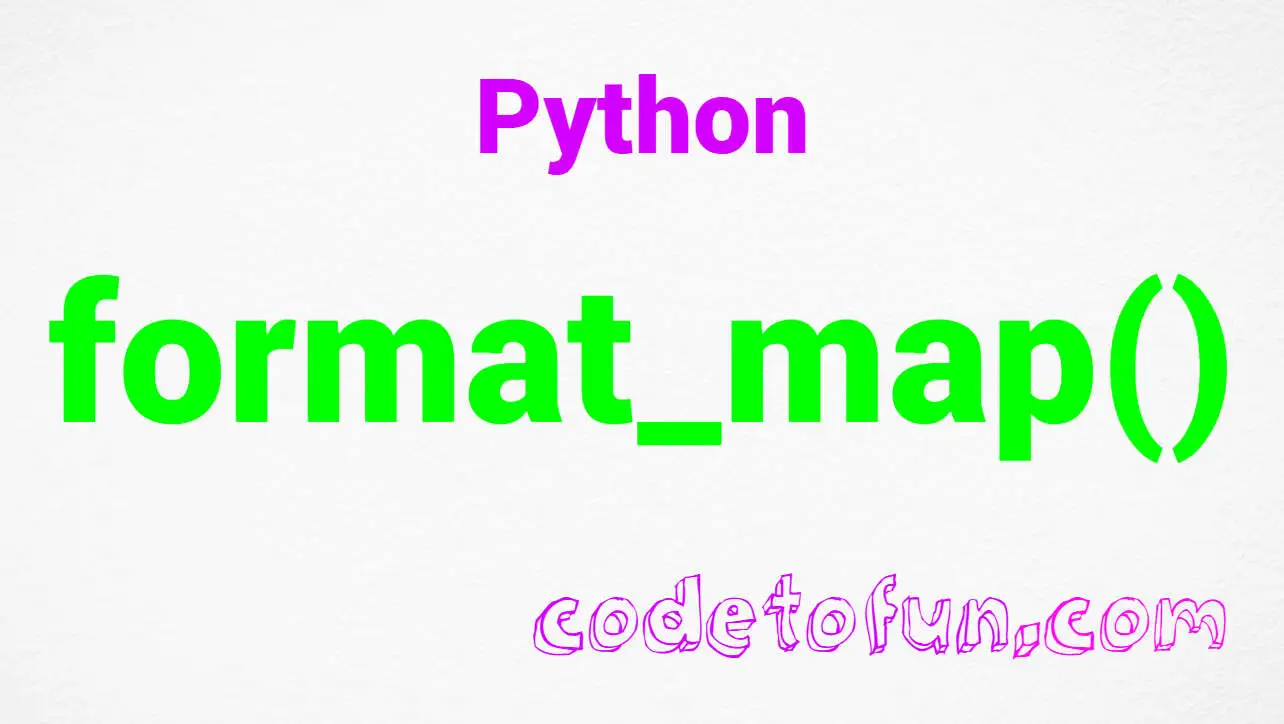
Python Basic
Python String Methods
Python string capitalize() Method
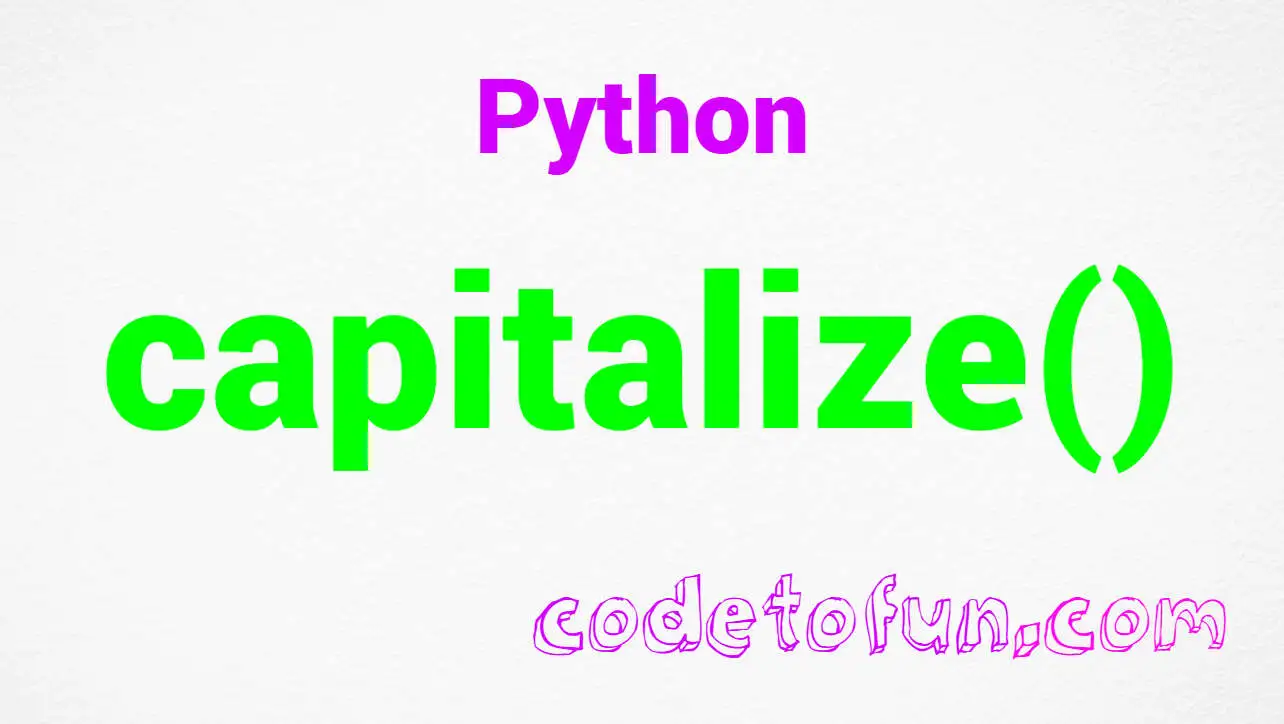
Photo Credit to CodeToFun
🙋 Introduction
In Python, strings are a fundamental data type, and Python provides a rich set of string manipulation methods. One such method is capitalize()
, which is used to capitalize the first character of a string.
This method is particularly useful when you want to present data in a consistent and visually appealing manner.
🤓 Understanding the capitalize() Method
The capitalize()
method is a built-in string method in Python that returns a copy of the original string with its first character capitalized and the rest of the characters in lowercase.
If the string is already capitalized or is empty, the method returns the original string.
💡 Syntax
Here is the syntax of the capitalize()
method:
string.capitalize()
Here, string is the original string for which you want to capitalize the first character.
📄 Example
Let's explore a simple example to understand how the capitalize()
method works:
# Using capitalize() method
original_string = "python is fun"
capitalized_string = original_string.capitalize()
# Displaying the results
print("Original String:", original_string)
print("Capitalized String:", capitalized_string)
💻 Testing the Program
Original String: python is fun Capitalized String: Python is fun
🧠 How the Program Works
In this example, the capitalize()
method capitalizes the first character of the original string "python is fun," resulting in "Python is fun."
📚 Common Use Cases
User Input Handling:
user-input-handle.pyCopiedusername = input("Enter your username: ").capitalize()
Formatting Names:
user-input-handle.pyCopiedfull_name = "john doe".capitalize()
Title Case Conversion:
user-input-handle.pyCopiedtitle = "the art of programming".capitalize()
📝 Notes
- The
capitalize()
method does not modify the original string; instead, it returns a new string with the desired capitalization. - If the string contains non-alphabetic characters after the first character, they remain unchanged.
🎢 Optimization
While the capitalize()
method is convenient for capitalizing the first character, if you need more sophisticated capitalization, consider exploring the title() method for title case conversion or custom functions.
🎉 Conclusion
The capitalize()
method is a handy tool when you need to ensure that the first character of a string is capitalized. It plays a role in improving the readability and presentation of text data in Python.
Feel free to incorporate and modify the code examples for your specific use case. Happy coding!
👨💻 Join our Community:
Author
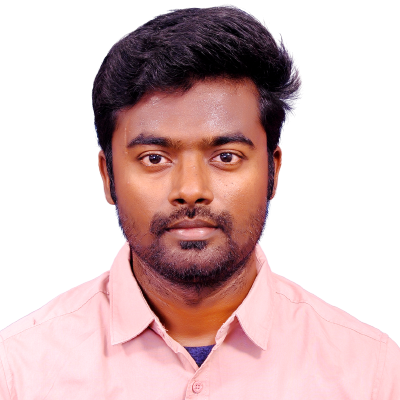
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (Python string capitalize() Method), please comment here. I will help you immediately.