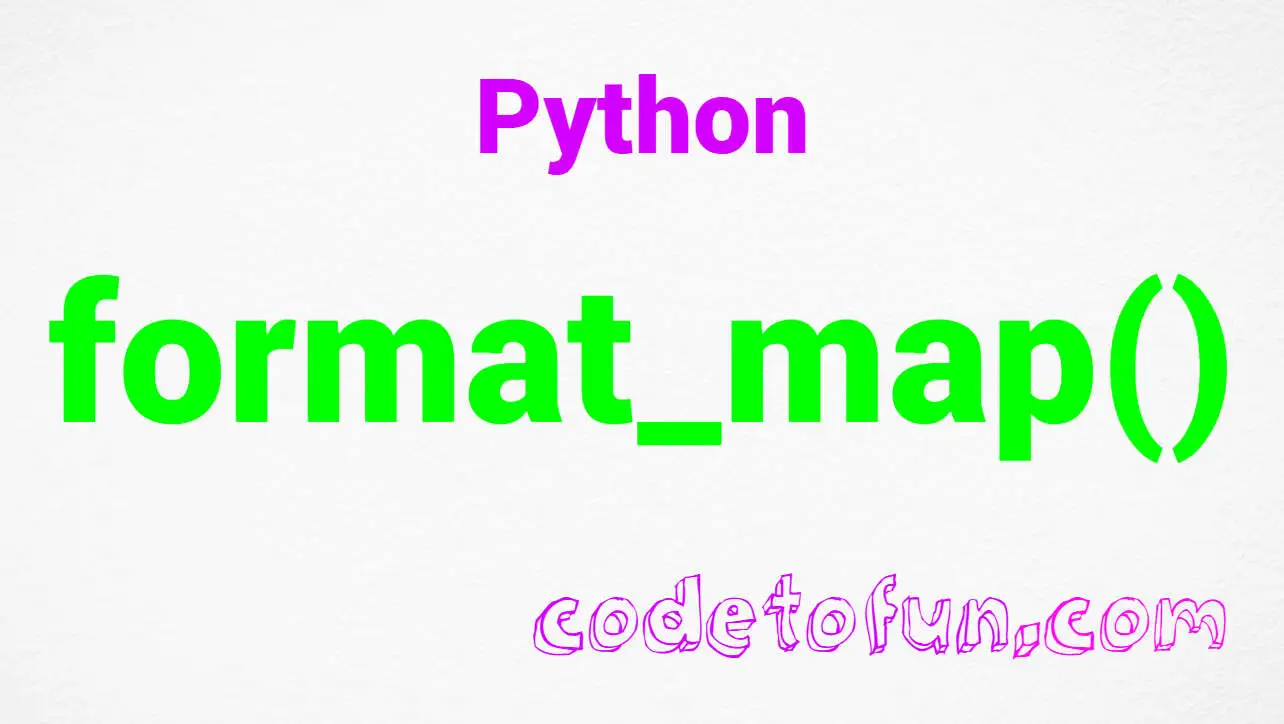
Python Basic
Python String Methods
Python string center() Method
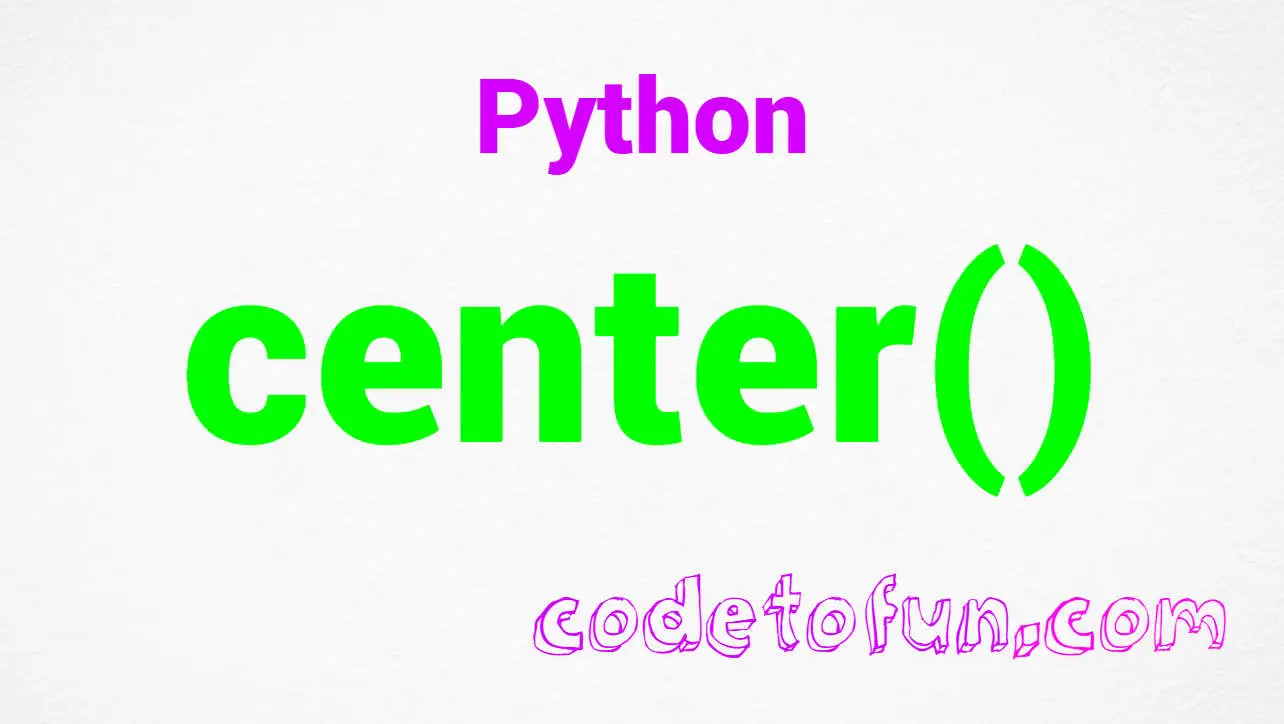
Photo Credit to CodeToFun
🙋 Introduction
In Python, strings are versatile and come with a variety of built-in methods for manipulation and formatting.
The center()
method is one such method used to center-align a string within a specified width.
This is particularly useful when you want to present text in a visually appealing way, such as when formatting output or creating tables.
🤓 Understanding the casefold() Method
The center()
method is a built-in string method in Python that returns a centered version of the original string.
It takes two parameters: width and an optional fillchar.
The width parameter specifies the total width of the centered string, and fillchar (if provided) is used to fill the remaining space.
If fillchar is not provided, it defaults to a space.
💡 Syntax
The syntax for the center()
method is:
string.center(width, fillchar)
Here, string is the original string, width is the total width of the centered string, and fillchar is an optional parameter for filling the remaining space.
📄 Example
Let's explore a simple example to understand how the center()
method works:
# Using center() method
original_string = "Python"
centered_string = original_string.center(10, '*')
# Displaying the results
print("Original String:", original_string)
print("Centered String:", centered_string)
💻 Testing the Program
Original String: Python Centered String: **Python**
🧠 How the Program Works
In this example, the center()
method centers the original string "Python" within a width of 10 characters, using * as the fill character.
📚 Common Use Cases
Text Alignment in Output:
text-alignment.pyCopiedheader = "Welcome to Python".center(30, '-')
Creating Tables:
creating-tables.pyCopiedtable_row = "Data 1 | Data 2".center(30, ' ')
Formatting Displayed Text:
formatting-displayed-text.pyCopiedformatted_text = "Important Message".center(50, '*')
📝 Notes
- If the specified width is less than or equal to the length of the original string, the method returns the original string unchanged.
- If the fillchar is not specified, it defaults to a space character.
🎢 Optimization
For more dynamic alignment, consider using the ljust() and rjust() methods for left and right justification, respectively.
🎉 Conclusion
The center()
method is a valuable tool for formatting and aligning strings in Python.
Whether you're creating visually appealing output or organizing data in a table, this method provides an easy way to achieve centered alignment.
Feel free to incorporate and modify the code examples for your specific use case. Happy coding!
👨💻 Join our Community:
Author
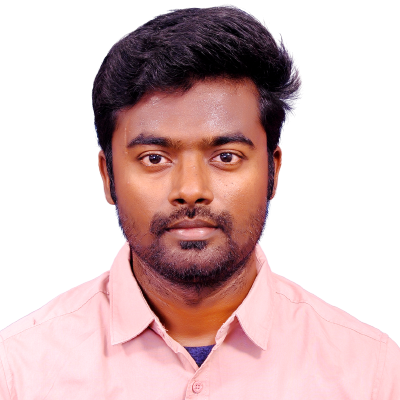
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (Python string center() Method), please comment here. I will help you immediately.