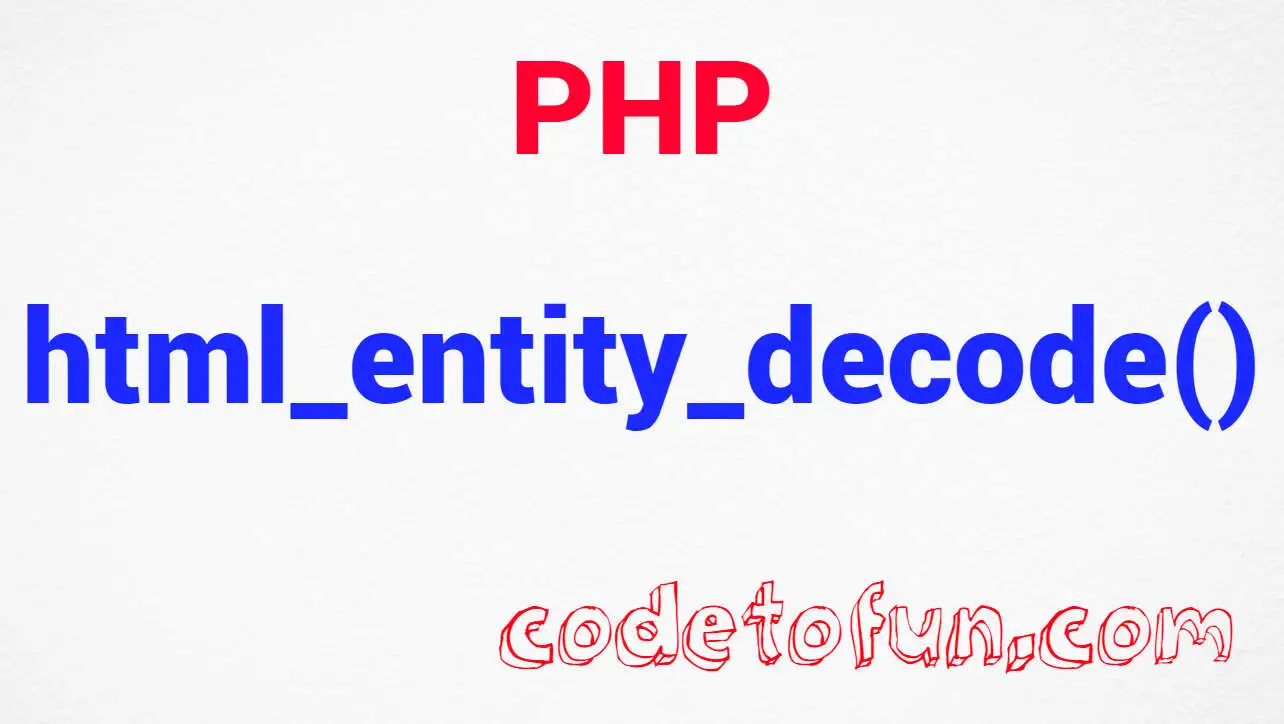
PHP Basic
- PHP Intro
- PHP Star Pattern
- PHP Number Pattern
- PHP Alphabet Pattern
- PHP String Functions
- PHP addcslashes()
- PHP addslashes()
- PHP bin2hex()
- PHP chop()
- PHP chr()
- PHP chunk_split()
- PHP convert_cyr_string()
- PHP convert_uudecode()
- PHP convert_uuencode()
- PHP count_chars()
- PHP crc32()
- PHP crypt()
- PHP explode()
- PHP fprintf()
- PHP get_html_translation_table()
- PHP hebrev()
- PHP hebrevc()
- PHP hex2bin()
- PHP html_entity_decode()
- PHP htmlentities()
- PHP htmlspecialchars_decode()
- PHP htmlspecialchars()
- PHP implode()
- PHP join()
PHP String html_entity_decode() Function
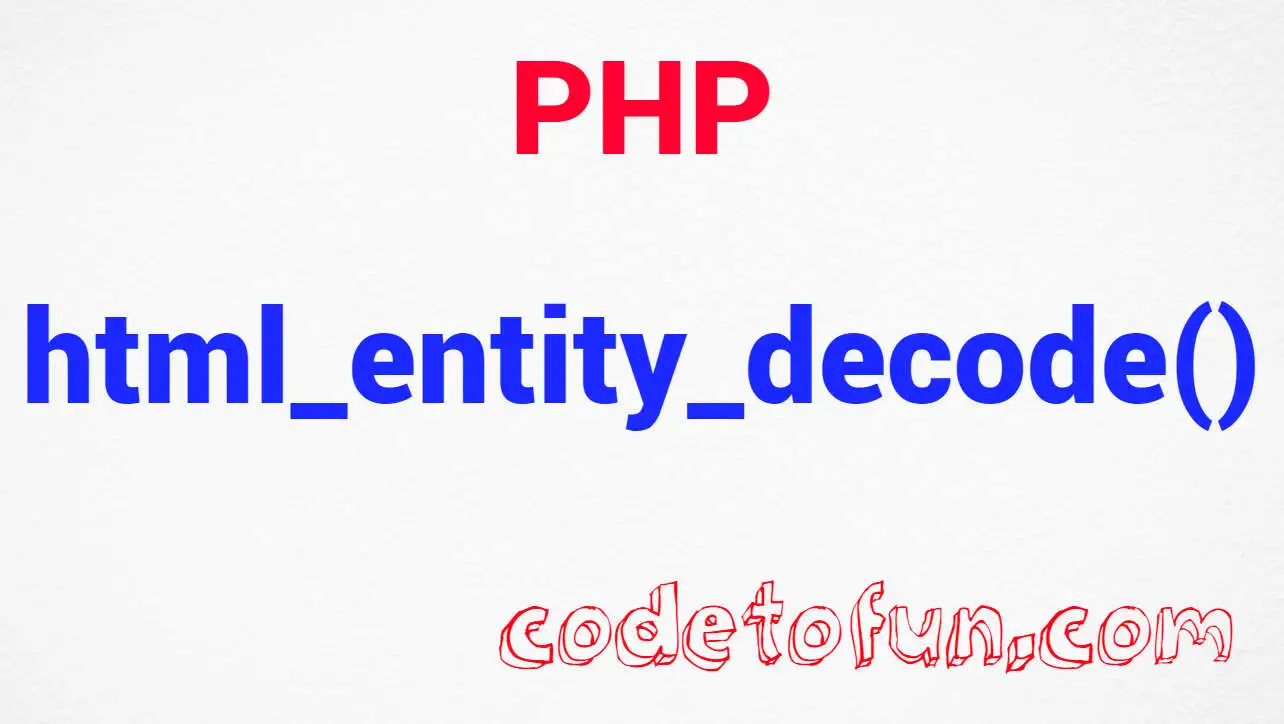
Photo Credit to CodeToFun
đ Introduction
In PHP programming, dealing with HTML entities is a common task, especially when working with user-generated content or parsing HTML.
The html_entity_decode()
function is a built-in PHP function that helps in converting HTML entities back to their corresponding characters.
In this tutorial, we'll explore the usage and functionality of the html_entity_decode()
function.
đĄ Syntax
The signature of the html_entity_decode()
function is as follows:
string html_entity_decode ( string $string , int $flags = ENT_COMPAT | ENT_HTML401 , string|null $encoding = ini_get("default_charset") )
- $string: The string to decode.
- $flags (optional): A bitmask of one or more of the following flags:
- ENT_COMPAT: Default. Will convert double-quotes and leave single-quotes alone.
- ENT_QUOTES: Will convert both double and single quotes.
- ENT_NOQUOTES: Will leave both double and single quotes unconverted.
- ENT_HTML401: Default. Handle code as HTML 4.01.
- ENT_XML1: Handle code as XML 1.
- ENT_XHTML: Handle code as XHTML.
- ENT_HTML5: Handle code as HTML 5.
- $encoding (optional): The encoding to use. If not provided, the default_charset configuration option will be used.
đ Example
Let's delve into an example to illustrate how the html_entity_decode()
function works.
<?php
$htmlString = "This is an <b>example</b> string.";
// Decode HTML entities
$decodedString = html_entity_decode($htmlString);
// Output the result
echo "Original String: $htmlString\n";
echo "Decoded String: $decodedString\n";
?>
đģ Output
Original String: This is an <b>example</b> string. Decoded String: This is an <b>example</b> string.
đ§ How the Program Works
In this example, the html_entity_decode()
function is used to decode HTML entities in a string and then prints the original and decoded strings.
âŠī¸ Return Value
The html_entity_decode()
function returns the decoded string.
đ Common Use Cases
The html_entity_decode()
function is particularly useful when you need to display or manipulate HTML content in a human-readable format. It's commonly used when working with user input in web applications.
đ Notes
- If you're working with XHTML, it's recommended to use the ENT_XHTML flag to ensure proper decoding.
- Make sure to handle character encoding appropriately based on your application requirements.
đĸ Optimization
The html_entity_decode()
function is optimized for performance. However, consider the use of appropriate flags and encoding options based on your specific needs.
đ Conclusion
The html_entity_decode()
function in PHP is a valuable tool for handling HTML entities and converting them back to their original characters. It provides a convenient way to work with HTML content in a human-readable format.
Feel free to experiment with different strings and explore the behavior of the html_entity_decode()
function in various scenarios. Happy coding!
đ¨âđģ Join our Community:
Author
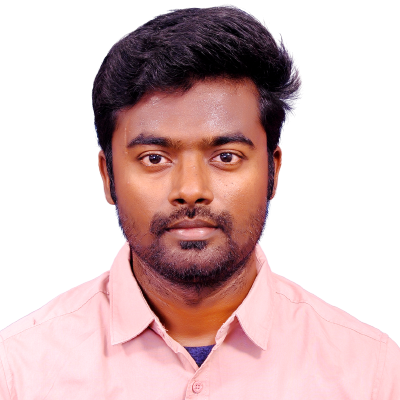
For over eight years, I worked as a full-stack web developer. Now, I have chosen my profession as a full-time blogger at codetofun.com.
Buy me a coffee to make codetofun.com free for everyone.
Buy me a Coffee
If you have any doubts regarding this article (PHP String html_entity_decode() Function), please comment here. I will help you immediately.