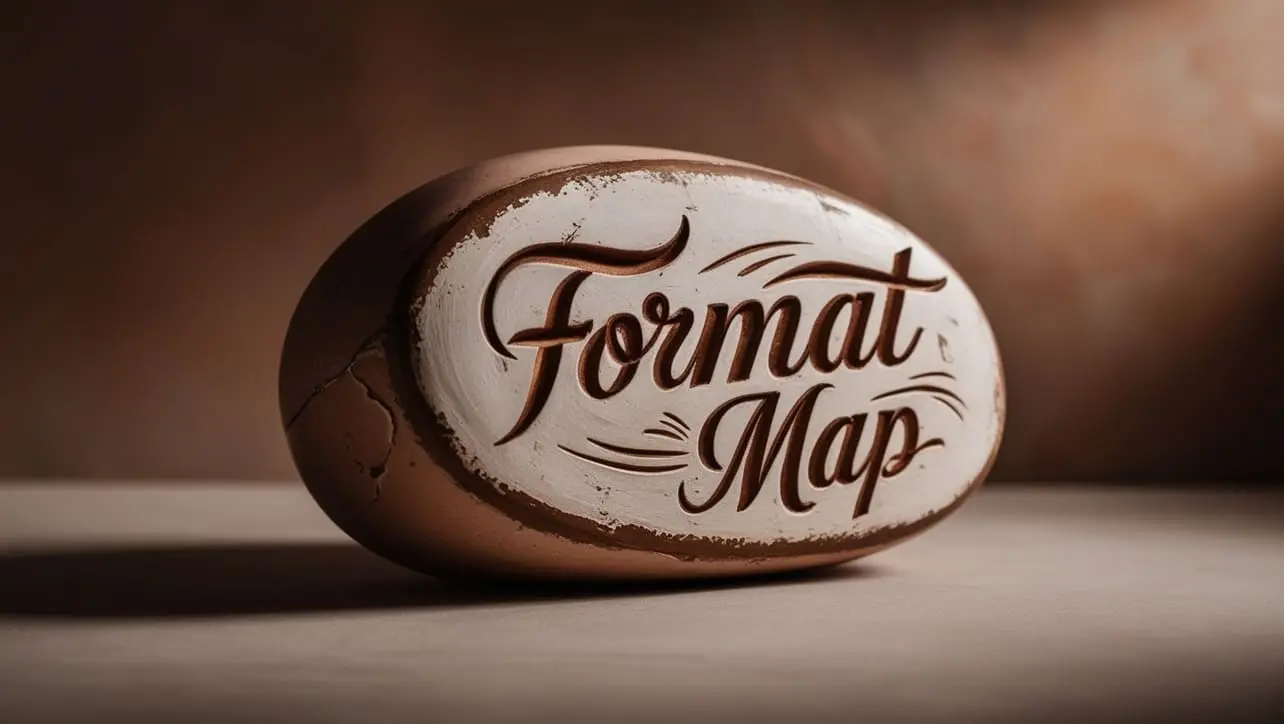
Python Topics
- Python Intro
- Python String Methods
- Python Interview Programs
- Abundant Number
- Amicable Number
- Armstrong Number
- Average of N Numbers
- Automorphic Number
- Biggest of three numbers
- Binary to Decimal
- Common Divisors
- Composite Number
- Condense a Number
- Cube Number
- Decimal to Binary
- Decimal to Octal
- Disarium Number
- Even Number
- Evil Number
- Factorial of a Number
- Fibonacci Series
- GCD
- Happy Number
- Harshad Number
- LCM
- Leap Year
- Magic Number
- Matrix Addition
- Matrix Division
- Matrix Multiplication
- Matrix Subtraction
- Matrix Transpose
- Maximum Value of an Array
- Minimum Value of an Array
- Multiplication Table
- Natural Number
- Number Combination
- Odd Number
- Palindrome Number
- Pascalβs Triangle
- Perfect Number
- Perfect Square
- Power of 2
- Power of 3
- Pronic Number
- Prime Factor
- Prime Number
- Smith Number
- Strong Number
- Sum of Array
- Sum of Digits
- Swap Two Numbers
- Triangular Number
- Python Star Pattern
- Python Number Pattern
- Python Alphabet Pattern
Python Program to find Minimum Value of an Array
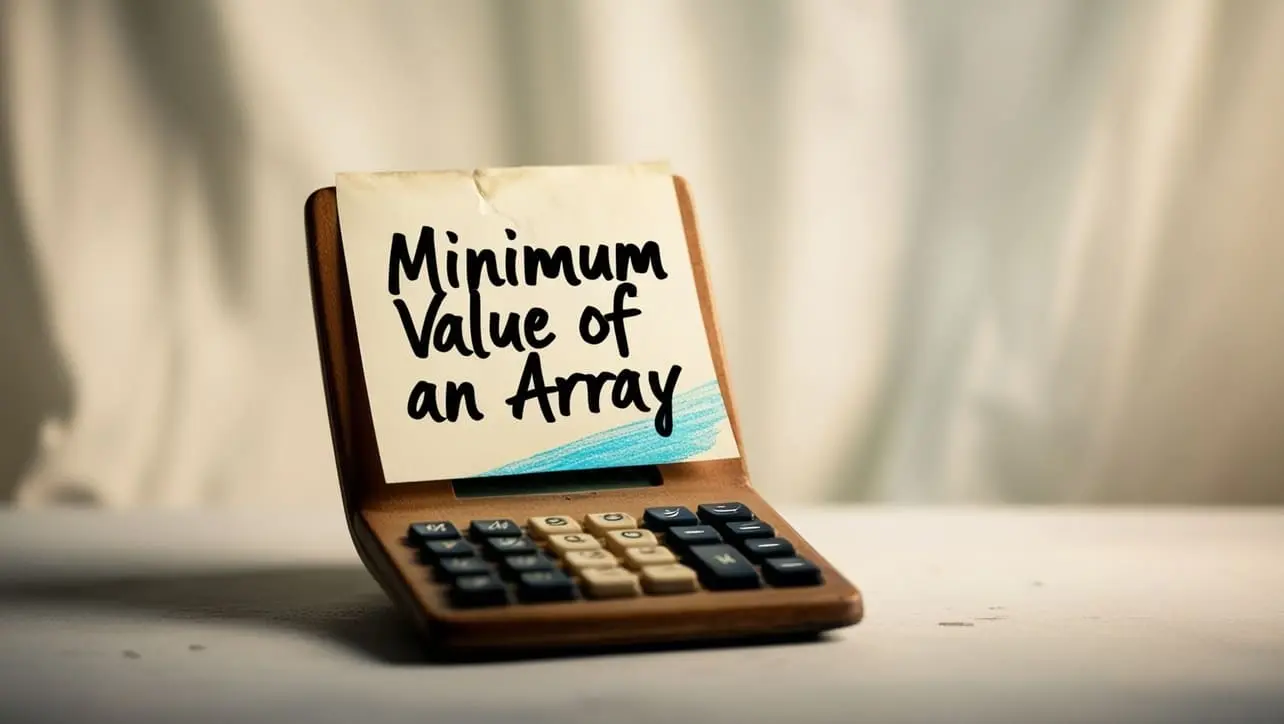
Photo Credit to CodeToFun
Introduction
In the world of programming, manipulating arrays is a fundamental skill. One common task is finding the minimum value within an array.
This involves iterating through the array elements and identifying the smallest value present.
In this tutorial, we will walk through a python program that efficiently finds the minimum value of an array..
Example
Let's dive into the python code to achieve this functionality.
# Function to find the minimum value in an array
def find_min_value(arr):
# Assume the first element is the minimum
min_value = arr[0]
# Iterate through the array to find the minimum value
for element in arr[1:]:
if element < min_value:
min_value = element
return min_value
# Driver program
if __name__ == "__main__":
# Replace these values with your array
array = [12, 5, 7, 3, 2, 8, 10]
# Call the function to find the minimum value
min_value = find_min_value(array)
# Print the result
print(f"The minimum value in the array is: {min_value}")
Testing the Program
To test the program with a different array, simply replace the values in the array declaration in the main function.
The minimum value in the array is: 2
Run the program to see the minimum value of the array.
How the Program Works
- The program defines a function find_min_value that takes an array as input and returns the minimum value.
- Inside the function, it assumes the first element of the array is the minimum value.
- It then iterates through the array, updating the minimum value whenever a smaller element is encountered.
- The driver program initializes an array, calls the function, and prints the result.
Understanding the Concept of Minimum Value of an Array
Before diving into the code, let's understand the concept of finding the minimum value in an array.
The minimum value is the smallest element present in the array. The algorithm iterates through the array elements, updating the minimum value whenever a smaller element is encountered.
Optimizing the Program
While the provided program is effective, consider exploring and implementing optimizations, such as using additional data structures or algorithms, depending on the specific requirements and constraints of your application.
Feel free to incorporate and modify this code as needed for your specific use case. Happy coding!
Join our Community:
Author
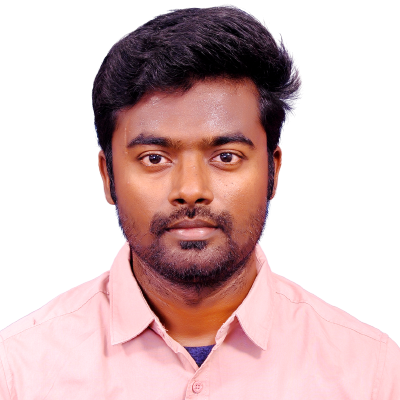
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a CoffeeShare Your Findings to All
Recent Post in Python
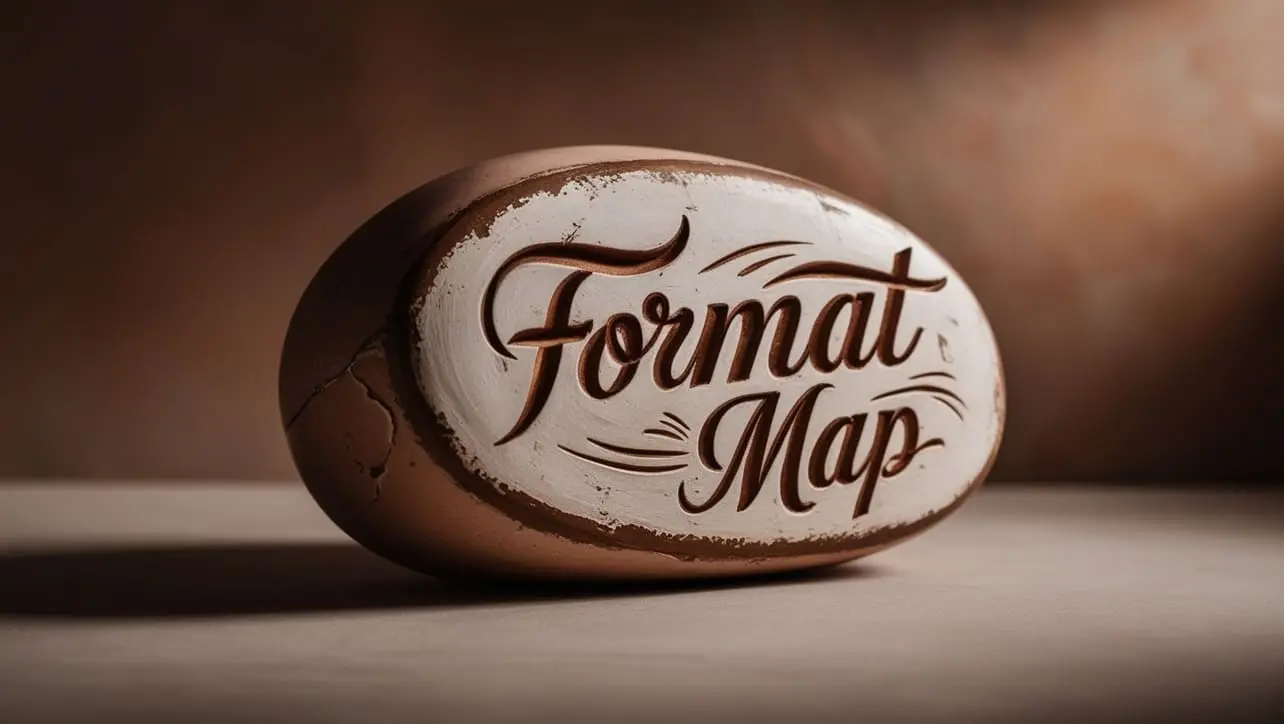
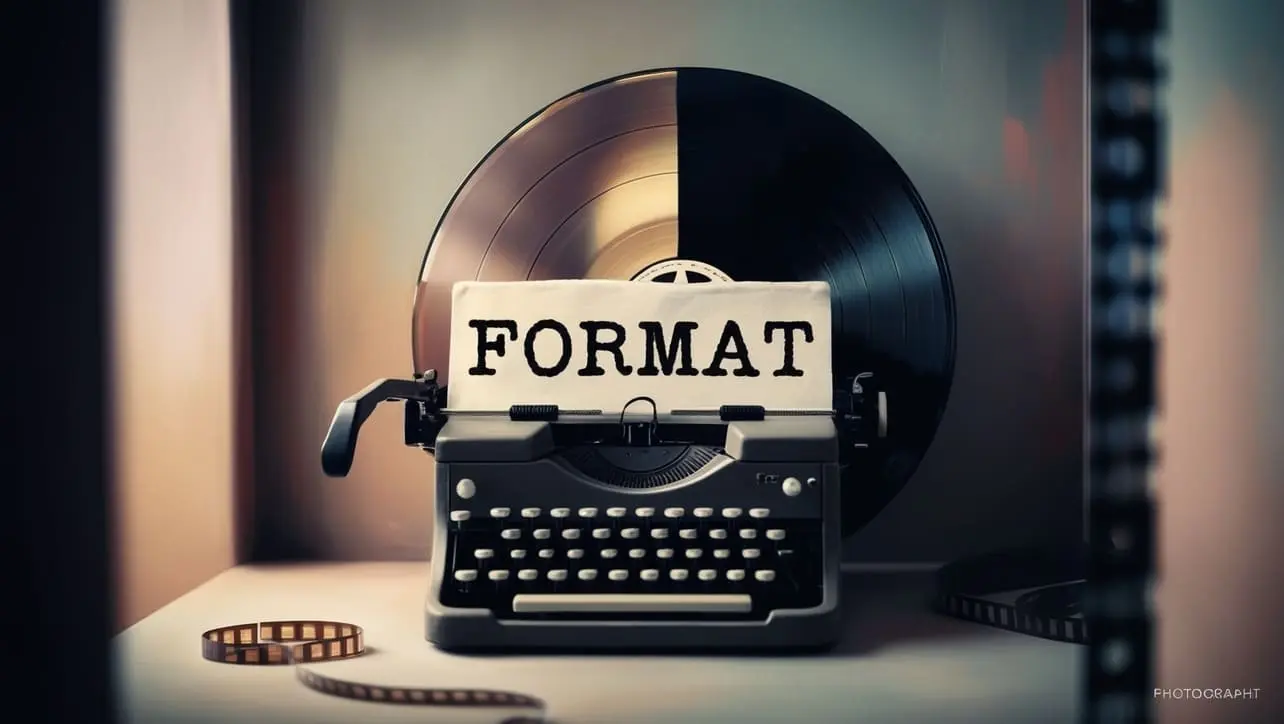
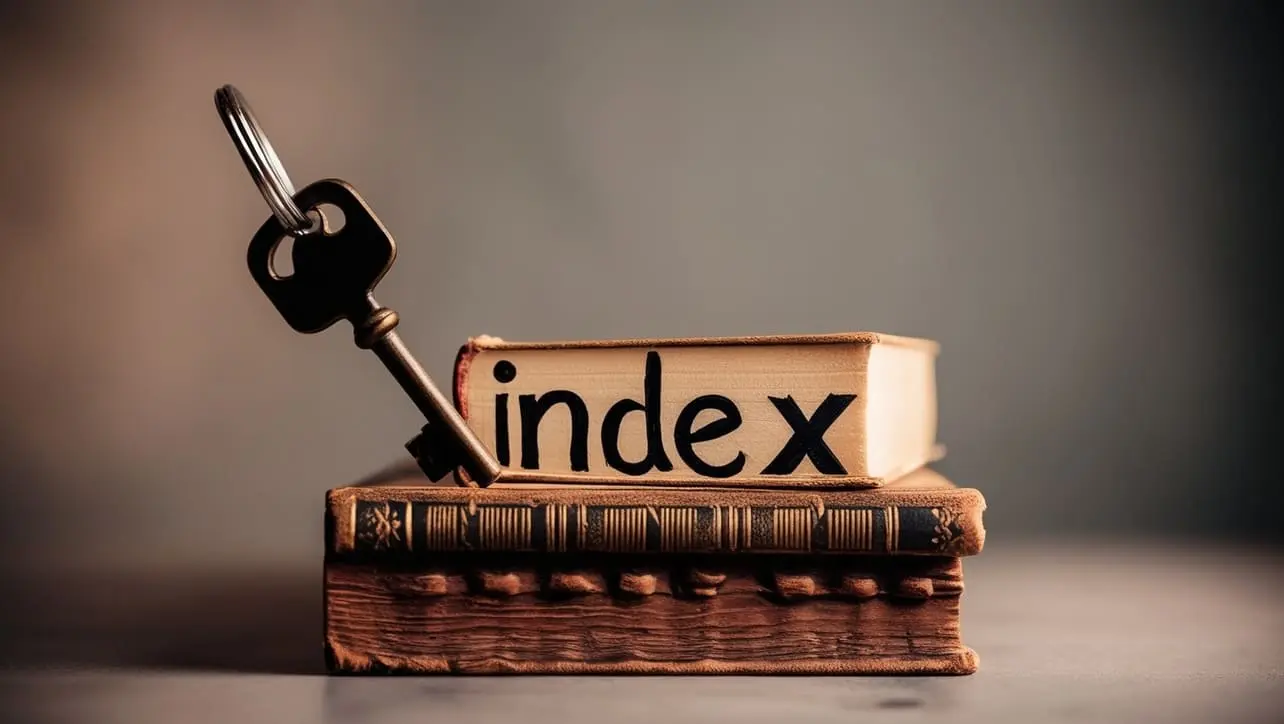
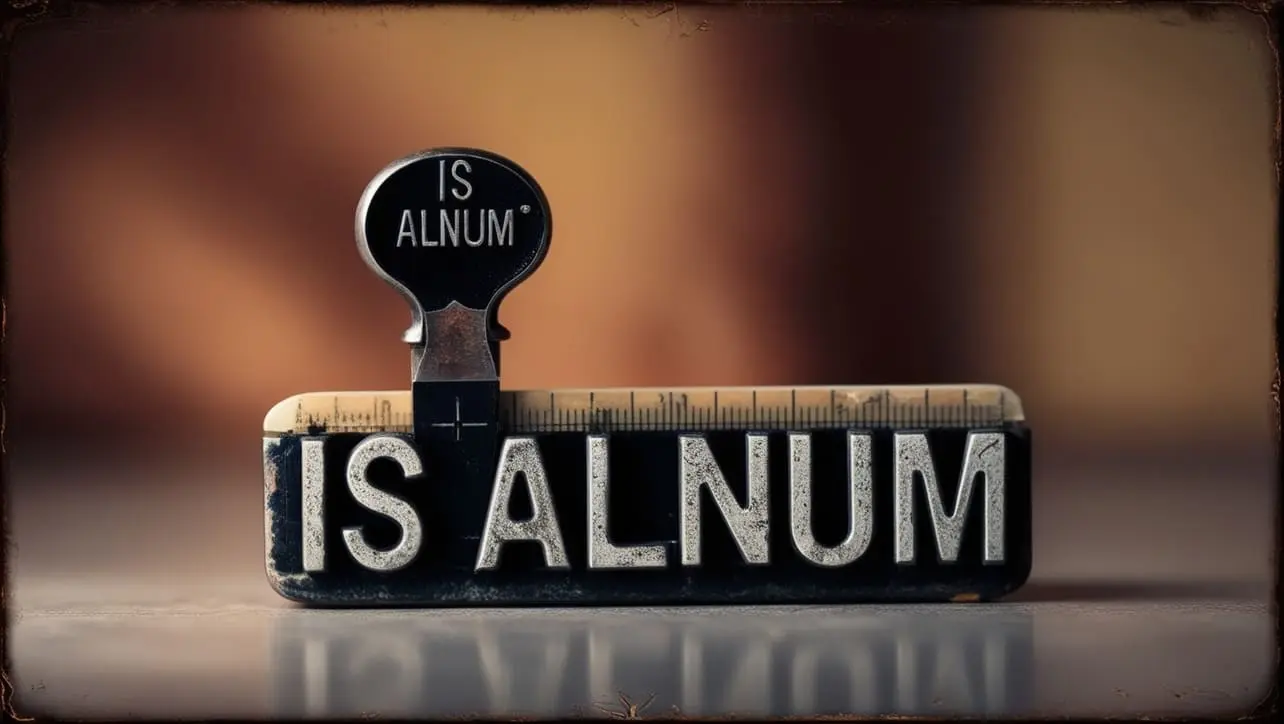
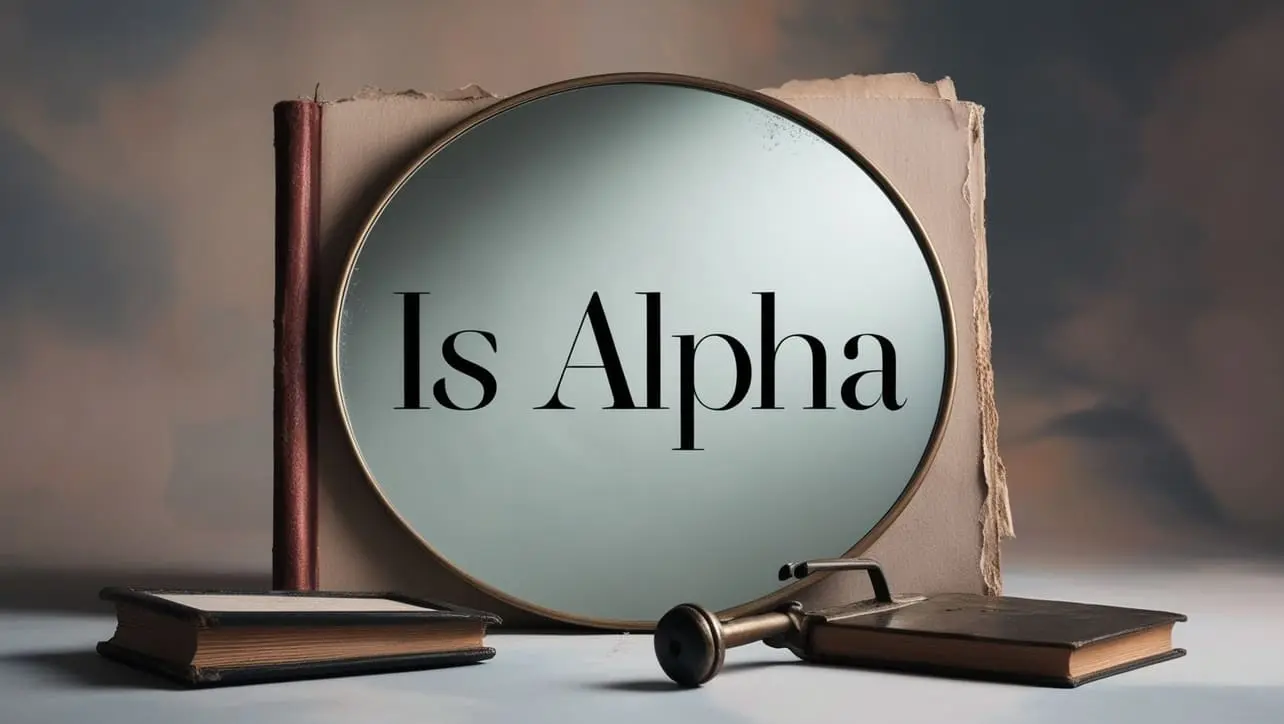
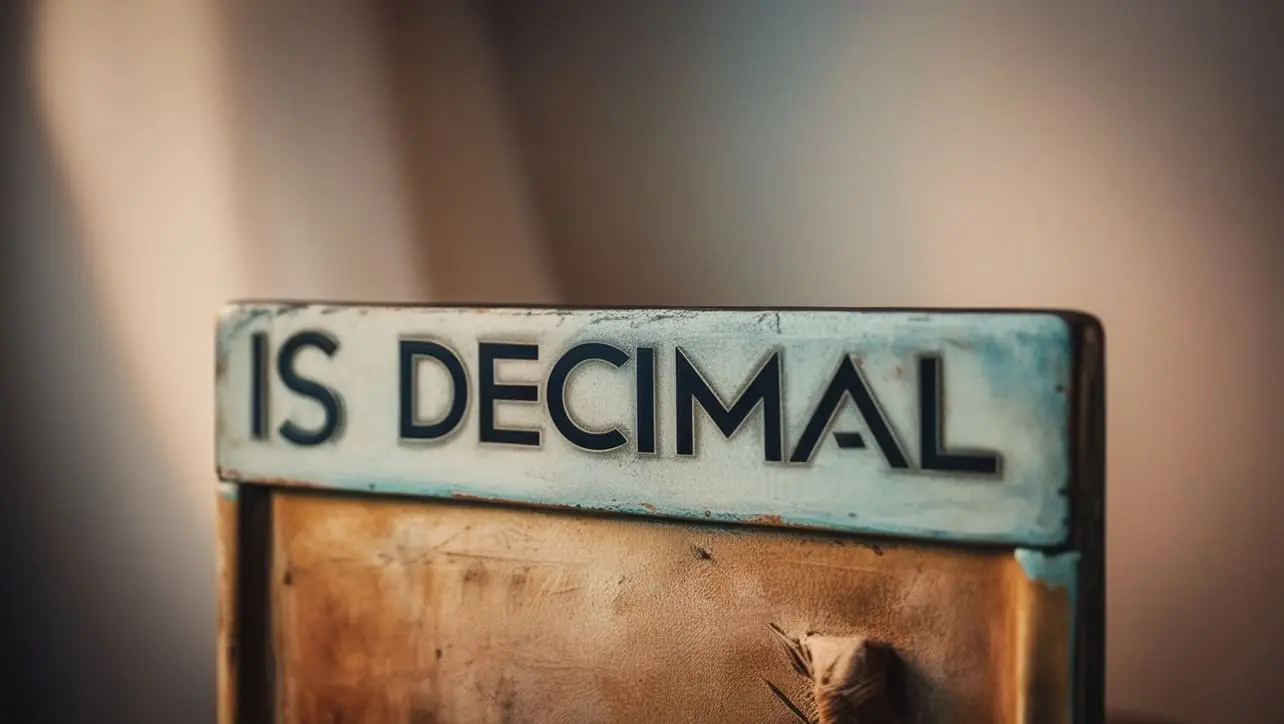
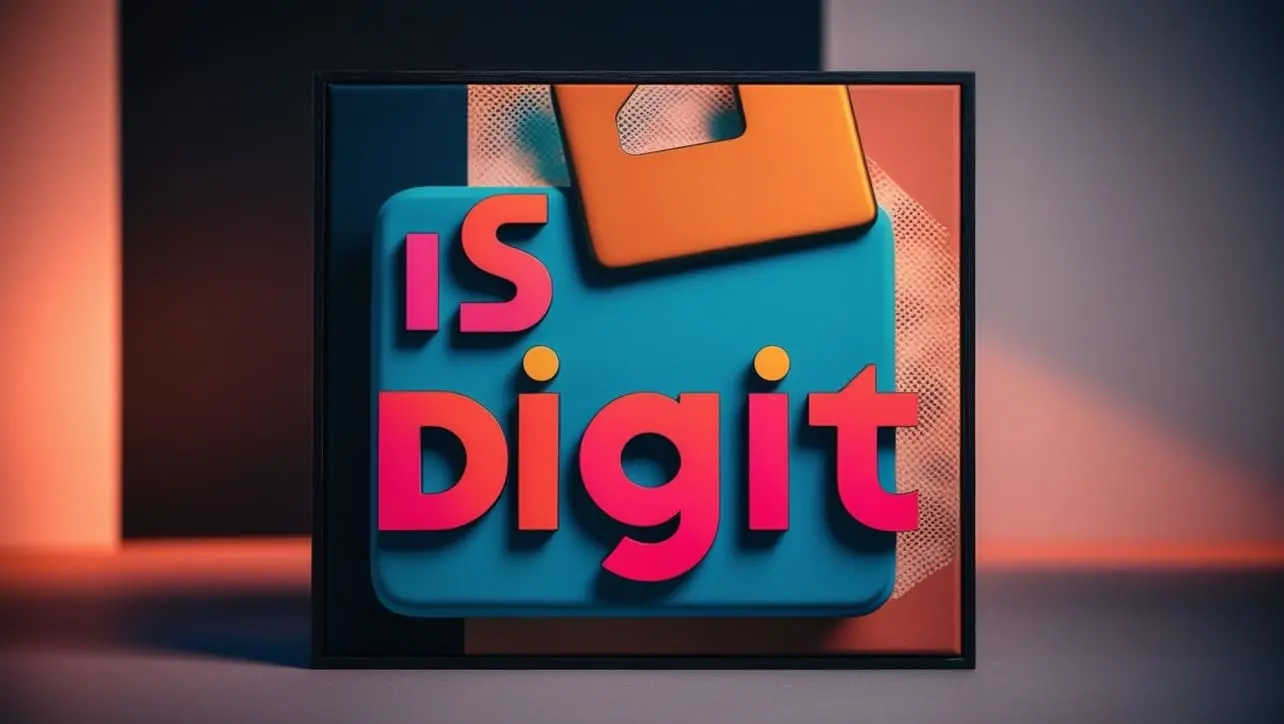
If you have any doubts regarding this article (Python Program to find Minimum Value of an Array), please comment here. I will help you immediately.