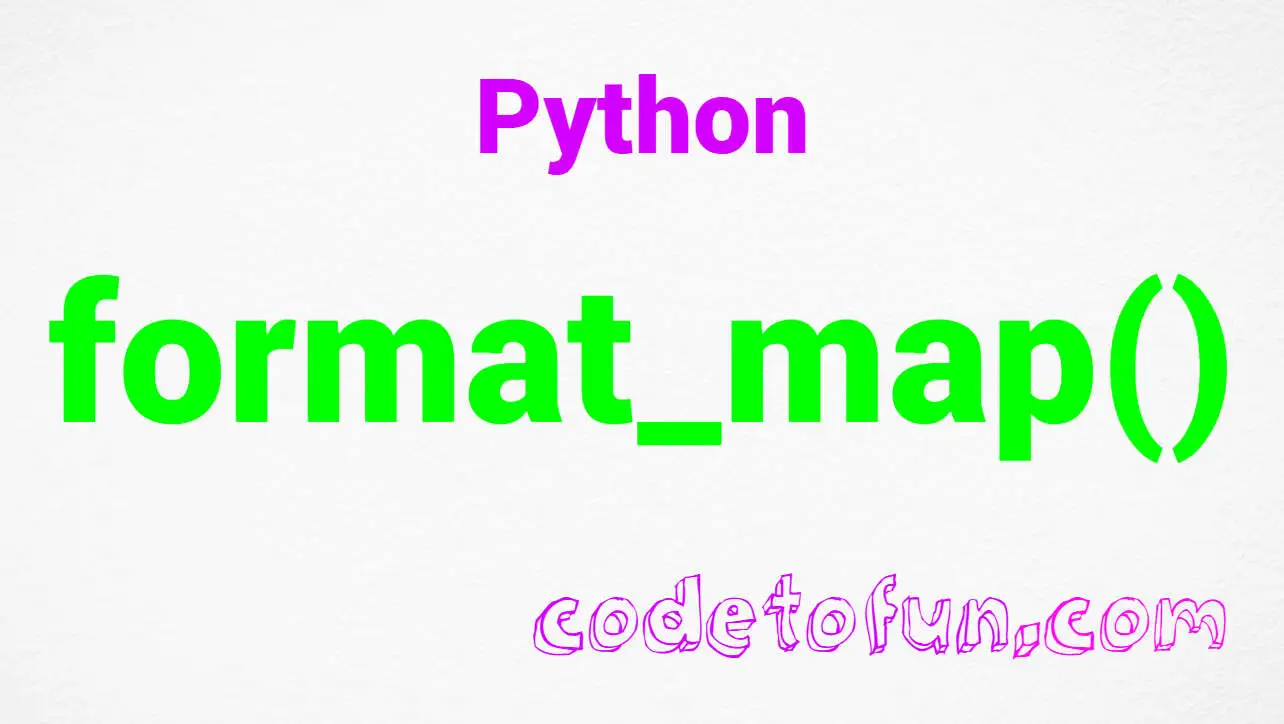
Python Basic
Python Interview Programs
- Python Interview Programs
- Python Abundant Number
- Python Amicable Number
- Python Armstrong Number
- Python Average of N Numbers
- Python Automorphic Number
- Python Biggest of three numbers
- Python Binary to Decimal
- Python Common Divisors
- Python Composite Number
- Python Condense a Number
- Python Cube Number
- Python Decimal to Binary
- Python Decimal to Octal
- Python Disarium Number
- Python Even Number
- Python Evil Number
- Python Factorial of a Number
- Python Fibonacci Series
- Python GCD
- Python Happy Number
- Python Harshad Number
- Python LCM
- Python Leap Year
- Python Magic Number
- Python Matrix Addition
- Python Matrix Division
- Python Matrix Multiplication
- Python Matrix Subtraction
- Python Matrix Transpose
- Python Maximum Value of an Array
- Python Minimum Value of an Array
- Python Multiplication Table
- Python Natural Number
- Python Number Combination
- Python Odd Number
- Python Palindrome Number
- Python Pascalβs Triangle
- Python Perfect Number
- Python Perfect Square
- Python Power of 2
- Python Power of 3
- Python Pronic Number
- Python Prime Factor
- Python Prime Number
- Python Smith Number
- Python Strong Number
- Python Sum of Array
- Python Sum of Digits
- Python Swap Two Numbers
- Python Triangular Number
Python Program to Check Disarium Number
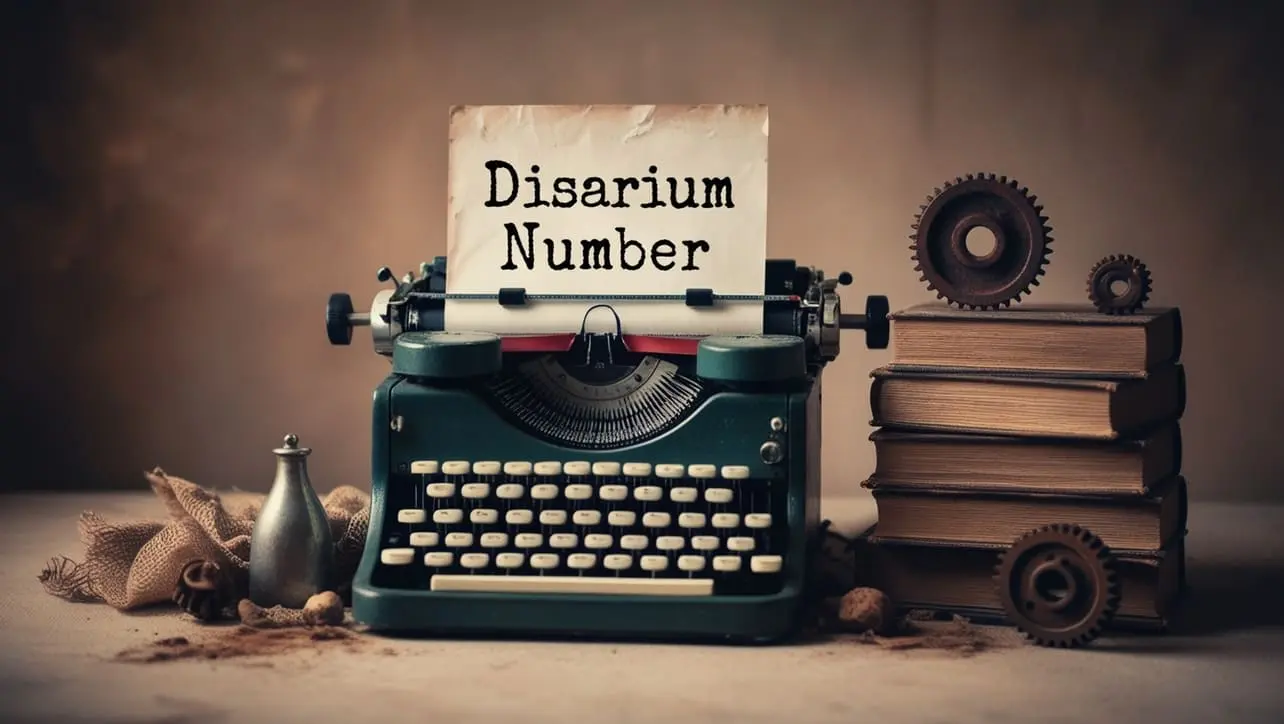
Photo Credit to CodeToFun
π Introduction
In the realm of programming, exploring mathematical patterns is a fascinating endeavor. One such numeric pattern is the Disarium number.
A Disarium number is a number defined by the sum of its digits each raised to the power of its respective position.
In this tutorial, we'll delve into a Python program designed to check whether a given number is a Disarium number.
π Example
Let's explore the Python code that checks whether a given number is a Disarium number.
def count_digits(number):
count = 0
while number != 0:
count += 1
number //= 10
return count
def is_disarium(number):
original_number = number
digit_count = count_digits(number)
total_sum = 0
while number != 0:
digit = number % 10
total_sum += digit ** digit_count
digit_count -= 1
number //= 10
return total_sum == original_number
# Replace this value with your desired number
input_number = 89
# Check if the number is a Disarium number
if is_disarium(input_number):
print(f"{input_number} is a Disarium number.")
else:
print(f"{input_number} is not a Disarium number.")
π» Testing the Program
To test the program with different numbers, replace the value of input_number in the code.
89 is a Disarium number.
Run the script to check if the number is a Disarium number.
π§ How the Program Works
- The program defines a function count_digits to count the number of digits in a given number.
- The is_disarium function checks if a number is a Disarium number by summing the digits each raised to the power of its position.
- The program tests the is_disarium function with a sample number (replace it with your desired number).
π Between the Given Range
Let's take a look at the Python code that checks for Disarium numbers in the range from 1 to 100.
def is_disarium(num):
num_str = str(num)
length = len(num_str)
total = sum(int(digit) ** (index + 1) for index, digit in enumerate(num_str))
return total == num
# Display Disarium numbers in the range 1 to 100
disarium_numbers = [num for num in range(1, 101) if is_disarium(num)]
print("Disarium numbers in the range 1 to 100:")
print(" ".join(map(str, disarium_numbers)))
π» Testing the Program
Disarium numbers in the range 1 to 100: 1 2 3 4 5 6 7 8 9 89
Run the script to see the Disarium numbers in the range 1 to 100.
π§ How the Program Works
- The program defines a function is_disarium that takes a number and checks if it is a Disarium number based on the specified criteria.
- Inside the function, it converts the number to a string to extract individual digits.
- It calculates the sum of each digit raised to the power of its respective position.
- The main section generates a list of Disarium numbers in the range from 1 to 100 and prints them in the specified format.
π§ Understanding the Concept of Disarium Numbers
Before we dive into the code, let's grasp the concept of Disarium numbers.
A Disarium number is a number such that the sum of its digits, each raised to the power of its position, equals the number itself.
For example, the number 89 is a Disarium number because 8^1 + 9^2 equals 89.
π Conclusion
Understanding and implementing programs to identify numeric patterns, such as Disarium numbers, is a valuable skill in the world of programming.
The provided python program offers a practical example of checking whether a given number follows the Disarium pattern.
Feel free to use and modify this code for your specific use cases. Happy coding!
π¨βπ» Join our Community:
Author
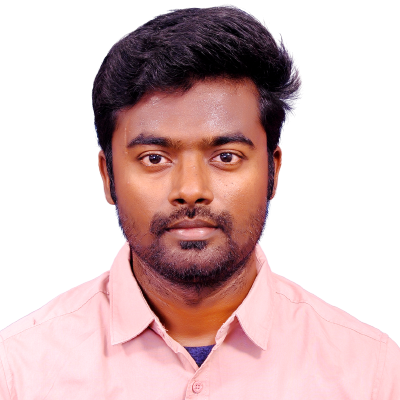
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (Python Program to Check Disarium Number), please comment here. I will help you immediately.