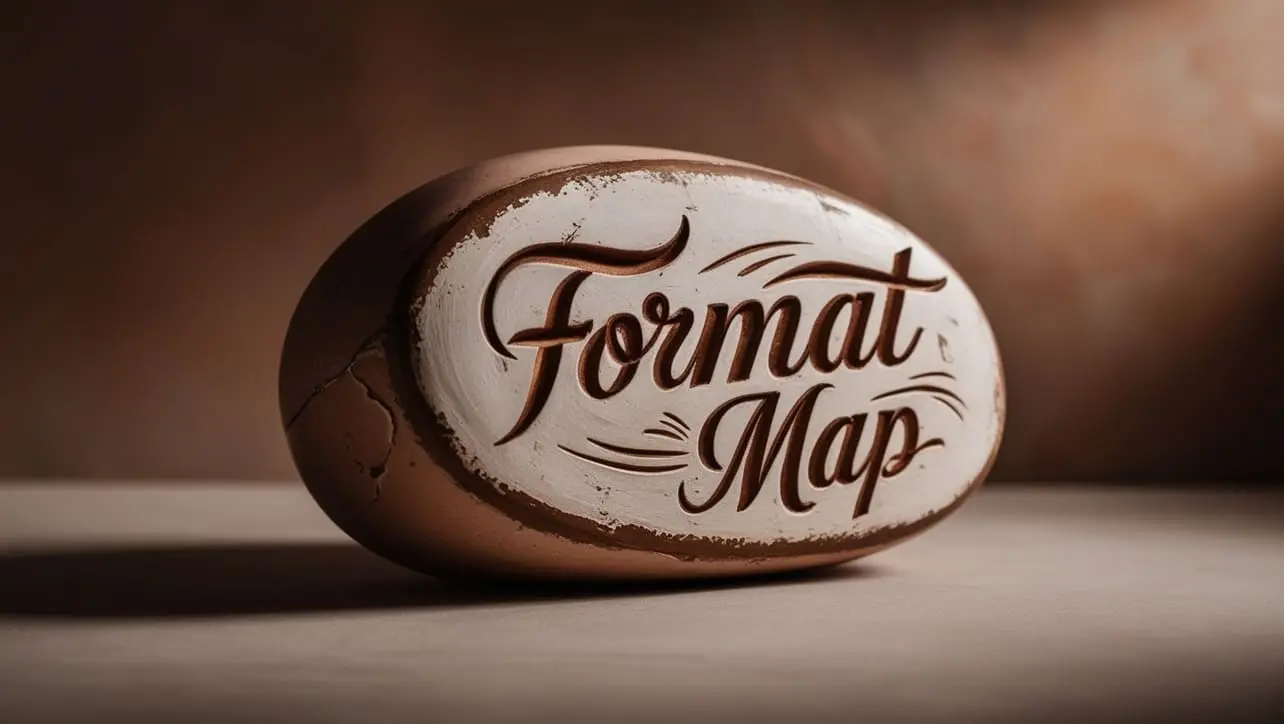
Python Topics
- Python Intro
- Python String Methods
- Python Interview Programs
- Abundant Number
- Amicable Number
- Armstrong Number
- Average of N Numbers
- Automorphic Number
- Biggest of three numbers
- Binary to Decimal
- Common Divisors
- Composite Number
- Condense a Number
- Cube Number
- Decimal to Binary
- Decimal to Octal
- Disarium Number
- Even Number
- Evil Number
- Factorial of a Number
- Fibonacci Series
- GCD
- Happy Number
- Harshad Number
- LCM
- Leap Year
- Magic Number
- Matrix Addition
- Matrix Division
- Matrix Multiplication
- Matrix Subtraction
- Matrix Transpose
- Maximum Value of an Array
- Minimum Value of an Array
- Multiplication Table
- Natural Number
- Number Combination
- Odd Number
- Palindrome Number
- Pascalβs Triangle
- Perfect Number
- Perfect Square
- Power of 2
- Power of 3
- Pronic Number
- Prime Factor
- Prime Number
- Smith Number
- Strong Number
- Sum of Array
- Sum of Digits
- Swap Two Numbers
- Triangular Number
- Python Star Pattern
- Python Number Pattern
- Python Alphabet Pattern
Python Program to Display Multiplication Table
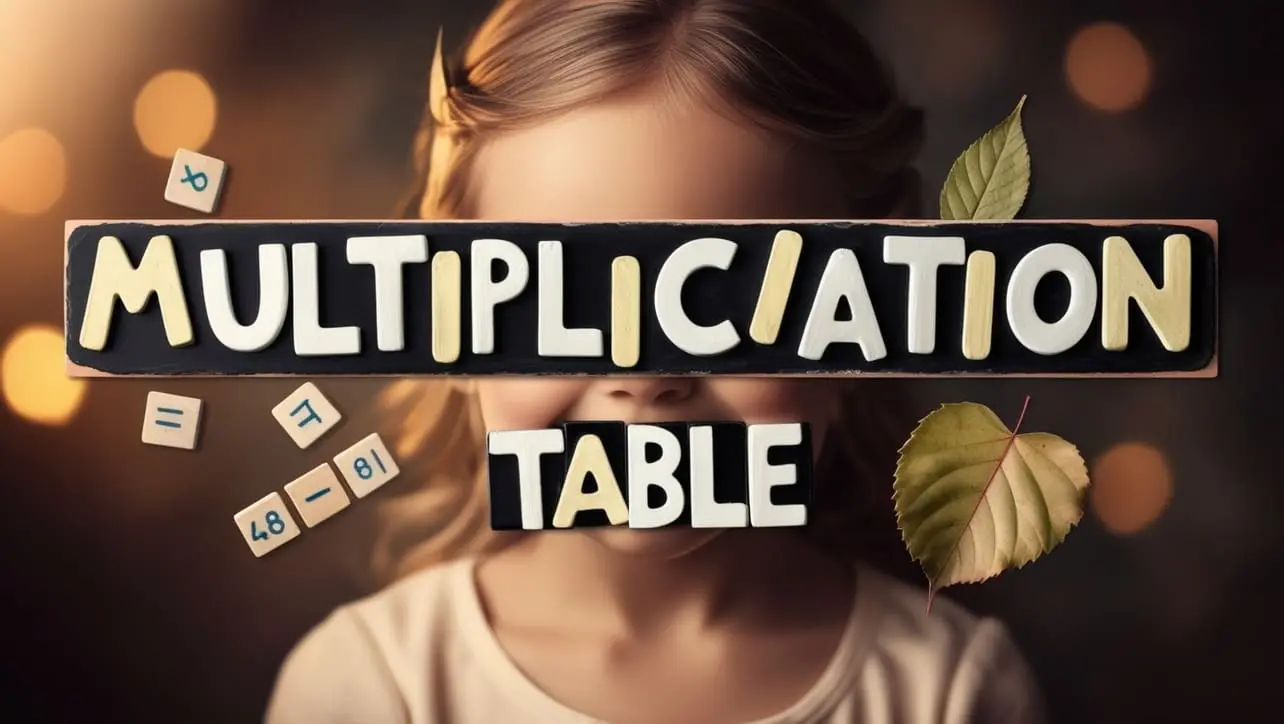
Photo Credit to CodeToFun
Introduction
Multiplication tables are fundamental in mathematics and play a crucial role in various programming applications. Creating a program to display the multiplication table for a fixed number is a common exercise for beginners in programming.
In this tutorial, we'll explore a Python program that displays the multiplication table for the fixed number 5.
Example
Let's delve into the Python code that accomplishes this task.
# Function to display the multiplication table for the fixed number 5
def display_multiplication_table():
# Fixed multiplication factor
number = 5
print(f"Multiplication Table for {number}:\n")
# Iterate from 1 to 10 and display the result of multiplication
for i in range(1, 11):
print(f"{number} x {i} = {number * i}")
# Call the function to display the multiplication table
display_multiplication_table()
Testing the Program
Run the program, and it will display the multiplication table for the fixed number 5 from 1 to 10.
Multiplication Table for 5: 5 x 1 = 5 5 x 2 = 10 5 x 3 = 15 5 x 4 = 20 5 x 5 = 25 5 x 6 = 30 5 x 7 = 35 5 x 8 = 40 5 x 9 = 45 5 x 10 = 50
How the Program Works
- The program defines a function display_multiplication_table that displays the multiplication table for the fixed number 5 using a for loop.
- The function is then called to display the multiplication table.
Understanding the Concept of Multiplication Table
Multiplication tables are essential in mathematics and are often used in programming for various applications. This program provides a simple and effective way to display the multiplication table for a given number.
Optimizing the Program
While the provided program is straightforward, consider exploring and implementing alternative approaches or optimizations for displaying multiplication tables.
Feel free to incorporate and modify this code as needed for your specific use case. Happy coding!
Join our Community:
Author
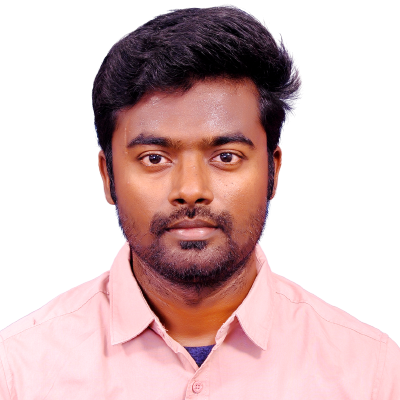
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in Python
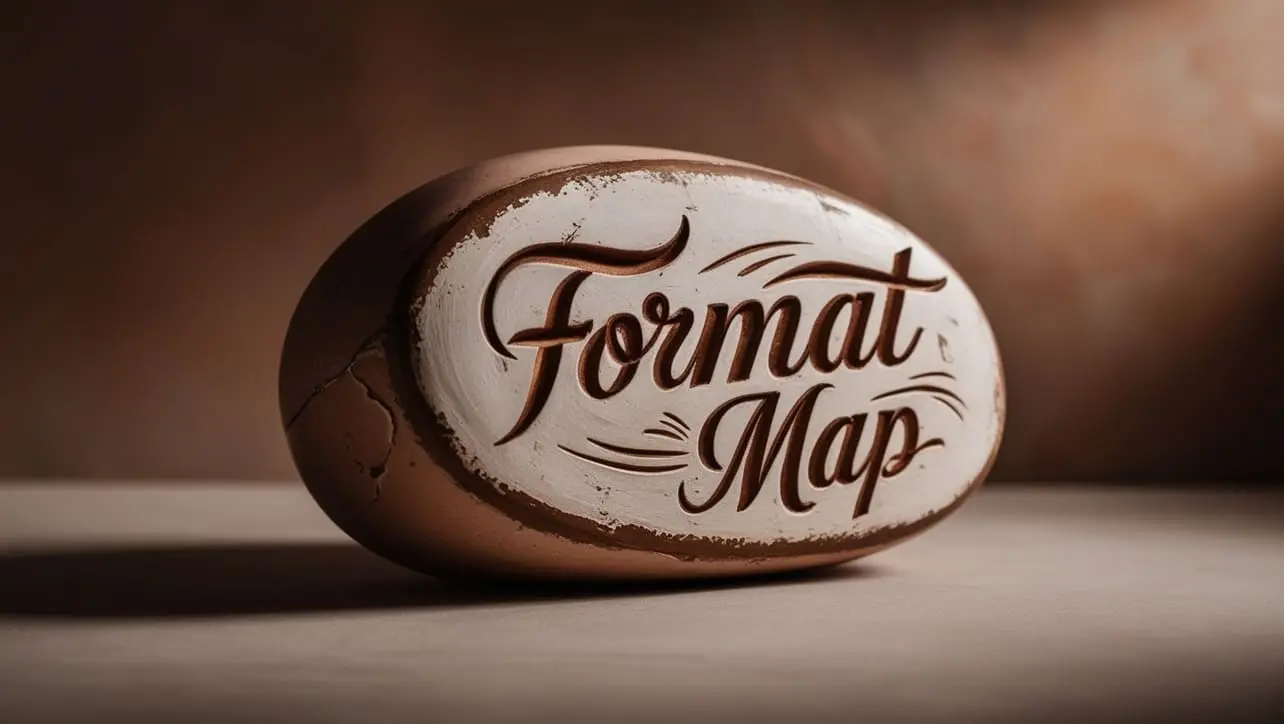
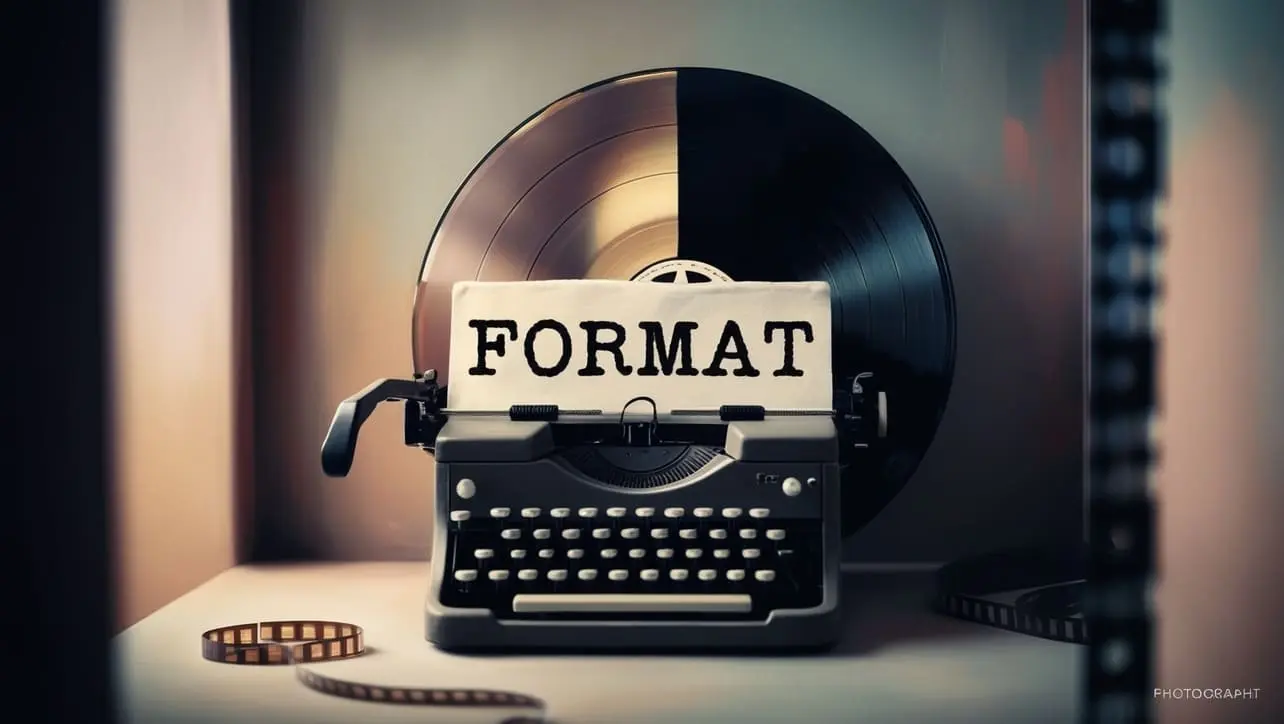
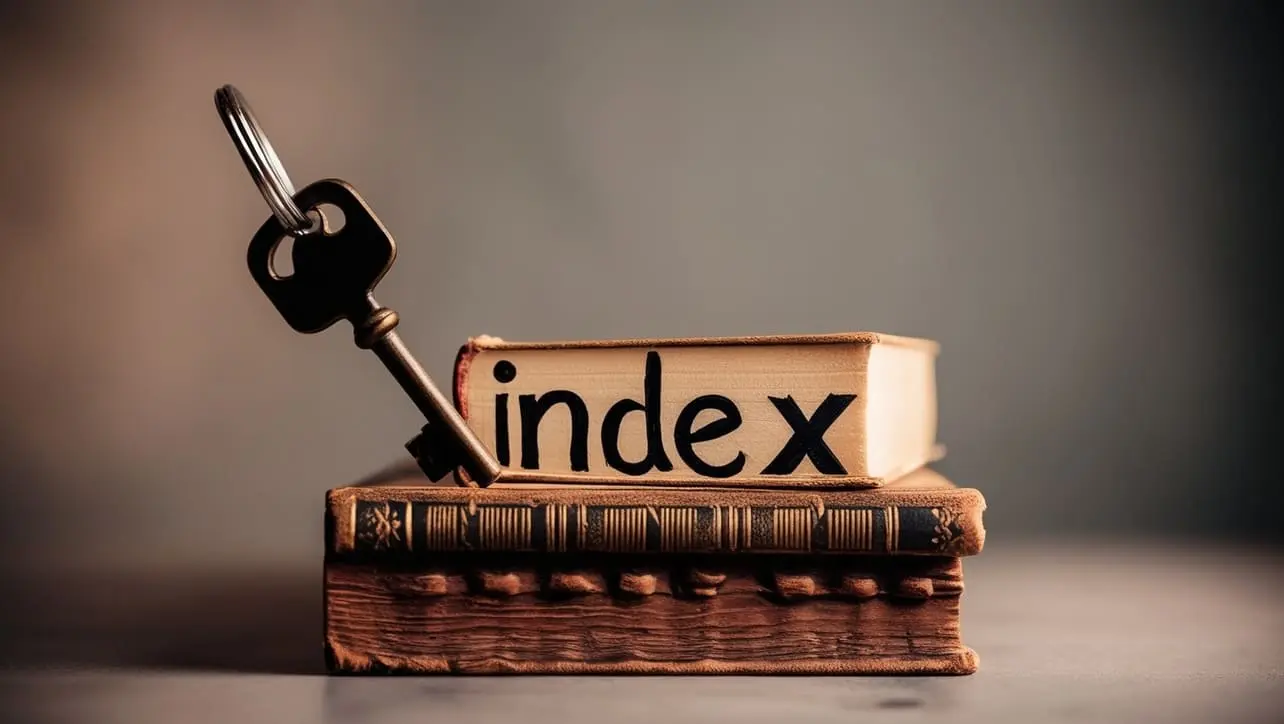
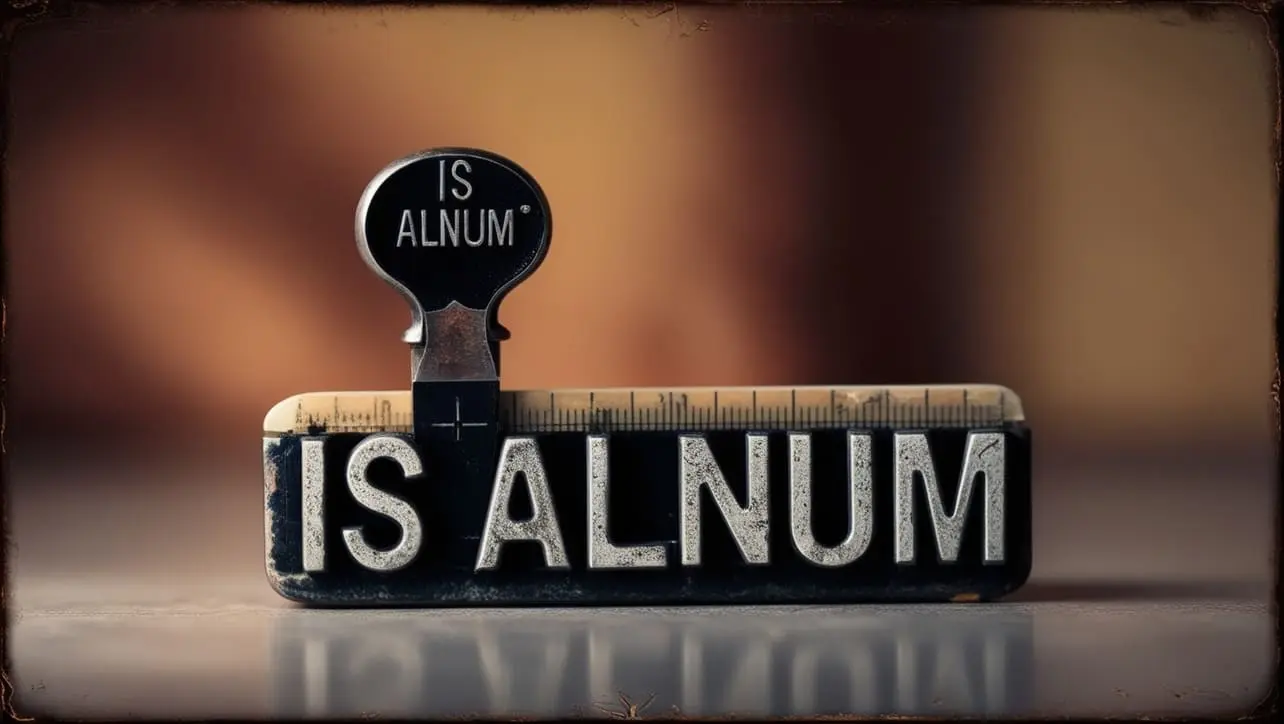
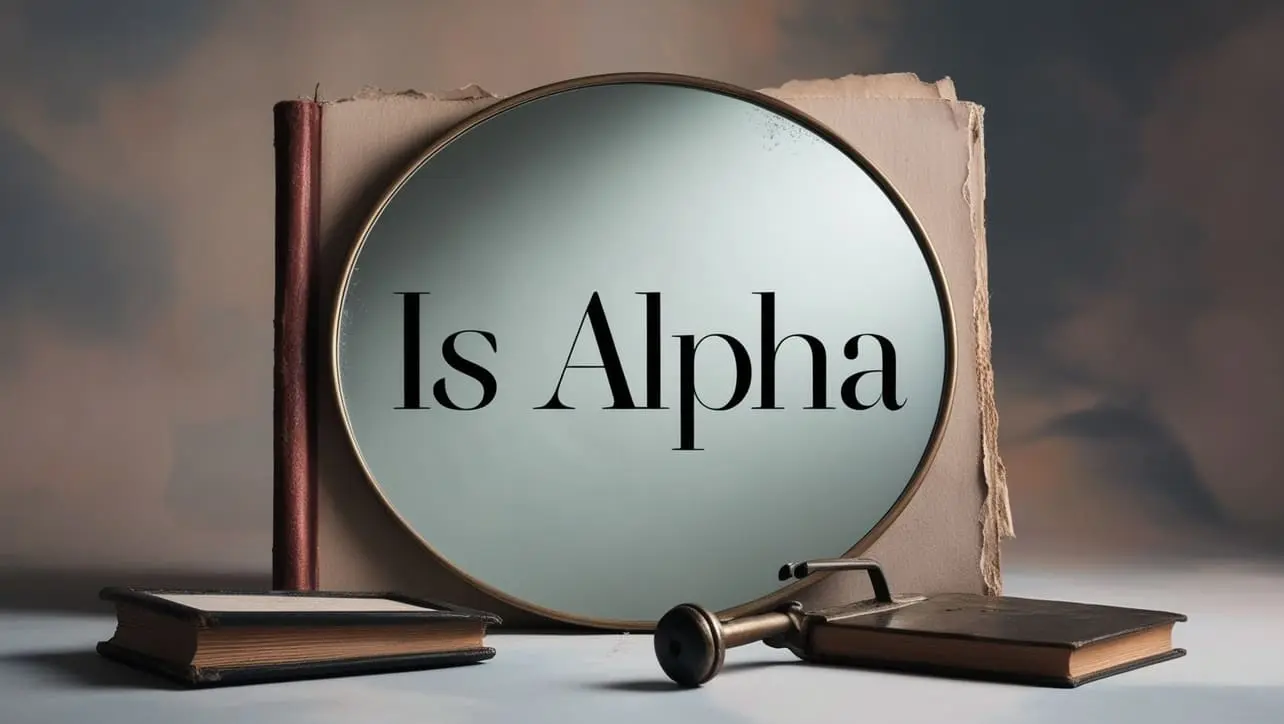
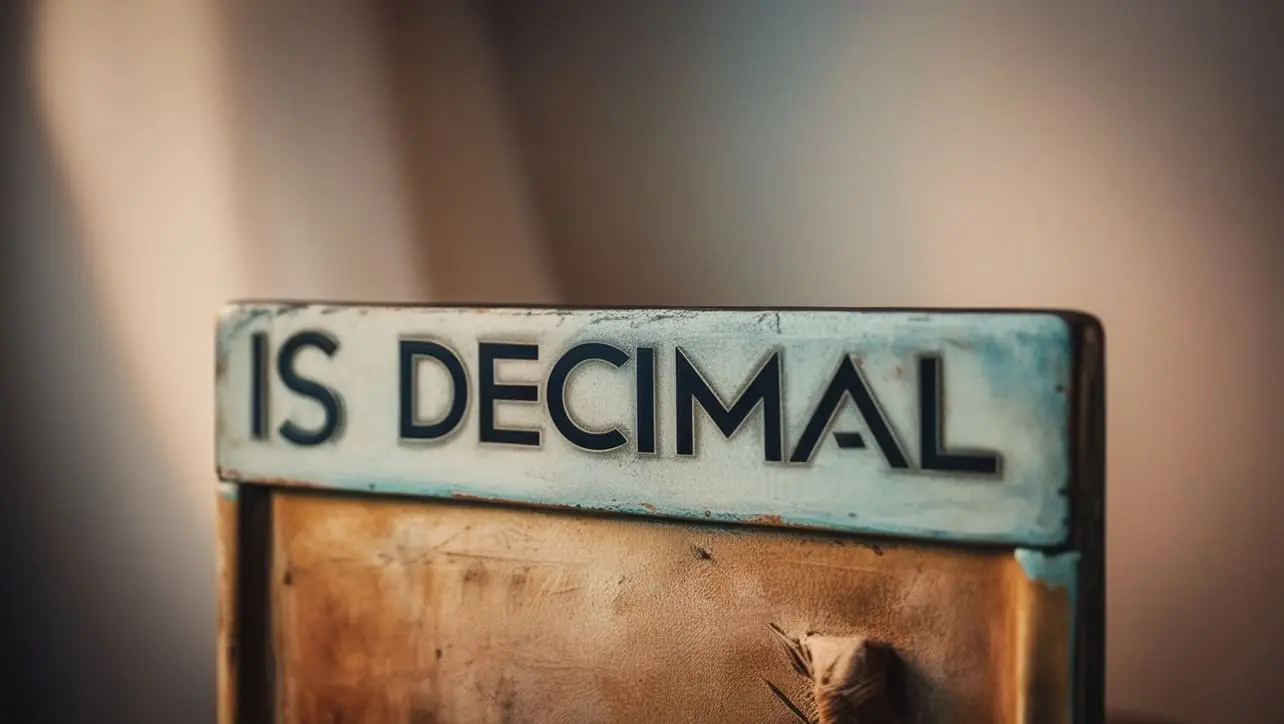
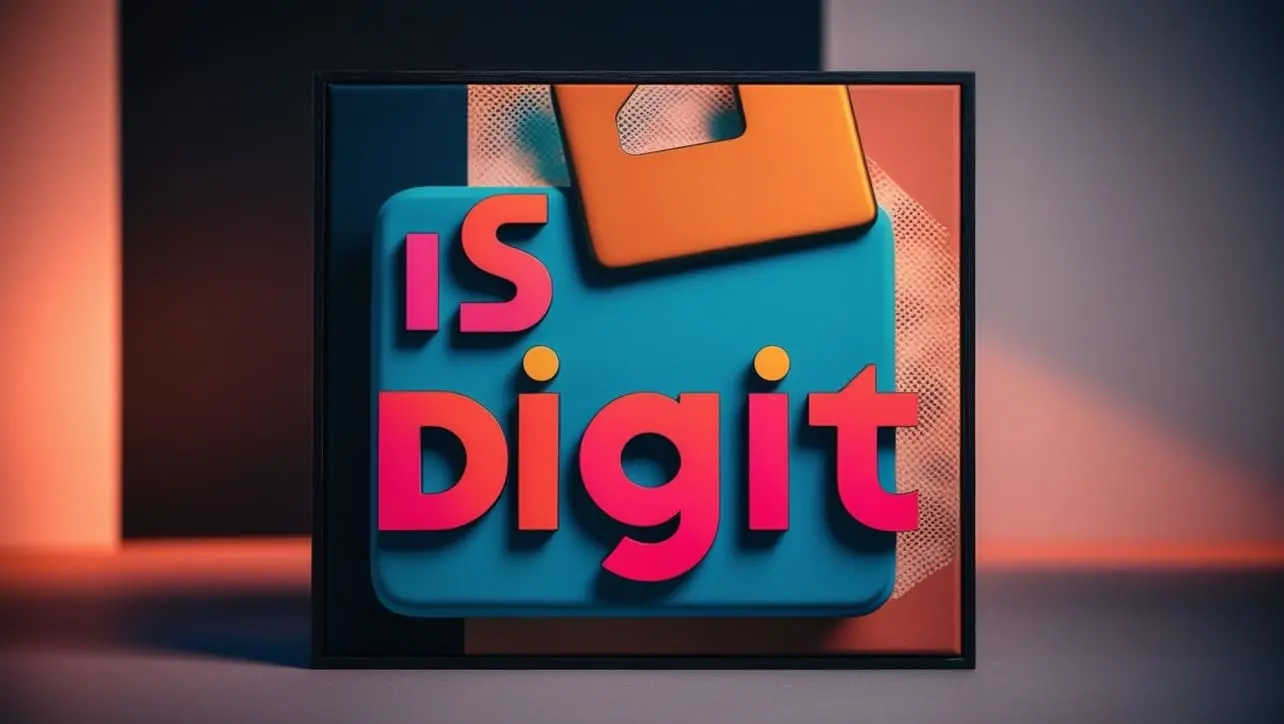
If you have any doubts regarding this article (Python Program to Display Multiplication Table), please comment here. I will help you immediately.