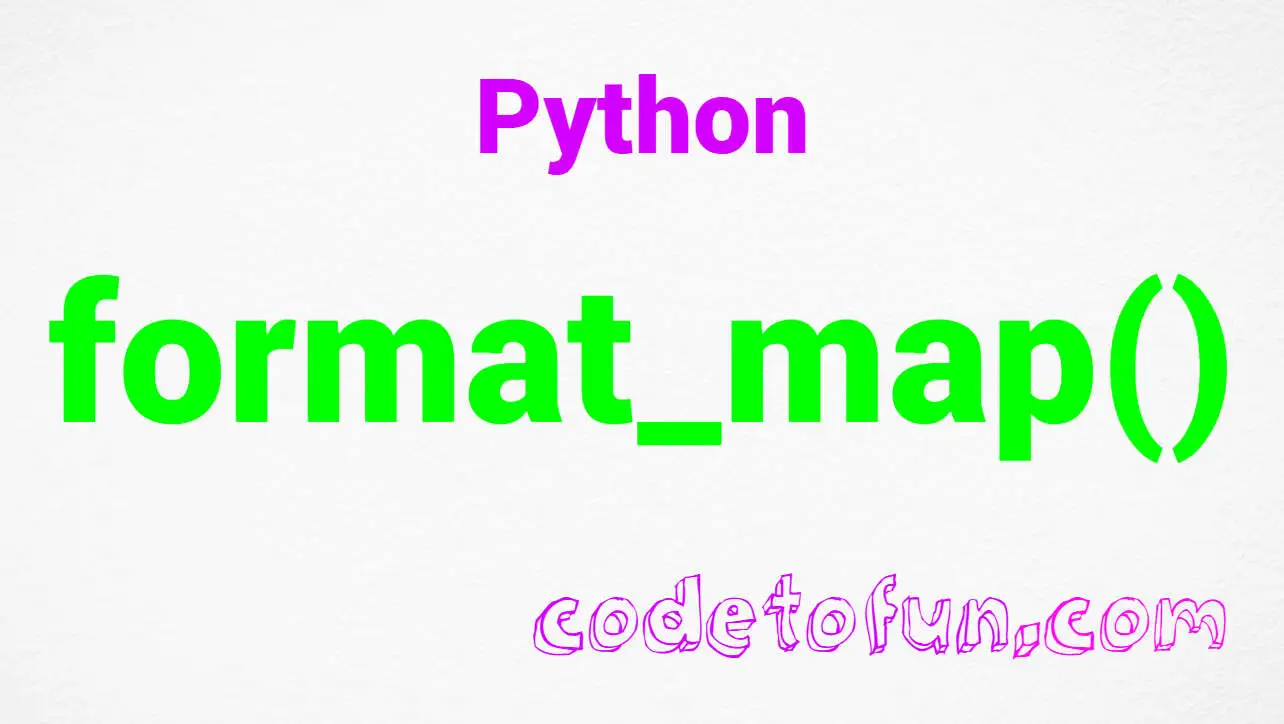
Python Basic
Python String Methods
Python string encode() Method
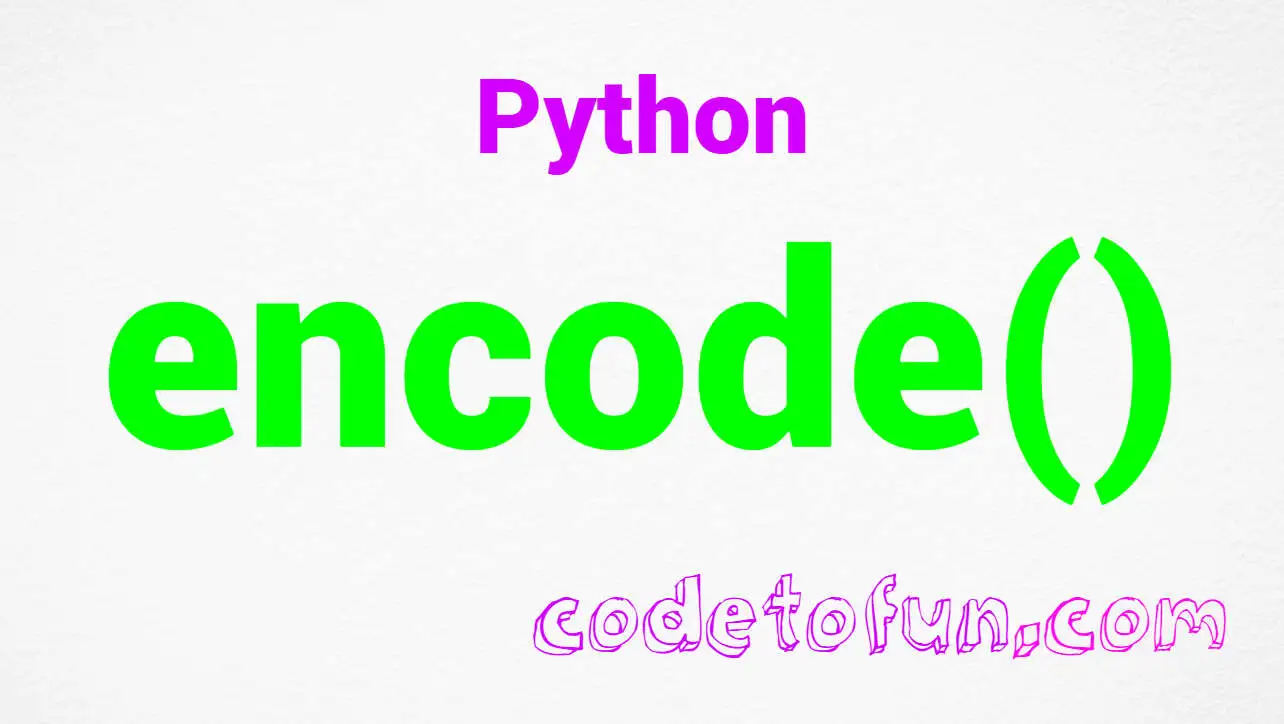
Photo Credit to CodeToFun
🙋 Introduction
In Python, the encode()
method is a powerful tool for string manipulation, particularly when dealing with Unicode and character encoding.
This method is used to encode a string into a specified encoding format, such as UTF-8 or ASCII.
Understanding and using the encode()
method is essential when working with text data in different contexts, such as file handling or network communication.
🤓 Understanding the casefold() Method
The encode()
method is a built-in string method in Python that returns an encoded version of the string.
It takes an optional parameter, the encoding format, which specifies the character encoding to be used. If no encoding is specified, the default encoding is used.
💡 Syntax
The syntax for the encode()
method is:
string.encode(encoding='UTF-8', errors='strict')
- string: The original string you want to encode.
- encoding (optional): The character encoding to be used (default is 'UTF-8').
- errors (optional): The error handling scheme (default is 'strict').
📄 Example
Let's explore a simple example to understand how the encode()
method works:
# Using encode() method
original_string = "Python is amazing!"
encoded_string = original_string.encode(encoding='utf-8')
# Displaying the results
print("Original String:", original_string)
print("Encoded String:", encoded_string)
💻 Testing the Program
Original String: Python is amazing! Encoded String: b'Python is amazing!'
🧠 How the Program Works
In this example, the encode()
method encodes the original string "Python is amazing!" using the UTF-8 encoding, resulting in a bytes object.
📚 Common Use Cases
File Handling:
file-handling.pyCopiedwith open('file.txt', 'w', encoding='utf-8') as file: file.write("This is a sample text.")
Network Communication:
network-communication.pyCopieddata = "Hello, server!".encode('utf-8')
URL Encoding:
url-encoding.pyCopiedimport urllib.parse encoded_url = urllib.parse.quote("https://example.com/page with space")
📝 Notes
- The
encode()
method returns a bytes object, not a string. The 'b' prefix before the output indicates a bytes object. - The errors parameter specifies the response when encoding fails. The default, 'strict', raises a UnicodeEncodeError.
🎢 Optimization
If you need to decode the encoded bytes back into a string, you can use the decode() method.
🎉 Conclusion
The encode()
method is a crucial tool for handling character encoding in Python, especially when working with diverse data sources and formats. Understanding how to encode and decode strings is essential for robust and efficient text processing in your Python programs.
Feel free to incorporate and modify the code examples for your specific use case. Happy coding!
👨💻 Join our Community:
Author
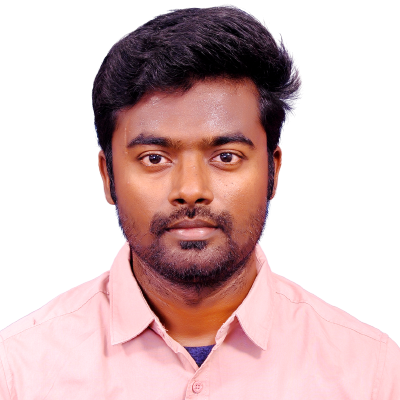
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (Python string encode() Method), please comment here. I will help you immediately.